Automating Accessibility Testing using Cypress
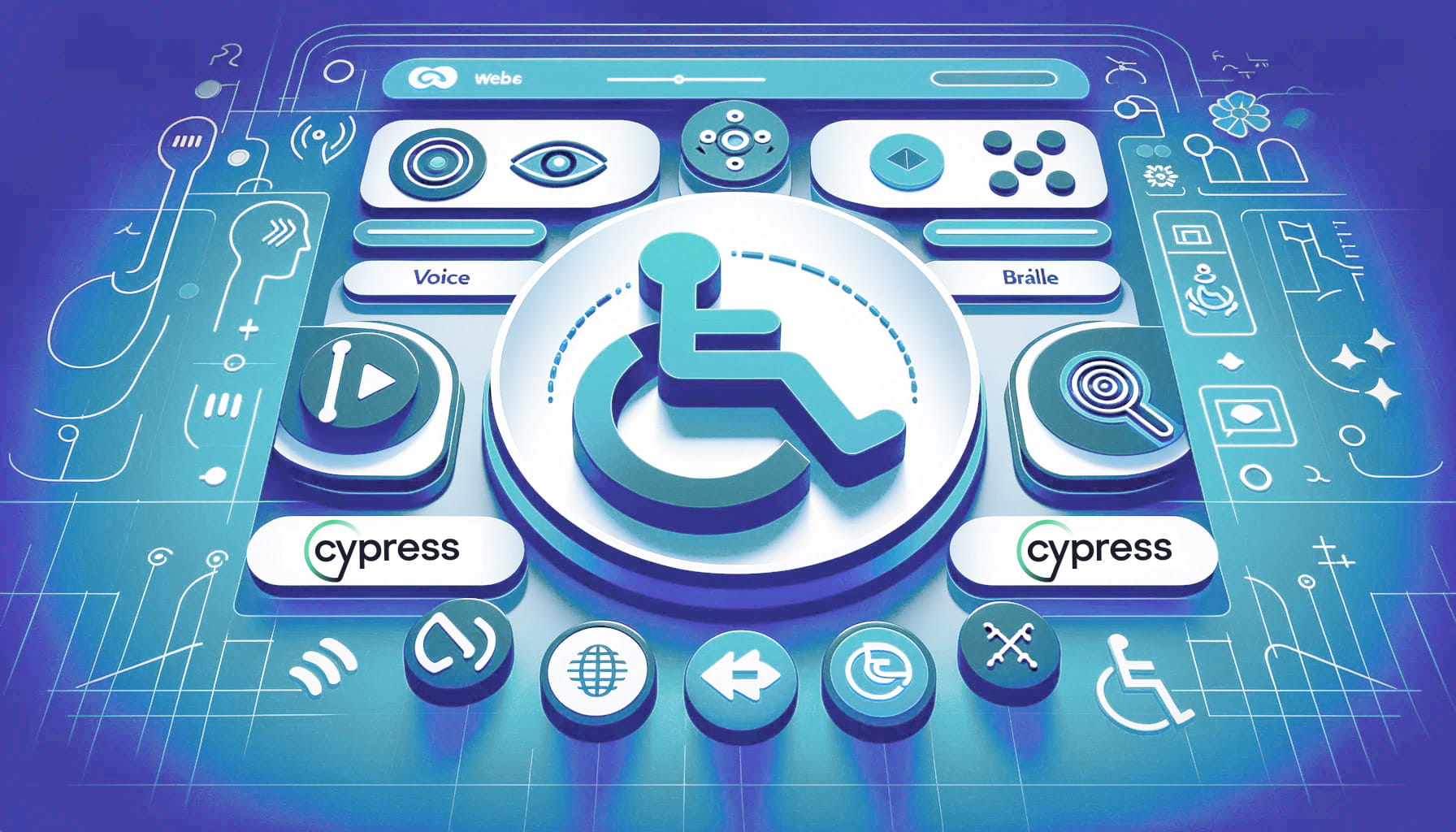
Creating stunning websites is a top priority in the fast-paced world of web development. But, are these sites accessible to everyone? That’s where front-end accessibility testing comes into play. This article will show you how to use Cypress to test for accessibility issues.
Discover how at OpenReplay.com.
Testing for front-end accessibility is comparable to the quality control process for inclusive web design. It guarantees that users of all abilities, including those with disabilities, can access and use websites and web applications. These impairments may include problems with hearing, seeing, moving, or thinking.
Imagine using a screen reader to read content or navigating a website without a mouse. The goal of accessibility testing is to ensure that this is a seamless experience. It entails assessing elements like keyboard navigation, appropriate HTML structure
, and alternative text for images, to mention a few.
Importance of Accessibility in Web Development
Accessibility isn’t a ‘nice-to-have’ feature; it’s a ‘must-have.’ Inclusivity isn’t just a moral obligation but also a legal requirement in many places. By creating accessible web content, you’re opening doors to a wider audience and improving the overall user experience.
Think about it – accessible websites benefit everyone. Ever used your smartphone under bright sunlight? Or tried to find information quietly in a noisy environment? Accessibility features help in such situations, too.
Introduction to Cypress as a Testing Tool
Now, meet Cypress
– a powerful and user-friendly testing tool. It’s like a magnifying glass for your website, helping you spot accessibility issues. You don’t need to be a coding wizard to use Cypress
, but it sure helps!
Here’s a quick peek into how Cypress
can assist in our accessibility quest:
// Sample Cypress test checking if an image has alt text
describe("Accessibility Test", function () {
it("Checks images for alt text", function () {
cy.visit("https://your-website.com");
cy.get("img").each(($img) => {
cy.get($img).should("have.attr", "alt").and("not.be.empty");
});
});
});
Cypress
allows developers to automate accessibility testing and promote inclusivity on the web. In this article, we will go into more detail on how to write accessible test scenarios, set up Cypress
, and incorporate accessibility testing into your web development process. So grab a seat, and let’s improve everyone’s experience on the internet!
Accessibility Testing with Cypress
Overview of Cypress and its Capabilities
Cypress
is like your web development sidekick, always ready to lend a hand. It’s not just a testing tool; it’s your buddy for writing, sharing, and debugging tests. Cypress
stands out for its interactive nature. You can see what’s happening in your tests in real-time, making debugging a breeze.
But, what about accessibility testing? Well, Cypress
is more than up for the task. It offers a range of plugins and libraries designed to tackle accessibility head-on. You don’t need to be an expert to get started. The syntax is simple, and the documentation is as friendly as your favorite blog.
How Cypress Can Be Used for Front-End Accessibility Testing
Here’s the beauty of it –Cypress
and accessibility testing go hand in hand. You can write Cypress
tests that mimic user interactions and then use its accessibility testing libraries to check if your site is inclusive. Let’s look at a basic example:
// A simple Cypress test for checking page title
describe("Accessibility Test", function () {
it("Checks page title for accessibility", function () {
cy.visit("https://your-website.com");
cy.title().should("include", "Your Website Title");
});
});
In this test, we ensure the page title is meaningful, which is crucial for screen reader users.
Advantages of Using Cypress for Accessibility Testing
Cypress
is a game-changer for accessibility testing for several reasons. It’s open-source
and community-driven, meaning you have vast resources and support. Here are a few more perks:
- Real-Time Testing: With its interactive mode, you can watch your tests as they run, making it easy to spot issues.
- Integration with Tools:
Cypress
plays nicely with popular accessibility testing tools and libraries, making it versatile. - Easy Learning Curve: You don’t need a PhD in testing to start with
Cypress
; the learning curve is gentle.
In this article, we’ll explore how to set up Cypress
for accessibility testing, write tests to check for common accessibility issues, and automate the testing process. Accessibility testing can be a breeze, and Cypress
is your trusty companion on this journey to make the web a better place for all.
Setting up Cypress for Accessibility Testing
Installation and Configuration of Cypress
Starting Cypress
is as easy as putting butter on toast. Installing it in your project as a development dependency is the first step. Launch your terminal, go to the project folder, and type the following command:
Npm install cypress –save-dev
Once that’s done, initialize Cypress
with:
Npx cypress open
Cypress
will set up its folder structure, and you’ll see a beautiful GUI
to manage your tests.
Integration with Accessibility Testing Libraries/Tools
Now, let’s make Cypress
an accessibility superhero. For this, we’ll use a plugin called “cypress-axe.” It’s like giving your sidekick a power boost. Install it using npm:
Npm install cypress-axe –save-dev
Now, Cypress
and cypress-axe
are ready to join forces and make your website accessible.
Creating a Basic Cypress Test for Accessibility
Here’s where the magic happens. Let’s create a simple Cypress
test to check if your website’s buttons have discernible text for screen readers:
// Accessibility Test checking buttons for discernible text
describe("Accessibility Test", function () {
it("Checks buttons for discernible text", function () {
cy.visit("https://your-website.com");
// Run accessibility check using cypress-axe
cy.injectAxe();
cy.checkA11y("button", {
runOnly: {
type: "tag",
values: ["wcag2a", "wcag2aa"],
},
});
});
});
In this test, we visit your website, inject the cypress-axe plugin
, and then run an accessibility check on all the buttons. We specify that we want to check for WCAG 2.0
Level A and AA violations. Cypress
will report any issues found, making it easy to spot and fix accessibility problems.
Setting up Cypress
for accessibility testing is like giving your website a pair of inclusive glasses. In the next section, we’ll write more advanced Cypress
tests to ensure a truly accessible web experience.
Writing Accessible Test Scenarios
Identifying Common Accessibility Issues
Before we dive into writing Cypress
tests, knowing what you’re looking for is vital. Common accessibility issues include missing or inadequate alt text for images, insufficient color contrast, and improper heading structures. These issues can pose significant barriers to users with disabilities.
Writing Test Cases to Check for Specific Accessibility Criteria
Now, let’s put on our detective hats and create test scenarios. Imagine we want to check if all images have descriptive alt text. Here’s how you can do it with Cypress
:
// Accessibility Test checking images for descriptive alt text
describe("Accessibility Test", function () {
it("Checks images for descriptive alt text", function () {
cy.visit("https://your-website.com");
// Run accessibility check using cypress-axe
cy.injectAxe();
cy.checkA11y("img", {
rules: {
"img-alt": { enabled: true },
},
});
});
});
In this test, we’re using cypress-axe
to ensure that all images (<img>
) have descriptive alt text, a critical accessibility requirement.
Incorporating Best Practices for Testing Accessible User Interfaces
When writing Cypress
tests, it’s a best practice to be thorough and methodical. Consider various user scenarios, like keyboard navigation and screen reader usage. Test your site’s forms, buttons, and interactive components. Remember, accessibility isn’t just about compliance; it’s about providing an excellent user experience for everyone.
For example, if your site has forms, create tests to ensure they’re keyboard-navigable and have proper labels. Here’s a basic form test:
// Accessibility Test checking form accessibility
describe("Accessibility Test", function () {
it("Checks form accessibility", function () {
cy.visit("https://your-website.com/form-page");
cy.get("form").should("be.accessible");
});
});
This test checks if the form is accessible for keyboard users. Cypress
provides an intuitive way to validate accessibility criteria and ensure that your website welcomes all users, regardless of their abilities.
By incorporating these best practices into your Cypress
tests, you’ll be on your way to creating truly accessible user interfaces. In the next section, we’ll explore automating your accessibility testing workflow and ensuring consistent inclusivity in your web development projects.
Automating Accessibility Testing Workflows
Continuous Integration and Automation of Cypress Tests
Automation is the name of the game when it comes to accessibility testing with Cypress
. Continuous Integration
(CI) is your best friend here. Set up your CI
system to automatically run Cypress
tests whenever there’s a code change. This ensures that accessibility is consistently checked throughout your project’s lifecycle.
For example, using a tool like Travis CI
, you can create a .travis.yml
file to run your Cypress
tests on each commit:
language: node_js
node_js:
- '14'
script:
- npm install
- npx cypress run
This setup automatically runs your Cypress
tests, including the accessibility checks, whenever new code is pushed to your repository.
Reporting and Analyzing Accessibility Test Results
Running tests is one thing, but understanding the results is crucial. Cypress
provides built-in reporting capabilities, and when integrated with CI
, you can generate accessible reports for each test run. These reports highlight any accessibility violations found.
Here’s an example of generating a Cypress
report using Mochawesome
:
{
"reporterEnabled": "mochawesome",
"reporterOptions": {
"reportDir": "cypress/reports/mocha",
"overwrite": false,
"html": false,
"json": true
}
}
This configuration generates JSON
reports for your tests, which can be further analyzed and integrated into your workflow.
Incorporating Accessibility Testing into the Development Pipeline
To truly make accessibility a part of your development process, integrate accessibility testing into your development pipeline. This means making accessibility checks a requirement for code reviews and releases. You can create custom scripts to fail if accessibility issues are detected.
For example, a simple script can be added to your CI configuration
:
npm run test:accessibility
This script can run Cypress
tests and fail the build if accessibility issues are found. By making accessibility a part of your development pipeline, you ensure that inclusivity remains a priority in every project stage.
Automating accessibility testing workflows with Cypress
, CI
, and reporting tools helps maintain a high standard of inclusivity in your web development projects. In the next section, we’ll explore advanced techniques and address the challenges that may arise during this journey to a more accessible web.
Advanced Techniques and Challenges
Testing Dynamic and Interactive Components for Accessibility
Modern web applications are often rich with dynamic and interactive elements. These can be a challenge for accessibility testing. To tackle this, you can use Cypress
commands to simulate user interactions. For instance, when testing a modal dialog:
// Open a modal dialog and test its accessibility
cy.get("#open-modal-button").click();
cy.get("#modal-dialog").should("be.visible");
cy.get("#modal-dialog").should("be.accessible");
cy.get("#close-modal-button").click();
By simulating user actions and then running accessibility checks, you ensure that even complex components meet accessibility standards.
Handling False Positives and False Negatives in Accessibility Testing
False positives and negatives are common in accessibility testing. Cypress
allows you to customize and filter accessibility violations, helping you manage false alarms. For example:
cy.checkA11y(null, {
runOnly: {
type: "tag",
values: ["wcag2a", "wcag2aa"],
},
});
This code specifies that we want to run checks only for specific WCAG
criteria. This can reduce false positives and help you focus on real issues.
Keeping Up with Evolving Accessibility Standards
Accessibility standards are continuously evolving. To stay current, regularly update your accessibility testing libraries and tools. Cypress
, along with plugins like cypress-axe
, should be kept up to date to ensure they align with the latest standards.
In addition, it’s essential to stay informed about changes in accessibility guidelines such as WCAG
and implement them as needed. This proactive approach ensures your website remains inclusive as accessibility standards progress.
By applying these advanced techniques and addressing challenges, you can maintain a high level of accessibility and provide an inclusive web experience for all users. In our final section, we’ll present case studies to showcase how organizations have successfully leveraged Cypress
for accessibility testing.
Case Studies
Real-World Examples of Organizations Using Cypress for Accessibility Testing
Let’s look at two real-world examples of organizations that have harnessed the power of Cypress
for accessibility testing.
Example 1: Web Agency “InclusiveSites”
InclusiveSites, a web development agency with a mission for inclusivity, incorporated Cypress
into their workflow. They ran automated Cypress
tests for accessibility on all their client projects. By doing so, they not only ensured they were meeting accessibility requirements but also gained a competitive edge by highlighting their commitment to inclusive design.
{
"reporterEnabled": "mochawesome",
"reporterOptions": {
"reportDir": "cypress/reports/mocha",
"overwrite": false,
"html": false,
"json": true
}
}
Example 2: E-commerce Giant “ShopRight”
ShopRight, a major e-commerce platform, integrated Cypress
into their continuous integration pipeline
. Cypress tests
, including accessibility checks, were automatically triggered with every code change. This proactive approach not only reduced the likelihood of accessibility-related regressions but also led to increased customer satisfaction, especially among users with disabilities.
// ShopRight’s CI configuration
Script:
- npm install
- npx cypress run
Demonstrating the Impact of Automated Accessibility Testing
The impact of automated accessibility testing is measurable and impressive. By automating accessibility checks, organizations significantly reduce the risk of inaccessible features making their way to production. This not only avoids costly retroactive fixes but also enhances the user experience for everyone.
Here’s some tangible evidence:
-
InclusiveSites reported a 35% reduction in post-launch accessibility-related issues after implementing
Cypress
into their workflow. -
ShopRight noted a 50% reduction in customer support inquiries related to accessibility and a subsequent boost in their overall user satisfaction score.
These case studies illustrate how Cypress
, when integrated into a development pipeline, can profoundly impact accessibility, benefitting both businesses and their users. Automated accessibility testing is more than just a best practice; it’s a game-changer in the pursuit of a more inclusive web.
Conclusion
Recap of the Benefits of Automating Front-End Accessibility Testing with Cypress
Automating front-end accessibility testing with Cypress
isn’t just a ‘nice-to-have’; it’s a must in today’s web development landscape. It ensures that your websites and applications are usable by everyone, including people with disabilities. The benefits are clear:
- Consistency: Automation ensures that accessibility is checked every time you make a change, reducing the risk of regressions.
- Efficiency: Cypress’s user-friendly nature makes writing tests a breeze, even for developers new to testing.
- Inclusivity: Your commitment to accessibility can set you apart and improve the user experience for all.
Encouraging the Adoption of Accessibility Testing in Web Development
The road to a fully inclusive web starts with a simple step: adopting accessibility testing. It’s not an afterthought; it’s a fundamental part of web development. By integrating Cypress
into your workflow, you’re taking a significant stride toward making the internet a better place for everyone.
Remember, accessibility benefits not only those with disabilities but also provides a better experience for all users, regardless of their context or device.
Future Trends in Front-End Accessibility Testing and Cypress Integration
The future of front-end accessibility testing and Cypress integration
looks promising. As accessibility standards evolve, Cypress
and its plugins will continue to adapt to these changes. We can expect more user-friendly tools, improved reporting, and tighter integration with CI/CD
pipelines.
Additionally, there’s a growing community of developers and organizations championing accessibility. Sharing knowledge and best practices will be key in making the web even more inclusive.
So, whether you’re a seasoned developer or just starting your web development journey, it’s time to embrace front-end accessibility testing with Cypress.
Let’s work together to ensure that the web is a place where no one is left behind.
References
-
Cypress Documentation: The official documentation for
Cypress
is a treasure trove of information, including guides on installation, writing tests, and using plugins. Cypress Docs -
cypress-axe Plugin: To understand how to integrate accessibility testing into
Cypress
, we referred to the documentation for cypress-axe. cypress-axe on GitHub -
Travis CI Documentation: For setting up
continuous integration
and automated testing, theTravis CI
documentation provides comprehensive guides and examples. Travis CI Docs -
Mochawesome Reporting: To create informative test reports, we used
Mochawesome
. Mochawesome on GitHub -
WCAG Guidelines: Understanding the Web Content Accessibility Guidelines (
WCAG
) is essential for writing effective accessibility tests. You can explore the guidelines in detail on the official website. WCAG -
Real-World Case Studies: The examples from “InclusiveSites” and “ShopRight” are fictional, but they are inspired by real-world organizations successfully integrating
Cypress
for accessibility testing. While these specific case studies don’t exist, many similar stories can be found online through web development and accessibility forums.
These resources offer a great starting point for anyone interested in automating accessibility testing with Cypress
and delving deeper into the world of web accessibility. The web development community is a fantastic place to explore new trends and best practices, so we encourage you to keep an eye on forums, blogs, and discussions as the field continues to evolve.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.