Securing Front-end Apps with CORS and CSP
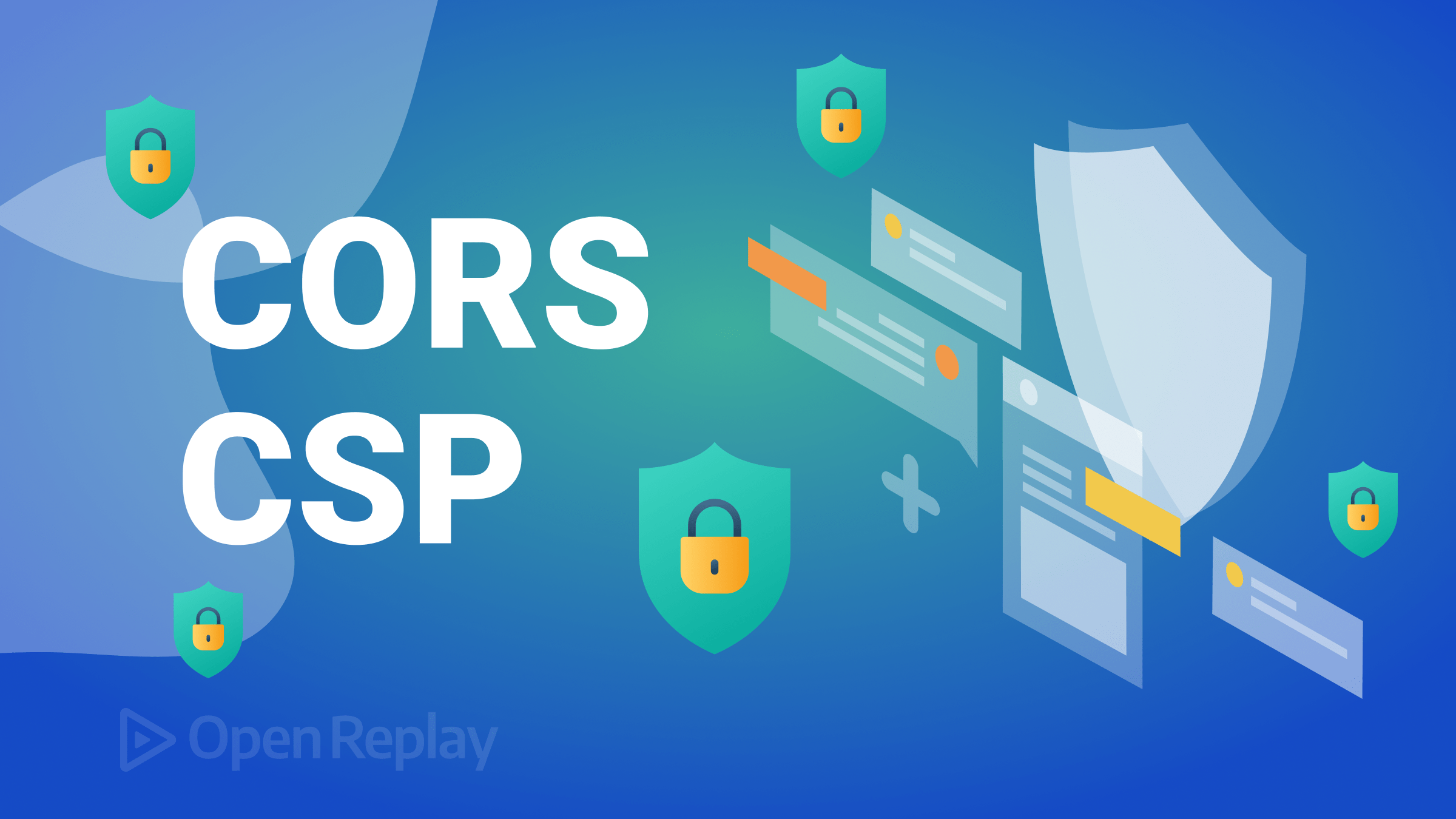
Front-end applications play a central role in delivering a seamless user experience. In today’s interconnected web, where third-party integrations and APIs are prevalent, ensuring robust security is paramount. Security breaches can lead to data theft, unauthorized access, and brand reputation damage. This article will show you how to use CORS and CSP to add security to your web pages.
Discover how at OpenReplay.com.
Hey there, fellow developers! 🖐️ Welcome to our article on “Securing Frontend Apps with CORS and CSP” – an essential read in today’s ever-evolving web landscape.
Imagine a malicious script injected into your app, stealing sensitive user data or redirecting users to fraudulent sites. Scary, right? But fear not! With proper CORS and CSP implementations, we can fortify our front-end apps and stay ahead of potential threats.
Purpose and Scope of this Article
In this article, we dive deep into CORS and CSP to demystify these security measures for you. We’ll learn how to implement them effectively in various front-end frameworks like React, Angular, and Vue.js, with practical examples and code snippets. By the end, you’ll be equipped with the knowledge to secure your front-end apps like a pro!
So, if you’re eager to protect your users and bolster your app’s security, let’s roll up our sleeves and delve into the world of CORS and CSP. Your apps and your users will thank you for it! Let’s get started! 💪
What are CORS and CSP?
Let’s begin with the fundamentals. The crucial security feature known as CORS, or Cross Origin Resource Sharing, enables servers to manage which external resources can access a web application. This keeps our apps safer by preventing every malicious cross origin request.
A strong defense mechanism against content injection attacks like Cross Site Scripting (XSS) and data exfiltration is CSP, or Content Security Policy. It lowers the possibility of unauthorized script execution by enabling developers to specify the sources from which their front-end application can load resources.
// Sample code block demonstrating a simple CORS configuration in Node.js
const express = require("express");
const app = express();
// Enable CORS for all routes
app.use((req, res, next) => {
res.setHeader("Access-Control-Allow-Origin", "*");
res.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE");
res.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization");
next();
});
// ... the rest of your routes and logic
Note: The provided code snippet is a basic example of CORS configuration in Node.js using Express, which allows requests from any origin. In production, you should specify trusted origins instead of using ’*‘.
Understanding CORS
Alright, let’s dive into the nitty-gritty of CORS. 🏊♂️
Same-Origin Policy and Its Limitations
The Same-Origin
Policy, which every web browser enforces, prevents web pages from making requests to domains other than the one that originally served the page. With the help of this policy, potential security risks like unauthorized data access are avoided by ensuring that scripts running in one origin cannot access resources from another origin without express permission.
The Same-Origin
Policy does, however, have some restrictions. For example, it obstructs valid cross origin requests, which are necessary for web applications that depend on APIs from various servers. Your front-end app wouldn’t be able to retrieve data from, say, an API that is hosted on a different domain without CORS. And that’s where CORS steps in to save the day!
Introducing CORS as a Security Mechanism
A web server can explicitly grant web clients permission to access resources from other origins using the CORS mechanism. Servers can tell browsers which origins are allowed access to their resources by using specific HTTP request headers.
How CORS Works and Its Role in Securing Frontend Apps
When a front-end app makes a cross origin request, the browser checks if the server’s response includes the necessary CORS headers. If the headers grant permission (e.g., “Access-Control-Allow-Origin
”), the browser allows the front-end app to access the requested resources. If the headers are missing or incorrect, the browser blocks the request due to security concerns.
CORS plays a pivotal role in securing front-end applications by ensuring that only trusted origins can interact with your app’s back-end resources. This prevents unauthorized access and potential data breaches while still enabling legitimate cross origin requests, fostering a safe and functional web ecosystem. 🌐
// Sample CORS response headers set by the server
app.use((req, res, next) => {
res.setHeader(
"Access-Control-Allow-Origin",
"https://www.trusted-origin.com",
);
res.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE");
res.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization");
// Optionally, allow credentials (e.g., cookies) to be sent in cross origin requests
res.setHeader("Access-Control-Allow-Credentials", "true");
next();
});
Note: In the provided code snippet, the server explicitly allows cross origin requests only from the origin
https://www.trusted-origin.com
. You should replace this with the actual trusted origin(s) of your front-end app.
Implementing CORS
Now that we grasp the significance of CORS, let’s roll up our sleeves and implement it in our front-end apps! 💪
Configuration Options and Headers for CORS
To enable CORS in your back-end server, you need to set specific response headers. The most essential header is “Access-Control-Allow-Origin
,” which specifies the origins allowed to access your resources. You can use wildcard (*) to permit access from any origin, but it’s safer to specify trusted origins explicitly.
Other crucial headers include “Access-Control-Allow-Methods
” (defining allowed HTTP methods), “Access-Control-Allow-Headers
” (listing allowed request headers), and optionally “Access-Control-Allow-Credentials
” (if you need to include credentials, like cookies, in cross origin requests).
Step-by-Step Guide on Enabling CORS in Different Frameworks
Enabling CORS varies depending on your back-end framework. Let’s look at a step-by-step guide for popular front-end frameworks:
1. Express (Node.js):
const express = require("express");
const app = express();
// Enable CORS for all routes
app.use((req, res, next) => {
res.setHeader(
"Access-Control-Allow-Origin",
"https://www.trusted-origin.com",
);
res.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE");
res.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization");
// Optionally, allow credentials (e.g., cookies) to be sent in cross origin requests
res.setHeader("Access-Control-Allow-Credentials", "true");
next();
});
2. Django (Python):
# In settings.py
CORS_ORIGIN_WHITELIST = [
'https://www.trusted-origin.com',
]
CORS_ALLOW_METHODS = ['GET', 'POST', 'PUT', 'DELETE']
CORS_ALLOW_HEADERS = ['Content-Type', 'Authorization']
CORS_ALLOW_CREDENTIALS = True # Optionally, allow credentials
Common Pitfalls and Best Practices for CORS Implementation
Watch out for potential pitfalls when implementing CORS, such as excessively permissive “Access-Control-Allow-Origin
” settings that might expose your resources to unauthorized origins. To avoid security vulnerabilities, validate and sanitize input data at all times.
To reduce risks, best practices call for handling preflight requests, setting strict “Access-Control-Allow-Origin
” values, and specifying the proper “Access-Control-Allow-Methods
” and “Access-Control-Allow-Headers
.”
To create a strong defense for your front-end apps, other security measures like input validation and authentication should be added on top of CORS, which should be considered an essential layer of security. Be vigilant and guard against threats to your apps!
Introduction to CSP
Alright, folks, let’s shift gears and explore the realm of Content Security Policy
(CSP) – a powerful ally in safeguarding our front-end apps! 🛡️
Overview of Content Security Policy and Its Objectives
Your front-end app’s content security policy (CSP) acts as a bouncer, deciding who is allowed inside and who is not. By limiting the sources from which your app can load external content, like scripts, stylesheets, and images, it aims to reduce content injection attacks like cross Site Scripting (XSS).
Even if malicious scripts manage to enter your app via user-generated content or external resources, you can stop them from being executed by defining a strict policy. By giving you precise control over what your app can and cannot load, CSP functions as an additional security layer, minimizing the attack surface.
Understanding the Need for Restricting External Content
In today’s web, front-end apps often rely on external resources like libraries, fonts, or analytics scripts. However, such dependencies can be exploited by attackers to inject harmful code into your app, compromising user data and undermining trust. Restricting external content through CSP ensures that only trusted sources are permitted, effectively curbing such threats.
Comparison of CSP with Other Security Mechanisms
CSP stands out from other security mechanisms like XSS filters and Cross Site Request Forgery (CSRF) tokens. While XSS filters attempt to detect and neutralize malicious scripts, they aren’t foolproof and may have compatibility issues. On the other hand, CSRF tokens focus on preventing unauthorized actions but can’t address content injection attacks.
CSP tackles the root cause by blocking malicious content from loading altogether, making it more robust and reliable. Combining CSP with other security measures creates a formidable defense, protecting your front-end app from a wide range of threats.
<!-- Sample CSP meta tag to restrict external content -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' 'trusted-scripts.com'; style-src 'self' 'trusted-styles.com'; img-src 'self' data:">
Note: In the provided
code snippet
, the CSP policy allows loading scripts only from the same origin and ‘trusted-scripts.com’, stylesheets from the same origin and ‘trusted-styles.com’, and images from the same origin and data URLs. You should customize the policy based on your app’s requirements.
Implementing CSP
Time to tighten the security screws on our front-end apps with Content Security Policy (CSP)! Let’s dive right in! 🛡️
Defining a Content Security Policy through Headers and Meta Tags
CSP can be defined either through HTTP response headers or meta tags. For HTTP headers, the server includes the “Content-Security-Policy” header in its response, specifying the policy directives. On the other hand, using a meta tag in the HTML allows you to define the policy directly within the document.
Setting CSP via HTTP Headers (in Node.js using Express):
const express = require("express");
const app = express();
// Set the CSP header for all responses
app.use((req, res, next) => {
res.setHeader(
"Content-Security-Policy",
"default-src 'self'; script-src 'self' 'trusted-scripts.com'; style-src 'self' 'trusted-styles.com'; img-src 'self' data:",
);
next();
});
Setting CSP via Meta Tag (in HTML):
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' 'trusted-scripts.com'; style-src 'self' 'trusted-styles.com'; img-src 'self' data:">
<!-- Rest of the head section -->
</head>
<body>
<!-- Your app’s content -->
</body>
</html>
Exploring every CSP Directive
CSP provides various directives that control specific types of resources and actions. For instance:
default-src
: Defines the default behavior for other directives if they are not explicitly specified.script-src
: Specifies the allowed sources for JavaScript.style-src
: Sets the sources for stylesheets.img-src
: Determines the allowed sources for images.
You can also use nonce
and hash
attributes to add dynamic scripts and inline styles while still adhering to the policy.
Case Studies Showcasing How CSP Mitigates Common Frontend Security Vulnerabilities
CSP is a superhero when it comes to thwarting security vulnerabilities! It prevents XSS attacks by blocking unauthorized script executions, stops data exfiltration
by restricting resource loading to trusted origins, and mitigates clickjacking attacks by controlling frame embedding. By implementing CSP, many prominent websites have successfully thwarted attacks and kept their users safe.
With the right CSP directives tailored to your app’s requirements, you can confidently defend against a wide range of front-end security threats and ensure your users enjoy a secure browsing experience. So, get ready to wield the power of CSP and fortify your front-end app like a pro! 🔒
Combining CORS and CSP
Now that we’ve armed ourselves with CORS and CSP, it’s time to unleash their combined might to create an impenetrable fortress around our front-end apps! 🏰
The Synergistic Effect of CORS and CSP in Strengthening Frontend App Security
CORS and CSP complement each other like a dynamic duo, working hand-in-hand to defend your app from different angles. CORS focuses on controlling cross origin requests, ensuring that only trusted sources can access your back-end resources. Meanwhile, CSP tackles content injection attacks, preventing unauthorized scripts from executing on your front-end.
By combining both mechanisms, we’re not only safeguarding data transmission but also protecting the integrity of our front-end. Malicious scripts attempting to exploit cross origin weaknesses or bypass server-side security measures are thwarted by CSP’s vigilant watch.
Addressing Challenges and Potential Conflicts
Implementing CORS and CSP together might pose some challenges and conflicts. For instance, when CORS allows cross origin requests from specific domains, those domains should be included in the CSP policy to enable loading resources from them.
Additionally, if you’re using inline scripts/styles or dynamic script loading, you’ll need to set appropriate CSP nonces or hashes to allow them while still adhering to the policy. This coordination between the two mechanisms requires careful consideration and testing.
<!-- Sample CSP policy with CORS trusted origin included -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' 'trusted-scripts.com'; style-src 'self' 'trusted-styles.com'; img-src 'self' data: 'trusted-origin.com'">
In the end, the benefits of combining CORS and CSP outweigh the challenges. Your front-end app becomes a fortress of security, bolstered by multiple layers of protection. Remember to regularly review and fine-tune your policies as your app evolves, ensuring a strong defense against emerging threats.
So, let’s unite CORS and CSP in harmony and create a safe, trustworthy user experience for everyone! 🛡️
Testing and Debugging
As guardians of front-end app security, we must thoroughly test and debug our CORS and CSP configurations to ensure their effectiveness. Let’s explore some tools and techniques to tackle this crucial task! 🔍🚀
Tools and Techniques for Testing CORS and CSP Configurations
-
Browser Developer Tools: Modern browsers offer powerful developer tools that display CSP violations in the console and network tabs. They help you identify and rectify any policy-related issues.
-
Online CSP Analyzers: Several online tools allow you to analyze your CSP headers and provide detailed reports on potential vulnerabilities and misconfigurations.
-
CORS Tester Extensions: Browser extensions like “CORS Everywhere” or “CORS Toggle” let you test different CORS configurations for your app, helping you ensure that cross origin requests are functioning as expected.
-
Security Headers Checkers: Online security header checkers can assess your CORS and CSP headers, making it easier to spot any inconsistencies or weaknesses.
Identifying and Resolving Issues Related to Cross Origin Requests and Content Restrictions
-
Console Errors: Check the browser console for CORS-related errors and CSP violation reports. Use this information to fine-tune your configurations.
-
Testing with Different Origins: Verify your app’s behavior by testing it with various origins, both trusted and untrusted. This ensures that your CORS and CSP policies are adequately restricting access.
-
Testing Dynamic Content: If your app generates scripts dynamically, test and adjust CSP nonces or hashes to accommodate them.
-
Opt-In Reporting: Enable CSP reporting to collect violation reports from the browser and gain insights into potential issues. These reports aid in refining your policy.
<!-- Example of enabling CSP Reporting through the report-uri directive -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; report-uri /csp-violation-report-endpoint">
- Iterative Testing: As your app evolves, conduct regular testing to ensure your policies align with the latest requirements.
Remember, testing and debugging are ongoing processes. Stay vigilant, be proactive in addressing potential problems, and leverage the insights gained from testing to fine-tune your CORS and CSP configurations. By doing so, you’ll achieve an optimized, ironclad security posture for your front-end app, safeguarding your users and their data from any lurking threats. Happy testing! 🛡️🔍
Real-world Examples
Let’s venture into the real-world realm, where the dynamic duo of CORS and CSP have proven their mettle, safeguarding front-end app security with valor! 🛡️🌐
Examining Real-World Scenarios
-
Preventing Cross Site Scripting (XSS) Attacks: Imagine a blog website that allows users to post comments. With a well-crafted CSP policy, inline scripts and unauthorized external scripts are blocked from executing. This thwarts potential XSS attacks, protecting both the website’s integrity and its visitors.
-
Securing Cross Origin Requests in Single-Page Applications (SPAs): SPAs often fetch data from multiple APIs hosted on different domains. By implementing CORS, these SPAs restrict cross origin requests to only authorized servers, preventing attackers from exploiting cross origin weaknesses.
Analyzing Security Breaches that Could Have Been Prevented
-
Data Leakage due to Misconfigured CORS: In a misconfigured back-end, sensitive data might be exposed to unauthorized domains through CORS. With a proper CORS policy that restricts origins, such data leaks could have been avoided.
-
Injection Attacks through CSP Bypass: A website lacking a CSP policy or with lax restrictions may fall prey to content injection attacks. By enforcing a robust CSP policy, such security breaches could have been mitigated.
<!-- Sample strict CSP policy to mitigate content injection -->
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self'; style-src 'self'; object-src 'none'; base-uri 'self'; form-action 'self'; block-all-mixed-content;">
In these real-world examples, we witness how the strategic use of CORS and CSP acts as an armor, repelling potential threats and ensuring a safer browsing experience for users. By diligently applying these security measures, developers fortify their front-end apps, earning the trust of their audience and protecting sensitive data from falling into the wrong hands. Stay vigilant and embrace the power of CORS and CSP to create a safer digital world for all! 🛡️🌐
Conclusion
Congratulations, fearless developers! 🎉 You’ve journeyed through the realm of CORS and CSP, learning how these mighty guardians defend our front-end apps from malicious threats. Let’s recap the significance of CORS and CSP and inspire you to lead the charge in safeguarding web applications! 🛡️💻
Recapitulation of the Significance of CORS and CSP
CORS, our trusted ally in cross origin protection, ensures that only authorized domains can access our back-end resources. By controlling cross origin requests, it thwarts unauthorized access and keeps data safe from prying eyes. On the other hand, CSP fortifies the front-end by restricting content sources, preventing content injection attacks and XSS breaches. Together, they form an impregnable defense, creating a safe and secure environment for our users.
Encouraging Developers to Adopt Best Practices
As defenders of the digital realm, it’s our responsibility to adopt best practices in implementing CORS and CSP. Use strict policies tailored to your app’s needs, allow only trusted origins, and diligently test and debug your configurations. Regularly update your policies as your app evolves, staying ahead of emerging threats.
Although no security measure is foolproof, you can give your front-end apps multiple layers of protection by combining CORS and CSP with other security measures. We can strengthen our digital works and help build a safer web for everyone if we are proactive and watchful.
So let’s promise to be the protectors our apps need! Adopt CORS and CSP, use their influence wisely, and protect our users’ and their data’s security. We’ll create a safer digital future together! 🛡️🌐💪
Truly understand users experience
See every user interaction, feel every frustration and track all hesitations with OpenReplay — the open-source digital experience platform. It can be self-hosted in minutes, giving you complete control over your customer data. . Check our GitHub repo and join the thousands of developers in our community..