Enhancing Web Performance with Event Delegation
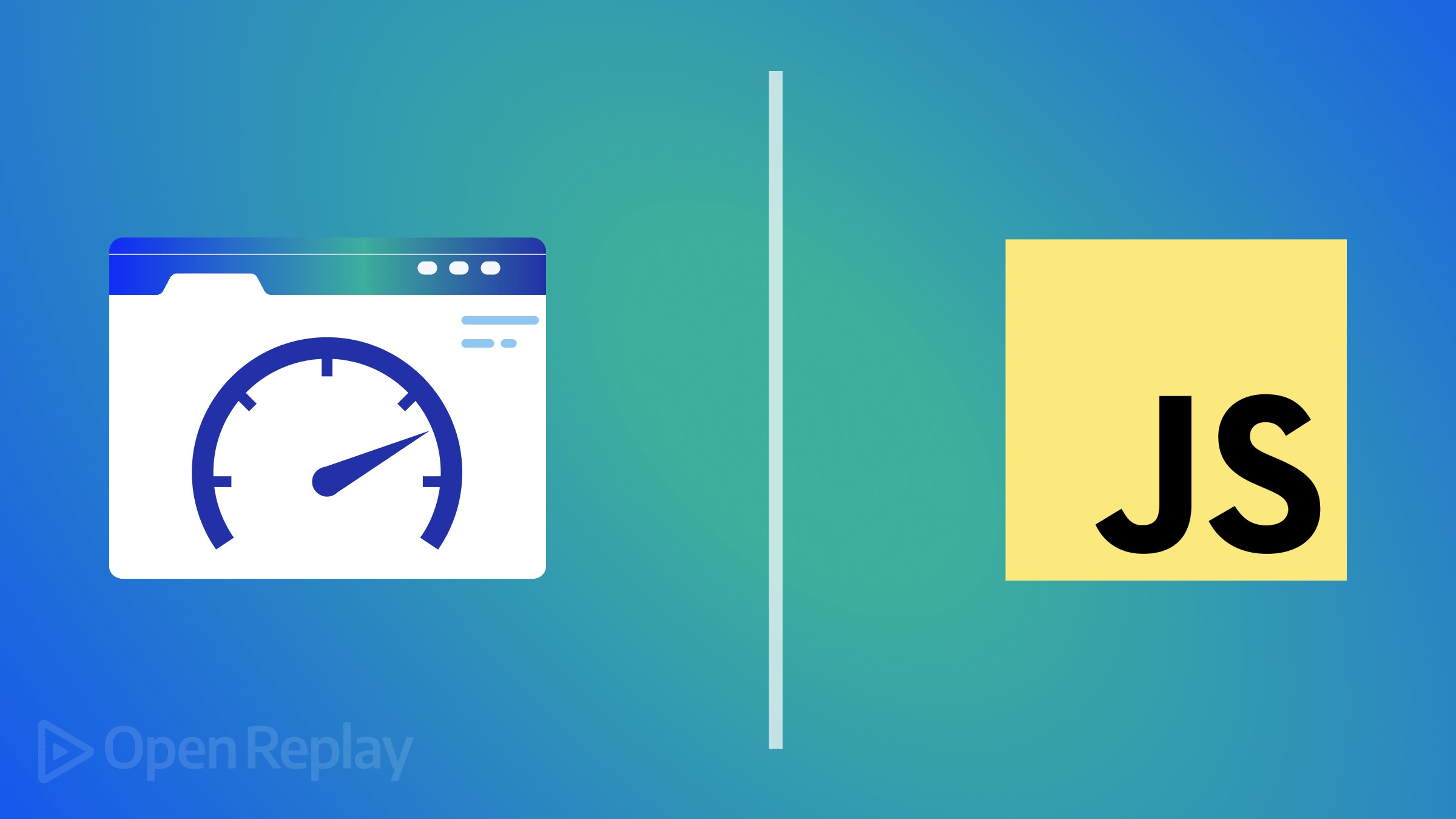
Event delegation is a technique in web development used to manage events more effectively. Rather than adding event listeners to each element, you can attach one listener to a parent element to improve efficiency. This parent then handles events for all of its child elements and is more efficient, as this article shows.
Discover how at OpenReplay.com.
Imagine a web page with multiple interactive buttons. Handling each button’s click event separately can be cumbersome and inefficient. How do developers streamline this process and keep performance in check? The answer lies in JavaScript event delegation.
This method is particularly useful when dealing with dynamic content where new elements are introduced after the page loads. This approach simplifies your code, making managing dynamic changes in the DOM easier.
Benefits of Event Delegation
Event delegation offers several advantages that improve both performance and code maintainability. Below are some key benefits:
-
Efficient Handling of Large Lists or Grids: In apps with large lists or grids—like product listings, data tables, or photo galleries—event delegation can greatly reduce the work of managing numerous event listeners. Instead of attaching a click event to each product or cell in a table, you can delegate the event handling to the parent container. For instance, in an e-commerce site with hundreds of product items displayed in a grid, a single event listener on the grid container can manage clicks for all products. This reduces memory usage and simplifies the code, making it easier to implement and maintain features like product selection, hover effects, or inline editing.
-
Handling Events for Nested Elements: Managing events for elements within other elements can be simplified with event delegation. Instead of creating individual handlers for each element, you can control all the events from one central place. For example, suppose you have a multi-level menu with different items and sub-items. In that case, you can use event delegation to track clicks on any of these menu items, whether in the main menu, sub-menus, or categories. By attaching an event listener to the top-level menu container, you can manage clicks for all nested elements. The event listener can use conditional logic to determine which specific item was clicked, allowing you to apply the appropriate action based on the element’s role within the menu structure. This approach keeps the codebase clean and makes implementing and updating complex interactive elements easier.
-
Managing User Interactions in Form Fields: Forms with multiple input fields, buttons, and other interactive elements can also benefit from event delegation. For instance, if you need to validate form fields or track user interactions across various inputs, you can attach a single event listener to the form element itself. This listener can then manage events like
focus
,blur
, orinput
across all form fields. This approach is especially useful when dealing with forms that include dynamically added inputs, such as when users can add or remove fields in a survey or questionnaire. Event delegation ensures that all fields, whether initially present or added later, are handled consistently by the same event logic. -
Implementing Global Event Handlers: In some applications, you may want to implement global event handlers that manage interactions across the entire document or within a specific section of the page. For example, you might want to implement a feature that tracks all clicks within a certain section to trigger analytics events or to manage keyboard shortcuts across the entire page.
These real-world scenarios highlight the versatility and efficiency of event delegation, making it an essential tool in modern web development and ensuring a clean code.
The Concept of Event Bubbling
Event Bubbling is an important concept to familiarize yourself with before learning how event delegation works. This will enable you to know how events work in the DOM. When an event is triggered on an element, it doesn’t end at that point. Rather than stopping there, it “bubbles” up, moving from the target element to its parent elements and then to the next parent element. This pattern continues until it reaches the root element.
For example, if you click a button
within a div
, the click event starts on the button
and then moves up to the div
. Event delegation takes advantage of this phenomenon by enabling developers to manage events more effectively. By attaching a single event listener to just one parent element, you can handle events for multiple elements with one listener and improve efficiency without multiple event listeners.
How Events Propagate Up the DOM Tree
Event propagation refers to how information travels through a web page’s tree-like structure after an action occurs. This movement can either bubble up from the target element to its ancestors or capture down from the top-level document to the target. There are three phases in event propagation:
-
Capturing Phase: The event starts from the root of the DOM tree and moves down towards the target element. This phase is also called the trickling phase because the event trickles down the tree.
-
Target Phase: The event reaches the target element, where it is executed. This is the phase where the event listener attached directly to the target element is triggered.
-
Bubbling Phase: After the event is handled at the target element, it bubbles up from the target element back through the DOM tree to the root. This phase allows parent elements to listen for events triggered by their child elements.
Event bubbling is the most commonly used phase in event delegation. By understanding how events propagate, developers can effectively manage event listeners and handle events at different levels of the DOM tree.
Event Bubbling in Action
In this section, you can visualize how event bubbling works through a practical example. To see event bubbling in action, consider a simple example involving nested HTML elements:
<div id="parent">
<button id="child">Click Me!</button>
</div>
The example given above contains the div
and button
elements. If you make something happen when you click the div
and someone clicks the button inside that div
, the button gets clicked first. But since the button is inside the div
, the click event will trigger actions for both the button
and the div
, as the event bubbles up the DOM.
document.getElementById('parent').addEventListener('click', function(event) {
console.log('Parent clicked');
});
document.getElementById('child').addEventListener('click', function(event) {
console.log('Child clicked');
});
When the button is clicked, the following will be displayed in the console
Child clicked
Parent clicked
How Event Delegation Works
Event delegation involves delegating event handling from individual child elements to a common ancestor (usually a parent or a higher-level container). Doing so allows you to manage events more efficiently and avoid attaching listeners to every element. It involves attaching an event listener to a parent element rather than to each child element individually. The key advantage of event delegation is efficiency.
Code Examples Illustrating the Approach
Suppose there is a list of items that need to be assigned a click event; you can simply add a click event to just the parent element (ul
) instead of adding event listeners to each of the elements (li
).
Consider the code below:
<!-- Here’s the HTML structure: -->
<ul id="item-list">
<li class="item"> Item 1 </li>
<li class="item"> Item 2 </li>
<li class="item"> Item 3 </li>
</ul>
And here’s the JavaScript code to implement event delegation:
document.getElementById('item-list').addEventListener('click', function(event) {
// Check if the clicked element is a list item
if (event.target && event.target.matches('li.item')) {
console.log('You clicked on', event.target.textContent);
}
});
Inside the event listener function, we check if the event’s target (the element that was actually clicked) matches the selector li.item
. If it does, the event is handled, and the text content of the clicked item is logged into the console.
Here’s what happens when you click on “Item 2”:
You clicked on Item 2
The parent ul
element capture the event, but it’s only handled if the clicked element is one of the list items (li.item
). This efficient approach eliminates the need to attach separate event listeners to each list item.
Handling Dynamic Elements
One significant benefit of event delegation is that it automatically handles dynamic elements. If you add new list items to the ul
element after the page has loaded, the same event listener will still capture and handle click events for the new items without any additional code.
For example, if you add a new item dynamically:
const newItem = document.createElement('li');
newItem.textContent = 'Item 4';
newItem.classList.add('item');
document.getElementById('item-list').appendChild(newItem);
Clicking on “Item 4” will still trigger the same event handler:
You clicked on Item 4
This illustrates the power of event delegation, where a single event listener on a parent element can manage events for both existing and dynamically added child elements.
Potential Drawbacks of Event Delegation
While event delegation offers significant advantages in terms of performance and code simplicity, it’s important to recognize potential drawbacks that may arise when using this technique. Below are some of its disadvantages:
-
Performance Overhead: Even though event delegation reduces the number of event listeners, it can also introduce some performance overhead when dealing with large collections of child elements. The parent element’s event listener must identify which child element triggered the event, which involves checking the
event.target
or matching selectors. This process can slow down performance, especially in scenarios involving thousands of child elements. Consideration: In such cases, optimizing the event delegation logic by narrowing down the event-handling conditions can help. For instance, you can add specific conditions to minimize unnecessary checks. -
Handling Empty Lists or Containers: A situation to watch out for is when the parent element (e.g., a
ul
) has no child elements (e.g.,li
items). In this case, the event listener will still be attached, but there may be no meaningful action to take if no child elements exist. This could result in unnecessary event handling for clicks on empty areas. Consideration: To address this, consider including checks(such asif
statements) in your event handler to verify the presence of child elements before performing any actions. For example, ensure that theevent.target
matches an expected child selector before proceeding with the event-handling logic. Additionally, you could dynamically add or remove the event listener based on whether the child elements exist. -
Complex Event Handling Logic: Event delegation can become complicated when dealing with complex DOM structures or when child elements have different behaviors. For instance, if you have multiple types of child elements within the parent container, the event delegation code may need to account for various scenarios, making the logic more complex and harder to manage. Consideration: If the complexity of the event delegation logic increases significantly, it might be better to use a combination of delegation and direct event listeners for specific elements. This can simplify the code and make it more maintainable in some situations.
Conclusion
In conclusion, event delegation is a valuable strategy for writing more efficient and scalable JavaScript code. As your projects grow in complexity, utilizing event delegation could help you create clean codes with efficient applications. It not only makes your web applications run better, but it also makes your code easier to maintain and adapt. By using event delegation, you can add a listener to a main parent element (like document
or a specific container) and handle events from any of its child elements. This lets you handle multiple events with less code, making your application more efficient and easier to manage.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.