How to Fix 'Cannot Set Headers After They Are Sent to the Client' Error in Node.js and Express.js
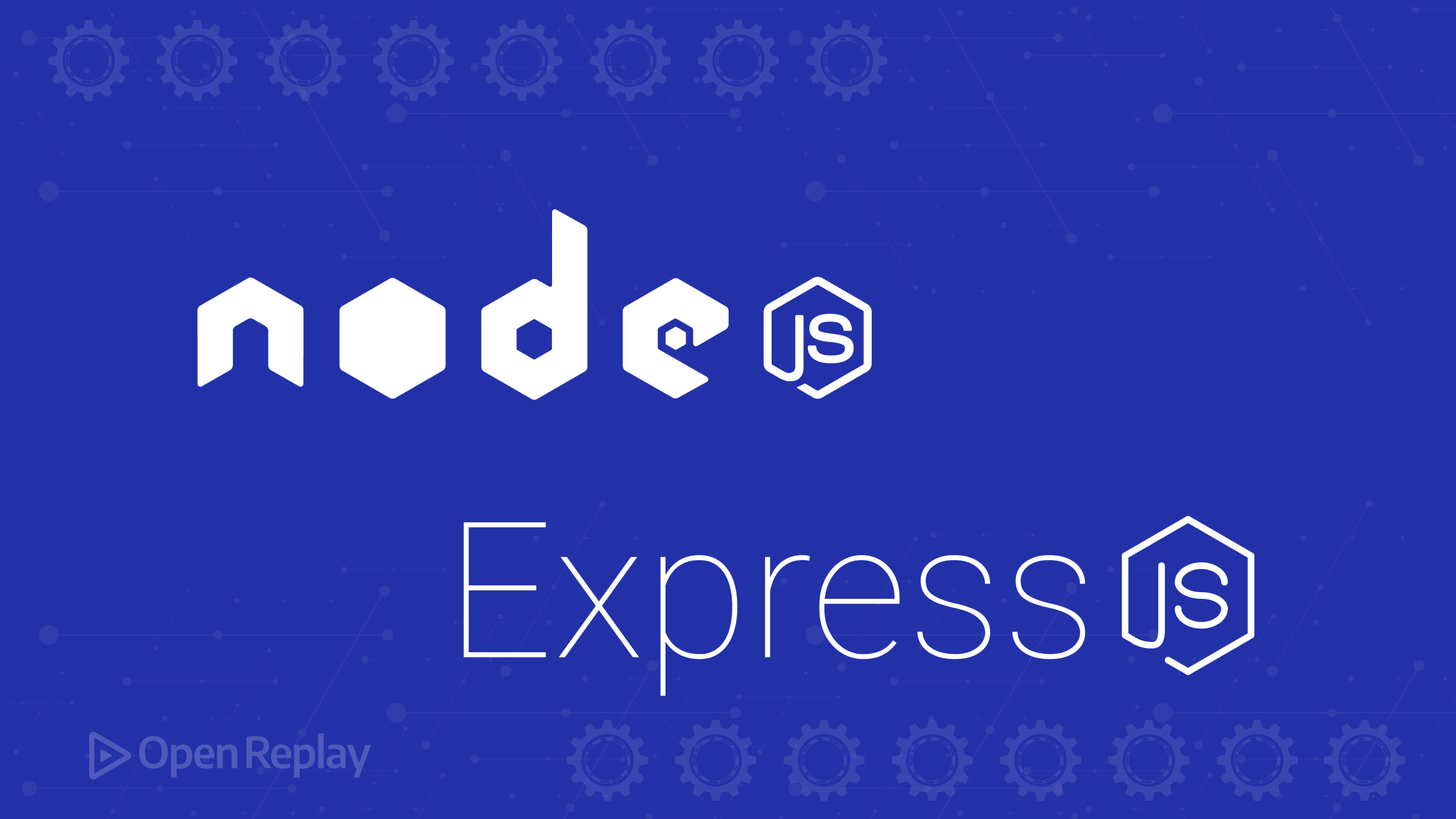
If you’ve encountered the “Cannot set headers after they are sent to the client” error while working with Node.js or Express.js, you’re not alone. This common issue occurs when trying to modify response headers after the response has already been sent or when multiple responses are triggered for a single request. In this article, we’ll explain the causes of this error and provide practical solutions to fix it in your Node.js and Express.js applications.
Key Takeaways
- The “Cannot set headers after they are sent to the client” error occurs when trying to modify response headers after the response has been sent or when sending multiple responses for a single request.
- To fix the error, ensure you send only one response per request, set headers before sending the response, and handle asynchronous code correctly.
- Follow best practices like using
return
statements, implementing error handling, and utilizing middleware to prevent the error.
Understanding the HTTP Request-Response Cycle
To grasp why the “Cannot set headers after they are sent to the client” error occurs, it’s essential to understand the HTTP request-response cycle:
- The client sends a request to the server (e.g., GET, POST).
- The server processes the request and generates a response.
- The server sends the response back to the client.
In Node.js and Express.js, each request should receive only one response. Attempting to send multiple responses or modifying headers after sending the response leads to the error.
Common Causes of the Error
The “Cannot set headers after they are sent to the client” error can happen due to several reasons:
Sending Multiple Responses
One of the most common causes is sending multiple responses for a single request. For example:
app.get('/example', (req, res) => {
res.send('Response 1');
res.send('Response 2'); // Error: Cannot set headers after they are sent to the client
});
Setting Headers After Sending Response
Another cause is trying to set response headers after the response has been sent:
app.get('/example', (req, res) => {
res.send('Response');
res.setHeader('Content-Type', 'application/json'); // Error
});
Improper Handling of Asynchronous Code
Asynchronous operations, such as database queries or API calls, can also lead to this error if not handled properly:
app.get('/example', (req, res) => {
asyncOperation((err, data) => {
if (err) {
res.status(500).send('Error');
}
res.send(data);
});
res.send('Response'); // Error
});
Solutions to Fix the Error
Here are some solutions to fix the “Cannot set headers after they are sent to the client” error:
Ensure Single Response per Request
Make sure you send only one response per request. Avoid calling res.send()
, res.json()
, or similar methods multiple times:
app.get('/example', (req, res) => {
if (condition) {
return res.send('Response 1');
}
res.send('Response 2');
});
Set Headers Before Sending Response
Set any necessary headers before sending the response:
app.get('/example', (req, res) => {
res.setHeader('Content-Type', 'application/json');
res.send({ message: 'Response' });
});
Handle Asynchronous Code Correctly
When dealing with asynchronous operations, ensure the response is sent only after the operation completes:
app.get('/example', (req, res) => {
asyncOperation((err, data) => {
if (err) {
return res.status(500).send('Error');
}
res.send(data);
});
});
Use Middleware and Error Handling
Utilize middleware functions and error handling to manage responses centrally:
app.use((err, req, res, next) => {
console.error(err);
res.status(500).send('Internal Server Error');
});
Best Practices to Prevent the Error
To avoid encountering the “Cannot set headers after they are sent to the client” error, follow these best practices:
- Send only one response per request.
- Set headers before sending the response.
- Handle asynchronous operations properly.
- Use
return
statements to exit early when sending a response. - Implement proper error handling and middleware.
FAQs
The error occurs when attempting to modify response headers after the response has already been sent or when sending multiple responses for a single request.
Ensure that you send only one response per request. Use `return` statements or conditional logic to prevent sending multiple responses.
Yes, improper handling of asynchronous operations can lead to this error. Make sure the response is sent only after the asynchronous operation completes.
Follow best practices such as sending a single response per request, setting headers before sending the response, handling asynchronous code correctly, using `return` statements, and implementing proper error handling and middleware.
Conclusion
By understanding the causes of the “Cannot set headers after they are sent to the client” error and applying the solutions and best practices discussed in this article, you can effectively fix and prevent this issue in your Node.js and Express.js applications. Remember to send a single response per request, set headers before sending the response, and handle asynchronous code correctly to ensure smooth execution of your application.