Date and Time Handling with Luxon
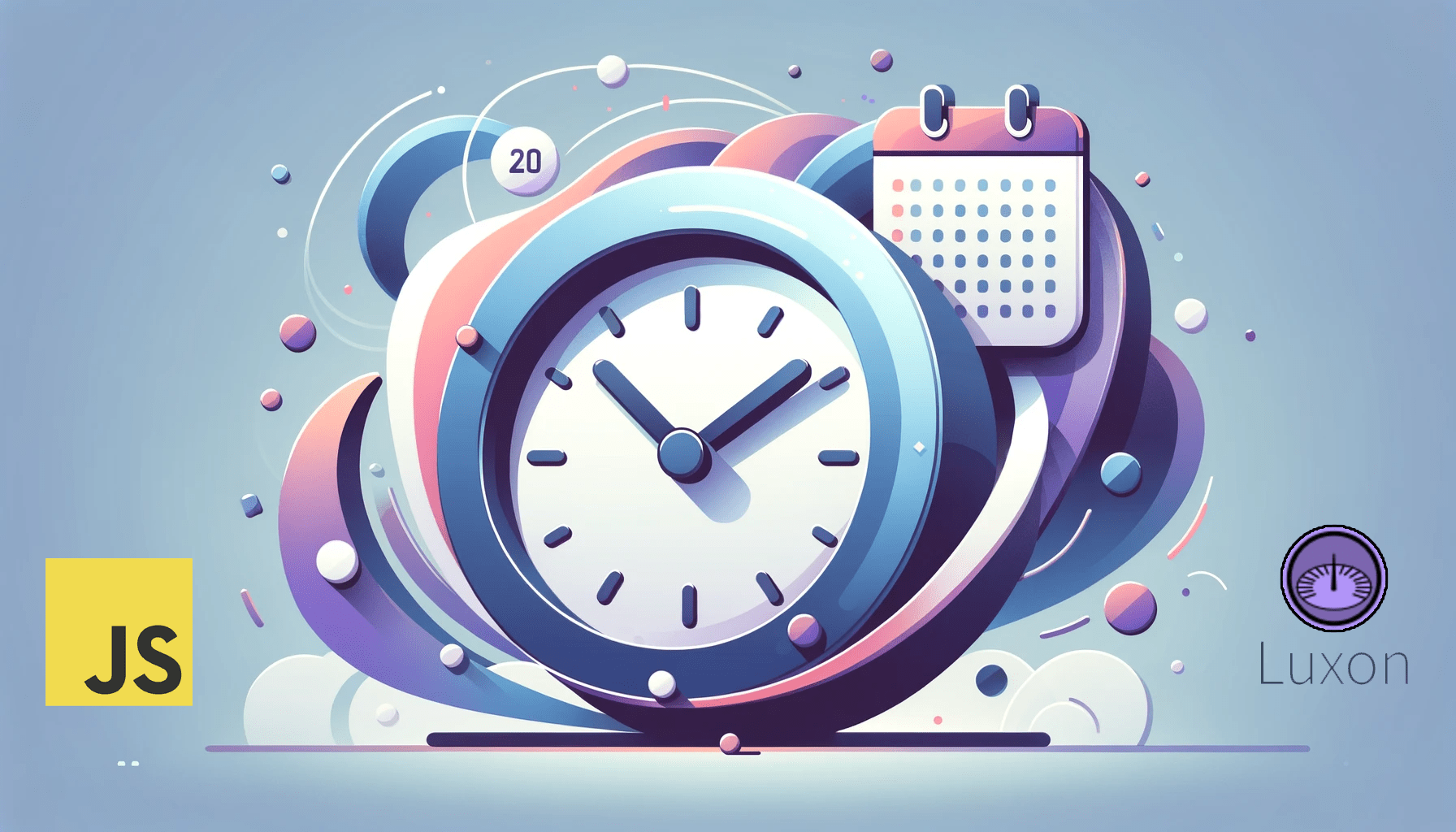
Luxon.js is a fully open-source JavaScript library designed for time and date manipulation. In this ever-evolving web development landscape, accurate date and time representation remains crucial for various applications, from scheduling events to handling data analysis spanning various time zones. Luxon.js steps in as a comprehensive solution, offering an intuitive API that not only simplifies the process but also ensures precise and reliable results, and this article will show you how to use it.
Discover how at OpenReplay.com.
Hello folks! In this blog post, I will walk you through using Luxon.js to manipulate dates and times in your JavaScript application.
Luxon is a result of continuous improvement in date-time libraries. It was created by the same team responsible for Moment.js, a widely popular JavaScript library for manipulating dates. However, as the JavaScript ecosystem advanced, Moment.js became a huge library with a big bundle size for just date and time manipulation. Additionally, it was lagging in terms of performance and features. This led to the emergence of Day.js, which had a smaller bundle size and more features than moment.js, and as a result, it addressed Moment’s shortcomings. The JavaScript developer community shifted from using the moment to day.js; however, even Day.js had its limitations, especially in timezoning, prompting some engineers in the Moment team to start fresh and develop Luxon. As a result, Luxon combines the knowledge gained from Moment.js and Day.js, offering a modern, robust, and feature-packed solution for manipulating dates and times.
Installation through a CDN
To get started, we need to install the Luxon library in our working environment. Two major ways of installing Luxon in an application are via a CDN or npm install. The installation type entirely depends on your application’s tech stack and the Luxon library’s purpose. Now, when running an HTML vanilla JavaScript application, you will install Luxon via a Content Delivery Network (CDN). For that, in your HTML <head>
, include the link to the Luxon library below.
<script src="https://cdn.jsdelivr.net/npm/luxon@3.4.3/build/global/luxon.min.js"></script>
Having done that, you can now access the various Luxon classes to confirm everything is good; in your Javascript file, you can have the line of code below just to print out the date, the time, and the zone.
//test
const DateTime = luxon.DateTime;
const now = DateTime.local();
console.log(`the time is ${now.toISO()}`);
Installation via NPM
If you run a Javascript front-end library or a build tool such as Webpack, npm install would be a more precise way to install Luxon. To install, open your terminal on your working directory and run the below command.
npm install --save luxon
The —save option instructed NPM to include the package inside of the dependencies section of your package.json.To utilize the Luxon classes, we bring it in via the line below.
const { DateTime } = require("luxon");
The ES6 syntax is written below.
import { DateTime } from "luxon";
Passing and formatting dates
Luxon provides a robust API for working with dates and times in JavaScript. Now, to pass the date, let’s first create a date instance.
const date = luxon.DateTime.local();
We have used the Datetime
luxon class, which will give us the date and time based on your device’s current date and time. You can also define a specific date via a parameter to the Datetime
class.
const birthDay = luxon.DateTime.local(2023,9,7,11,0);
The above code stores a date variable for September, date 7, and the time, which is 11:00 p.m.
Now, to format the date, we will use the format
luxon method. The function allows us to format dates according to specific patterns.
const date = luxon.DateTime.local(2023, 9, 7, 12, 0);
const formattedDate = date.toFormat('MMMM dd, yyyy hh:mm a');
The above function will output “September 07, 2023, 12:00 PM”. You can adjust the format by rearranging the year, month, day, hours, and minutes dummy variables. Luxon also provides some predefined formats.
const date = luxon.DateTime.local(2023, 9, 7, 12, 0);
const formattedDate = date.toLocaleString(luxon.DateTime.DATETIME_FULL); // Output: "Wednesday, September 7, 2023, 12:00 PM"
The DATETIME_FULL
function will restructure the given date and time and output “Wednesday, September 7, 2023, at 12:00 PM”. Additionally, you can internationalization your date and time, allowing you to format dates based on different locales:
const date = luxon.DateTime.local(2023, 9, 7, 12, 0);
const formattedDate = date.setLocale('fr').toFormat('MMMM dd, yyyy hh:mm a'); // Output (in French locale): "septembre 07, 2023 12:00 PM"
The formatted date will be based in the French locale for the time of September 07, 2023, 12:00 PM.
Time intervals
You can use the’ Duration’ class to handle time intervals using Luxon. This class represents a specific duration of time and allows you to specify it in different units, such as milliseconds, seconds, minutes, hours, days, and more.
Here is an illustration of how you can create and manipulate time intervals using Luxon.
// Import Luxon
const { Duration } = require('luxon');
// Create a duration of 1 day and 3 hours
const interval = Duration.fromObject({ days: 1, hours: 3 });
// Add 30 minutes to the interval
const updatedInterval = interval.plus({ minutes: 30 });
// Get the total number of minutes in the interval
const totalMinutes = interval.as('minutes');
console.log(`Original Interval: ${interval.toISO()}`);
console.log(`Updated Interval: ${updatedInterval.toISO()}`);
console.log(`Total Minutes: ${totalMinutes}`);
In this example, we begin by importing the Duration
class from Luxon. After that, we create a duration that represents a time interval of 1 day and 3 hours. We then use the plus()
method to add 30 minutes to this interval. Lastly, we utilize the as()
method to obtain the total number of minutes in the interval.
Using the Intl API
To incorporate the Luxon library with the Intl API, you can combine them to format and display dates based on the user’s locale. Here is an illustration of how you can achieve this:
const { DateTime } = require('luxon');
const date = DateTime.fromISO('2023-09-09T12:34:56');
const formattedDate = date.toLocaleString(DateTime.DATETIME_FULL);
console.log(formattedDate);
In this example, we create a DateTime
object using Luxon’s fromISO
method, which parses the given ISO date string. Following that, we utilize the toLocaleString
method provided by Luxon, which internally utilizes the Intl
API.
We specify the DateTime.DATETIME_FULL
argument to determine the desired format. In this case, the date will be formatted as “weekday, month day, year - hour: minute: second AM/PM” (for example, “Saturday, September 9, 2023 - 12: 34: 56 PM”).
The toLocaleString
method utilizes the system’s default locale by default, but if you wish to override it, you can provide a specific locale as the second argument.
const formattedDate = date.toLocaleString(DateTime.DATETIME_FULL, { locale: 'en-US' });
This will format the date in the US.
Luxon calendar
Luxon offers the Calendar
class to support various calendar systems. By default, Luxon uses the Gregorian calendar. However, Luxon also provides support for alternative calendar systems like the Islamic, Hebrew, and Chinese calendars. Here is an example that demonstrates how to work with different calendar systems in Luxon.
const { DateTime, Settings } = require('luxon');
// Set the default calendar to Islamic
Settings.defaultZone = 'Africa/Cairo';
Settings.defaultLocale = 'ar'; // Set locale to Arabic for proper month names
// Create a DateTime using the Islamic calendar
const islamicDate = DateTime.fromObject({ year: 1445, month: 3, day: 25, calendar: 'islamic' });
// Format the date
const formattedIslamicDate = islamicDate.toLocaleString(DateTime.DATE_FULL);
console.log(formattedIslamicDate);
In this example, we begin by importing the required modules from Luxon. Following that, we establish the default zone as ‘Africa/Cairo’, which is frequently used in regions that follow the Islamic calendar. Additionally, we set the default locale to ‘ar’ for Arabic.
Subsequently, we generate a DateTime
object using the Islamic calendar system by specifying the islamic
option for the calendar.
Lastly, we utilize the toLocaleString
function with the DATE_FULL
option to format the date and obtain a comprehensive representation.
Time zones in Luxon
Luxon shines when it comes to time zones, it offers support for working with time zones through the luxon.Settings
object. This allows you to set the default time zone and customize how Luxon handles time zones in your application.
Here is an example that demonstrates how to work with time zones in Luxon:
const { DateTime, Settings } = require('luxon');
// Set the default time zone to 'America/New_York'
Settings.defaultZone = 'America/New_York';
// Create a DateTime in the specified time zone
const dateTime = DateTime.local(); // This creates a DateTime in the 'America/New_York' time zone
// Convert to a different time zone
const dateTimeInTokyo = dateTime.setZone('Asia/Tokyo');
// Format the dates
const formattedDateTime = dateTime.toISO(); // Outputs in 'America/New_York' time zone
const formattedDateTimeInTokyo = dateTimeInTokyo.toISO(); // Outputs in 'Asia/Tokyo' time zone
console.log(formattedDateTime);
console.log(formattedDateTimeInTokyo);
In this example, the process begins by importing the required modules from Luxon. Following that, the default time zone is set to ‘America/New_York’ using the Settings.defaultZone
property.
Next, a DateTime
object is created using DateTime.local()
. This generates a DateTime
object in the previously specified default time zone (‘America/New_York’).
Afterward, the setZone
method is utilized to convert the DateTime
object to a different time zone (in this case, ‘Asia/Tokyo’).
Finally, the dates are formatted using toISO
. The resulting output will include the date and time in their respective time zones.
Conclusion
In conclusion, Luxon is a comprehensive JavaScript library designed for efficient handling and manipulation of dates and times. With its user-friendly interface, compatibility with multiple calendars, and seamless integration with the Intl
API, Luxon proves to be an invaluable resource for precise date and time operations. Whether utilized in web applications or server-side programming, Luxon streamlines intricate tasks, making it an essential component in the toolkit of every JavaScript developer.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.