Choosing Between call(), apply(), and bind() in JavaScript: A Developer's Guide
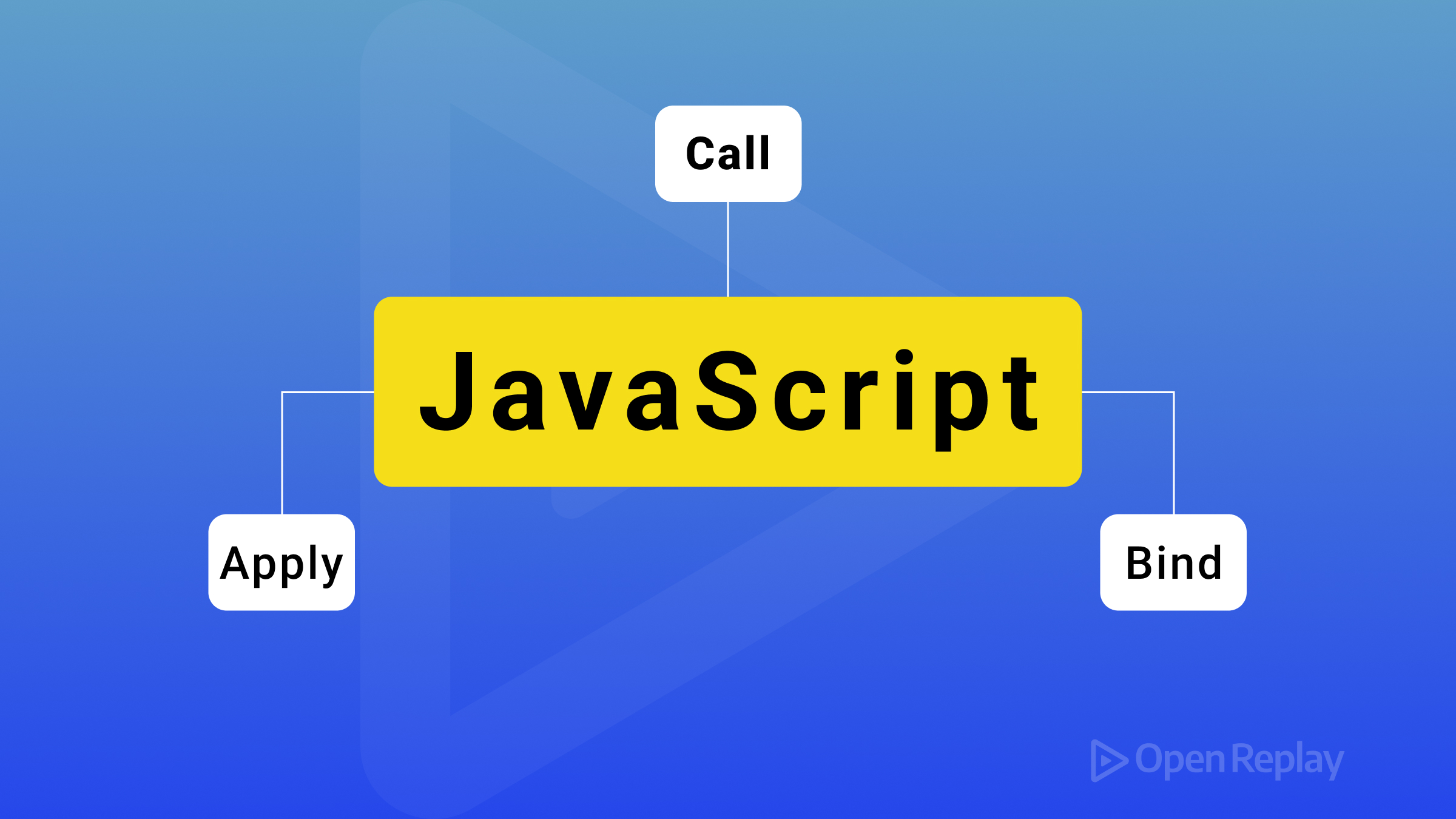
JavaScript’s function context management can be challenging, especially when dealing with the this
keyword. The built-in methods call()
, apply()
, and bind()
provide powerful solutions for controlling function execution context, but knowing which one to use and when can be confusing. This guide will help you understand these methods and make informed decisions about which one is right for your specific use case.
Key Takeaways
call()
executes a function immediately with a specified context and individual argumentsapply()
executes a function immediately with a specified context and arguments as an arraybind()
creates a new function with a fixed context for later execution- Arrow functions and spread syntax offer modern alternatives in many scenarios
- Choose the right method based on execution timing, argument format, and context persistence needs
Understanding the Problem: JavaScript’s this
Context
Before diving into the solutions, let’s clarify the problem these methods solve. In JavaScript, the value of this
inside a function depends on how the function is called, not where it’s defined. This can lead to unexpected behavior, especially when:
- Passing methods as callbacks
- Working with event handlers
- Using functions across different objects
- Dealing with asynchronous code
Consider this common scenario:
const user = {
name: ""Alex"",
greet() {
console.log(`Hello, I'm ${this.name}`);
}
};
// Works as expected
user.greet(); // ""Hello, I'm Alex""
// The context is lost
const greetFunction = user.greet;
greetFunction(); // ""Hello, I'm undefined""
When we extract the method and call it directly, the this
context is lost. This is where call()
, apply()
, and bind()
come to the rescue.
The Three Context-Setting Methods
All three methods allow you to explicitly set the this
value for a function, but they differ in how they execute and what they return.
call(): Execute with a Specified Context
The call()
method executes a function immediately with a specified this
value and individual arguments.
Syntax:
function.call(thisArg, arg1, arg2, ...)
Example:
const user = {
name: ""Alex"",
greet() {
console.log(`Hello, I'm ${this.name}`);
}
};
const anotherUser = { name: ""Sam"" };
// Borrow the greet method and use it with anotherUser
user.greet.call(anotherUser); // ""Hello, I'm Sam""
Key characteristics:
- Executes the function immediately
- Accepts arguments individually after the context
- Returns the function’s result
- Doesn’t create a new function
apply(): Execute with Arguments as an Array
The apply()
method is almost identical to call()
, but it takes arguments as an array or array-like object.
Syntax:
function.apply(thisArg, [argsArray])
Example:
function introduce(greeting, punctuation) {
console.log(`${greeting}, I'm ${this.name}${punctuation}`);
}
const user = { name: ""Alex"" };
introduce.apply(user, [""Hi"", ""!""]); // ""Hi, I'm Alex!""
Key characteristics:
- Executes the function immediately
- Accepts arguments as an array
- Returns the function’s result
- Doesn’t create a new function
bind(): Create a New Function with Fixed Context
The bind()
method creates a new function with a fixed this
value, without executing the original function.
Syntax:
const boundFunction = function.bind(thisArg, arg1, arg2, ...)
Example:
const user = {
name: ""Alex"",
greet() {
console.log(`Hello, I'm ${this.name}`);
}
};
const greetAlex = user.greet.bind(user);
// The context is preserved even when called later
setTimeout(greetAlex, 1000); // After 1 second: ""Hello, I'm Alex""
Key characteristics:
- Returns a new function with bound context
- Doesn’t execute the original function immediately
- Can pre-set initial arguments (partial application)
- Preserves the original function
When to Use Each Method: A Decision Guide
Use call()
when:
- You need to execute a function immediately with a different context
- You have individual arguments to pass
- You’re borrowing a method from another object for one-time use
Use apply()
when:
- You need to execute a function immediately with a different context
- Your arguments are already in an array or array-like object
- You’re working with variadic functions like
Math.max()
orMath.min()
Use bind()
when:
- You need a function with a fixed context for later execution
- You’re passing methods as callbacks and need to preserve context
- You’re working with event handlers that need access to a specific
this
- You want to create partially applied functions with preset arguments
Practical Examples
Example 1: Method Borrowing
const calculator = {
multiply(a, b) {
return a * b;
}
};
const scientific = {
square(x) {
// Borrow the multiply method
return calculator.multiply.call(this, x, x);
}
};
console.log(scientific.square(4)); // 16
Example 2: Working with DOM Events
class CounterWidget {
constructor(element) {
this.count = 0;
this.element = element;
// Using bind to preserve the class instance context
this.element.addEventListener('click', this.increment.bind(this));
}
increment() {
this.count++;
this.element.textContent = this.count;
}
}
const button = document.getElementById('counter-button');
const counter = new CounterWidget(button);
Example 3: Working with Math Functions and Arrays
const numbers = [5, 6, 2, 3, 7];
// Using apply with Math.max
const max = Math.max.apply(null, numbers);
console.log(max); // 7
// Modern alternative using spread syntax
console.log(Math.max(...numbers)); // 7
Example 4: Partial Application with bind()
function log(level, message) {
console.log(`[${level}] ${message}`);
}
// Create specialized logging functions
const error = log.bind(null, 'ERROR');
const info = log.bind(null, 'INFO');
error('Failed to connect to server'); // [ERROR] Failed to connect to server
info('User logged in'); // [INFO] User logged in
Modern Alternatives
Arrow Functions
Arrow functions don’t have their own this
context. They inherit it from the enclosing scope, which can eliminate the need for binding in many cases:
class CounterWidget {
constructor(element) {
this.count = 0;
this.element = element;
// Using arrow function instead of bind
this.element.addEventListener('click', () => {
this.increment();
});
}
increment() {
this.count++;
this.element.textContent = this.count;
}
}
Spread Syntax
Modern JavaScript provides the spread syntax (...
) which can often replace apply()
:
// Instead of:
const max = Math.max.apply(null, numbers);
// You can use:
const max = Math.max(...numbers);
Method Comparison Table
Feature call()
apply()
bind()
Execution Immediate Immediate Returns function Arguments Individual As array Individual (preset) Returns Function result Function result New function Use case One-time execution Array arguments Callbacks, events Context setting Temporary Temporary Permanent
Performance Considerations
When performance is critical, consider these factors:
bind()
creates a new function object, which has memory overhead- Repeatedly binding the same function in loops can impact performance
- For high-frequency operations, pre-bind functions outside loops
- Modern engines optimize
call()
andapply()
well, but direct calls are still faster
Conclusion
Understanding when to use call()
, apply()
, and bind()
is essential for effective JavaScript development. Each method serves a specific purpose in managing function context. While call()
and apply()
provide immediate execution with different argument formats, bind()
creates reusable functions with fixed contexts. Modern JavaScript features like arrow functions and spread syntax offer additional options for handling context and arguments. By selecting the appropriate method for each situation, you can write more maintainable code and avoid common pitfalls related to function context.
FAQs
Yes, but it won't affect the `this` value of arrow functions since they don't have their own `this` binding. Arrow functions inherit `this` from their surrounding lexical context.
In non-strict mode, `this` will be the global object (`window` in browsers). In strict mode, `this` will remain `null` or `undefined`.
Yes, they work with any function including class methods. This is particularly useful when you need to pass class methods as callbacks while preserving their context.
Direct function calls are fastest, followed by `call()`/`apply()`, with `bind()` being slightly slower due to the function creation overhead. For performance-critical code, consider these differences.
`apply()` is ideal for variadic functions when arguments are in an array. With modern JavaScript, spread syntax (`...`) is often cleaner and more readable for the same purpose.