Understanding and Using Abort Controllers in JavaScript
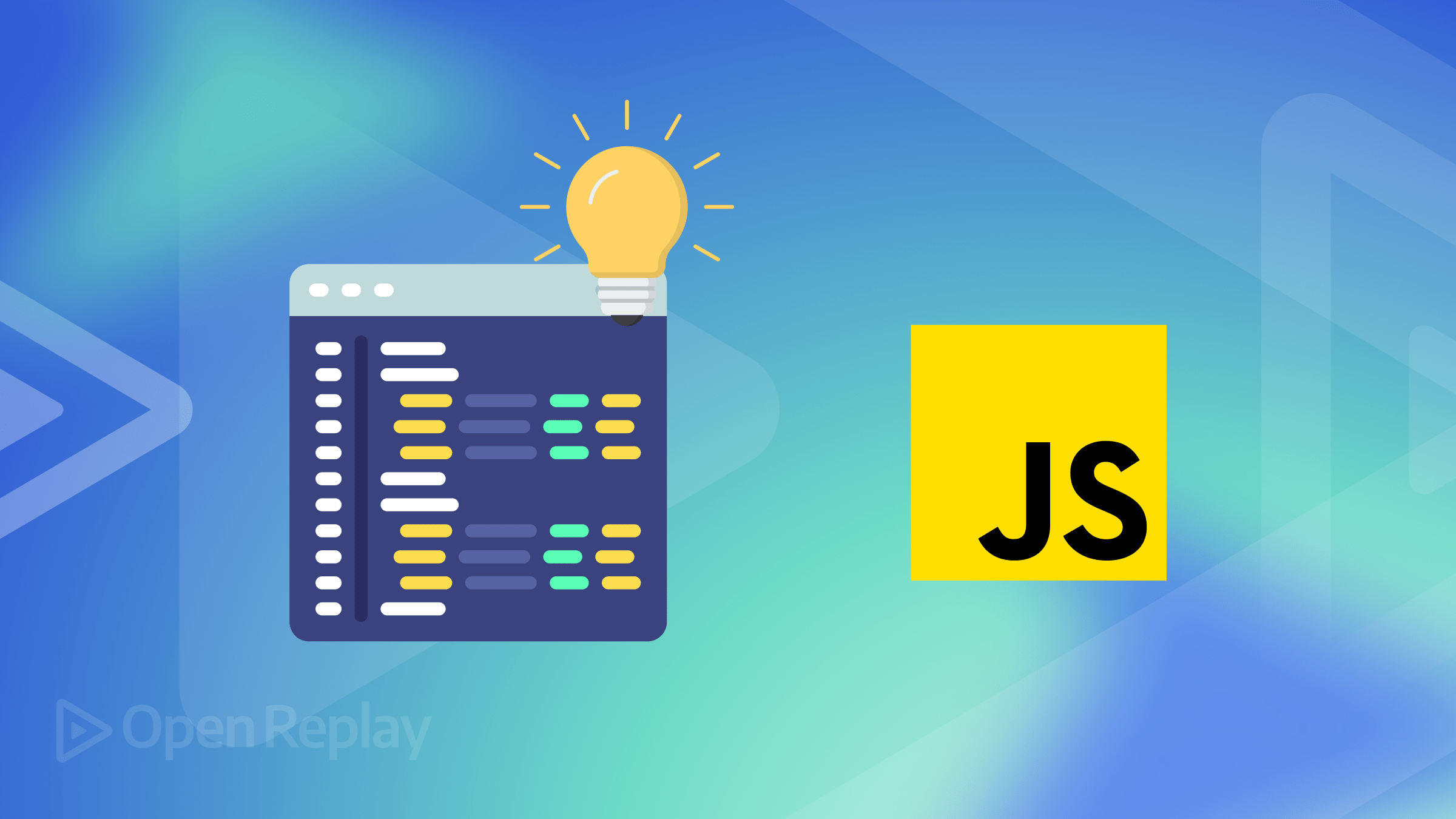
In modern web development, managing asynchronous tasks is crucial for creating responsive and efficient applications. Asynchronous operations, such as fetching data from a server or executing time-consuming computations, often require the ability to cancel or abort them before completion. Here, Abort Controllers come into play, as this article will show.
Discover how at OpenReplay.com.
Abort Controllers are a recent addition to the JavaScript language introduced as a part of the DOM
(Document Object Model) specification. They provide a means to cancel asynchronous tasks. Although primarily used with fetch
requests, they can also work with other asynchronous operations, such as setTimeout
or setInterval
functions.
An Abort Controller is created by instantiating the AbortController class
as shown below:
const controller = new AbortController();
The controller
object has a method named abort()
that can be called to cancel an associated asynchronous task. To associate the controller with an asynchronous operation, you pass its signal
property as an option when initiating the asynchronous operation. For example, with a fetch
request, we implement it as shown below:
const controller = new AbortController();
fetch("https://api.example.com/data", { signal: controller.signal })
.then((response) => {
// Process the response
})
.catch((error) => {
//handle aborted request
if (error.name === "AbortError") {
console.log("Request was aborted");
} else {
//handle other errors
console.error("Error occurred:", error);
}
});
To cancel the fetch
request, you can call the abort()
method on the controller as shown below:
controller.abort();
Some advantages of using Abort Controllers include the following:
- Improved User Experience: Allows efficient management of multiple unexpected user interactions with asynchronous events, such as repeatedly clicking the submit form button.
- Network Efficiency: Helps reduce unnecessary network traffic by canceling pending requests that are no longer needed or are taking longer than expected to resolve.
- Cleaner Code: Provides a standardized way to handle request cancellation, resulting in cleaner and more maintainable code.
Demo on using the Abort Controller
In this section, we will practice implementing abort controllers on various asynchronous events, such as AJAX
requests, and with native asynchronous Javascript functions like setTimeout
or setInterval
.
Using Abort Controller with Fetch API
In this simple demo, we will walk through a practical example of using an Abort Controller to cancel a fetch
request. Suppose we have two buttons in our web application, one that would initiate a fetch request to load some data and another that would terminate that initial request. We can couple this functionality as shown in the code example below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<button id="loadDataBtn">Load Data</button>
<button id="abortFetchBtn">Abort Fetch</button>
<script>
const controller = new AbortController();
const loadBtn = document.getElementById("loadDataBtn");
const abortBtn = document.getElementById("abortFetchBtn");
const getData = (abortSignal) => {
fetch("https://api.example.com/data", { signal: abortSignal })
.then((response) => response.json())
.then((data) => {
// Process the data
})
.catch((error) => {
if (error.name === "AbortError") {
console.log("Request was aborted");
} else {
console.error("Error occurred:", error);
}
});
};
const cancelFetchRequest = () => {
controller.abort();
};
loadBtn.addEventListener("click", () => {
getData(controller.signal);
});
abortBtn.addEventListener("click", () => {
cancelFetchRequest();
});
</script>
</body>
</html>
In this example, clicking the Load Data
button initiates a fetch
request. If the user wants to cancel the request before it completes, they can do so by clicking the Abort Fetch
button, which calls the’ cancelFetchRequest ()function and aborts the associated
fetch` request.
Using Abort Controller with setTimeout
and setInterval
Let us start by looking at an example using the setTimeout
function.
// Create an AbortController instance
const controller = new AbortController();
const signal = controller.signal;
const timeoutId = setTimeout(() => {
console.log("Timeout completed");
}, 5000);
// If the abort signal is triggered, clear the timeout
signal.addEventListener("abort", () => {
clearTimeout(timeoutId);
console.log("Timeout aborted");
});
// Set a timeout to abort the operation after 3 seconds
setTimeout(() => {
controller.abort();
}, 3000);
This simple example shows us how we could associate a setInterval
call with an Abort Controller and use the abort controller to terminate the setInterval
call. A similar approach works for the setTimeout
function. An argument immediately comes to mind that this could be implemented by passing the timeoutId
to the clearTimeout
function without the added complexity of using the Abort Controller. This holds quite true for a single request.
However, in more realistic scenarios seen in the developer space, developers often find themselves managing multiple asynchronous events and functions; in this case, the Abort Controller provides a standardized and more predictable approach to managing these events. This scenario is illustrated in this example using the setInterval
function and will be further explained in upcoming sections.
// Create an AbortController instance
const controller = new AbortController();
const signal = controller.signal;
// Array to store interval IDs
const intervalIds = [];
// Function to create and start intervals
const startIntervals = () => {
for (let i = 0; i < 5; i++) {
const intervalId = setInterval(() => {
console.log(`Interval ${i + 1} tick`);
}, (i + 1) * 1000); // Interval duration increases with each interval
intervalIds.push(intervalId);
}
};
// Start the intervals
startIntervals();
// If the abort signal is triggered, clear all intervals
signal.addEventListener("abort", () => {
intervalIds.forEach((id) => clearInterval(id));
console.log("Intervals aborted");
});
// Abort the operation after 7 seconds
setTimeout(() => {
controller.abort();
}, 7000);
Use cases for Abort Controllers
Now that we have covered the basics of Abort Controllers let us explore some real-world scenarios where they can be incredibly useful.
Debouncing Events
Debouncing is a technique for limiting the rate at which a function is called, typically in response to user input events such as typing or resizing. When implementing debouncing, you often want to cancel any pending function calls if the event occurs again before the function is completely executed. Abort Controllers provide a convenient way to achieve this behavior. You can associate each event listener with an Abort Controller and abort the previous call whenever the event occurs again, ensuring that only the latest call is executed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Debouncing Example with AbortController</title>
</head>
<body>
<form>
<input id="inputField" placeholder="Type something..." />
</form>
<script>
const formInput = document.getElementById("inputField");
let abortController = null;
// Function to perform a debounced operation
const debounceOperation = () => {
const controller = new AbortController();
const signal = controller.signal;
// Perform the asynchronous operation here, such as fetching data
fetch("https://api.example.com/data", { signal })
.then((response) => response.json())
.then((data) => {
// Process the data
console.log("Debounced operation completed:", data);
})
.catch((error) => {
if (error.name === "AbortError") {
console.log("Debounced operation was aborted");
} else {
console.error(
"Error occurred during debounced operation:",
error
);
}
});
};
// function to debounce user input events
const debounceEvent = () => {
// If there is an ongoing debounced operation, abort it
if (abortController) {
abortController.abort();
}
// Create a new AbortController for the current operation
abortController = new AbortController();
const signal = abortController.signal;
// Start a new timeout for the debounced operation
setTimeout(() => {
debounceOperation();
abortController = null; // Reset the Abort Controller; is not necessary for this implementation since the debounce function is attached to a key-up event
}, 500); // Adjust the debounce delay as needed
};
// Example: Debouncing a key-up event
formInput.addEventListener("keyup", debounceEvent);
</script>
</body>
</html>
The code snippet demonstrates an implementation of debouncing a user input event using an Abort Controller in JavaScript. When a user types in the input field (inputField
), a keyup
event listener triggers the debounceEvent
function. Inside this function, if there is an ongoing debounced operation (tracked by the abortController
variable), it aborts the previous operation. Then, it creates a new Abort Controller instance and associates it with the current operation. After a specified delay (500 milliseconds in this case), it executes the debounceOperation
function, which performs an asynchronous operation (in this case, fetching data from an API
). If the operation completes within the delay, the data is processed; otherwise, an appropriate message is logged to the console if it’s aborted due to a subsequent event. This approach ensures that only the latest event triggers the operation, effectively debouncing user input events to prevent unnecessary and repetitive function calls.
Long-Polling and Server-Sent Events
In applications where real-time updates are crucial, such as chat applications or live sports scores, long-polling or server-sent events (SSE) are commonly used to maintain a persistent connection with the server. However, there may be scenarios where the client may have to terminate the connection prematurely, such as navigating away from the page or closing the browser tab. Abort Controllers allow you to gracefully close these connections by aborting the associated requests, preventing unnecessary resource consumption on both the client and server sides.
User Interaction Management
In web applications, users often trigger multiple asynchronous actions through interactions like button clicks, form submissions, or dropdown selections. Often, users get impatient and could trigger these asynchronous events repeatedly and in an unexpected, random other that does not follow the flow of our web application.
With the aid of Abort Controllers, we can manage these asynchronous actions effectively by allowing the cancellation of ongoing requests when a new interaction occurs. This technique ensures that only the most recent action is processed, preventing potential conflicts or unwanted behavior caused by outdated requests. This approach is quite similar to debouncing but on a much broader scale for multiple seemingly unrelated asynchronous events.
Asynchronous Task Control
-
Search Suggestions Imagine you’re building a search feature for a website where users can type in a query and receive real-time suggestions. You might use an asynchronous request to fetch these suggestions from a server as the user types. However, if the user rapidly changes their query, you don’t want to waste resources fetching outdated suggestions. Abort Controllers come to the rescue here. You can associate each request with an Abort Controller and abort previous requests whenever the user’s query changes, ensuring that only the latest request is processed, improving efficiency and responsiveness.
-
Infinite Scrolling Infinite scrolling is a pattern used in many web applications to load more content as the user scrolls along a page. Multiple fetch requests might be initiated to load additional data as the user scrolls quickly. However, those pending requests become unnecessary if the user suddenly scrolls back to the top or navigates away from the page. With Abort Controllers, you can cancel these requests when they are no longer needed, preventing unnecessary network traffic and freeing up resources.
-
Form Submissions When submitting a form asynchronously, such as when posting a comment on a blog or submitting user feedback, you want to provide a smooth experience for the user. Suppose the user decides to cancel the submission midway through, perhaps by navigating away from the page or clicking a cancel button. In that case, you can use an Abort Controller to cancel the submission request. This ensures no unnecessary data is sent to the server and prevents any potential side effects of the incomplete submission.
Integrating Abort Controllers with Reactive Frameworks
Reactive programming has gained significant popularity in recent years due to its ability to handle asynchronous data streams and events in a more predictable and manageable way. Many JavaScript frameworks, such as React.js
, Vue.js
, and Angular.js
, support reactive programming paradigms.
These popular frameworks support a form of application rendering popularly called SPAs
where a shell
(an empty page) is first loaded, and content is progressively and dynamically added to the page using JavaScript and fetch requests. This approach works great until a user begins to interact with your application and navigate to various pages. Due to the nature of these applications, there is a risk of a memory leak while running AJAX requests
as a request which is valid for content on one page will keep running in the background even though that page has been swapped out of view and another page is in view.
This issue inevitably leads to a slower-performing application and is indeed one of the areas where abort controllers truly shine as an optimal solution. Using abort controllers to terminate any pending requests on a particular page as a cleanup function, we can fix such leaks and have a smoother and faster application. This implementation is demonstrated in the code snippets below for both React.js
and Vue.js
frameworks.
React.js
Demo
In React applications, managing asynchronous operations often involves hooks such as useState
and useEffect
. When making AJAX
requests, particularly with the aid of the useEffect
hook, Abort Controllers are invaluable. You can leverage these hooks to create more responsive, manageable, and cancellable operations, helping you facilitate appropriate page cleanup.
import React, { useState, useEffect } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.example.com/data', { signal })
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Request was aborted');
} else {
console.error('Error occurred:', error);
}
setLoading(false);
});
return () => {
controller.abort();
};
}, []); // Empty dependency array ensures the effect runs only once
return (
<div>
{loading ? <p>Loading...</p> : <p>{data}</p>}
</div>
);
}
export default MyComponent
In the code snippet above, a fetch
request is made using the useEffect
hook once MyComponent
is mounted. However, before the MyComponent
is unmounted, the Abort Controller is used within the cleanup function of the useEffect
to cancel any ongoing requests.
Vue.js
Demo
In Vue.js, you can handle asynchronous operations using the mounted lifecycle hook or watch properties. Similarly, Abort Controllers can be integrated into Vue components to manage fetch requests and other asynchronous tasks.
<template>
<div>
<p v-if="loading">Loading...</p>
<p v-else>{{ data }}</p>
</div>
</template>
<script>
export default {
data() {
return {
data: null,
loading: true,
controller: null
};
},
mounted() {
this.controller = new AbortController();
const signal = this.controller.signal;
fetch('https://api.example.com/data', { signal })
.then(response => response.json())
.then(data => {
this.data = data;
this.loading = false;
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Request was aborted');
} else {
console.error('Error occurred:', error);
}
this.loading = false;
});
},
beforeDestroy() {
if (this.controller) {
this.controller.abort();
}
}
};
</script>
In the code snippet above, a fetch
request is made when the Vue
component is mounted. However, before the component is unmounted, the Abort Controller is used in the beforeDestroy
function to cancel any pending request.
By incorporating Abort Controllers into reactive frameworks like React, Vue.js, and Angular, developers can seamlessly integrate cancellation logic into their asynchronous operations and help solve the issue of stale tasks running in the background.
Advanced Techniques and Best Practices
These advanced techniques and best practices, discussed below, will help optimize the use of Abort Controllers in web applications, ensuring efficient request management, robust error handling, efficient performance, and broad browser support.
Aborting Multiple Requests
In complex web applications, there may be situations where multiple asynchronous requests need to be aborted simultaneously, such as when a user navigates to a new page or initiates a batch action.
To tackle this peculiar challenge, start by maintaining a collection (e.g., an array) of AbortControllers
, each associated with an individual asynchronous request. When the need arises to abort multiple requests, iterate through the collection and call abort()
on each controller. This technique ensures that all ongoing requests are canceled efficiently. The code implementation is shown below:
// Array to hold AbortControllers
const abortControllers = [];
// function to create and start a new asynchronous request
const startNewRequest = () => {
const controller = new AbortController();
const signal = controller.signal;
fetch("https://api.example.com/data", { signal })
.then((response) => response.json())
.then((data) => {
// Process the data
})
.catch((error) => {
// Handle errors
});
// Add the controller to the array
abortControllers.push(controller);
};
// Function to abort all ongoing requests
const abortAllRequests = () => {
abortControllers.forEach((controller) => {
controller.abort();
});
};
// Example usage:
startNewRequest(); // Start the first request
startNewRequest(); // Start another request
// Somewhere in your application, when the need arises to abort all requests (e.g., when navigating to a new page):
abortAllRequests();
Error Handling and Cleanup
Handling errors gracefully and performing cleanup tasks when aborting requests is crucial to maintain application stability and prevent memory leaks. We should always implement robust error-handling mechanisms within the fetch or asynchronous function callbacks. Handle specific error types, such as abort, network, or server errors, appropriately. Additionally, perform any necessary cleanup tasks, such as closing connections, releasing resources, or updating UI state, to maintain consistency and recover gracefully from aborted requests. This implementation is shown below:
// function to perform an asynchronous request
const fetchData = () => {
const controller = new AbortController();
const signal = controller.signal;
fetch("https://api.example.com/data", { signal })
.then((response) => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.json();
})
.then((data) => {
// Process the data
})
.catch((error) => {
if (error.name === "AbortError") {
console.log("Request was aborted");
} else {
console.error("Error occurred:", error.message);
}
})
.finally(() => {
// Perform cleanup tasks
// For example, close connections, release resources, or update UI state
console.log("Cleanup tasks performed");
});
// Function to abort the request
const abortRequest = () => {
controller.abort();
};
// Example usage:
// setTimeout(abortRequest, 5000); // Abort the request after 5 seconds
};
// Start the asynchronous request
fetchData();
Browser Support and Polyfills
Although most modern browsers, including Chrome, Firefox, Safari, and Edge, support Abort Controllers, older browser versions may lack support, potentially affecting cross-browser compatibility.
To tackle this drawback, feature detection should be used to determine if Abort Controllers are supported in the user’s browser. If not supported, consider using a polyfill library, such as abortcontroller-polyfill
, which provides a compatible implementation of the AbortController interface. Include the polyfill in your project to ensure consistent behavior across different browser environments, allowing you to leverage the benefits of Abort Controllers while maintaining broad compatibility.
Summary
Abort Controllers offer a powerful mechanism for managing asynchronous tasks in JavaScript, allowing developers to gracefully cancel ongoing operations. This guide to using abort controllers explores the fundamentals of Abort Controllers, including their creation, usage with fetch requests, and integration with other asynchronous functions like setTimeout and setInterval. It also delves into advanced techniques and best practices, covering Abort Controllers with reactive frameworks, error handling, cleanup, and browser support considerations.
References
- MDN Web Docs: AbortController
- MDN Web Docs: Using Fetch - Aborting Fetch
- Clean Up Async Requests in
useEffect
Hooks
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.