A Guide to HTTP POST requests in JavaScript
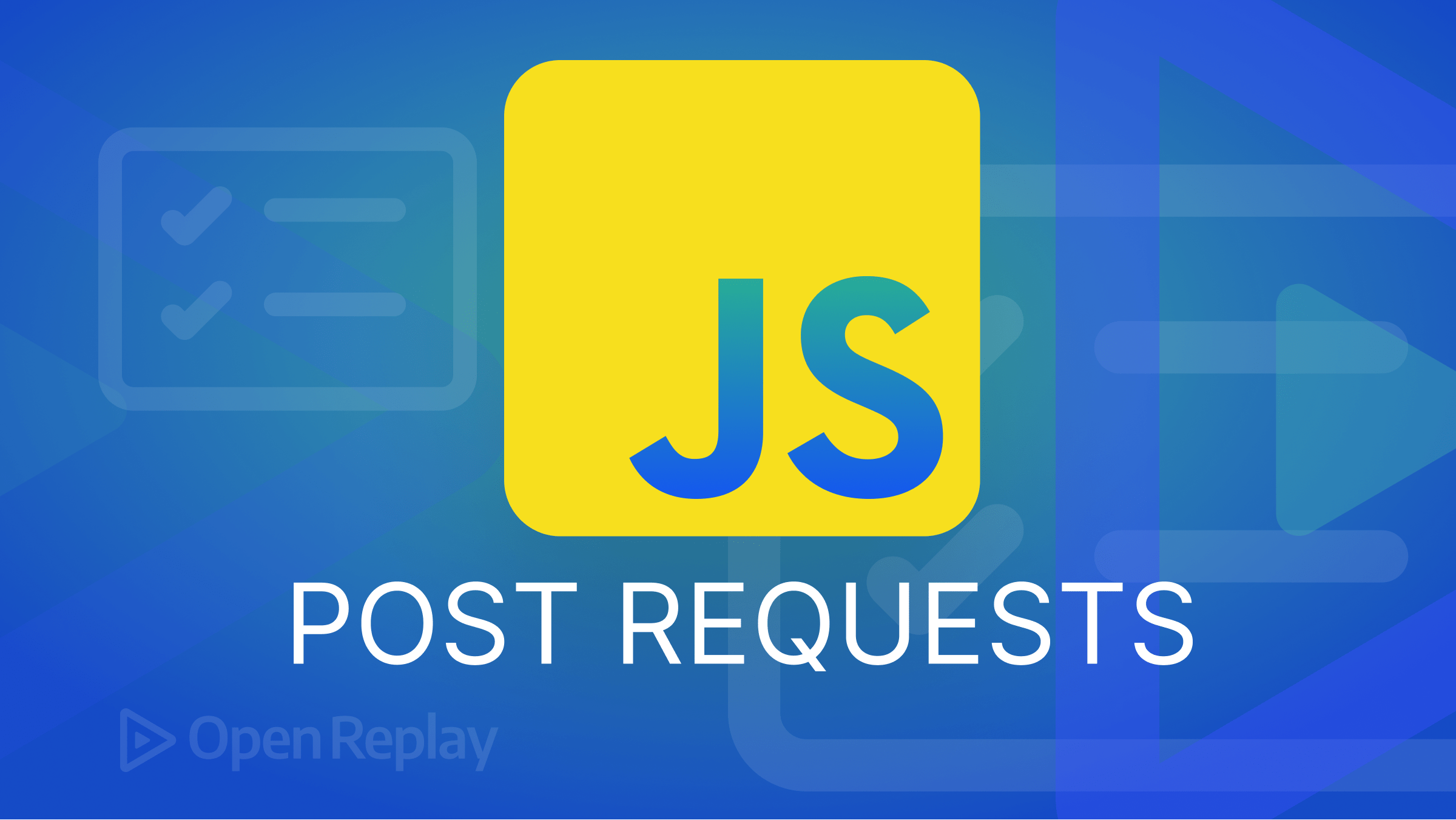
HTTP requests enable front-end applications to communicate with a back-end server or database. The POST method, which you may use to transmit data to a server, is one of the five primary HTTP ways to send requests and communicate with your servers. There are several reasons to use POST requests:
-
To submit (send) data to a server to be processed and stored, such as when submitting a form or uploading a file.
-
To update data on the server, such as updating the status of a task or modifying an entry in a database.
-
To send sensitive information, such as passwords or other confidential data, as they are less likely to be intercepted than other requests.
-
To send large amounts of data, because they can handle more significant amounts of data than other requests, making them useful for sending large files or data sets.
-
To support API interactions, because many APIs use such requests to create, update, or retrieve data from a server.
-
To send multiple parameters, because these requests can send multiple parameters in the body, allowing more complex data to be sent to the server.
This article will demonstrate numerous approaches for sending an HTTP POST request
to the back-end server using JavaScript. There are two built-in JavaScript techniques for performing an HTTP POST request that does not need the installation of a library or the usage of a CDN: the Fetch API, based on JavaScript promises, and XMLHttpRequest, based on callbacks. You will also learn how to use additional techniques, such as Axios and jQuery.
How to Send a POST Request with Fetch API
The FetchAPI is a built-in method that requires only one parameter: the endpoint (API URL). While the additional arguments are not required for a GET request, they are highly beneficial for a POST HTTP request.
The second parameter provides the body (data to be sent) and the kind of request to be made, while the third parameter is a header that identifies the type of data to be transmitted, such as JSON.
fetch("https://jsonplaceholder.typicode.com/todos", {
method: "POST",
headers: { "Content-type": "application/json" },
body: JSON.stringify({ userId: 1, title: "Fix the bugs", completed: false })
});
This code is a Fetch API call that sends an HTTP POST request to ”https://jsonplaceholder.typicode.com/todos“.
The body of the request comprises a JSON string that represents a to-do item with the following properties: userId 1, title “Fix the bugs,” and finished as false
.
The request headers specify the content type as application/json
; charset=UTF-8
. This header is necessary to let the server know what format the data is sent in.
Because the fetch API is built on JavaScript promises, you must retrieve the promise or answer returned using the .then
function.
fetch("https://jsonplaceholder.typicode.com/todos", {
method: "POST",
body: JSON.stringify({
userId: 1,
title: "Open Replay",
completed: false
}),
headers: {
"Content-type": "application/json; charset=UTF-8"
}
})
.then((response) => response.json())
.then((json) => console.log(json));
If this works, it will return the updated JSON
data you sent to the server.
How to Send POST Request with XMLHttpRequest
Like the Fetch API, XMLHttpRequest is built-in and has been around for far longer than the Fetch API. This implies that practically all current browsers have an XMLHttpRequest object
that may be used to request data from a server.
To begin, create a new XMLHttpRequest
object and save it in a variable named xhr. This is important since it allows you to access all of the variable’s objects.
Example:
You can then use JavaScript .open()
to create a connection and specify the request type and endpoint (the URL of the server). You will provide the data using the setRequestHeader method, just as you did with the FetchAPI
.
const xhr = new XMLHttpRequest();
xhr.open("POST", "https://jsonplaceholder.typicode.com/todos");
xhr.setRequestHeader("Content-Type", "application/json; charset=UTF-8")
Below step is to generate the data that will be transmitted to the server. Make careful you serialize the data before storing it in a variable that will be sent using the JavaScript .send()
function after certain checks with the.onload method.
const body = JSON.stringify({
userId: 1,
title: "Open Replay",
completed: false
});
xhr.onload = () => {
if (xhr.readyState == 4 && xhr.status == 201) {
console.log(JSON.parse(xhr.responseText));
} else {
console.log(`Error: ${xhr.status}`);
}
};
xhr.send(body);
When you finish the code, it should look like this and return the JSON
data you sent to the server:
const xhr = new XMLHttpRequest();
xhr.open("POST", "https://jsonplaceholder.typicode.com/todos");
xhr.setRequestHeader("Content-Type", "application/json; charset=UTF-8");
const body = JSON.stringify({
userId: 1,
title: "Fix the bugs",
completed: false
});
xhr.onload = () => {
if (xhr.readyState == 4 && xhr.status == 201) {
console.log(JSON.parse(xhr.responseText));
} else {
console.log(`Error: ${xhr.status}`);
}
};
xhr.send(body);
The main distinction between the fetch API and the XMLHttpRequest
technique is that the fetch API
has a more readable and understandable syntax.
You’ve now learned how to send POST HTTP
requests using the two JavaScript built-in methods.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
How To Pass Multiple Kinds of Values in the Body
You can pass multiple values in the body of a JavaScript POST request
by creating an object that holds the values and then stringifying the object using the JSON.stringify method
before passing it as the request’s body.
Here’s an example:
fetch("https://your-api-endpoint.com", {
method: "POST",
body: JSON.stringify({
name: "John Doe",
age: 30,
location: "San Francisco",
isAdmin: true
}),
headers: {
"Content-Type": "application/json; charset=UTF-8"
}
})
In the example above, the body of the request contains an object with four properties: name, age, location, and isAdmin. The JSON.stringify
is used to convert this object into a JSON string
, which can then be passed as the body of the request.
How to send different types of data with the Fetch () API
The JavaScript Fetch() API
provides a simple way to send different types of data, such as images, blob files, and form data, in an HTTP request
. In this part, we’ll look at how to transfer various types of data using the Fetch () API
, as well as discuss the code behind each example.
Sending Form Data
To send form data with Fetch, you can use the FormData object
. The FormData object
can be used to send key-value pairs, similar to the format of an HTML
form.
const formData = new FormData();
formData.append('key', 'value');
Here, we create a new instance of FormData, and then add a key-value pair to it using the append()
method. The first argument of append()
is the key, and the second argument is the value.
fetch('https://example.com/api/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code sends an HTTP POST request
to the endpoint https://example.com/api/upload using Fetch ()
. The body property of the object passed to fetch()
is set to formData
, which is the instance of the FormData
object created earlier.
In the .then
blocks, we handle the response from the server. First, we convert the response to a JSON object
using response.json()
. Then, we log the data to the console or catch any errors that occur during the process.
Sending Images
To send an image with Fetch ()
, we first need to convert the image file into a Blob object. The Blob object represents a file-like object of immutable, raw data.
const image = new Blob([imageFile], {type: 'image/jpeg'});
In the first line, we create a new Blob object and pass it two arguments: imageFile and an object with the type of image. The Blob constructor takes an array of data as its first argument and an object with the type of the Blob as its second argument.
fetch('https://example.com/api/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code sends an HTTP POST request to the endpoint https://example.com/api/upload using Fetch ()
. The body property of the object passed to Fetch()
is set to formData
, which contains the file we want to send.
In the then blocks, we handle the response from the server. First, we convert the response to a JSON object
using response.json()
. Then, we log the data to the console or catch any errors that occur during the process.
Note that this technique can also be applied to send other types of generic files, not just images.
Conclusion
In conclusion, sending an HTTP POST request in JavaScript can be easily achieved using the fetch API
. The fetch API
provides a simple and convenient way to make network requests and handle responses. To send a POST request
, you can specify the desired endpoint URL, the request method as POST
, the body of the request, and the request headers. The request body can contain multiple values and be passed as a stringified
JSON object
. Understanding how to make HTTP POST requests
in JavaScript
is an essential aspect of web development and can be used to create dynamic and interactive web applications.