A Guide to Using localStorage in JavaScript Apps
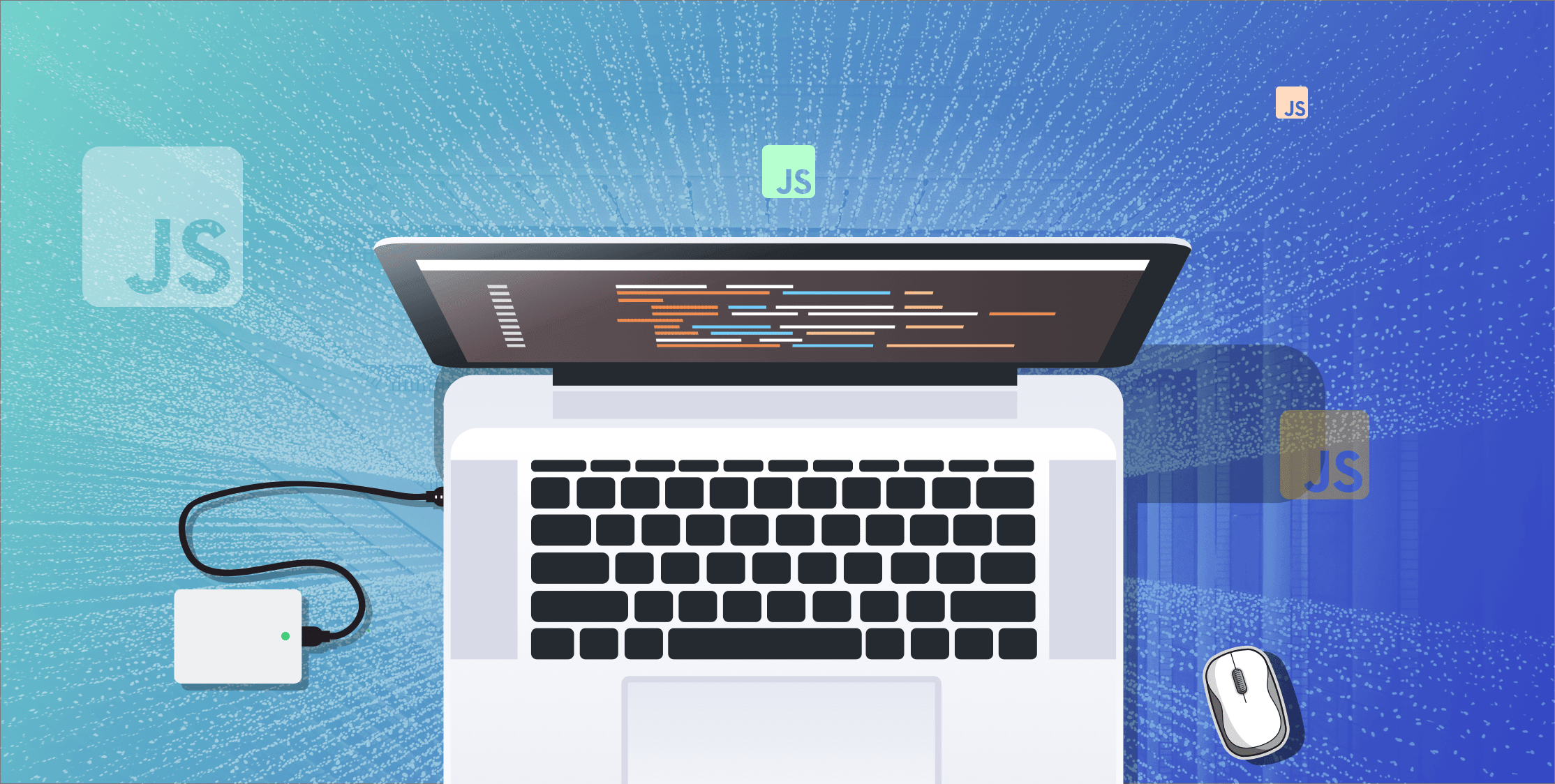
The localStorage object is one of the most widely used objects in web programming. It provides a simple solution for storing key-value pairs locally on the user’s computer.
Most web developers love the localStorage API for its straightforward syntax and ability to store up to 5MB of data.
On top of that, the latest version of all major browsers supports the Web Storage API, which includes both localStorage and sessionStorage. Only Opera Mini doesn’t support the webstorage API.
You can quickly verify if your browser supports the webstorage API by opening the Chrome DevTools. Navigate to the “Console”, type the below code snippet, and hit enter.
typeof(Storage)
If you receive an undefined result, your browser doesn’t support the webstorage API. If your browser supports it, then you should see “function” as a result.
This article explores the following questions:
- What is localStorage?
- What’s the difference between localStorage and sessionStorage?
- How to perform CRUD operations using the localStorage API
- What are common localStorage pitfalls?
- What are the limitations of localStorage?
What is localStorage?
As mentioned in the introduction, the localStorage object is part of the web storage API the browser supports natively. It’s a simple yet effective key/value storage solution.
For web developers, the most significant benefit of using the localStorage object is persistent offline storage. On top of that, we don’t lose data when a user closes their browser or restarts their computer. Even after a computer restart, a website can still read the data stored locally on the user’s computer using the localStorage API.
This solution provides web developers with a couple of interesting use cases.
- Store user settings offline for a website, such as enabling dark mode for YouTube
- Remember user search history
- Remember items in shopping cart
Next, let’s compare localStorage and sessionStorage.
localStorage vs. sessionStorage: What’s the difference?
While both APIs are seemingly identical, there are small differences in the way they behave.
The localStorage API stores data locally. Therefore, locally saved data doesn’t get lost when the user refreshes a tab, closes their browser, or restarts their computer. It’s an ideal storage solution to store essential data long-term, such as user preferences.
The sessionStorage API, on the other hand, survives a page refresh but only works in the same tab. Imagine YouTube using the sessionStorage API to store user preferences. You open the YouTube application in one tab and enable dark mode. YouTube will store this user preference in your browser’s sessionStorage. Let’s continue with opening a new tab in the same browser window. When navigating to the YouTube application, you’ll be presented with a white screen again as the sessionStorage object works per browser tab.
In brief, be mindful when picking a storage solution for your application needs. For example, it’s a best practice to store user preferences in localStorage. In contrast, sessionStorage works best for storing data for a specific session. For example, temporarily storing shipping information during the checkout process so the user can return to the product search if they change their mind.
Experimenting with CRUD operations for localStorage
This section shows you how to use the localStorage API to add, read, update, or remove items. On top of that, I’ll show you a useful trick to clear the entire localStorage for a specific page.
First, let’s create a new key-value pair item in our localStorage object. The setItem
function accepts a key and its value. Choose a purposeful name for your key as you’ll use the key name to retrieve the object again.
localStorage.setItem(‘my-key’, ‘some-value’)
Let’s retrieve our newly created object again using its key now.
let item = localStorage.getItem(‘my-key’)
console.log(item) // Output: “some-value”
That’s simple.
Ok, let’s continue with updating the value for our my-key
item. Note that we use the same setItem
function to overwrite its value.
localStorage.setItem(‘my-key’, ‘new-value’)
Lastly, let’s delete this key as it was a mistake. The removeItem
function accepts one argument, which is the key of the item you wish to delete.
localStorage.removeItem(‘my-key’)
Awesome! To make sure we’ve removed all keys, let’s use the clear
function to clear everything that’s stored in localStorage for our application.
localStorage.clear()
Done! We are ready for more advanced localStorage operations.
Advanced localStorage operations: Iterating over items
Let’s take a look at alternative methods for looping over the localStorage object and finding keys.
The first method uses the most straightforward for-loop. Note that we can use the length
property directly on the localStorage object.
for(let i=0; i<localStorage.length; i++) {
let key = localStorage.key(i)
console.log(`${key} with value ${localStorage.getItem(key)}`)
}
We can also use the i
value to directly retrieve the corresponding key using the key
method.
for (let i = 0; i < localStorage.length; i++){
let key = localStorage.key(i)
console.log(key)
}
That’s it! Let’s take a look at common pitfalls to avoid when using the localStorage API.
Common localStorage Pitfalls
Let’s take a look at the two most common pitfalls when interacting with the localStorage API.
First of all, trying to store a JSON object. The localStorage API is designed as key-value pair storage. Therefore, the value
only accepts strings, not objects. However, that doesn’t mean we can’t store an object. We need to serialize it to a string.
const dinner = { apples: 5, oranges: 1 }
localStorage.setItem(‘my-dinner’, JSON.stringify(dinner))
When reading the stringified object, we need to parse it again to JSON.
let dinner = JSON.parse(localStorage.getItem(‘my-dinner’))
Secondly, trying to store a boolean. Again, the localStorage API only supports strings. Be careful when storing booleans. Luckily, the solution is similar to storing a JSON object. When storing a boolean, the setItem
function will convert it to a string like this - “true”.
To read the stringified boolean, we can use the JSON.parse
method to convert it back to a boolean.
let myBool = JSON.parse(localStorage.getItem(‘my-bool’))
localStorage limitations you should know
Here’s a quick recap of the limitations of localStorage.
- String-based storage only
- Limited storage up to 5 MB for most browsers
- Synchronous storage solution, which means it blocks the main thread when trying to store huge strings. Make sure not to update the same key simultaneously, as that will lead to mutability issues. In this case, it’s better to seek alternative storage solutions, such as state management solutions, because the localStorage API is not designed for this purpose.
- Web workers or service workers can’t access localStorage
- No built-in security mechanisms. Therefore, we don’t recommend storing passwords or authentication-related data. Anyone who can access a user’s browser can open a page and read the information stored in localStorage like a publicly available computer in a library.
That’s it! If you found this article helpful, make sure to share it.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.