Authenticating Vue Apps with Okta
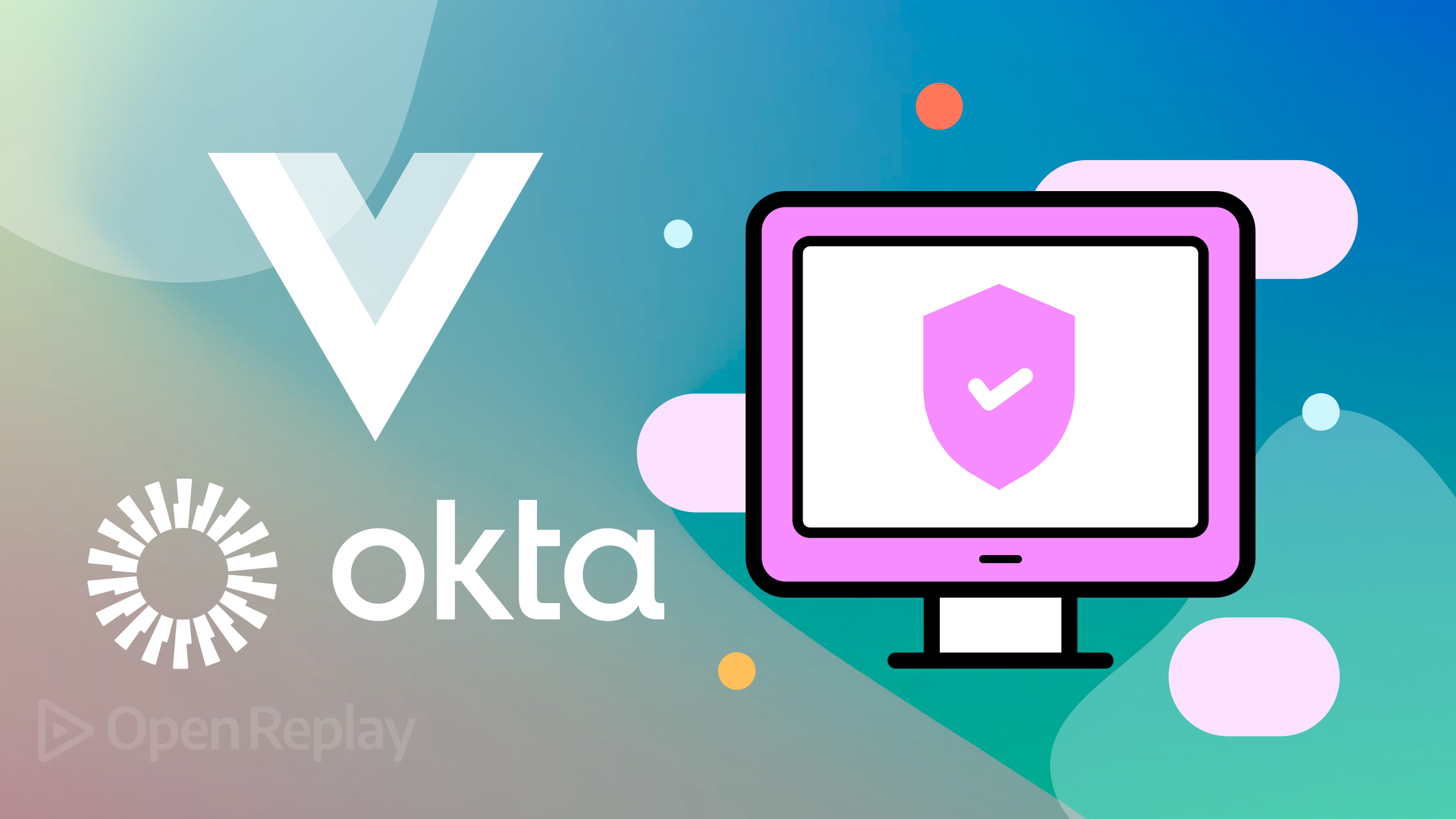
Securing user access and data privacy is paramount in today’s digital landscape. In Vue.js applications, authentication is pivotal in safeguarding user identities and enhancing application security. As developers strive to create seamless user experiences, effective authentication mechanisms are essential to streamline login processes, enable Single Sign-On (SSO), and efficiently manage user access. This article delves into authentication in Vue apps, exploring how implementing robust authentication solutions like Okta can resolve challenges related to user identity, data protection, and seamless user journeys.
Discover how at OpenReplay.com.
Okta is a cloud-based platform for authentication and authorization. In contrast to other identity platforms, Okta seamlessly integrates with both on-premises and cloud apps.
Okta is a valuable choice for authentication due to its robust security features, enabling secure user access. It streamlines the authentication process through Single Sign-On (SSO), enhancing user experience and reducing password management burden. Okta’s developer-friendly SDKs simplify integration into Vue applications, ensuring efficient implementation. Its support for identity federation and compliance aids seamless integration and adherence to regulations.
Setting up Okta
Let’s get started with creating an Okta account. Click on this link to create one. After creating your Okta account, you will be redirected to the admin dashboard. On the admin dashboard, click on the “Applications” tab and then on “Create App Integration.”
Next, select “OIDC - OpenID Connect,” then click “Single-Page Application” and Next.
Next, add your app integration name and logo(optional), then leave everything else at default.
Next, scroll down to the end of the modal and select “Allow everyone in your Next, scroll down to the end of the modal and select “Allow everyone in your organization to access,” then Save.
Finally, copy and save the Client ID and the Issuer Address at the dashboard’s top right corner.
Creating Okta Users. Now that the app is all set, let’s create some users that can interact with our app. Head to your Okta admin dashboard and click “Directory > People” in the sidebar. Next, click on “Add Person”.
Then, fill in the user’s details, as seen below, and save.
Setting up Work Environment
With the Okta Authenticator set up, let’s scaffold our Vue app using the Vue CLI tool. Run the command below in the terminal.
vue create Vue-auth
Next, Select the setup shown In the image below.
Installing Packages
We’ll use the Okta/okta-auth-js and the Okta/okta-vue package for this project. To install these, change the directory to the project folder and install the above packages using the command below.
cd vue-auth && yarn add @okta/okta-vue @okta/okta-auth-js
Or using Npm
cd vue-auth && npm i @okta/okta-vue @okta/okta-auth-js
Building Authentication UI
Let’s start building our Vue app. Head to the main.js
in the src/
folder and replace the code below.
/* eslint-disable */
import { createApp } from 'vue'
import { OktaAuth } from '@okta/okta-auth-js'
import OktaVue from '@okta/okta-vue'
import App from './App.vue'
import router from './router'
const oktaAuth = new OktaAuth({
clientId: `${Your_ClientId}`,
issuer: `https://${Your_Issuer_Address}/oauth2/default`,
redirectUri: `${window.location.origin}/login/callback`,
scopes: ['openid', 'profile', 'email']
});
createApp(App)
.use(router)
.use(OktaVue, { oktaAuth })
.mount('#app')
In the code above, we’re setting up the Okta authentication in our Vue.js application by configuring the OktaAuth
instance, registering the OktaVue
package, and setting up routing into the Vue DOM.
In the OktaAuth
object, we’re assigning our Client ID and Issuer Address that we copied from the Okta dashboard. We’ve also ensured the redirectUri
path is identical to the one on our Okta console.
Next, head over to the App.vue
in the src/
folder and replace the code in it with the code below.
<template>
<main id="app">
<router-view />
</main>
</template>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
#app {
padding: 3rem;
color: whitesmoke;
background-color: black;
height: 110vh;
}
button {
color: red;
box-shadow: 0 5px 8px 0 rgba(115, 115, 115, 0.24),
0 7px 20px 0 rgba(72, 72, 72, 0.19);
background-color: #4caf50; /* Green */
border: none;
color: white;
padding: 12px 28px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 19px;
}
</style>
In the code above, we render the Vue router components and create custom styles for our pages and button.
import { createRouter, createWebHistory } from "vue-router";
import { LoginCallback } from "@okta/okta-vue";
import HomeView from "@/views/HomeView";
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes: [
{
path: "/",
component: HomeView,
},
{
path: "/login/callback",
component: LoginCallback,
},
],
});
export default router;
In the code above, we’re rending the HomeView
component as our default and home component. Then, we display the LoginCallback
component from the okta-vue
package as our redirect Component when we log in. This component handles our login state by storing our login token in the Local storage.
Next, paste the code below and Create a HomeView.vue
app in the src/views/
folder.
<template>
<main>
<div v-if="!authState?.isAuthenticated">
<h1>Welcome To HomePage</h1>
<br />
<p>
🌟 Welcome to our Home Page! 🌟 We're excited to have you here. To get
started on your journey, simply click the button below to initiate the
secure login process. Our seamless integration with Okta ensures your
information is well-protected while granting you access to all the
amazing features that await you. Click to Login and Explore ➡️ 🚀🔐
</p>
<br />
<button>Login</button>
</div>
</main>
</template>
In the code above, we’re rending the welcome message and a Login button when the user is authenticated.
Authenticating App with Okta
With our setup complete, let’s write our authentication logic. Head to the HomeView.vue
file and paste the code below the </template>
tag.
<script>
export default {
name: "HomeView",
data: function () {
return {
userData: null,
userClaims: [],
};
},
async created() {
this.userDetails();
},
methods: {
async userDetails() {
if (this.authState?.isAuthenticated) {
const authToken = await this.$auth.tokenManager.get("idToken");
this.userArray = await this.$auth.getUser();
this.userClaims = Object.entries(authToken.claims).map(
([claimType, claimValue]) => ({
claimType,
claimValue,
})
);
}
},
async userLogout() {
await this.$auth.signOut();
},
async userLogin() {
await this.$auth.signInWithRedirect();
},
},
};
</script>
In the code above, we manage the user’s authentication and details using the Okta authentication service. When the component is created, the user details are fetched and assigned to the userData
and userClaims
values if the user is authenticated.
Finally, we also created the user’s login and logout functions.
Integrating Okta Auth Logic
Add the userLogin
function to our Login button in our HomeView
template.
<button id="login-button" v-on:click="login">Login</button>
Finally, let’s inject our auth logic into our template. For this, we’ll display a welcome message with the user’s name and a table containing the user’s login information. To achieve this, paste the code below into the <template>
tag below the first closing div
.
<div v-if="authState?.isAuthenticated">
<h1>Welcome {{ userData && userData.name }}!</h1>
<br />
<p>
🎉 Congratulations! 🎉 You're now successfully logged in to your
account. We're thrilled to have you as part of our community. Your
journey starts here, and we're here to support you every step of the
way. Below are your account details. 🔍 🌟🔐
</p>
<br />
<div>
<h1>User Information</h1>
<br />
<table class="detail-table">
<thead>
<tr>
<th>Properties</th>
<th>Value</th>
</tr>
</thead>
<tbody>
<tr v-for="(x, index) in userClaims" :key="index">
<td>{{ x.claimType }}</td>
<td :id="'id-' + x.claimType">{{ x.claimValue }}</td>
</tr>
</tbody>
</table>
<br />
<button class="logoutBtn" v-on:click="userLogout">Logout</button>
</div>
</div>
The code above displays user information when the user is authenticated. It includes a welcome message, user details in a table, and a logout button. Also, in the code above, we’re using Vue’s directives (v-if
, v-for
) to dynamically display the content based on the user’s authentication status and provided user claims.
To preview what we’ve done, run the command below in the project terminal.
vue serve
Conclusion
In this article, we’ve seen how to set up an Okta Single Page Application authentication and authenticate users into our application using the Okta/okta-auth-js and the Okta/okta-vue packages. By leveraging the power of Okta’s advanced authentication capabilities, developers can ensure data integrity and user trust while seamlessly implementing secure user experiences.
Here is the link to the complete source code on GitHub.
Resources
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.