How to Build a Splash Screen in React Native (With Code Examples)
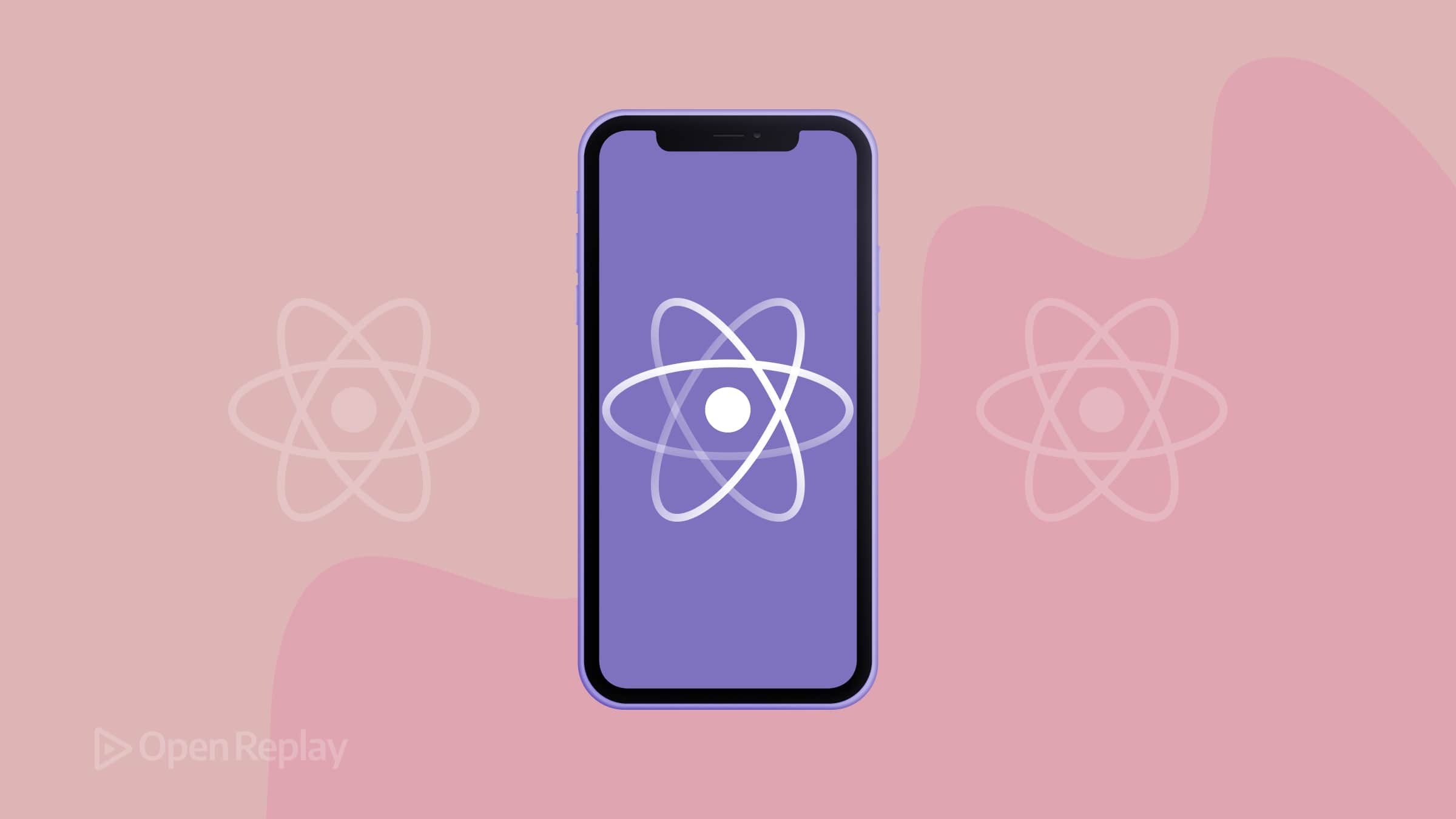
First impressions are crucial in mobile apps. When a user launches your React Native app, a splash screen is the first thing they see. A splash screen displays your app logo or branding while the app initializes, giving your app a more polished feel by avoiding a blank screen and reassuring users that the app is loading.
Key Takeaways
- A splash screen is the initial screen showing your branding when a React Native app launches
- iOS requires a launch screen as part of the platform’s user experience
- Two implementation approaches: manual setup via Xcode or using libraries like react-native-splash-screen
- Proper timing and smooth transitions are essential for a professional user experience
- Match your splash screen’s background with your app’s first screen for seamless transitions
What is a Splash Screen in React Native?
A splash screen is the initial screen that appears when your app launches. In React Native, the splash screen typically shows your app’s name, logo, or other branding elements on a plain background. It stays visible while the native code loads and prepares the React Native app. The main purposes of a splash screen are:
- Branding: It makes your app’s brand immediately visible
- User Experience: It prevents the user from seeing a blank or unresponsive screen during app startup
- Perception of speed: A splash screen gives the impression that something is happening, making load time feel shorter
Why Use a Splash Screen in React Native for iOS?
All mobile apps can benefit from splash screens, but on iOS they are especially important. In fact, Apple requires apps to have a launch screen – on iOS, the splash screen isn’t just for show; it’s part of the expected user experience. Here are key reasons:
- Enhanced User Experience: iOS apps that launch with a nicely designed splash screen feel smoother and more professional
- App Branding: The splash screen showcases your app’s logo, tagline, or artwork
- Manage Loading Times: iOS will display the launch screen until the app is ready, masking any delays during startup
Setting Up a Splash Screen in React Native (iOS-Specific)
Step 1: Create a Splash Screen Image
First, you need an image for your splash screen:
- Size: Use a high-resolution image (at least 2000 x 2000 pixels) that looks good on all device screens
- Format: PNG is the most commonly used format for splash screens
- Orientation and Safe Area: Keep important content toward the center of the image
Pro Tip: Tools like App Icon Generator (appicon.co) can help create correctly sized images for various iOS screens.
Step 2: Add the Splash Screen in Xcode
- Open the iOS project in Xcode: Navigate to the
ios
folder and open the.xcworkspace
or.xcodeproj
file. - Add image assets: In Xcode, find Images.xcassets, create a New Image Set named
""Splash""
, and add your splash image. - Edit Launch Screen storyboard: Open LaunchScreen.storyboard, delete any default elements, and add an Image View. Set the image to your splash image, resize or constrain it, and set the Content Mode (Aspect Fit or Aspect Fill).
- Set safe area (if needed): If necessary, disable ""Use Safe Area"" to allow the image to extend under status bar/notch areas.
Step 3: Modify Info.plist for Proper Display Settings
- Open Info.plist and look for the key ""UILaunchStoryboardName"".
- Ensure its value is the name of your storyboard (e.g., ""LaunchScreen"").
- Verify that the LaunchScreen file is included in the app target.
Now run your app on an iOS simulator or device to see your splash screen appear briefly at launch.
Using a Library for a Splash Screen
Using a library can simplify the process and provide additional control. Two popular libraries are:
- react-native-splash-screen – Widely used for both iOS and Android
- expo-splash-screen – For Expo managed workflow
Let’s use react-native-splash-screen:
-
Install the library:
npm install react-native-splash-screen --save
or
yarn add react-native-splash-screen
-
iOS: Install pods:
cd ios pod install cd ..
-
Configure iOS native code:
-
Modify AppDelegate.m:
#import ""RNSplashScreen.h"" // add this import
In the
application:didFinishLaunchingWithOptions:
method, add:- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { // ... existing React Native bootstrapping code ... [self.window makeKeyAndVisible]; [RNSplashScreen show]; // show the splash screen return YES; }
-
Verify Info.plist: Ensure UILaunchStoryboardName is still set to your LaunchScreen storyboard.
-
-
Configure Android native code (if needed):
- Import SplashScreen in MainActivity
- Call
SplashScreen.show(this)
beforesuper.onCreate
- Add a
launch_screen.xml
layout - Update the AndroidManifest
-
Hide the splash screen in your React Native code:
// App.js (or index.js) import React, { useEffect } from 'react'; import { View, Text } from 'react-native'; import SplashScreen from 'react-native-splash-screen'; // import the library const App = () => { useEffect(() => { // Hide the splash screen once the app is ready SplashScreen.hide(); }, []); return ( <View>{/* Your app UI components start here */} <Text>Hello, Main App!</Text> </View> ); }; export default App;
Handling Splash Screen Timing & Transitions
- Keep it Brief: A splash screen should only be visible for as long as necessary (ideally 1-3 seconds)
- Hide When Ready: Call
SplashScreen.hide()
as soon as your app’s main content is ready to display - Match the Transition: Use the same background color in your app’s initial screen as the splash screen
- Smooth Animations: Consider adding a subtle animation when hiding the splash screen
- Consistent Duration: Some apps show the splash for a minimum time (e.g., 2 seconds) even if the app loads faster
Common Issues & Debugging Tips
- Splash screen not showing: Check UILaunchStoryboardName in Info.plist, verify the LaunchScreen file is in the app target, and confirm image assets are included
- Image is stretched or cut off: Review Content Mode settings, check constraints, and verify you provided the right image sizes
- Splash screen flashes then blank screen appears: Hide the splash only when your UI is ready, and match background colors
- Splash screen stays stuck: Ensure
SplashScreen.hide()
is being called and check for early errors - Incorrect aspect ratio on iPad: Use constraints instead of fixed sizes, set storyboard devices to Universal, and provide high-resolution images
- Changes not reflecting: Clean the build, reinstall the app, and make sure you’re editing the correct files
Conclusion
Adding a splash screen to your React Native app is a small step that yields a more professional and user-friendly experience. By either setting up a splash screen manually in Xcode or using libraries like react-native-splash-screen, you can integrate a splash screen that displays your branding and keeps users engaged during startup.
Remember to keep timing in mind and ensure smooth transitions between the splash screen and your app’s first screen. With proper implementation, your splash screen will enhance your app’s perceived quality from the moment it’s launched.
FAQs
Replace the old splash image in your Xcode asset catalog with the new image (or add a new image set and update the Image View in LaunchScreen.storyboard). If you used a background color, adjust that in the storyboard as well. Then rebuild your app. Because the splash screen is part of the native code, you'll need to recompile the app to see the changes.
Native splash screens are static by nature. However, you can create an illusion of animation by: 1) Animating the transition between the splash screen and your first screen, 2) Designing your first screen to look similar to the splash and using a fade-out or slide-up animation, or 3) Using Expo SplashScreen with animation control. Any animation will start after the app has loaded since the actual native splash can't animate.
To test your splash screen, run a cold start of your app on the iOS simulator or device. In Xcode, press the Run button to install and launch the app fresh. After making changes, clean the build (Product > Clean Build Folder) or uninstall the app before re-running. Test on multiple simulator sizes to see how the splash scales. For Expo apps, you may need to do an Expo prebuild or use a simulator build to see your custom splash.