Client to Client (C2C) communication in Node.js
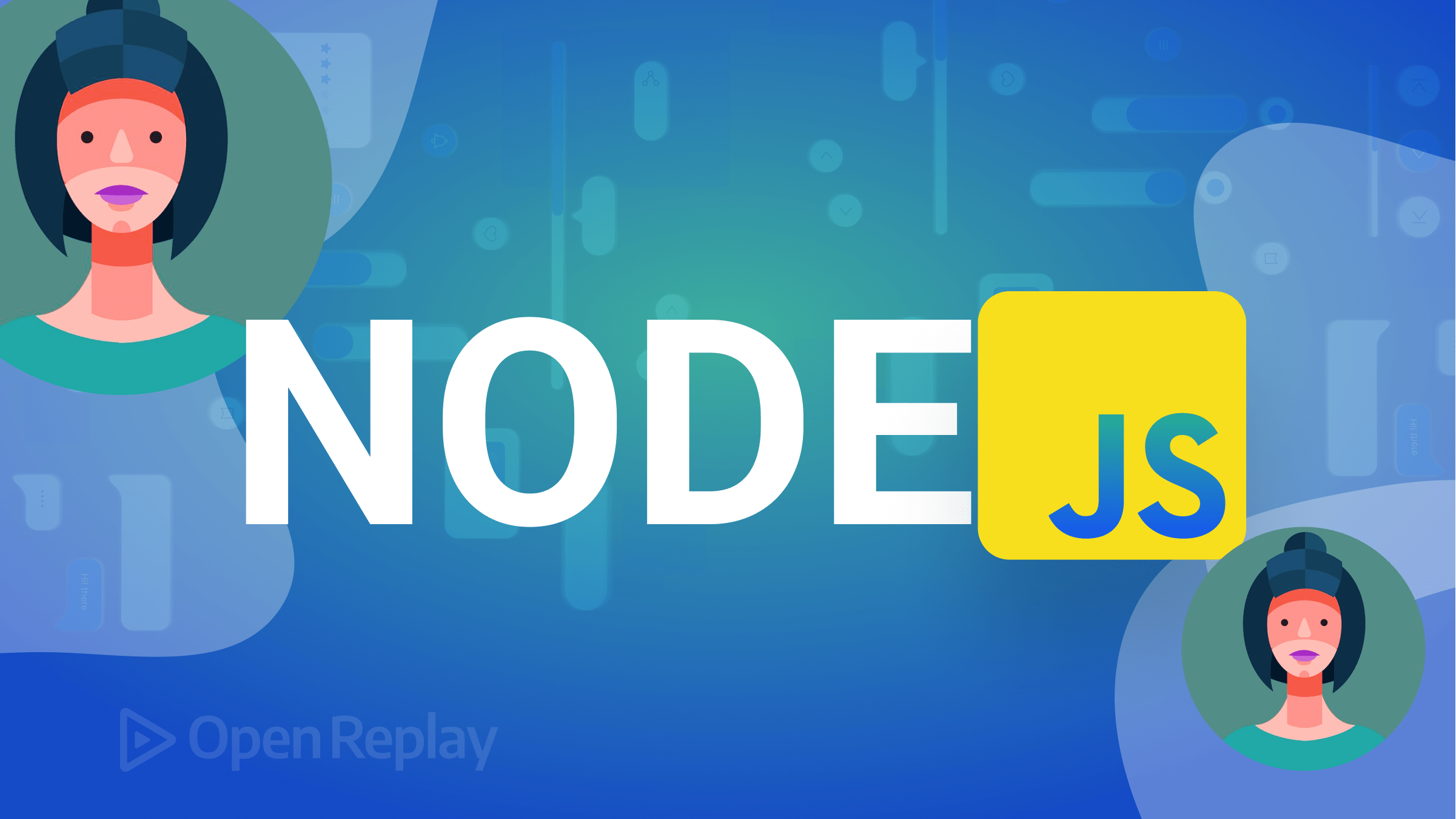
Client-to-client (or peer-to-peer) communication is a type of network communication in which two or more clients or nodes talk directly without a centralized server or middleman. Each participant serves as a client and a server and can communicate directly with other clients to exchange information, data, or resources, increasing stability and reducing load. P2P is frequently used in distributed applications such as file sharing and online gaming, and this article will show you how to implement this in Node.js.
The widely used runtime environment Node.js offers a platform for creating scalable, high-performance JavaScript applications. Client-to-client communication, also known as peer-to-peer (P2P) communication, is one of the key characteristics of Node.js . In this article, we will examine the various methods and libraries that developers can use to implement client-to-client communication in Node.js .
Node.js’s C2C communication Components
The following are the basic C2C components:
-
WebSockets: With the help of the WebSockets protocol , clients and servers in Node.js can communicate in real time and both directions. They are perfect for real-time applications like chat and gaming because they support persistent connections. WebSockets enable direct connections between clients in a client-to-client communication system, which is more effective and dependable. WebSocket servers and clients can be easily created using the Node.js ws library , which offers an easy-to-use WebSocket implementation.
-
Connection management: In a Node.js client-to-client communication system, connection management entails creating and maintaining WebSocket connections between clients, handling errors and controlling data flow. Implementing features like automatic reconnection or load balancing to handle large client loads is a necessary component of effective connection management, which is essential for ensuring system stability and responsiveness. A positive user experience is ensured by effective connection management.
-
Message handling: In a Node.js client-to-client communication system, message handling entails encoding , decoding , and processing and sending and receiving messages over WebSocket connections . It might also include features like prioritization or queuing for effective delivery. Good’ message handling’ is essential for reliable and timely message delivery, accurate client-side processing, and a seamless user experience.
-
Scalability and performance: In a client-to-client communication system using Node.js , scalability and performance are crucial. Performance involves tuning the system’s code, architecture, and network infrastructure to reduce latency and increase throughput . Scalability involves designing the system to handle increasing numbers of clients or connections. In real-time applications, both are essential for delivering a responsive and dependable user experience. Scalability can be helped by strategies like load balancing and clustering , and performance can be improved by message compression or binary protocols .
Implementing client-to-client communication in Node.js: Techniques
Developers can use several techniques and libraries to implement client-to-client communication in Node.js . In this section, we will explore some of the most common techniques.
Web Sockets
Developers can use various methods and libraries to implement client-to-client communication in Node.js . We will look at some of the most popular methods in this section.
Using Node.js , programmers can build a Web Socket server that waits for client connections to initiate client-to-client communication. A Web Socket connection can be established between each client and the server, which will then be able to pass messages and data between clients as necessary. The ws library offers a variety of options and configuration settings that can be tailored to a particular application’s requirements.
Peer.js
A JavaScript library called Peer.js makes it easier to create P2P browser applications. It offers a straightforward and understandable API for establishing WebRTC connections between clients, enabling fast and secure data transfer without a server. Peer.js offers a variety of features and options that make it simple to create P2P applications in Node.js and is built on top of WebRTC , a well-liked technology for real-time communication in the browser.
Using Peer.js in Node.js , programmers can implement client-to-client communication by building a Peer server that watches for client connections. Each client can establish a peer connection with the server, which will then be able to transfer messages and data as necessary between clients. Peer.js offers a variety of options and configuration settings that can be tailored to a particular application’s requirements.
Socket.io
Building real-time, event-based applications with Web Sockets and other real-time technologies is made easy with the help of the popular library Socket.io. It supports various platforms and technologies and offers a straightforward and user-friendly API for creating P2P applications in Node.js . Online games, chat programs, and other real-time applications that demand effective, low-latency communication are all developed using socket.io.
Developers can build a Socket.io server that waits for client connections to arrive to implement client-to-client communication in Node.js .
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
How to establish a basic client-client dialogue
It is possible to develop real-time applications with effective data transfer between clients without a central server by building a client-to-client communication system in Node.js . In this article, we’ll look at how to use Web Sockets and the Node.js ws library to create a basic client-to-client communication system.
Step 1: Setting up the environment
The development environment must be properly configured before the implementation begins. The ws library and Node.js should both be installed on developers’ computers. Use the npm (Node Package Manager) command to install the library:
npm install ws
Step 2: Creating the server
Making a Web Socket server is the first step in building a client-to-client communication system. Incoming connections from clients are listened for by the server, which then relays messages and data as necessary between clients.
We can create a new Web Socket server instance using the ws library to create the server:
const WebSocket = require("ws");
const wss = new WebSocket.Server({ port: 8080 });
Using port 8080 as its listening port , we create a new Web Socket server in this example. The Web Socket server instance is represented by the wss object .
Step 3: Handling client connections
After building the server, we must manage incoming connections from clients. Every time a client connects to the server, the ws library’s connection event is launched. This event allows us to manage incoming connections and save them for use later.
wss.on("connection", (ws) => {
// Handle incoming connections
});
To listen for the connection event in this example, we use the on method . The callback function is activated when a client connects to the server, and we can manage the connection by using the ws object .
Step 4: Broadcasting messages to all clients
We must repeatedly iterate over all connected clients and send the message to each one to broadcast messages to all connected clients.
wss.client.forEach((client) => {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
In this example, we iterate through all connected clients using the forEach method. The client’s state must be OPEN for it to be prepared to receive messages. We use the send method to send the message if the client is open.
Step 5: Handling messages from clients
We must watch for the message event on the ws object to manage incoming messages from clients. This event is triggered whenever a client sends a message to the server.
ws.on("message", (message) => {
// Handle incoming messages
});
In this illustration, the on method is used to watch for the message event . The callback function is invoked when a client sends a message to the server, and we can process the message using the message parameter .
Step 6: Forwarding messages to other clients
We must iterate over all connected clients and send the message to each client aside from the one that sent it in order to forward messages to other clients.
wss.clients.forEach((client) => {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
In this example, we iterate through all connected clients using the forEach method . The client must be in an OPEN state and not be the one who sent the message before we continue. We use the send method to send the message if the client satisfies both requirements.
Step 7: Testing the communication
We can test the system by connecting two clients and sending messages between them after the server and client communication logic has been implemented.
We can build two basic HTML files that connect to the server and communicate with one another using the WebSocket API to test the client-to-client communication system.
<!-- client.html -->
<!DOCTYPE html>
<html>
<head>
<title>Client</title>
</head>
<body>
<script>
const ws = new WebSocket('ws://localhost:8080'); ws.onmessage = (event) =>{" "}
{console.log(event.data)}; ws.onopen = () => {ws.send("Hello, server!")};
</script>
</body>
</html>
This example connects to the server at ws://localhost:8080 by creating a new WebSocket instance. The onmessage event is used to listen for incoming messages, and the send method is used to send messages to the server.
<!-- client2.html -->
<!DOCTYPE html>
<html>
<head>
<title>Client 2</title>
</head>
<body>
<script>
const ws = new WebSocket('ws://localhost:8080'); ws.onmessage = (event) =>{" "}
{console.log(event.data)}; ws.onpen = () => {ws.send("Hello, server!")};
</script>
</body>
</html>
In this example, we create a second instance of the WebSocket and connect to the server at ws://localhost:8080 . The onmessage event is used to listen for incoming messages, and the send method is used to send messages to the server.
We can check the console output to see if the messages are being sent and received correctly by opening both HTML files in two different browser windows.
Conclusion
Using Node.js , the ws library , and WebSockets , we looked at how to create a client-to-client communication system. These guidelines can help developers create dependable real-time applications that enable direct client-to-client communication without the need for a centralized server . The approach can be expanded to support a range of use cases and scenarios, from chat applications to multiplayer games, and more, even though this is just a simple example.