How to Convert a String to an Integer in JavaScript
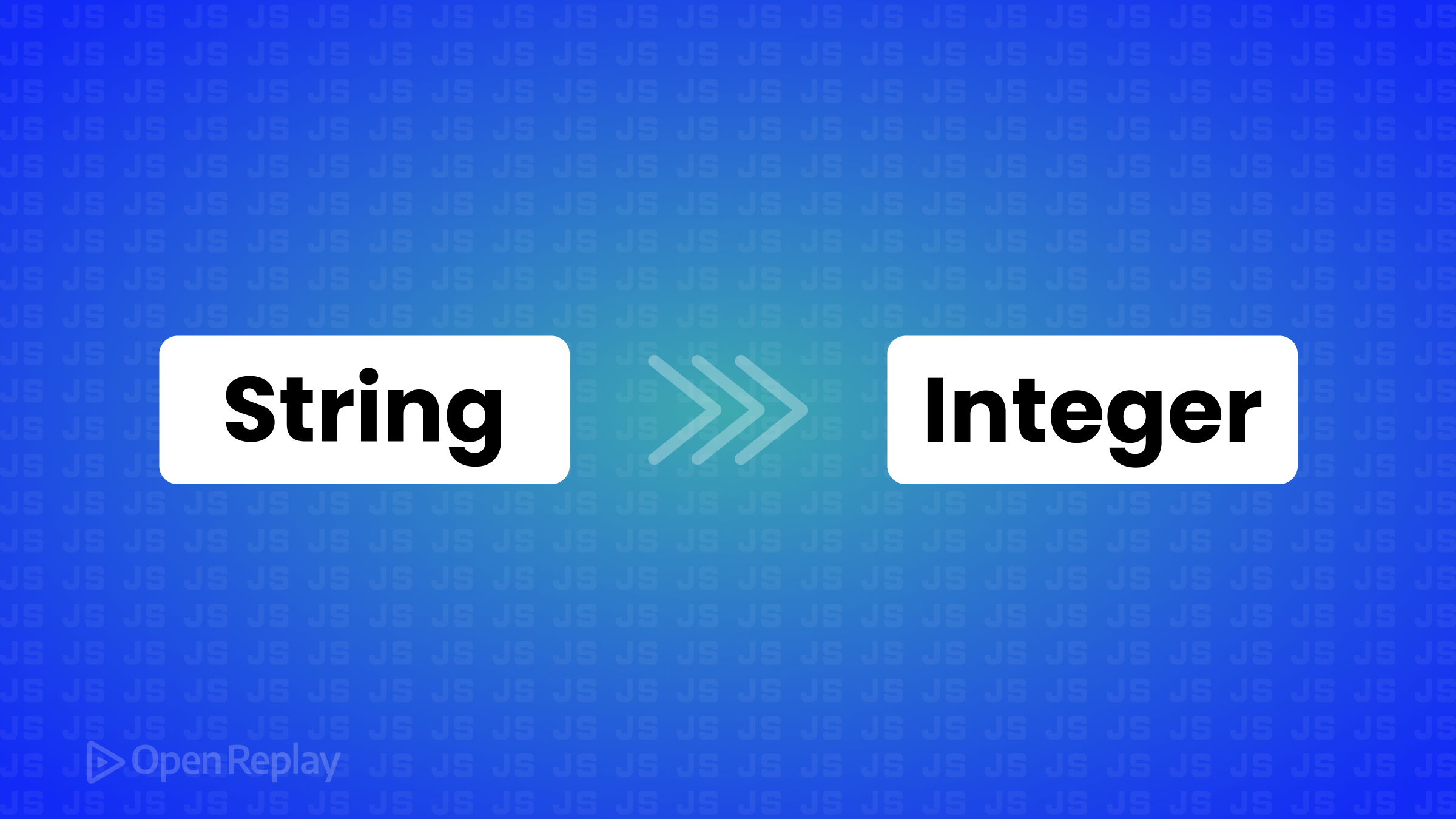
Converting a string to an integer is a common task in JavaScript. Whether you’re processing user input or working with data from APIs, JavaScript provides several easy-to-use methods for this conversion. This guide covers three reliable ways to convert strings to integers.
Key Takeaways
- Use
parseInt()
for flexible conversions with optional radix specification. - Use
Number()
for straightforward string-to-integer conversion. - Handle invalid input to prevent unexpected results.
1. Using parseInt()
The parseInt()
function parses a string and returns an integer. It also allows specifying the number system (radix) for conversion.
const str = ""42"";
const number = parseInt(str, 10);
console.log(number); // Output: 42
Key Details
- The second argument,
10
, specifies the radix (base 10). Always include it to avoid unexpected behavior with non-decimal numbers. - If the string starts with non-numeric characters,
parseInt()
ignores them until it encounters numbers:
parseInt(""42abc"", 10); // Output: 42
parseInt(""abc42"", 10); // Output: NaN
2. Using Number()
The Number()
function converts the entire string into a number. Unlike parseInt()
, it does not ignore non-numeric characters and fails if the string contains invalid data.
const str = ""42"";
const number = Number(str);
console.log(number); // Output: 42
Key Details
- Returns
NaN
if the string contains invalid characters:
Number(""42abc""); // Output: NaN
- Works best when the string is strictly numeric.
3. Using the Unary +
Operator
The unary +
operator is a shorthand way to convert a string into a number. It’s concise and works like the Number()
function.
const str = ""42"";
const number = +str;
console.log(number); // Output: 42
Key Details
- Like
Number()
, it converts the entire string and returnsNaN
for invalid input:
+""42abc""; // Output: NaN
Handling Invalid Input
To avoid unexpected results, always check if the conversion is valid using isNaN()
:
const str = ""42abc"";
const number = parseInt(str, 10);
if (isNaN(number)) {
console.log(""Invalid number"");
} else {
console.log(""Valid number:"", number);
}
Conclusion
Converting a string to an integer in JavaScript is simple with parseInt()
, Number()
, or the unary +
operator. Choose the method that fits your use case, and always handle invalid input to ensure reliable results.
FAQs
For `parseInt()` and `Number()`, an empty string will return `NaN`. Always validate input before conversion.
`parseInt()` truncates the decimal part, while `Number()` and `+` handle floating-point numbers directly.
`parseInt()` stops parsing when it encounters invalid characters, while `Number()` fails completely if the string isn’t fully numeric.