如何在React中使用Toastify创建Toast消息
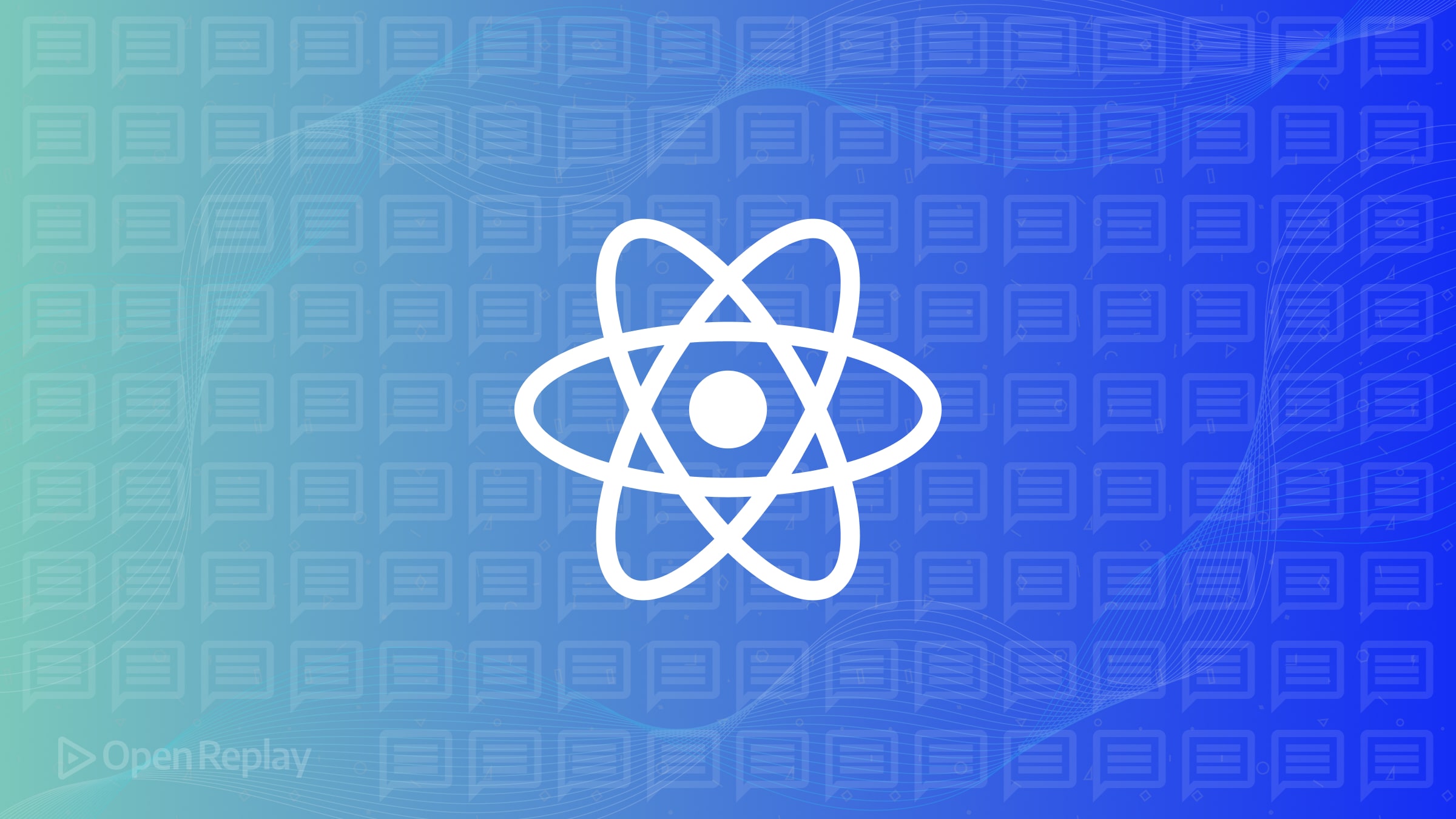
Toast通知是快速、非侵入式的提醒,能在不打断用户工作流程的情况下保持用户知情。React-Toastify简化了这些通知的实现,平衡了易用性和自定义性。
要点
- React-Toastify简化了添加toast通知的过程。
- 支持多种通知类型(成功、错误、警告、信息)。
- 可自定义位置和样式。
- 提供基于Promise的通知等高级功能。
React-Toastify入门
安装
安装React-Toastify:
npm install react-toastify
# 或
yarn add react-toastify
基本设置
导入必要的组件和CSS:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
在主App中放置ToastContainer
:
function App() {
return (
<div>
<button onClick={() => toast(""Hello, Toastify!"")}>通知</button>
<ToastContainer />
</div>
);
}
Toast通知类型
使用特定的toast类型以提高清晰度:
- 成功:
toast.success(""保存成功!"");
- 错误:
toast.error(""发生错误!"");
- 警告:
toast.warning(""检查您的输入!"");
- 信息:
toast.info(""有可用更新!"");
- 默认:
toast(""中性消息"");
自定义Toast通知
位置设置
自定义toast位置:
toast(""消息"", { position: ""bottom-center"" });
位置选项包括top-right
、top-center
、top-left
、bottom-right
、bottom-center
和bottom-left
。
样式设置
应用自定义样式:
toast(""样式化toast"", { className: ""custom-toast"" });
CSS示例:
.custom-toast {
background: navy;
color: white;
border-radius: 4px;
}
条件样式
基于类型设置样式:
const notify = (type, message) => {
toast[type](message, { className: `${type}-toast` });
};
高级功能
基于Promise的Toast
无缝处理异步操作:
const promise = fetch('url').then(res => res.json());
toast.promise(promise, {
pending: ""加载中..."",
success: ""加载成功!"",
error: ""加载数据失败。""
});
自定义内容
包含React组件:
toast(<div>自定义HTML内容 <button onClick={() => alert('已点击!')}>点击</button></div>);
通知中心
轻松跟踪通知:
import { useNotificationCenter } from 'react-toastify/addons/use-notification-center';
const NotificationCenter = () => {
const { notifications, markAllAsRead } = useNotificationCenter();
return (
<div>
<button onClick={markAllAsRead}>标记所有为已读</button>
{notifications.map(n => <div key={n.id}>{n.content}</div>)}
</div>
);
};
最佳实践
- 适度使用: 避免让用户感到不堪重负。
- 选择适当类型: 清晰传达消息意图。
- 谨慎设置持续时间: 重要消息需要更长的可见时间。
- 可访问性: 确保内容可读性和清晰对比度。
- 简洁消息: 明确指出需要什么。
完整实现示例
import React, { useState } from ""react"";
import { ToastContainer, toast } from ""react-toastify"";
import ""react-toastify/dist/ReactToastify.css"";
function ProfileForm() {
const [data, setData] = useState({ username: """", email: """" });
const handleSubmit = (e) => {
e.preventDefault();
if (!data.username || !data.email) {
toast.warning(""所有字段都必填!"");
return;
}
const saveData = new Promise((resolve, reject) => {
setTimeout(() => {
data.email.includes(""@"") ? resolve() : reject();
}, 1500);
});
toast.promise(saveData, {
pending: ""保存中..."",
success: ""个人资料已更新!"",
error: ""无效的电子邮箱!""
});
};
return (
<form onSubmit={handleSubmit}>
<input
type=""text""
placeholder=""用户名""
value={data.username}
onChange={(e) => setData({ ...data, username: e.target.value })}
/>
<input
type=""email""
placeholder=""电子邮箱""
value={data.email}
onChange={(e) => setData({ ...data, email: e.target.value })}
/>
<button type=""submit"">提交</button>
<ToastContainer />
</form>
);
}
结论
React-Toastify帮助在React应用中创建有效、不引人注目的通知。它的简单性和灵活性使得快速集成成为可能,同时允许深度自定义以适应您的应用需求。
常见问题
是的,React-Toastify支持将自定义React组件作为toast内容。
在toast选项中设置`autoClose: false`使通知持续显示。
是的,使用toast的ID通过`toast.update(toastId, options)`来更新。
在`ToastContainer`上使用`limit`属性控制同时显示的通知数量。
是的,确保客户端导入和渲染,特别是在使用Next.js等框架时。
Listen to your bugs 🧘, with OpenReplay
See how users use your app and resolve issues fast. Loved by thousands of developers