9 Common CSS Mistakes We Make
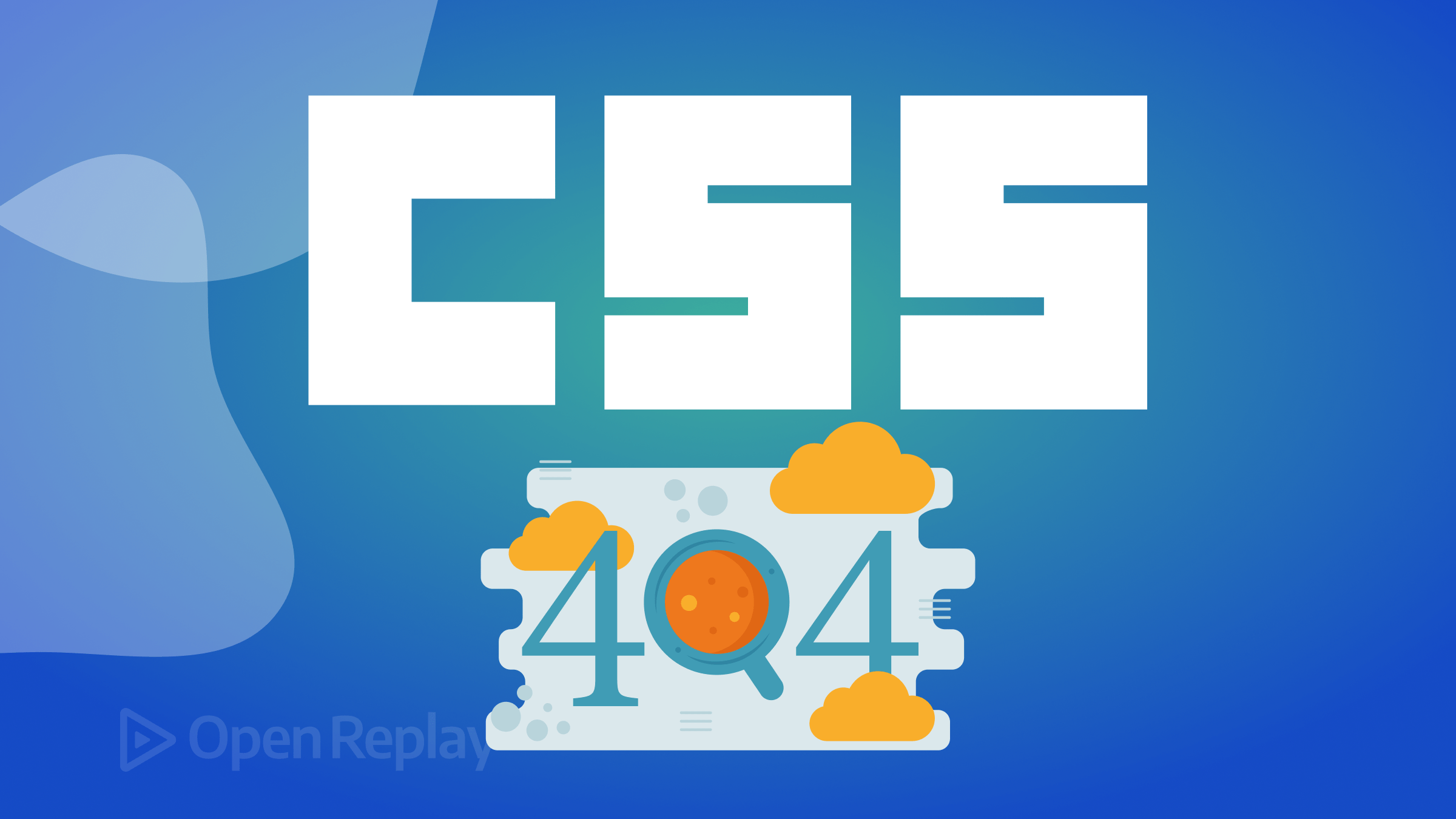
Cascading Style Sheet (CSS) is a powerful stylesheet language that helps front-end developers style a plain web page. However, there are mistakes developers can make while using this stylesheet language. These mistakes will hinder developers from writing efficient code. This article examines some common mistakes and offers a solution for each.
Discover how at OpenReplay.com.
The following are nine of the most common mistakes we developers often make; have you also made some of them?
Overusing “!important”
!important
is a keyword in CSS that helps us set a property value to take a higher preference (to be more important) than other styling options. For instance, if you apply the !important
declaration to an element style, that style will override all other styles for that property category.
Here is the syntax:
selector {
property: value !important;
}
For example, if you have a header element - h1
like this:
<h1 class="css-mistake">CSS Mistakes</h1>
Then you apply the following style rules:
h1 {
color: red;
}
.css-mistake {
color: green;
}
By default, the header text will have a color of “green” because the class selector has a higher CSS specificity selector than the element (tag) selector. But with !important
, you can override that styling CSS rule (thereby making the element (tag) selector override the class selector).
With this, the color of the header text will be red, taking precedence over green, which the class selector sets.
h1 {
color: red !important;
}
Overusing !important
will lead to specificity war - a state whereby styles begin to override each other and not work as the author of the stylesheet has defined them. The next section proffers a solution to this issue by itemizing the right instances for the usage of !important
.
When should it be used?
Just like the title of this section says - “Overusing !important
”, one must be careful with the usage of this keyword. !important
should be used sparingly, only when there is a dire need for it.
Here are some of those instances of its usage:
- In a stylesheet where you have a third-party library or framework, and you need your custom styles (the custom CSS written by you) to override styles from that library without you modifying the themes of that library.
- When you need to override some pre-defined styles to enhance accessibility. An everyday use case for this is when you try to make your website accessible to all users, including the visually impaired. For instance, you’ve set some color values that you eventually find challenging for visually impaired people (those with low vision) to resonate with. You can use
!important
to override the default color values. - If you encounter a layout issue that CSS style adjustments haven’t resolved effectively, you can employ
!important
to address that specific style problem. However, always remember that this approach should be considered a last resort. - It is essential that you know that some browsers have default styles for CSS. In this situation, you can use
!important
to override default styles, enabling style consistency across various browsers. - You can also use it for testing and debugging your stylesheet. If a style isn’t working, you can apply
!important
to enforce the desired style, overriding any conflicting styles. This approach lets you quickly pinpoint where the issue originates in your code.
Using Absolute Units
When styling, using the right length unit is essential for creating a responsive design. CSS has two categories of length units: absolute and relative. Many CSS properties like “width,” “height,” “font-size,” and so on utilize units for their value representation. The code snippet below gives an example of the usage of a unit:
selector {
font-size: 14px;
}
In the code snippet above, we declared the “font-size” CSS property and then assigned a value of 14px to it.
What are Absolute Units?
These are length units with fixed values irrespective of the rendering medium–regardless of the device’s screen size. These units are excellent at maintaining the same size for elements across various screen sizes. Some standard absolute units you would have worked with are pixels - px,
points - pt,
inches - in,
millimeters - mm,
centimeters - cm,
and picas - pc.
Absolute units provide us with accurate, fixed, and precise styling values, but they do not scale according to the screen size. Absolute units are unsuitable for achieving responsiveness. Therefore, restrict their usage to elements that don’t need to adapt to the browser’s dimensions or the device’s screen size.
On the other hand, relative units, as the name suggests, are relative to another property (in most cases, their parent element). These units are flexible in that they grow dynamically, adjusting according to the viewport, which makes them suitable for implementing responsive designs, unlike absolute units. Some examples of relative units are percentage-(%), rem,
em,
and so on.
How to use Relative units and their interpretation
Understanding the significance of each relative unit equips you with the knowledge to use them effectively. Here are some relative units alongside their explanations:
- % - this unit solely depends on the parent element. Thus, it is relative to the parent element. For example, if you set the width of an element to 80%, it will be 80% of the width of its parent element. Note, use percentage for the value of the following properties: width, height, margins, and paddings.
- em - this is relative to the parent element’s font size. For example, if you set the font size of an element to 4em, it will be four times the font size of the parent element. Use
em
when working with typography (text) - this lets the text scale proportionally. - rem - this is relative to the root element’s font size.
rem
is usually employed to achieve consistent styling across the entire layout. - vh - relative to 1% of the height of the viewport.
- vw - relative to 1% of the width of the viewport.
Using Inline Style
Inline style is a styling system that passes a direct style to an HTML element via the style
attribute. While this styling system exists, it’s essential to understand that its usage doesn’t support best practices for application building because it creates non-reusable code. Use inline style only when testing or beautifying a simple HTML file.;
Here are some reasons why you should avoid this styling system:
- With inline style, you do not get to follow the DRY (Do Not Repeat Yourself) principle. Inline style results in code repetition and non-reusable code because each element gets styled individually, even if it shares the same styling as another element. Inline style leads to redundant code.
- Code becomes challenging to read and also gets bloated. It results in a larger HTML file size, which affects performance. Also, your code becomes messy and not structured; hence, there should be a distinction between structure (HTML) and styling (CSS).
- It leads to a condition referred to as “Maintainability Complexity,” which essentially means that your code becomes challenging to upkeep. For example, if you need to modify the appearance of an element, you’ll have to locate the corresponding HTML tag and make direct style adjustments. Imagine a situation where inline styles are scattered across various locations; this approach won’t be suitable for scaling.
Best Practices
To overcome the drawbacks of inline style, you must use internal stylesheets (styles within the <style>
tag) or external stylesheets to keep your code healthy and organized.
- External Stylesheet: Create an external CSS file. Store your styles, then link your HTML with them. With this, you achieve a distinction between HTML and CSS code, making your styles maintainable and reusable.
- Internal Stylesheet: Right inside your HTML file, use the
<style>
tag inside your HTML document’s<head>.
Though this approach is inside the HTML file, you still achieve separating content (element) from presentation (styles).
Not using CSS Resets
Different browsers have various default styles, which vary, leading to inconsistent appearances of elements. This is why we must define some styles for a consistent starting point for styling web pages across other browsers. These styles are called “CSS Resets.”
CSS resets are CSS styles that serve as rules to remove the default styles that come with the browser. If you are conversant with CSS, and you would sometimes have in your journey noticed that there is a default style for some specific properties like margin.
CSS resets help us level our styling ground to have a consistent foundation.
Here are the reasons behind the use of these resets:
- Consistent Style: We can override browsers’ default styles with CSS resets, making the stylesheet consistent.
- To maintain control over the stylesheet, CSS reset guarantees that your styles derive influence from you—the stylesheet’s author—rather than your browser.
How to create your own CSS Resets
Here are simple steps to follow:
- First, you must identify the default styles you want to remove or override. This is the starting point because you must identify a problem before coming up with a solution.
- Then, target the identified styles and write your own CSS styles. These styles will serve as the rules that your stylesheet will adhere to.
- Finally, test your stylesheet on various browsers to ensure that your CSS resets take precedence over the browser-specific styles. To make things look less abstract, here is a simple CSS Reset implementation done with the universal CSS selector (*):
* {
margin: 0;
padding: 0;
border: 0;
}
The code above is a fundamental CSS reset that targets the margin, padding, and border properties, assigning a value of 0 to each. This process is called the “Normalisation process,” as it neutralizes the default spacing and borders the browser introduces. Here are some CSS resets online:
- Normalize.css
- Josh Comeau custom CSS reset - This is the one I currently use, and Josh has done great work by explaining this custom CSS rules explicitly
- HTML5 Boilerplate
- The popular Eric Meyer’s Reset CSS
Not using CSS one-liners
CSS one-liners are shorthand styles for writing CSS code. They make code cleaner and well-structured. Knowing these shorthands will help you write more concise code (compressing lines of code into a single line). For instance, when you want to style an element margin at all sides (top, bottom, left, and right), you don’t need to explicitly state all the properties like margin-top,
margin-right,
margin-bottom,
and margin-left,
you can simply use the shorthand for margin property. The code below explains that:
/* Don't do this */
margin-top: 10px;
margin-bottom: 10px;
margin-right: 15px;
margin-left: 15px;
/* Instead, use Margin Shorthand */
margin: 10px 20px 10px 20px;
/* Margin Shorthand can even be more simplified */
margin: 10px 20px;
With these one-liners come excellent benefits:
- Concise code: You can write shorter code that is neat and readable.
- Efficient code: You get to write efficient code, having reduced stylesheet file size and increasing performance.
- Maintainable code: Stylesheet becomes more maintainable and error-free. It will also be easy to alter styles and easily debug styles. To explore the full power of these one-liners, you must check out the available CSS one-liners. Here are a few curated resources to aid your adventure:
- Mozilla
- Plainenglish
- Web dev
Not using Selectors Efficiently
CSS selectors target HTML elements in an external or internal stylesheet. There are numerous selector methods in CSS, including tags (elements), classes, Ids, and pseudo-elements Knowing when to use a selector and when not to is vital. Also, you must know how CSS selectors work.
- Tag Selectors: these selectors target a particular HTML element using the tag name (for example:
div,
p
). They have the lowest specificity as they also result in broad styling, meaning they apply the style to all HTML elements with the specified tag. - Class Selectors: these selectors are more specific, unlike the tag selectors, since they use a class attribute applied to an HTML element. For example:(
.container
), (.header
). - Id Selectors: ID in our regular day-to-day activity is a unique value; likewise, Id selectors in CSS. Id selectors are unique Id attributes used to target a single or unique HTML element. For example, (
#name
), (#title
). They possess high specificity, and using them sparingly renders them unique. Above are a few CSS selectors. This guide expects that you are already conversant with CSS selectors. However, this guide still explains some common selectors and how they work. Now, what should you look out for when choosing a selector? - Specificity: Use selectors particular to your target elements. This will create a styling constraint and help avoid overly specific selectors, which can distort code reusability.
- Readability: The selector chosen should be easy to read and understand, helping us achieve clean code architecture.
- Minimal usage of the Id Selector: Keep Id selector usage as minimal as possible. Use it only for unique Ids. With its high specificity comes great complexity when used without caution.
- Use class selectors when you have a goal for reusable code.
How to avoid using overly complicated selectors
If you ever come across a code snippet like the one below in your stylesheet, then it means you are using overly complicated selectors.
#container > .box > content .section .title p > span .italic {
color: blue;
font-weight: bold;
}
Here are the tips on how to avoid overly complicated selectors:
- Engage the most specific selector for an element.
- Avoid nesting selectors - keep the hierarchy low.
- Use selector combinators for elements having the same style.
- Learn to do code refactoring often (you get to spot complex selectors).
- Employ CSS modules for code structure.
- Avoid using descendant selectors. For example, using (
ul,
li,
anda
) to select an element. It might be efficient, but with time, they eventually lead to overly complicated selectors.
Ignoring Browser Compatibility
Browser compatibility is important because you don’t want a style to function well on one browser and malfunction on another. Ignoring browser compatibility can lead to inconsistent user experiences across different browsers. This is because different browsers have their own way of rendering CSS styles. However, you can achieve style consistency across the board by considering browser compatibility and ensuring your styles are compatible with different browsers. One way to achieve browser-compatible code is by implementing the following:
- Using Vendor Prefixes: Some CSS properties require you to use prefixes for it to render irrespective of the browser. Some browsers that require these prefixes are Internet Explorer, Mozilla Firefox, and Safari. The prefixes are called vendor prefixes because they are unique to some specific types of browsers. Examples of the prefixes:
-webkit-,
-moz-,
and-ms-.
/* webkit-prefixed version - used by browsers that use the WebKit layout engine, such as Chrome and Safari- */
-webkit-border-radius: 8px;
/* ms-prefixed version -used by browsers that use the Microsoft layout engine, such as Internet Explorer. */
-ms-border-radius: 10px;
/* standard version */
border-radius: 8px;
- Declaring font fallbacks: You must add fallback fonts when assigning value to properties like “font-family.” The fallback font will be an option for what to implement if the custom fonts aren’t available.
/* This is a CSS code snippet utilizing fallback fonts (sans-serif) */
selector {
font-family: "Courier New", sans-serif;
}
- Leveraging the can I use website to check for CSS properties compatibility with various browsers.
Using Color names instead of Hex codes
Hex codes are the hexadecimal representation of colors. It is a 6-digit code prefixed with the “#” symbol followed by three pairs of hexadecimal digits, where the first pair represents the red value, the second pair represents the green value, and the last pair represents the blue value. Syntax:
selector {
color: #rrggbb;
}
Using direct color keywords has some limitations:
- Color names are inconsistent across browsers. If you set a color property to a value of green, for instance, how one browser interprets it differs from how another will. Hence, the rendering is inconsistent across various browsers.
- Color names are restricted because you can’t tweak them in tone, shadow, and the like.
- Color names have limited options. Due to the limitations of using color names, we need to opt to use Hex codes, which don’t have these limitations. Here are the reasons why Hex codes are more suitable compared to color keywords:
- Hex codes are precise and consistent across various browsers.
- Modern browsers widely support them
- They have a wide range of colors that are accessible to developers
- Not restricted in implementation - you can easily tweak them to suit your preference.
An efficient way of finding Hex codes
Here are a few ways by which you can get Hex codes:
- Using online color picker - open your browser and input this query - “online color picker,” then search it. On the page returned, you get a color picker.
- You can also get to learn about Hex code using color charts.
- Right in the browser developer tool in the console, you can also access a color picker tool.
- Lastly, you can check for resources online that have done proper research and curated a list of these Hex codes.
Additional mistakes we overlook
- Using 0px instead of 0: There is no doubt that this might not affect the stylesheet, but for best practice, ensure you use 0 and not 0px.
selector {
/*Don't do this*/
padding: 10px 12px 15px 0px;
/*Do this*/
padding: 10px 12px 15px 0;
}
- Setting a high value for z-index: Assigning a high value to
z-index
is very unnecessary and overkill. Sooner or later, this will cause an issue in your stylesheet, especially when there is more than one implementation ofz-index.
selector {
/*Don't do this*/
z-index: 50;
/*Do this*/
z-index: 2; /*Let the value be minimal*/
}
Conclusion
This article has ventured into the landscape of prevalent CSS errors, offering insightful solutions for each misstep. By reading this guide, there is no doubt that you have learned about some familiar CSS mistakes, and henceforth, you will know how to tackle them, as we also have to look into the solution for each mistake. Feel free to leave your thoughts in the comments below. If you found this article helpful, kindly share it with your network. Thank you.
References
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.