Creating Animations with React Spring
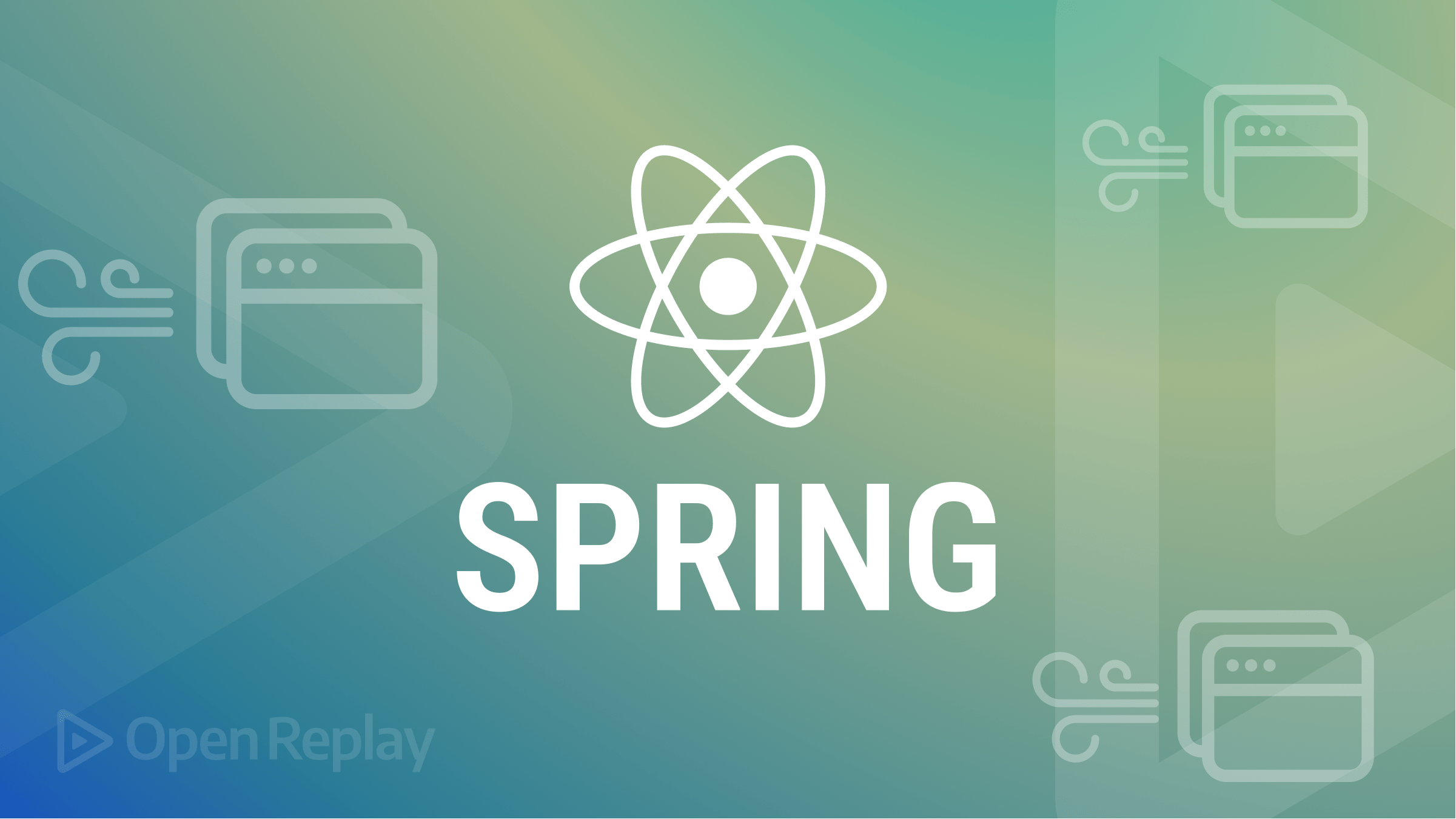
Animations are integral to any UI and are very useful in capturing a user’s attention. There are many popular animation libraries for React, and in this article, we will discuss one of the most powerful and popular ones: React Spring.
React Spring and Spring-Based Animations
React Spring is an animation library that uses spring physics to create motion effects instead of the more commonly used time-based animations. So, what are spring-based animations? Spring-based animations use spring physics, which involves mass, tension, and friction. Spring animations are much more fluid and organic than time-based animations, which means they are a lot smoother and feel more “real”.
The Basics
React Spring uses two main components, Spring Values, and the animated component. The animated component is a higher-order function that is a React component that can be used like any other JSX element. Example:
import { animated } from '@react-spring/web'
export default function TestComponent() {
return (
<animated.div/>
)
}
This animated component can be anything: a div, image or heading, etc.
The animated component will take SpringValues
through the style prop and then update the component without re-rendering.
The Main React Spring Hooks
React Spring provides multiple React Hooks that allow us to animate data values:
useSpring
This is the simplest hook. It is used to animate a component from one point to another. It has many useful props, but the main ones are:
- from: The initial value
- to: The final value or point
- loop: whether you want to run the animation infinitely or not.
- delay: Provide a time delay before the animation starts.
- config: We can use more properties such as duration, mass, tension, etc.
Let’s create a simple animation using this hook:
Results are:
As you can see, in the above example we are simply animating a div to move from top to bottom. We can give more props, like giving it a duration and making it loop.
useSprings
This is the same thing as useSpring
, the only difference being that you can animate multiple elements or springs. An everyday use is for animating lists. We create a spring object that can be mapped out, and we can specify the number of springs we need to create.
Let’s look at an example:
This is produced:
In the above example, we have two div’s moving up and down. We first create a springs array using the hook to achieve this. We specified the number of items in the hook and then passed a function. The function has four props: from, to, config, and loop, and these were discussed earlier. Then, we map out the array and create an animated div for each item.
useTrail
Similar to the useSprings
hook. However, here we have multiple springs that follow the first one. The first list item is animated, and the rest of the siblings follow it in a trail.
Let’s look at an example:
This is the result:
Similar to useSprings
, we are creating a trails array. The hook implementation is identical to useSprings
.
useChain
useChain
allows us to chain multiple animation hooks together to form a sequence. We can specify the order of each animation. For this hook, we will use refs
. The hook uses an array of refs
to determine the order in which the animation will run.
In this example, we will animate a few boxes to drop down like the previous examples and simultaneously change their colors. For this, we will first have to run a useSpring
hook, then a useTrail
hook, and lastly, we will run the useChain
hook with the refs
from the above-mentioned hook. Refs
will be created for both the trail and the spring.
This is generated:
useTransition
This hook is used to create animated transition groups. It is commonly used for showing/hiding, mounting, and unmounting transitions on datasets. Transitions such as fade in/out or slide in/out are common examples.
The useTransition
hook has a couple of custom props in addition to the ones found in all the above-discussed hooks, and it does not have the to
prop.
- initial: The initial state/value
- enter: When the animation is entering
- leave: When the animation is finishing
Let’s look at an example of creating a simple fade in animation. Once we click the button, each box will fade out, and the next one will come.
Similar to the useSprings
and useTrail
hooks, we are using an items array for the useTransition
hook. We store the index of the current item in a state. Each time the button is clicked, we increment the state. The modulus operator ensures that once we reach the last item, the state automatically gets set to the first index.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Scroll Animations with React Spring
React Spring also provides a couple of hooks to assist in creating scroll animations, which play an important role in an interactive user experience.
The useScroll
Hook
The useScroll
hook provides either the exact value of how much the user has scrolled or a progress value between 0 and 1. You can get the scrolling info about both the X and Y directions.
Let’s create a simple effect in which the size of a box increases as you scroll down. For this, we will simply get the scrollYProgress
and add 1 to it. Then we will use this value for the transform scale property. We are adding 1 as we want to scale up from 1 onwards. We will use an onChange
in the hook to get the value each time the user scrolls and then store the value in a state.
The useInView
Hook
React Spring also provides the useInView
hook, which targets an element once it is visible in the viewport. This is useful for creating scroll reveal animations like images sliding in once a user scrolls to them.
We can either use it to get a boolean value or pass a function to create spring animations. We shall use the former for our example. As you scroll down, more boxes will fade into the viewport.
We are creating refs
for each item separately. If the inView
is true, the opacity is set to 1 and vice versa.
Conclusion
In this article, we’ve seen how to do impressive animations with React Spring; give it a try!