Directives In Vue
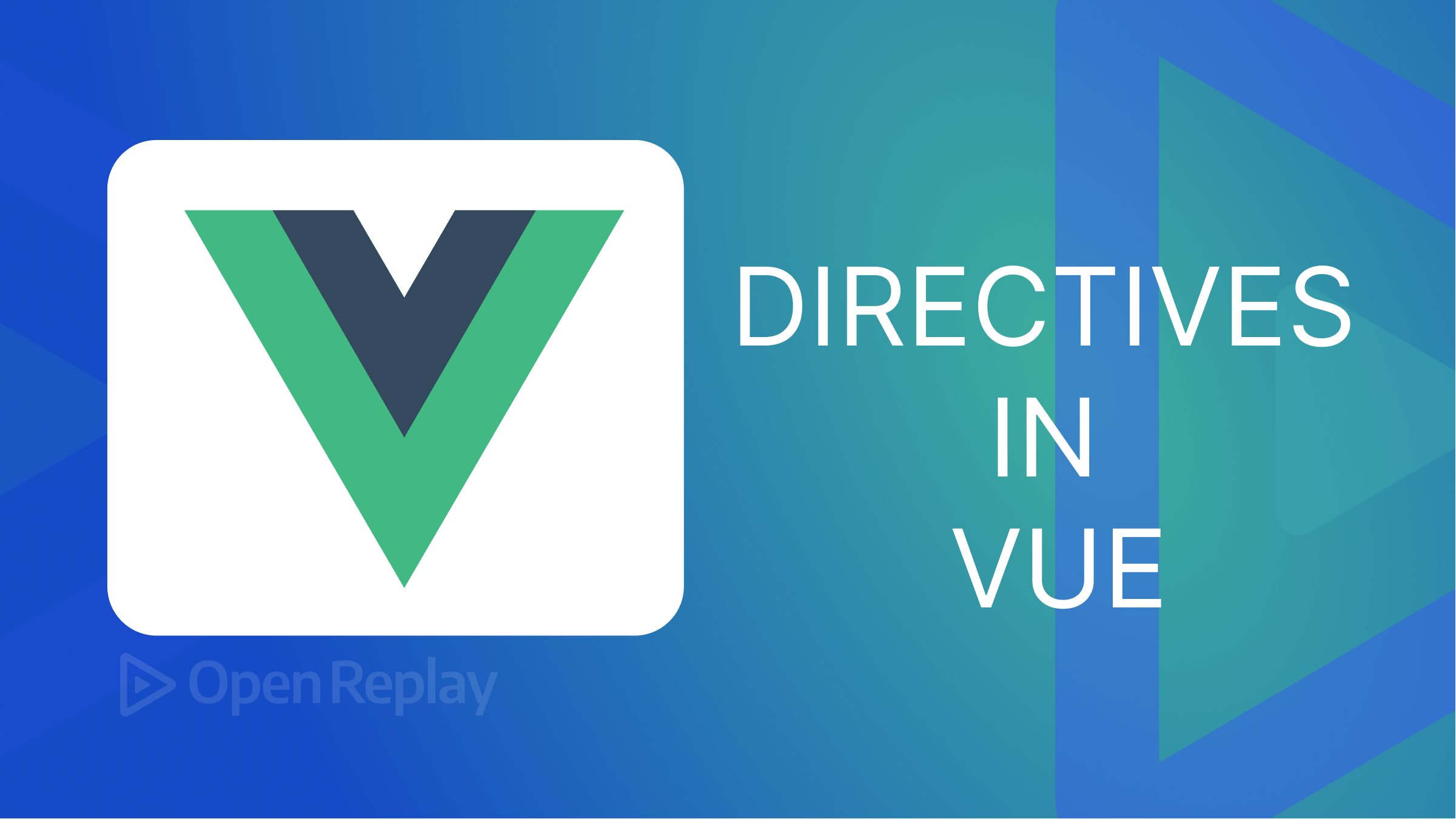
Vue.js is a Javascript framework for developing web user interfaces. In addition, it is used for mobile UI frameworks, Android and iOS app development, and desktop programming using the electron framework. The core library for Vue is simple to incorporate into other libraries and projects. I’ll review the built-in vue.js directives in this article and show you how to create custom directives.
What are the directives in vue.js?
Directives in Vue.js are special attributes added to HTML elements that allow you to bind data and control the behavior and appearance of an element. Directives are prefixed with the “V-” character, and this prefix tells Vue that the attribute is a directive and not just a regular HTML attribute. The purpose of directives is to apply dynamic behavior to the DOM, making it easier to manage and manipulate elements based on the application state.
To use a directive in Vue.js, you add it as an attribute to the HTML element you want to modify. The directive’s value should be a JavaScript expression that returns the value or action you want to bind to the element.
For example, the following code binds the value of the message
property to the text content of a p
element using the v-bind
directive:
<template>
<div>
<p v-bind:textContent="message"></p>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello, World!'
}
}
}
</script>
The following sections will explain the primary directives of Vue.
v-If
v-if is a vue.js directive that renders an element based on the truthiness of a javascript expression. This means you can display or hide elements in your HTML based on whether a condition is true or false. It prompts transition when its condition changes. For example:
<template>
<div>
<p v-if="showText">This text is shown when showText is true.</p>
</div>
</template>
<script>
export default {
data() {
return {
showText: true
}
}
}
</script>
In the example above, “This text is shown when showText is true.” is displayed only when the value of showText
is true. If the value of showText
is false, the text will not be displayed.
The v-if
directive can also be used in conjunction with the v-else
directive to provide an “else” branch to the conditional rendering. For example:
<template>
<div>
<h1 v-if="showTitle">Hello, OpenReplay!</h1>
<p v-else>The title is hidden.</p>
<button @click="showTitle = !showTitle">Toggle Title</button>
</div>
</template>
<script>
export default {
data() {
return {
showTitle: true
};
}
};
</script>
In this example, the p
element is only rendered if the showTitle
data property is false
.
It is important to note that the v-if
directive operates on the element it is applied to and all its children. If you want to render only a portion of the element’s content conditionally, you can use the v-if
directive on a template
element. For example:
<template>
<div>
<template v-if="showTitle">
<h1>Hello, OpenReplay!</h1>
<p>The title is visible.</p>
</template>
<template v-else>
<p>The title is hidden.</p>
</template>
<button @click="showTitle = !showTitle">Toggle Title</button>
</div>
</template>
<script>
export default {
data() {
return {
showTitle: true
};
}
};
</script>
In this example above, only the h1
and p
elements within the first template
element are conditionally rendered, not the entire parent div
element.
The v-if
directive is a powerful way to render elements in Vue.js conditionally. Whether you’re working with a single element or a portion of an element’s content, the v-if
directive allows you to show or hide elements based on a truthy or falsy value.
V-for
v-for is a built-in directive in Vue.js. The v-for directive allows you to loop through an array of items and render each in a template. Here’s an example of how you might use the v-for directive in Vue:
<template>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.text }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, text: 'apple' },
{ id: 2, text: 'banana' },
{ id: 3, text: 'cherry' }
]
}
}
}
</script>
In the example above, the v-for directive is used to loop through the items
array and render each item as a list item in an unordered list. The :key
attribute provides a unique identifier for each item, which is recommended for performance reasons.
The output will be:
- apple
- banana
- cherry
V-on
The v-on directive in Vue.js is used to attach event listeners to DOM elements. It allows you to bind JavaScript event handlers to DOM events like click, input, and more. Below is an example:
<template>
<div>
<button v-on:click="incrementCounter">Click me</button>
<p>You have clicked the button {{ counter }} times.</p>
</div>
</template>
<script>
export default {
data() {
return {
counter: 0
};
},
methods: {
incrementCounter() {
this.counter++;
}
}
};
</script>
In the above example, the v-on:click
directive listens to the click
event on the button element and triggers the incrementCounter
method when the button is clicked.
The v-on
directive can be written as an abbreviation using the @
symbol:
<button @click="incrementCounter">{{ counter }}</button>
You can also use v-on
to listen to multiple events by passing an object with multiple event listeners:
<template>
<input v-on="{ input: updateValue, focus: focusHandler, blur: blurHandler }" />
</template>
<script>
export default {
data() {
return {
value: ''
};
},
methods: {
updateValue(event) {
this.value = event.target.value;
},
focusHandler() {
console.log('Focus event');
},
blurHandler() {
console.log('Blur event');
}
}
};
</script>
In this example, the v-on
directive attaches multiple event listeners to an input element. The input
event listener is used to update the value
data property with the value of the input, while the focus
and blur
event listeners are used for logging messages to the console. Whether you’re working with a single event or multiple events, v-on
makes responding to user interactions in your Vue.js app easy.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
V-model
The v-model directive in Vue.js is used to bind the values of an input element to a data property in the Vue instance. The v-model directive creates a two-way data binding, meaning that changes made in the input element will automatically update the data property and vice versa. Here’s an example:
<template>
<div>
<input v-model="message">
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello OpenReplay!'
}
}
}
</script>
The “message” data property is bound to the value of the input element using the “v-model” directive. The “message” data property will be displayed in a paragraph below the input element. Whenever the user changes the input value, the change will be reflected in the “message” data property and displayed in the paragraph, and vice versa. V-model can also be used with other input elements, such as checkboxes and radio buttons, to bind a value to a data property:
<template>
<input type="checkbox" v-model="isChecked" />
<span v-if="isChecked">Checked</span>
<span v-else>Not checked</span>
</template>
<script>
export default {
data() {
return {
isChecked: false
};
}
};
</script>
In this example, the v-model
directive is used to bind the value of a checkbox to the isChecked
data property. When the checkbox is checked or unchecked, the isChecked
data property is updated, and the text displayed by the span
elements changes accordingly.
The v-model
directive is a convenient way to bind values to inputs in your Vue components, and it eliminates the need to manually update the input value and the data property every time one of them changes.
V-else
The v-else directive in Vue.js is used in conjunction with “v-if” and “v-else-if” directives to conditionally render elements based on the truthiness of an expression. The v-else directive specifies the template to render when the expression passed to the “v-if” directive is falsy. The “v-else” directive must be placed immediately after a “v-if” or “v-else-if” directive. It can show or hide elements, components, or other content within the DOM. Here’s an example:
<template>
<div>
<p v-if="showMessage">{{ message }}</p>
<p v-else>No message to display.</p>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: false,
message: 'Hello OpenReplay!'
}
}
}
</script>
In this example, the value of the showMessage
data property determines whether the message is displayed or not. If showMessage
is truthy, the message will be displayed; otherwise, the text “No message to display” will be displayed.
V-bind
The v-bind directive in Vue.js is used to bind values of an HTML attribute to a data property in the Vue instance. The v-bind directive creates a one-way data binding, meaning that changes made in the data property will automatically update the value of the HTML attribute, but changes made in the HTML attribute will not affect the data property. For example:
<template>
<div>
<img v-bind:src="imageSrc">
</div>
</template>
<script>
export default {
data() {
return {
imageSrc: 'https://picsum.photos/200'
}
}
}
</script>
The “imageSrc” data property is bound to the “src” attribute of an image element using the “v-bind” directive. Whenever the value of the “imageSrc” data property changes, the change will be reflected in the “src” attribute of the image element and update the image displayed.
V-once
The v-once directive in Vue.js indicates that a component should render its content only once, and that its content should not change even if the data changes. Here is an example:
<template>
<div v-once>{{ message }}</div>
</template>
<script>
export default {
data () {
return {
message: 'OpenReplay is awesome!'
}
}
}
</script>
In the example above, the message “OpenReplay is awesome!” will be displayed once and will not update even if the data changes. V-once is useful when displaying static content that does not need to change, such as a disclaimer or a copyright notice. It can also be helpful for performance optimization, as it prevents the DOM from re-rendering the content every time the data changes.
Creating custom directives
Custom directives in Vue.js allow you to extend the functionality of the template language. You need to use the directive
method on the Vue instance or a component to create a custom directive. Here is an example of how to create a custom directive in Vue.js:
// main.js
const app = new Vue({
el: '#app',
directives: {
custom: {
bind(el, binding, vnode) {
el.style.color = binding.value;
}
}
}
});
<!-- index.html -->
<div id="app">
<p v-custom="'red'">This text will be red.</p>
</div>
In the above example, the custom directive v-custom
changes the color of the text to red. The bind
function is called when the directive is bound to an element, and it receives the element, the binding object, and the Vue virtual node as arguments.
You can also create more complex custom directives with logic in JavaScript, for example:
// main.js
const app = new Vue({
el: '#app',
directives: {
custom: {
bind(el, binding, vnode) {
const fontSize = binding.value || 14;
el.style.fontSize = `${fontSize}px`;
}
}
}
});
<!-- index.html -->
<div id="app">
<p v-custom="18">This text will have a font size of 18px.</p>
<p v-custom>This text will have a font size of 14px.</p>
</div>
In this example, the custom directive v-custom
changes the font size of the text. If a value is passed to the directive, it sets the font size to that value. If no value is passed, it sets the font size to 14px.
Conclusion
Directives in Vue provide a powerful way to manipulate HTML elements and extend their functionality. Whether using built-in directives or creating custom ones, they are an essential part of the vue.js framework and are used in almost all vue.js applications. Custom directives can be used to extend the functionality of HTML elements.