No Need For Nodemon: Native File Watching in Node.js
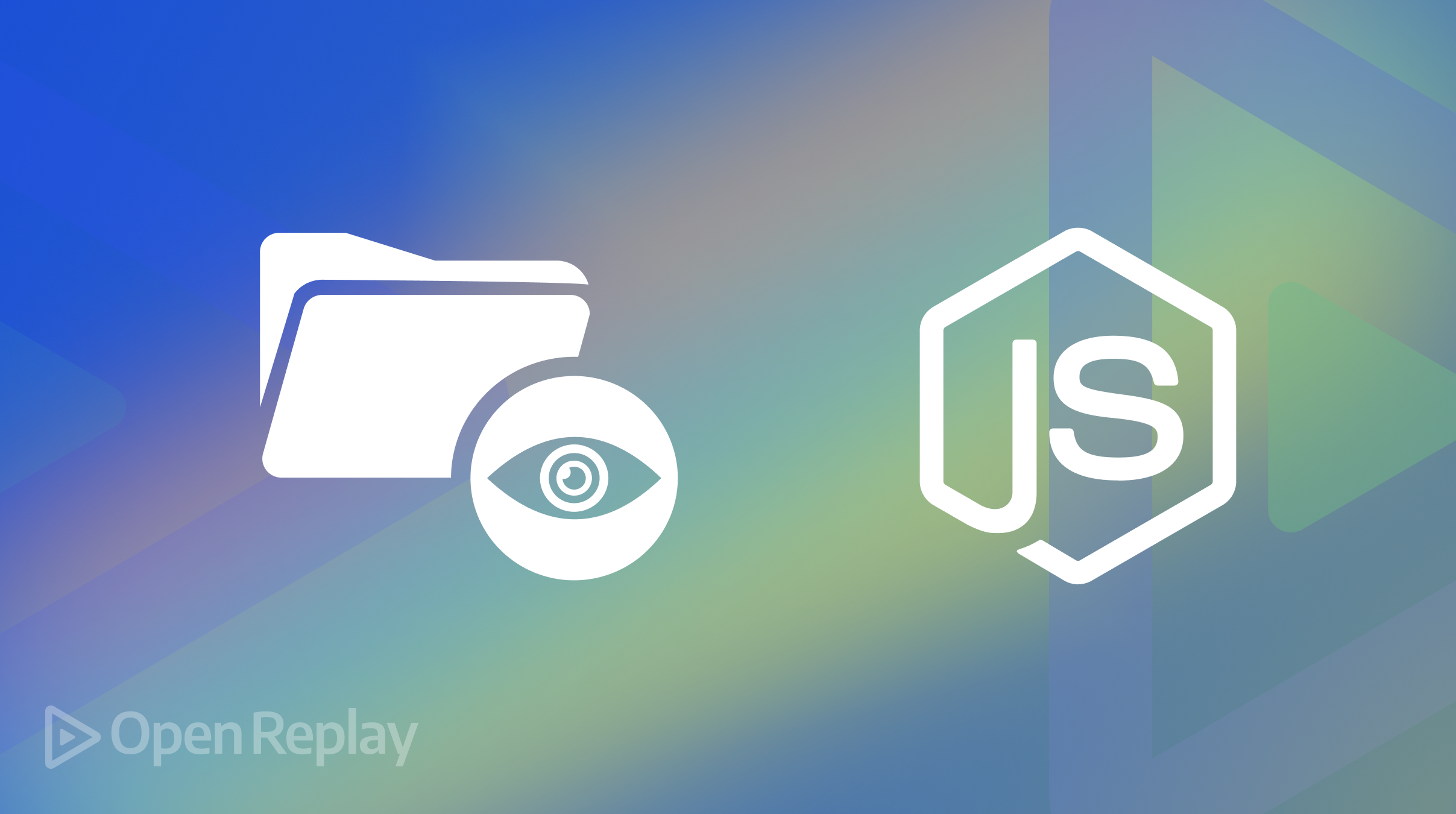
Modern JavaScript development is all about efficiency. We constantly seek ways to optimize our workflows. One common solution has been Nodemon, a tool that automatically restarts our applications upon file changes. However, with features like the native file watcher in Node.js, we now have a built-in alternative that eliminates the need for external tools and dependencies, keeping your development environment clean and efficient, as this article will explain.
Discover how at OpenReplay.com.
Native file watching offers several benefits that streamline development processes and project management. These are some reasons why you should embrace the native file watcher in Node.js applications:
-
Improved Development Workflow: By utilizing the native watcher, you can automate the process of monitoring file changes and restarting your Node.js application, leading to a more efficient and productive development workflow.
-
Reduced Dependencies: By leveraging native file watching, you minimize the number of external dependencies in your projects. This reduces the complexity of the project’s dependency tree, making it easier to manage and maintain in the long run.
-
Future-Proofing: Native file-watching features ensure that projects remain compatible with future versions of Node.js. While third-party tools may introduce breaking changes or become outdated over time, the Node.js ecosystem maintains and updates the native file watcher, providing a more sustainable solution in the long term.
-
Enhanced Performance: Leveraging native features can often result in better performance than third-party solutions, ensuring that your Node.js applications run smoothly and respond quickly to changes.
Overview of the Native File Watcher
Native file watcher is a built-in Node.js feature that enables efficient monitoring of file and directory changes within our applications. To leverage this functionality, use the node—-watch
command-line flag.
This feature was first introduced in Node.js version 18.11.0 as an experimental feature, and it has undergone significant improvements in subsequent releases.
Notably, the watch mode for the Node.js test runner was introduced in versions 18.13.0 and 19.2.0. This feature supports running tests in watch mode, which helps monitor changes in test files. (more on this later)
Despite still being labeled as experimental, the native file watcher remains a valuable feature in the latest versions of Node.js. It offers a reliable and efficient method for streamlining development workflows by automating file changes monitoring.
Advantages and Disadvantages of the Native File Watcher
Let’s explore some of the advantages and disadvantages of using the native file watcher in Node.js:
Advantages
The advantages of native file watcher include:
-
Simplicity: The
--watch
flag is a built-in feature, making it straightforward to implement. You do not need any additional libraries or complex setups. -
Efficiency: Native file watching leverages the operating system’s capabilities for monitoring file changes, potentially leading to efficient resource utilization.
Disadvantages
The disadvantages of native file watcher include:
-
Limited Functionality: The
--watch
flag offers basic file-watching functionality, like monitoring files and directories. Nodemon, on the other hand, provides a more comprehensive solution with features like automatic and manual restarting, file extension detection, and configuration options. -
Cross-Platform Limitations: Certain file-watching commands like the
--watch-path
command are only available on specific operating systems like Windows and macOS. (more on this later) -
Scalability Limitations: The native approach might not be optimal for large-scale applications with numerous files to monitor.
Implementing Native File Watcher in Node.js
Before you get started, ensure that you are running at least Node.js version 18.11.0 or higher. You can check your Node.js version by running the node -v
command in your terminal.
To implement native file watching, follow these basic steps:
First, open a terminal and navigate to the directory where your Node.js project is located.
Then, determine the file or directory you want to watch for changes.
Finally, use the node --watch
command and the path to the file you want to keep in watch mode. For example, to watch changes in a file named index.js
, you can run the following command:
node --watch index.js
To stop file-watching, you can terminate the Node.js process running in your terminal by pressing Ctrl + C
or Cmd + C
on your keyboard.
Using Watch Mode with Node.js Test Runner
When using the Node.js test runner from the command line interface (CLI), you can enable watch mode to automatically monitor changes in test files. To activate watch mode, use the following command:
node --test --watch
This command instructs the Node.js test runner to enter watch mode, allowing it to detect changes in test files and their dependencies. It will automatically rerun affected tests whenever a change is detected.
Watching Changes in Directories
Node.js offers a convenient method for monitoring a specific directory and automatically rerunning a file when a change occurs within that directory.
This functionality can be achieved using the --watch-path
flag. For example, consider a scenario where you have a directory named src
, and you aim to watch for changes in this directory and trigger the execution of a file named index.js
when any change occurs. In such a case, you can utilize the following command:
node --watch-path=./src index.js
Now that you’ve learned how to implement the native file watcher in your Node.js project and explored its functionalities, such as watching changes in directories and test files, let’s delve into a use-case scenario where we will apply it to a web server.
Auto-restarting a Web Server with Native File Watching
Consider a scenario where you have a server.js
file containing the code for a simple web server using Express.js:
// Importing required modules
const express = require("express");
// Creating an instance of Express
const app = express();
// Define a route
app.get("/", (req, res) => {
res.send("Hello World");
});
// Set up the server to listen on port 8000
const port = 8000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
With the native file watcher, you can start the server using the following command:
node --watch server.js
Now, whenever you save changes to the server.js
file, the server will automatically restart, reflecting those updates instantly. This feature streamlines the development process by eliminating the need to manually restart the server after making changes, ensuring a seamless and efficient workflow.
Best Practices and Tips for Efficient File Watching in Node.js
Let’s explore some practices and tips for efficient file-watching in Node.js:
Update Your Start Script
To enhance your development workflow, updating the start script in your package.json
file is important. This script serves as the entry point for running your Node.js application.
By configuring the start script to include file watching, you can automate monitoring file changes and restarting your application as needed. This improves convenience and centralizes the file-watching configuration within your project structure, making it easier to manage and maintain in the long run.
To update the start script, open your package.json
file and locate the “scripts” section. Within this section, modify the “start” script to include the --watch
flag and specify the entry point file, e.g., index.js
. Here’s an example of how the updated start script might look:
"scripts": {
"start": "node --watch index.js"
}
After updating the script, you can now start the application with file watching enabled using the following simple command:
npm start
This approach streamlines launching your application by watching files during development.
Documentation and Updates
Stay updated with Node.js documentation and community resources to learn about new features and improvements related to file-watching. Continuous learning ensures that you can make the most of native file-watching, regardless of your level of expertise.
Conclusion
Native file watching in Node.js, facilitated by the --watch
flag, offers us a powerful tool for monitoring file system changes within our applications. By leveraging this built-in feature, you can streamline your development workflow and automate processes in your applications. At the same time, it reduces reliance on external tools and minimizes dependencies, leading to cleaner and more efficient development environments. Overall, native file watching in Node.js empowers us to create applications with ease.
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.