All About Dynamic Routing in Single Page Applications (SPAs)
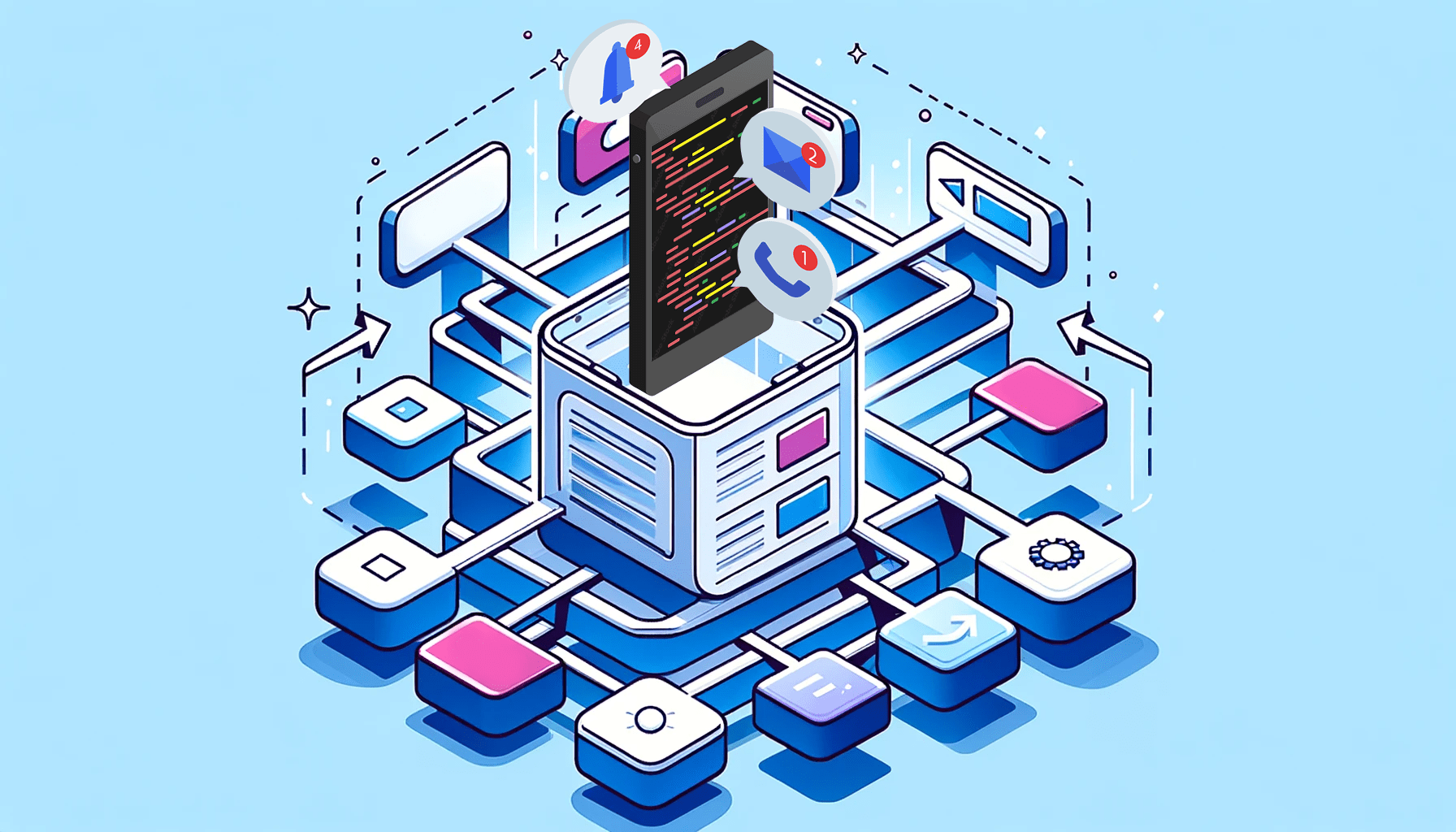
Single Page Applications (SPAs) are a type of web implementation that loads a single HTML page, which dynamically updates contents as a user interacts with a web app. Compared with multi-page applications, SPAs give users a seamless user experience by avoiding full-page reloads. To better enhance user navigation and overall performance in SPAs, it’s quite important to implement dynamic routing, and this article examines the importance of dynamic routing and its role in creating more responsive and engaging web experiences.
Discover how at OpenReplay.com.
Dynamic routing in SPAs involves the ability of a web app to update its content on a page without triggering a full reload. This is done through client-side routing, where all the navigation is done on the user’s device without having to fetch new HTML pages from the server. The basic components of dynamic routing include:
-
Component-Based Structure: Dynamic Routing is closely tied to the component-based structure of SPAs. Each route corresponds to a set of components that render the content related to that route. Components serve as the building blocks of SPAs, representing independent, self-contained functionality units.
-
Managing Browser History: This allows updating the URL displayed in the address bar without triggering a server request. The router manipulates the browser history by adding entries, allowing users to use forward and backward buttons to navigate.
-
Lazy Loading Components: Dynamic routing often implements lazy loading, which brings up only the required components for a specific route. It helps minimize page load times and optimize efficiency by reducing the amount of data transfer.
-
Route Mapping and Configuration: Dynamic Routing relies on a mapping between the web app’s route and corresponding components. A table or file can be used to configure the mapping, as this helps developers define how different URLs map to specific components of views.
-
Programmatic Navigation: Users can navigate different routes based on the web app’s logic and user interaction. This flexibility allows developers to create dynamic interfaces where content updates according to user responses/actions.
Dynamic routing relies on JavaScript to load and render the necessary components based on the requested route. It involves using a router to manage navigation and parsing URLs to determine the corresponding route within the web app. Without the need for page reloads, this results in quicker transitions between views, lower server load, and, therefore, a more responsive web app.
Implementation of Dynamic Routing
There are several steps to be followed and a few things to keep in mind when implementing dynamic routing in SPAs. Implementing dynamic routing starts with creating a SPA project, defining routes, handling security for routes, etc. In this section of the article, we will dive into the various steps to follow. Let’s dive in:
Setting up a Basic SPA Project
Here, you must set up the necessary project structure, including HTML, CSS, and JavaScript files. You’ll also use the tools and commands of a framework like React to initiate a basic SPA project.
The first thing you need to do is make sure you have installed development tools. Be sure that Node.js and npm are already installed for your project. You’ll also need to install the React app globally:
npm install -g create-react-app
Next, you will need to create a new project. You can create a new React project by running the code below:
npx create-react-app my-spa
After the above, you can navigate into your project directly:
cd my-spa
You can now explore the generated project structure with key folders like src
, public
, and node_modules
. To understand the key files, open the src
folder. index.js
is the entry point, and App.js
is the main component.
You can use the command npm start
to launch the development server. This command compiles your React codes, starts the server, and opens your SPA in a browser window.
Defining Routes and Route Components
Routes are essentially different pages or views. Each route aligns with a specific component that is responsible for displaying content related to that route. Developers make use of these routes in a way that reflects the structure of the web app.
To define routes, you must create a Routes
folder inside src
to help organize the route components. Next, create the necessary route components inside the “Routes” folder, e.g., Home.js
and About.js
. Here’s an example structure:
src/
Routes/
Home.js
About.js
After the above, you will import the necessary components and dependencies in App.js
:
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import Home from './Routes/Home';
import About from './Routes/About';
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<hr />
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
export default App;
You establish a structure and navigation flow in your SPA by effectively defining routes and their components. This process is what usually forms the foundation of a seamless user experience. It also sets the stage to further enhance your web app with dynamic routing features.
Handling Routes and Query Parameters
To efficiently make dynamic content updates, parameters should be included in routes. These parameters allow passing information to the route components, enabling personalized and context-specific content. Using query parameters also gives way to dynamic adjustments based on a user’s input.
So, how do you handle routes and query parameters? Firstly, you must define the route parameters using a colon followed by the parameter name. Example:
<Route path="/users/:id" component={UserProfile} />
To access the route parameters in your component, use the useParams
hook from react-router-dom
like below:
import { useParams } from 'react-router-dom';
function UserProfile() {
const { id } = useParams();
// Now, 'id' contains the value from the route parameter
// Use it in your component as needed
}
To access query parameters, use the useLocation
hook to access the present location of the object, including the search query. Example:
import { useLocation } from 'react-router-dom';
function ExampleComponent() {
const location = useLocation();
const searchParams = new URLSearchParams(location.search);
const paramValue = searchParams.get('paramName');
}
Alternatively, you can make use of withRouter
, which is a higher-order component (HOC), to inject the match
, location
, and history
props into your component:
import { withRouter } from 'react-router-dom';
function ExampleComponent({ location }) {
const searchParams = new URLSearchParams(location.search);
const paramValue = searchParams.get('paramName');
}
export default withRouter(ExampleComponent);
Properly handling routes and query parameters helps create a dynamic and more personalized user experience. It also helps to provide relevant data passage between different components. This enhances the flexibility and overall interactivity of your web app.
Redirecting and Navigating Between Routes
An important factor in dynamic routing is the ability to redirect users or navigate them between different routes. This helps to give users a smooth experience on a web app. You can use the several functions and components provided by a framework to control navigation and redirection based on application logic and user interaction.
Use the Link
component to create navigation links and the Redirect
component to redirect users based on several criteria. Here’s an example of how to use the Link
component:
import { Link } from 'react-router-dom';
function NavigationComponent() {
return (
<div>
<nav>
<ul>
<li>
<Link to="/home">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
</div>
);
}
To use the Redirect
component, you can follow the example below:
import { Redirect } from 'react-router-dom';
function LoginComponent({ isAuthenticated }) {
if (isAuthenticated) {
// Redirect to the dashboard if the user is authenticated
return <Redirect to="/dashboard" />;
}
return (
<div>
{/* Login form goes here */}
</div>
);
}
With the techniques mentioned above, navigating users within your SPA should be easy. Both Link
and Redirect
help create a smooth navigation experience, making interaction seamless for users.
Ensuring Security with Route Guards
Security is one aspect that is important in the development of SPAs. Route guards provide extra security by controlling access to certain routes. You can use these guards to check user authentication before giving them access to specific routes. This whole process helps protect sensitive information and restricts it to only verified users.
Here’s a simple example of a private route guard component:
import React from 'react';
import { Route, Redirect } from 'react-router-dom';
const PrivateRoute = ({ component: Component, isAuthenticated, ...rest }) => (
<Route
{...rest}
render={(props) =>
isAuthenticated ? (
<Component {...props} />
) : (
<Redirect to="/login" />
)
}
/>
);
export default PrivateRoute;
In the above example, the PrivateRoute
component takes the following props:
component
is the component to be rendered if the user is authenticated.IsAuthenticated
is a boolean that indicates whether the user is authenticated....rest
refers to additional props that should be passed to the underlying route.
From the code above, if a user gets authenticated, the provided component is rendered; otherwise, the user will be redirected to the login page.
Conclusion
In this article, while exploring dynamic routing in SPAs, we have delved into the basics, discussing client-side navigation, component-based structures, and even the lazy loading of components. All of which has given us a basic insight into the fundamental concepts. In addition to that, we have gone through setting up a basic SPA project, defining routes, handling parameters, and ensuring security.
The role of dynamic routing in the future of web development is significant. Its seamless integration with modern Javascript frameworks like React only seals its importance in the ever-changing world of web development. By harnessing its full potential, you can enhance the user experience as it facilitates personalization and responsiveness.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.