Automatically Creating Pull Requests on Every Push
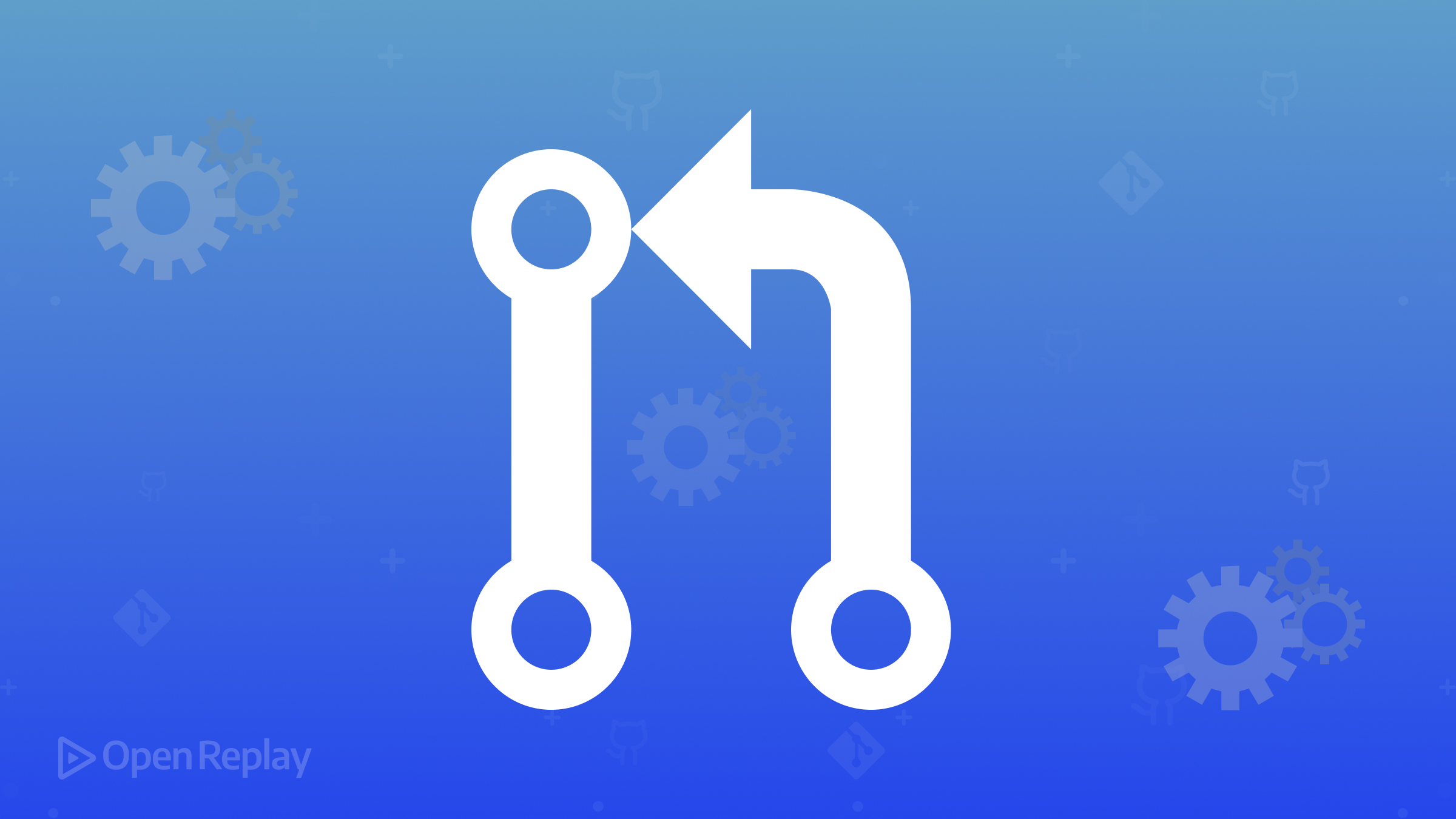
Git automation can significantly streamline your development workflow. This guide explores how to automatically create pull requests whenever code is pushed to your repository, using various tools and approaches.
Key Takeaways
- Learn three different methods to automate PR creation: GitHub Actions, GitHub CLI, and CI/CD tools
- Implement secure automation practices with proper authentication and permissions
- Understand best practices for managing automated pull requests
- Set up branch protection and conditional PR creation logic
Method 1: Using GitHub Actions (Recommended)
GitHub Actions provides a native, reliable way to automate PR creation. Here’s how to set it up:
Step 1: Create a GitHub Actions Workflow
- Create a directory
.github/workflows/
in your repository if it doesn’t exist - Create a new file called
auto-pr.yml
- Add the following configuration:
name: Auto PR on Push
on:
push:
branches:
- 'feature/**' # Matches feature/* branches
- 'bugfix/**' # Matches bugfix/* branches
# Add more branch patterns as needed
jobs:
create-pull-request:
runs-on: ubuntu-latest
permissions:
contents: write
pull-requests: write
steps:
- name: Checkout repository
uses: actions/checkout@v4
with:
fetch-depth: 0 # Required for proper branch comparison
- name: Create Pull Request
uses: peter-evans/create-pull-request@v6
with:
token: ${{ secrets.GITHUB_TOKEN }}
commit-message: ""Auto-created PR from ${{ github.ref_name }}""
title: ""Auto PR: ${{ github.ref_name }}""
body: |
Automated pull request created from push to ${{ github.ref_name }}
Branch: ${{ github.ref_name }}
Triggered by: ${{ github.actor }}
branch: ${{ github.ref_name }}
base: main
labels: |
automated-pr
needs-review
Step 2: Configure Repository Settings
- Go to your repository’s Settings → Actions → General
- Under ""Workflow permissions"", select ""Read and write permissions""
- Save the changes
Method 2: Using GitHub CLI
For developers preferring local automation, GitHub CLI offers a straightforward solution.
Step 1: Install GitHub CLI
Choose your platform:
- macOS:
brew install gh
- Ubuntu/Debian:
sudo apt install gh
- Windows:
winget install GitHub.cli
or download from<https://cli.github.com>
- Other Linux: Follow instructions at
<https://github.com/cli/cli#installation>
Step 2: Authenticate GitHub CLI
gh auth login
Step 3: Set Up Post-Push Hook
Create and configure the post-push hook:
#!/bin/bash
# Get current branch name
BRANCH=$(git rev-parse --abbrev-ref HEAD)
# Don't create PR for main/master branches
if [[ ""$BRANCH"" == ""main"" ]] || [[ ""$BRANCH"" == ""master"" ]]; then
exit 0
fi
# Check if PR already exists
PR_EXISTS=$(gh pr list --head ""$BRANCH"" --json number --jq length)
if [ ""$PR_EXISTS"" -eq 0 ]; then
# Create PR if it doesn't exist
gh pr create
--base main
--head ""$BRANCH""
--title ""Auto PR: $BRANCH""
--body ""Automated pull request created from $BRANCH""
--label ""automated-pr""
fi
Method 3: Using CI/CD Tools
For teams using other CI/CD platforms, here’s how to implement PR automation using GitHub’s REST API.
Jenkins Pipeline Example
pipeline {
agent any
environment {
GITHUB_TOKEN = credentials('github-token')
}
stages {
stage('Create PR') {
steps {
script {
def branchName = sh(
returnStdout: true,
script: 'git rev-parse --abbrev-ref HEAD'
).trim()
// Don't create PR for main/master
if (branchName != 'main' && branchName != 'master') {
def response = sh(
returnStdout: true,
script: """"""
curl -X POST
-H 'Authorization: token ${GITHUB_TOKEN}'
-H 'Accept: application/vnd.github.v3+json'
https://api.github.com/repos/${GITHUB_OWNER}/${GITHUB_REPO}/pulls
-d '{
""title"": ""Auto PR: ${branchName}"",
""head"": ""${branchName}"",
""base"": ""main"",
""body"": ""Automated pull request created from Jenkins pipeline""
}'
""""""
)
}
}
}
}
}
}
Best Practices and Security Considerations
- Enable branch protection rules for your main branch
- Create standardized PR templates
- Use labels to distinguish automated PRs
- Set up automatic reviewer assignment
- Store tokens securely using repository secrets
- Limit token permissions to required scopes
- Regularly rotate authentication tokens
- Review automated PR settings to prevent sensitive data exposure
Conclusion
Automating pull request creation can significantly improve your development workflow by ensuring consistent code review processes and reducing manual effort. Whether you choose GitHub Actions, GitHub CLI, or CI/CD tools, implementing these automation techniques will help streamline your development process and maintain better code quality control.
FAQs
Automating PR creation ensures consistency in your code review process, saves time, reduces human error, and helps maintain a clear history of code changes. It's especially useful for teams working on multiple features simultaneously.
GitHub Actions is recommended for most users as it provides native integration with GitHub, requires minimal setup, and offers robust features. However, if you prefer local automation, GitHub CLI is a good alternative, while CI/CD tools are better for complex enterprise environments.
The provided scripts include checks to prevent duplicate PRs. For GitHub CLI, we check existing PRs before creating new ones. With GitHub Actions, you can use the `peter-evans/create-pull-request` action which handles this automatically.
Yes, you can customize the PR template by modifying the body parameter in any of the methods. For labels, you can add them in the GitHub Actions workflow or CLI command. You can also set up repository-wide PR templates.
Use repository secrets for storing tokens, implement proper permission scoping, and regularly rotate credentials. For GitHub Actions, use the built-in GITHUB_TOKEN when possible. For CLI and CI/CD tools, create dedicated access tokens with minimal required permissions.