如何使用 TypeScript 和 Express 设置 Node.js 项目
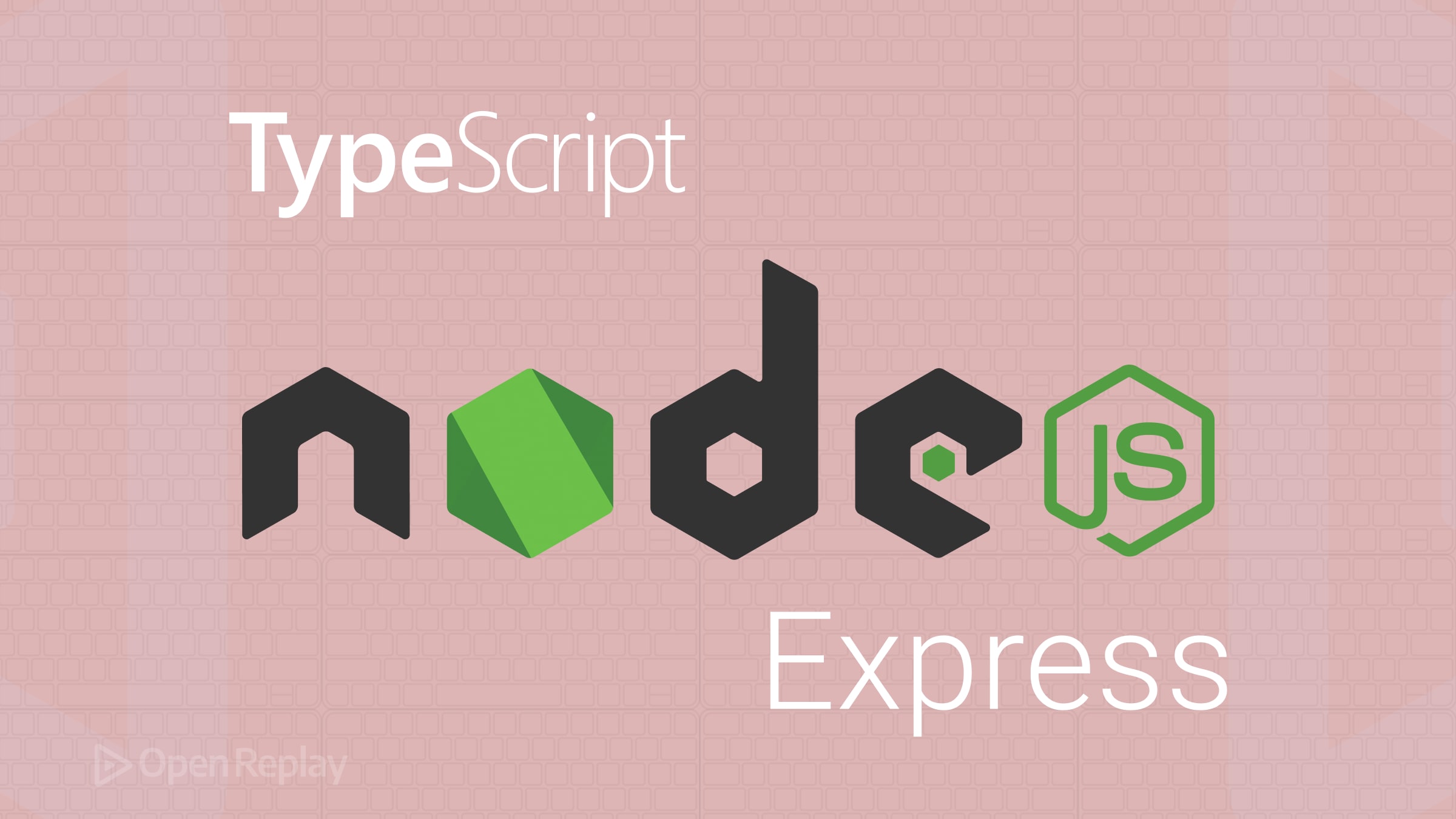
使用 TypeScript 和 Express 设置 Node.js 项目可以为您的应用程序提供结构、可扩展性和清晰度。在本指南中,我们将逐步介绍如何初始化 Node.js 项目、集成 TypeScript、Express 以及添加必要的开发工具。
关键要点
- 快速设置一个健壮的 Node.js 项目,包含 TypeScript 和 Express。
- 集成必要工具:ESLint、Prettier、Jest 和 nodemon,实现无缝开发。
步骤 1:初始化 Node.js 项目
创建项目文件夹并初始化 npm:
mkdir my-node-project
cd my-node-project
npm init -y
这将生成一个 package.json
文件。接下来,创建一个 .gitignore
文件以排除 node 模块和构建文件:
node_modules
dist
步骤 2:添加 TypeScript
安装 TypeScript 和相关依赖:
npm install --save-dev typescript ts-node @types/node
通过以下命令创建 TypeScript 配置:
npx tsc --init
调整 tsconfig.json
文件,使其看起来像这样:
{
"compilerOptions": {
"target": "ES2016",
"module": "commonjs",
"rootDir": "./src",
"outDir": "./dist",
"esModuleInterop": true,
"strict": true,
"skipLibCheck": true
}
}
为代码创建 src
文件夹:
mkdir src
步骤 3:设置 Express
安装 Express 及其类型定义:
npm install express
npm install --save-dev @types/express
在 src/index.ts
创建一个基本服务器:
import express, { Application, Request, Response } from 'express';
const app: Application = express();
const PORT = 3000;
app.get('/', (req: Request, res: Response) => {
res.send('Hello, TypeScript + Express!');
});
app.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}`);
});
使用以下命令运行:
npx ts-node src/index.ts
步骤 4:ESLint 和 Prettier 设置
安装 ESLint、Prettier 和插件:
npm install --save-dev eslint prettier @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-plugin-prettier eslint-config-prettier
通过 .eslintrc.json
设置 ESLint:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint", "prettier"],
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:prettier/recommended"
],
"env": { "node": true, "es6": true, "jest": true }
}
创建 .prettierrc.json
:
{
"semi": true,
"singleQuote": true,
"trailingComma": "all"
}
在 package.json
中更新脚本:
"scripts": {
"lint": "eslint 'src/**/*.{ts,js}'",
"format": "prettier --write ."
}
步骤 5:使用 Jest 进行测试
添加 Jest 和 TypeScript 支持:
npm install --save-dev jest ts-jest @types/jest
npx ts-jest config:init
编写一个简单的测试 src/__tests__/sum.test.ts
:
import { sum } from '../sum';
test('sum works', () => {
expect(sum(1, 2)).toBe(3);
});
对应的模块:
export function sum(a: number, b: number): number {
return a + b;
}
更新 package.json
:
"scripts": {
"test": "jest --watch"
}
步骤 6:使用 Nodemon 进行开发
安装并配置 nodemon:
npm install --save-dev nodemon
添加 nodemon.json
:
{
"watch": ["src"],
"ext": "ts,json",
"ignore": ["src/**/*.test.ts"],
"exec": "ts-node ./src/index.ts"
}
在 package.json
中添加开发环境脚本:
"scripts": {
"dev": "nodemon"
}
运行 npm run dev
以在开发过程中自动重新加载服务器。
最佳实践
- 使用单独的文件夹(
src
存放代码,dist
存放构建输出)。 - 只提交源文件,不提交编译后的代码(
dist
)或 node 模块。 - 经常运行
npm run lint
和npm run format
。 - 在部署前编译代码(
npm run build
),然后使用npm start
启动。
常见问题
是的。只需将 npm 命令替换为相应的 yarn 或 pnpm 命令即可。
不应该。在生产环境中,始终使用 `tsc` 编译项目,并从 `dist` 文件夹运行 JavaScript。
虽然 Jest 是可选的,但它显著提高了可靠性和代码质量,即使对于较简单的项目也很有价值。
Listen to your bugs 🧘, with OpenReplay
See how users use your app and resolve issues fast. Loved by thousands of developers