Alternatives to React: Preact
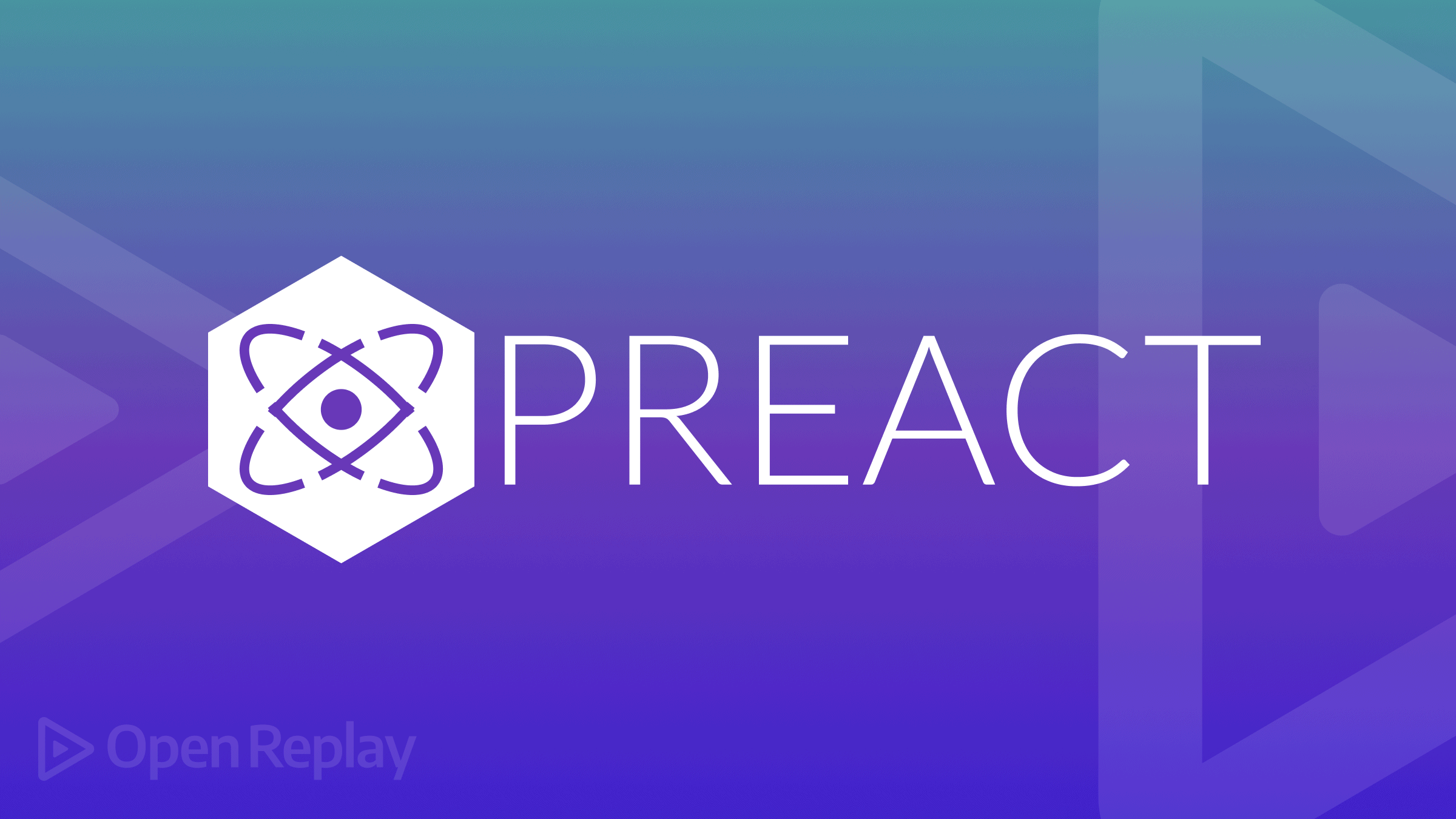
Alternatives to React is a series of articles looking at different JavaScript front-end frameworks. All the examined frameworks are like React in syntax and operation, but they may have benefits React doesn’t provide. The first two articles in the series were Alternatives to React: Solid JS and Alternatives to React: Inferno JS, with more to come.
Introduction
Preact is a front-end library for building User Interfaces (UI) just like React. According to the official documentation website, Preact is a “Fast 3kb alternative to React with the same Modern API”.
A quick look at the features of Preact shows that it is almost the same as React, considering that it uses a virtual DOM, context, hooks, etc.
In this article, we will deeply dive into the world of Preact. We would learn why the library was created, how it works, and how it compares to React. We would also discuss if we should ever use Preact.
Before proceeding, note that this article is not a tutorial on starting Preact. Preact provides an excellent tutorial on their website. Instead, this article compares React and Preact to aid you in making informed decisions on the framework to choose for a project.
On that note, let us begin.
Origin of Preact
Jason Miller, a Canadian software developer, is the author of Preact. In a talk titled “Putting the P in Preact”, Jason stated that he had built a lot of UI frameworks/templating engines for the web.
Jason could never build a perfect framework that supported everything he wanted. He kept having issues relating to the Document Object Model (DOM). He then discovered that JSX and React satisfied his need and what he wanted in a framework.
When Jason found this solution, his next step was learning React. To learn React, he needed to create a project that worked how React worked. He learned better when he was building.
Preact started as a Codepen file that rendered hundreds of DOM elements like React would. Jason kept optimizing the program and adding features. One day he ran speed tests on desktop and mobile for his program. The results he got were very good, and they were way faster than React. The speed was very close to that of plain JavaScript.
According to the talk “Putting the P in Preact”, Jason continued with the project. He made it into a library for developers who couldn’t afford the high cost of performance in React. Preact had almost the same features as React, just lighter and faster. The first release of the library on Github (v2.0.0) is dated back to November 13th, 2015.
Since then, the Preact community has been growing with users, contributors, and maintainers.
How does Preact work?
Preact works very similarly to React, but it still has its separate implementation of many of React’s features. In this section of the article, we will look at Preact’s core functionality, breaking it down into three categories. These categories are:
- Virtual DOM
- No Build Tools
- Embeddability
Virtual DOM
The Document Object Model (DOM) represents the hierarchy of elements in any HTML document. Every element on a web page can also be located on the DOM. On the other hand, the virtual DOM is a generated clone of the real DOM.
Most frameworks use virtual DOMs because the real DOM is slower to render when updated with JavaScript. This is a big issue for Dynamic applications because they need to update their elements constantly.
According to Codecademy, Virtual DOM-enabled rendering in React goes through the following phases:
- The whole of the Virtual DOM gets updated.
- The Virtual DOM gets compared to what it looked like before the update. React figures out the changed elements (This process is also known as Diffing).
- React updates only the changed elements on the real DOM (This process is also known as reconciliation).
- User sees updated change on the page.
Preact doesn’t implement its virtual DOM like React. On the documentation website, it states that:
“Preact provides the thinnest possible Virtual DOM abstraction on top of the DOM. It builds on stable platform features, registers real event handlers, and plays nicely with other libraries.” (Source: Preact)
In simple terms, this means that Preact’s virtual DOM only handles processes the real DOM would find time-consuming. Preact efficiently uses a virtual DOM, unlike React, which gives every task to the virtual DOM.
Preact also doesn’t use Synthetic events, which are an abstraction of a normal DOM Event Object. Instead, Preact uses the real event handlers of elements and their corresponding event objects. This makes Preact’s virtual DOM smaller and more performant.
No Build Tools
Build tools are libraries that perform recurring tasks for a developer and save time. These kinds of tasks include CSS Preprocessing, code linting, trans-compiling files, bundling files, compressing images to mention but a few. Some examples of build tools are Parcel, Webpack and Browserify. React JS, like other front-end frameworks, relies on build tools under the hood to create optimal production output after development.
Even though build tools help during development, they might increase the complexity of projects. The developer might have to deal with configuration files or simply spend time learning how to use a new tool.
Preact is shipped as an already working solution and does not need a build tool to function properly.
A simple Preact App can be built by importing the preact
library into an HTML page.
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Preact App</title>
</head>
<body>
<!-- PREACT APP -->
<script type="module">
import { h, Component, render } from 'https://unpkg.com/preact?module';
// Create your app
function App() {
return h('h1', null, 'Hello World!');
}
render(App(), document.body);
</script>
</body>
</html>
(Snippet modified from Preact Website)
From the above illustration, because App
is a javascript function that returns an h1
element, the function needs to be called as an argument of the render function.
With app
declared as a variable, this works too.
<script type="module">
import { h, Component, render } from 'https://unpkg.com/preact?module';
// Create your app
const app = h('h1', null, 'Hello World!');
render(app, document.body);
</script>
However, a build step is required to use JSX for DOM elements in Preact. Preact provides an alternative to JSX called htm
with similar functionality and syntax but doesn’t require a build step. We will look at htm
later in this article. Preact doesn’t need a build tool to get the best out of it.
Embeddability
HTML has an iframe
tag that enables a particular website to be displayed (embedded) on another. The importance of embedding websites on other websites is high. Great use of this is to display data that is not originally supported on the host website (videos, games, music, maps, and so on). Embedded Web Apps that have functionality are called Widgets.
Below is a Todo Widget built with Preact.
Preact was built with ease of embeddability, which is why Preact Applications can fit into any website and load as fast as possible.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
How does Preact compare to React?
In this article section, we will compare the two frameworks using some criteria. These criteria are essential when picking a framework/library for a project. They are:
- Syntax
- Size
- Speed
- Popularity
Syntax
Comparing the syntaxes of Preact and React, below are some differences.
- Passing of State and Props to the Render method: As mentioned earlier, the
render
method of class components can accept props and states. A representation of this is found below. In React, therender
method does not accept any parameters.
render(props, state) {
// Return Component
}
- Use of
class
instead ofclassName
in components: As we can see below, we can useclass
instead ofClassName
in Preact (className
is also accepted).
function Hello(){
return (
<h1 class='hello-box'>Hello World!</h1>
);
}
-
Components: In Preact, developers can create components in three different ways. They are:
- Using JSX. JSX is the most common way to render components in Preact.
function JsxComponent(props) {
return (
<h1>I am rendered with JSX</h1>
)
}
- Using
h
orcreateElement
.CreateElement
andh
are functions that return virtual DOM elements in Preact. React developers can usecreateElement
in Preact as it is modeled afterReact.createElement
.
import { h } from 'preact';
function HComponent(props) {
return h('h1', null, 'I an rendered with h')
}
- Using
htm
.htm
is the recommended way of creating components in Preact. This is because usingh
orcreateElement
can be tedious. Also, JSX needs to compile to standard JavaScript before it works.htm
combines the power ofh
and the ease of JSX.
import { h, render } from 'preact';
import { htm } from 'htm';
// Initialize htm with Preact
const html = htm.bind(h);
function HtmComponent(props) {
return (
html`<h1>I am rendered with HTM</h1>`
)
}
React developers can only create components in two ways: using JSX and using React.createElement
. A conclusion to draw here is that there is slightly more flexibility in creating Preact Components.
Size
As stated on Preact’s website, it is a library with a tiny size of 3kb. Bundlephobia reports that Preact’s size, minified and gzipped, is 4kb.
An npm package named package-size
also confirms this.
A possible reason is that newer updates have increased the source code size. However, Preact has always maintained the under 4kb size by periodically removing deprecated features or dedicating some APIs to separate modules.
It is still impressive for Preact to be that small, considering that using React requires over 40kb of memory. A React Application requires react
and react-dom
with sizes of 2.5kb and 42kb respectively (Minified + Gzipped).
Speed
Speed is a huge factor to consider when choosing a framework. We would compare Preact to React using Stefan Krause’s Javascript frameworks benchmark. The experiment tests frameworks on three categories. These categories are:
-
Duration: The duration category determines the number of milliseconds it takes javascript frameworks to render or update changes on a page. The tasks assigned to the frameworks here include creating 1,000 rows of a table, updating all 1,000 rows of the table, appending 1,000 rows to a table of 10,000 rows, and so on. While React appeared to be fast in the latest experiment published before this article, Preact beat React on average in this category. This means that, on average, Preact updates the DOM faster.
-
Startup metrics: These are taken with Lighthouse and simulate the website on a mobile device. It runs tests on how the frameworks properly utilize the CPU. This category also measures the build sizes of assets of a framework in production. Both frameworks performed well here, but Preact still beat React.
-
Memory Allocation: This measures the amount of memory a framework uses while in session on a browser. This memory is measured in Megabytes (MB). In this category, things like memory usage after page load, memory usage after adding 1000 rows, memory usage after creating 1000 rows five times, and so on are measured. On average, Preact performed slightly better than React utilizing lower memory in all scenarios. An article that goes in-depth on the interpretation of the benchmark can be found here on this blog post.
Popularity
One final factor we are going to consider is popularity. How prevalent are the frameworks compared to each other? The popularity determines the amount of help a developer would receive when they get stuck on a project.
In the last State of JS survey conducted before this article, Preact came sixth (80%) in awareness as a front-end framework. Most respondents had heard of the framework, even though React beat Preact.
In usage, it turned out that only 14% of the respondents use Preact. React also beat Preact in this category.
Preact happened to be falling in interest and satisfaction every year from the charts.
A curve like this isn’t a good one for a framework, but the interest and satisfaction of React are dropping too. Still, millions of websites are powered by React today. So I don’t think this is something to worry about too much.
The following holds for both frameworks in terms of NPM Weekly downloads and GitHub stars.
- Preact
- Github Stars: 32K
- NPM Downloads: 1.4M
- React
- Github Stars: 191K
- NPM Downloads: 16.2M
Conclusion
In this article, we have looked at Preact as an alternative to React. We started by finding out how the framework came to be, and then we looked at the features of the framework. We also compared the framework to React in some categories.
The small size and speed Preact has is truly an advantage over the relatively giant-sized React and React DOM. This advantage is what has attracted Dev.to, Etsy, Smashing Magazine, Uber and many other popular organizations to add Preact to their stack.
You should use Preact if you want to make a new Single Page Application more lightweight or if you want to build a dynamic widget for a webpage. If the application already exists and you would still like to move to Preact, Preact provides a feature called preact-compat
to do so.
Resources
A TIP FROM THE EDITOR: You may be interested in reading An introduction to Fresh, a framework that uses Preact for rendering and templating.