CSS Refactoring Techniques
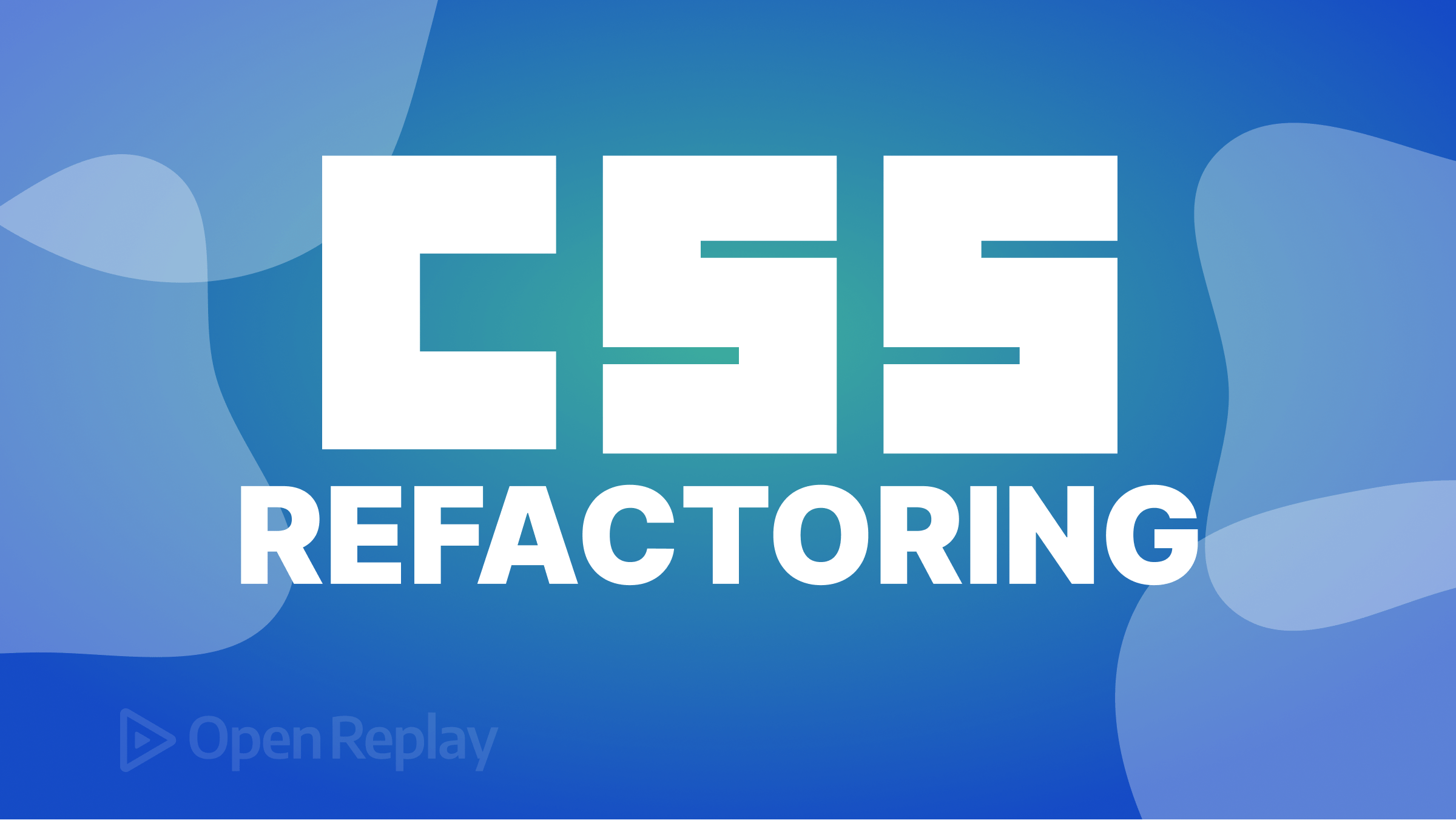
In the ever-evolving world of web development, with CSS being the key to styling, mastering CSS refactoring techniques is crucial for creating efficient and maintainable stylesheets. Refactoring CSS allows you to streamline your code, eliminate redundancy, and improve overall code quality. Applying the right techniques can transform tangled stylesheets into well-organized, modular, and easily maintainable code. This article will show you techniques for refactoring your CSS.
Design is not just what it looks like and feels like. Design is how it works.” — Steve Jobs, co-founder of Apple, Inc.
This comprehensive guide to mastering CSS refactoring techniques will equip you with the knowledge and tools necessary to refactor your CSS code. We’ll start by understanding the importance of CSS refactoring and its impact on performance and development workflow. Then, we’ll delve into techniques for refactoring CSS.
Ready to take your CSS skills to the next level? Let’s dive into the world of mastering CSS refactoring techniques and unlock the full potential of your stylesheets!
What is CSS Refactoring?
This refers to how we reorganize our CSS codebase to improve its readability, performance, reusability, and efficiency while maintaining its functionality.
CSS refactoring helps address several issues in web development and offers several benefits. Some of those benefits include:
-
Code maintainability: CSS refactoring plays a major role in creating maintainable code by organizing the codebase, making it easier to navigate through code and identify and remove unwanted code.
-
Code reusability and modularity: With CSS refactoring, we can easily apply consistent styles across different parts of a website and reuse certain styles if new features are added to our application in the future.
-
Enhanced collaboration: A well-refactored CSS improves collaboration among developers by using these conventions, making it easier for team members to work together.
-
Performance optimization: This is one major benefit of CSS refactoring; through refactoring, you can optimize CSS for improved performance leading to faster page load times, a better user experience, and improved search engine rankings.
-
Scalability and future-proofing: CSS refactoring helps create a flexible and adaptable codebase. Refactored CSS also makes it easier to add new design trends and technologies in the future.
Principles of CSS Refactoring
By following certain principles and best practices, developers can ensure their CSS code remains clean, organized, and efficient. Here are some of the principles:
- Single Responsibility Principle(SRP)
- Separation of Concerns principle
- DRY Principle (Don’t Repeat Yourself)
Single Responsibility Principle (SRP)
Just as the name implies (Single Responsibility), with this principle, we assign each selector a single responsibility, focusing on styling a specific element or group of elements. This principle promotes both modularity and reusability of our CSS code, making it easier to maintain and update styles in the future.
Take this CSS code as an example:
.container {
width: 500px;
height: 500px;
background-color: #333;
padding: 20px;
margin: 0;
}
As seen above, this selector is responsible for setting the width
, height
, background-color
, padding
, and margin
properties of the .container
element. This is too much responsibility for a single selector, making the code difficult to maintain and reuse.
A better way to write this CSS would be to use separate selectors for each property just like this:
.container {
width: 500px;
height: 500px;
}
.container__background {
background-color: #333;
}
.container__padding {
padding: 20px;
}
.container__margin {
margin: 0;
}
The code above is longer, yes, but in the long run, it is the right way to go as it is easier to maintain and extend. With each selector handling one property, we can easily change the styles of our properties.
Obviously, there are instances where adding different properties to a single selector is okay. Still, for something like a container selector, which most probably will be used in multiple elements, the SPR
principle is just the way to go.
Separation of Concerns Principle
This principle focuses on separating our CSS from HTML markup and any programming functionality. This simply means our style stays in our stylesheet.
With this principle, we enhance code maintainability because we won’t go through the stress of finding the styles that affect our code because we will know exactly where to look.
And yes, I get it; sometimes, inline styling may be great, especially for styles that won’t need changing or modification, but in my experience, it’s not a good practice.
DRY Principle (Don’t Repeat Yourself)
This principle is popular, not just in CSS but in coding at large. It is all about avoiding duplicating styles by consolidating common properties into reusable classes.
A common way this is done is through the use of CSS variables. I will explain CSS variables in depth later in this article, but for now, just know that they help ensure consistent styling across the website or application, and it is a key principle you should definitely consider when refactoring CSS.
Best Practices of CSS Refactoring:
What is worth doing is worth doing well. Let’s take a look at some best practices for CSS refactoring.
Organize Stylesheets
Having different stylesheets for different application components might seem unnecessary. Still, it’s one of the best ways to refactor your code by dividing your CSS into smaller, logical modules or files based on functionality, components, or pages. This improves code maintainability and readability, making locating and updating specific styles easier.
Use a Consistent Naming Convention
What is worth doing is worth doing well. Let’s take a look at some best practices for CSS refactoring.
Naming conventions help enhance collaboration among coders. Naming conventions provide guidelines for structuring and naming CSS classes and selectors to promote code organization, modularity, and reusability. Here is a list of some of these naming conventions:
- BEM (Block Element Modifier)
- SMACSS (Scalable and Modular Architecture for CSS)
- OOCSS (Object-Oriented CSS)
- Atomic CSS
- SuitCSS
The list above seems strange, right? It might shock you to know that you might have already been using these naming conventions unconsciously, probably because they were used in the tutorial course you took while learning how to code. A common example of the selector’s name is btn
, bg-dark
, cta
, and is-hidden
.
Naming conventions are great to use and are useful to make collaboration easier. You can learn more about them here
Reduce Specificity
Avoid using overly specific selectors that target individual elements. You might be wondering what overly specific selectors are. Here is a code example to show you.
<!DOCTYPE html>
<html>
<style>
div.container > ul.menu li:nth-child(3) a {
color: green;
font-weight: bold;
}
</style>
<body>
<div class="container">
<ul class="menu">
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#" class="highlight">Contact</a></li>
</ul>
</div>
</body>
</html>
As you can see from the code, to target the third a
tag, we specified all the paths explicitly that lead to it. This code can be refactored to follow best practices using classes and semantic HTML elements.
Here is what the refactored style looks like.
<style>
.container .menu li .highlight {
color: green;
font-weight: bold;
}
</style>
Both styles have the same function: to make the a
tag with the content (contact) of the last list item bold and have a green color. But the second way is just the right way to go about it.
Just in case you are interested in the result, here it is.
Remove Redundant Code
What’s the point of having CSS code that performs no functions? Always review CSS files to identify and eliminate unused styles. Doing this reduces file size, improves loading times, and saves yourself the confusion caused by conflicting styles.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
CSS Refactoring Techniques
In this section, we will look at some popular techniques we can use to refactor our CSS codebase.
Extracting reusable CSS patterns
This technique focuses on identifying recurring styles in your CSS codebase and extracting them into reusable classes.
To illustrate how this works, let’s consider this code:
<!DOCTYPE html>
<html>
<head>
</head>
<style>
.btn {
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
}
.button-primary {
background-color: #0088cc;
color: #fff;
}
.button-secondary {
background-color: #eee;
color: #333;
border: 1px solid #ccc;
}
</style>
<body>
<button class="btn button-primary">Click me</button>
<button class="btn button-secondary">Cancel</button>
</body>
</html>
The code above is a simple example of reusable CSS code patterns. We have a reusable class, btn
, which we used in all our button
elements. The class holds styles that should be present in all our buttons; rather than repeating the style on each button we create, we simply use the reusable CSS code technique.
Here is what our result looks like:
As you can see, the two buttons having the
btn
class possess the style assigned to the class (padding
, font-size
, and border-radius
) and still have their own specified style, which was assigned by the button-primary
and button-secondary
classes respectively.
Effective use of CSS Variables.
CSS variables, also known as CSS custom properties, are a feature in modern CSS that allows you to define and reuse values throughout your codebase. It is a great way to enhance code maintainability, improve consistency, and make it easier to update styles.
CSS variables work by defining a custom name in a :root
selector, which makes it globally available within our CSS, and assigning a value to it.
Here is an example:
:root {
--primary-color: #adc2ef;
--body-color: #eeaaaa;
--body-font: monospace;
}
aa With this style above, you can reuse them just by referring to their custom name, just like this.
<html>
<head> </head>
<style>
:root {
--primary-color: #adc2ef;
--body-color: #eeaaaa;
--body-font: monospace;
}
button {
background-color: var(--primary-color);
}
p {
font-family: var(--body-font);
color: var(--body-color);
font-size: 20px;
}
</style>
<body>
<p>Click this button</p>
<button>Cancel</button>
</body>
</html>
As seen in the code, we used our variable as the values of different properties (background-color
, font-family
, color
) in our code, and here is the result we got.
CSS variables are very common in the modern world; they are commonly used to set things like primary and secondary color values, store the different font-family
that will be used in code, and so on. This way, you can refactor your code, so if you want to change the primary color of your website, you don’t need to look at all the places where you used the color; you can simply change it from the root component and it changes in any part of our CSS codebase where we used the variable.
Tools and Resources for Refactoring
There are various modern-day tools and resources that can assist you in optimizing and refactoring CSS code. Here are some of them.
CSS linting and formatting tools
These tools are used to find and fix issues in code and maintain consistent code style throughout projects. They analyze code based on certain rules and configurations we have set and then refactor. A popular example of this tool is Prettier, which most developers use to automatically format their code. Other popular example is Stylelint and CSSLint.
CSS Minification and Compression Tools
CSS minification is the process of reducing our CSS file size by removing unnecessary code. On the other hand, CSS compression reduces our CSS file size by applying algorithms that compress code. All this helps to improve the performance of our application. Some tools we can use to achieve this include:
- UglifyCSS: This tool is used to minify CSS code; it optimizes code to reduce its size while retaining all its functionalities. Another code minification tool is CSSNano, which performs the same function.
- Online Compressors: We use online tools to perform code minification, such as CSS Minifier, without additional installations. They are mostly for one-time use.
Utilizing browser DevTools for CSS debugging and profiling
Browser Developer Tools is a very useful tool that can come in handy when trying to identify and resolve CSS-related issues and can assist in refactoring efficiently.
The DevTools allow us to inspect our code and modify the CSS properties of specific elements in real time. It helps that we modify CSS properties directly in the browser to test and debug changes before updating our codebase. Here is a table of shortcuts to access the DevTools on different browsers and devices.
Browser | Shortcut | Devices |
---|---|---|
Chrome | F12 | All devices |
Firefox | Ctrl+Shift+C | All devices |
Edge | Ctrl+Shift+J | All devices |
Safari | Cmd+Opt+I | macOS |
Safari | Ctrl+Shift+I | Windows, Linux |
Opera | Ctrl+Shift+I | All devices |
Vivaldi | Ctrl+Shift+I | All devices |
Introduction to popular CSS frameworks and libraries for refactoring
Not everyone likes frameworks; I personally prefer regular vanilla CSS, but when talking about refactoring, we must give accolades to CSS frameworks.
CSS frameworks offer many pre-built components, responsive grids, and predefined styles that significantly accelerate development and streamline refactoring.
There are lots of CSS frameworks out there that help streamline your refactoring process, but the most popular are Bootstrap, Tailwind CSS, Material UI, and Semantic UI.
Strategies for Large-Scale CSS Refactoring
You might wonder what the difference is between regular and large-scale refactoring. Still, it is simple: in large-scale refactoring, you are dealing with a larger codebase, which can be complex and challenging. However, with some tips you will see here, you can refactor your large-scale CSS code base effectively.
Planning and Prioritizing Refactoring Efforts:
The first step in embarking on a large-scale CSS refactoring project is planning.
Here are some stop-takes while you are in the planning phase of your refactoring process:
-
Analyze the existing codebase; this involves looking for duplication, inconsistent styles, outdated or unused selectors, and overall code complexity.
-
Set clear objectives that you wish to achieve by refactoring your codebase.
-
Break down the refactoring project into manageable tasks or modules.
-
Create a roadmap that will serve as a guide through the sequence of refactoring tasks, estimated timelines, and dependencies.
Establishing a Refactoring Workflow:
The next step to streamlining our large-scale CSS refactoring is establishing a well-defined workflow. Here are the ways this can be done:
-
Version control: This great tool allows us to track our changes, revert if necessary, and collaborate effectively with other team members. This can be achieved using different platforms such as Github, GitLab, Bitbucket, etc.
-
Branching strategy: This strategy isolates the development of refactoring tasks without affecting the main codebase. Once completed and tested, use branches for each refactoring task and merge them back into the main branch. This can be done using our version control software.
-
Documentation: while performing a large-scale refactoring process, documentation of the process helps keep records and serves as a reference for future maintenance.
Testing and Regression Strategies During Refactoring:
Refactoring CSS on a large scale may result in unanticipated problems and regressions. Here are some strategies to prevent that:
- Automated testing tools: In large code bases Leveraging automated CSS testing tools that analyze the codebase for potential issues is very important. Tools like CSSLint and StyleLint, which have already been discussed in the Tools and Resources section, are used for automated testing.
- Unit tests: These are designed to ensure every individual unit of our CSS code behaves as it should. It is a way to validate that the refactored code behaves as expected.
- User acceptance testing: This testing process involves users or other team members performing an acceptance test on the refactored CSS. This goes a long way toward providing valuable insights into any issues related to usability, accessibility, and functionality.
Conclusion
In conclusion, CSS refactoring is a crucial aspect of maintaining not just a well-structured but also an efficient codebase, and by understanding the principles and best practices of CSS refactoring, developers can significantly improve the readability, maintainability, and scalability of their code.
Various refactoring techniques have been discussed, including the extraction of reusable CSS patterns, simplification through selectors, and effective use of CSS variables, which empower developers to streamline their code.
To aid in the refactoring process, we discussed a range of tools and resources available. And we looked into large-scale CSS refactoring and the tips to refactor large-scale applications efficiently.
To be a good front-end developer, you must embrace good CSS refactoring practices, as they will ultimately contribute to a better user experience for your users and the long-term success of your web projects.