Shorten your URLs with React: Build a URL Shortener using Shrtcode
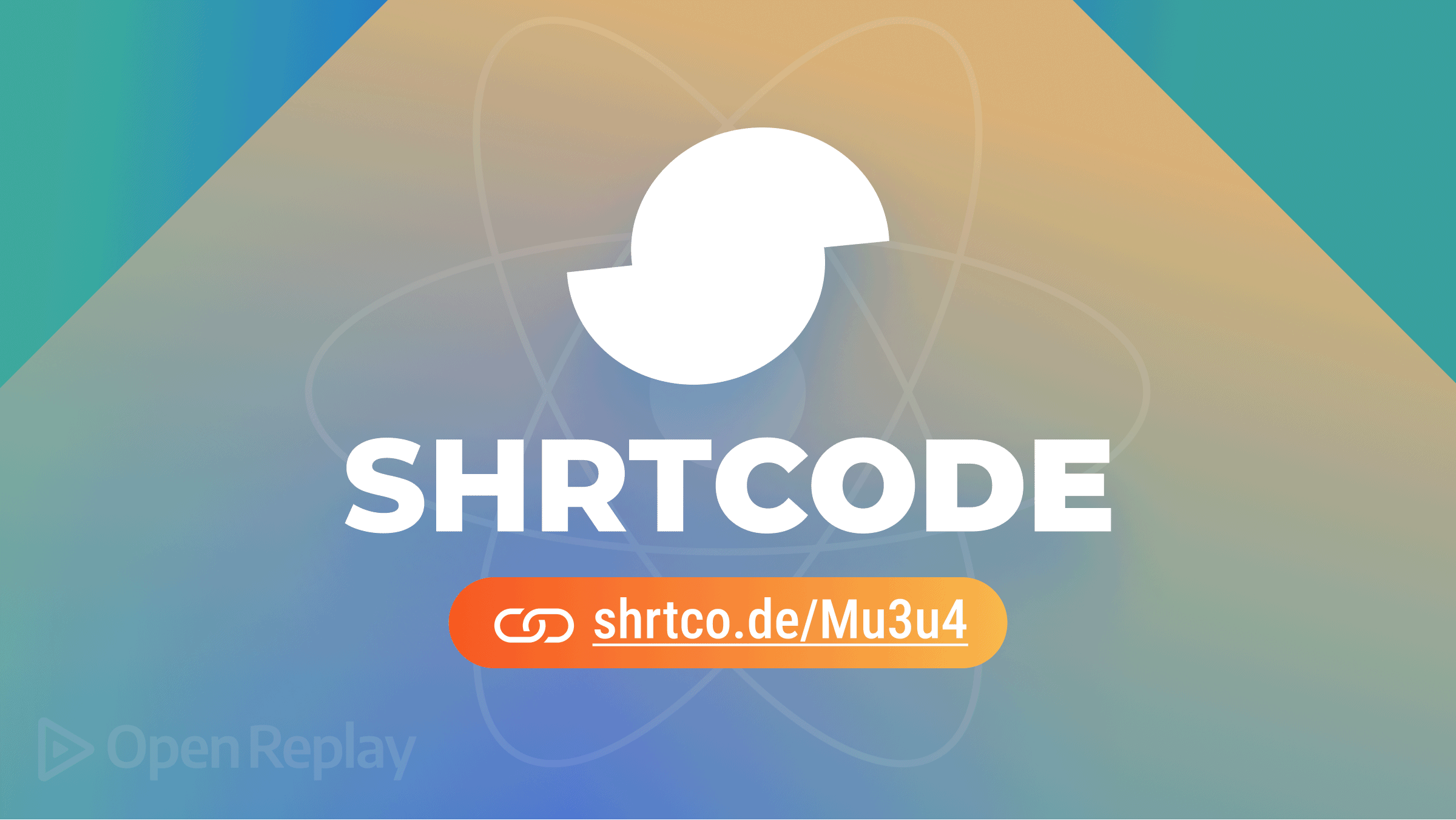
Have you ever tried to share a URL filled with query parameters with someone else? Did they like it? Was the URL short and easy to remember?
The answer to those questions is most likely “no”.
This can be quickly solved using URL shorteners. URL shorteners are tools used to create a unique URL that will redirect somewhere else. This new URL is shorter and easier to remember.
In this article, I’ll show you how to build a URL shortener using React.js and Shrtcode.
What is a URL Shortener?
URL shortening consists on taking a URL and reducing its length. At the same time, providing a new unique URL that directs to the original.
The general idea is to reduce long addresses into a shorter format that is easier to remember, track and share.
URL shorteners are websites that offer the service of URL shortening. These sites offer a service that maintains the connection between the original and shortened URLs. They are responsible for redirecting users to the desired web page. The new shortened URL contains the URL shortener’s domain address, plus a combination of characters and numbers. Some examples of websites that offer URL shortening services are Shrtco.de (which we’ll be using), Bit.ly, T.co, Tiny URL, Rebrandly, and Tiny.cc.
- You’ll want to shorten your URLs when they have parameters such as search query, pagination, or session data. These parameters make URLs difficult to distribute, share or memorize. That makes them perfect candidates for URL shorteners.
- When writing books, links may be longer than one line and, in the case of hard copies, they could be difficult to copy out manually.
- Some messaging platforms also have a character limit to messages, which can make sending long URLs challenging.
- Social media platforms, like Twitter, TikTok and others also limit the number of characters you can use. Having a shorter version of your URL gives you more space to write the actual message.
- URL shorteners make URLs more appealing and can increase conversion rates.
- URL shorteners can provide detailed analytics that you can use to understand metrics such as click-through rates, who’s clicking your links, where are they coming from (i.e the referrer URL), etc.
- You can shorten URLs with just a button click.
- Shortened URLs can prevent you from scaring people by sending long and ugly URLs which may look suspicious.
For this tutorial, we will build a URL shortener using Shrtco.de. Shrtco.de offers a free, easy-to-use API for shortening URLs, with no prior authentication needed to access it. It is secure and privacy-friendly.
Below are a few reasons why you should consider using Shrtcode to shorten your URLs:
- Shrtco.de ensures shortened URLs always redirect to the desired page. This is key, because over the years, various similar services have been hacked and made to redirect users to malicious sites.
- All shortened URLs are regularly scanned as a preemptive measure against malware.
- Shrtco.de cares about privacy, it does not track visitors to shortened URLs. It also provides the ability add a password to shortened URLs to prevent people from accessing without authorization.
- Shrtco.de gives you 3 domains to choose from, “shrtco.de”, “9qr.de” and “shiny.link”. This way you can customize and control the length of your URLs.
- Apart from normal URLs, Shrtco.de can generate emoji links or qr codes as shortened URLs.
Building the URL shortener
For the URL shortener, we will be using the React.js framework. We will need a simple UI featuring an input field, a button to submit the URL, a box to display the shortened result, and a copy to clipboard
button. We will use TailwindCSS
for easy styling of the application. We can create this in the App.js
file as shown below:
import "./App.css";
import {React, useState} from "react";
function App() {
return (
<div className=" container h-screen flex justify-center items-center">
<div className=" text-center">
<h1 className=" text-2xl font-medium text-blue-500 mb-4">
Our <span className=" text-yellow-400">URL Shortener
</h1>
<div>
className="outline-none border-2 border-blue-500 rounded-md backdrop-blur-xl bg-white/20 shadow-md px-3 py-3"
type="text"
placeholder="Enter link to be shortened"
/>
<button className=" bg-blue-500 text-white px-8 py-3 ml-4 rounded-md">
Submit URL
</button>
</div>
</div>
</div>
);
}
export default App;
In the code above, we have a simple input field and a button. If we run the code with the npm start
command, we get a result similar to the below image:
To handle user input in the field, we will create a state to manage this.
const [userInput, setUserInput] = useState("");
We can append this to the input field value
and onChange
properties:
//...
value={userInput}
onChange={(e)=>{setUserInput(e.target.value)}}
/>
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Link Preview Area
Next, we will add an area to show the shortened URL.
const [shortenedLink, setShortenedLink] = useState("")
Then, we add the preview area and “copy to clipboard” button after the submit URL
button.
//...
<div className="mt-5">
{shortenedLink}
<button className="border-2 border-blue-500 text-blue-500 font-medium px-5 py-2 ml-4 rounded-md">
Copy URL to Clipboard
</button>
</div>
We will use the ‘react-copy-to-clipboard’ package for the “copy to clipboard” feature. We will add an import for this package at the top level of our code:
import CopyToClipboard from "react-copy-to-clipboard";
We will use it as shown in the code block below:
<CopyToClipboard text={shortenedLink}>
<button className="border-2 border-blue-500 text-blue-500 font-medium px-5 py-2 ml-4 rounded-md">
Copy URL to Clipboard
</button>
</CopyToClipboard>
Here, the text
property references what gets copied when we click the button.
ShrtCo.de Setup
To set up Shrco.de, we will create a function using axios
fetch:
import axios from 'axios';
//...
const fetchData = async () => {
try {
const response = await axios(
`https://api.shrtco.de/v2/shorten/dXJsPQ== ${userInput}`
);
setShortenedLink(response.data.result.full_short_link);
} catch (e) {
console.log(e);
}
};
Finally, we will add this function to the Submit URL
function:
className=" bg-blue-500 text-white px-8 py-3 ml-4 rounded-md"
onClick={() => {
fetchData();
}}
>
Submit URL
</button>
Now, if we add a URL in the input field and click the submit button, we get a shortened link.
Conclusion
In this tutorial, we learned:
- What are URL shorteners and why you want to use them.
- And how to easily build one using React.js and Shrtco.de.
The entire source code used in this tutorial can be found here.
If you enjoyed the article, make sure to share it and leave a comment if you implemented this or a similar project!