Exploring different approaches to styling Svelte applications
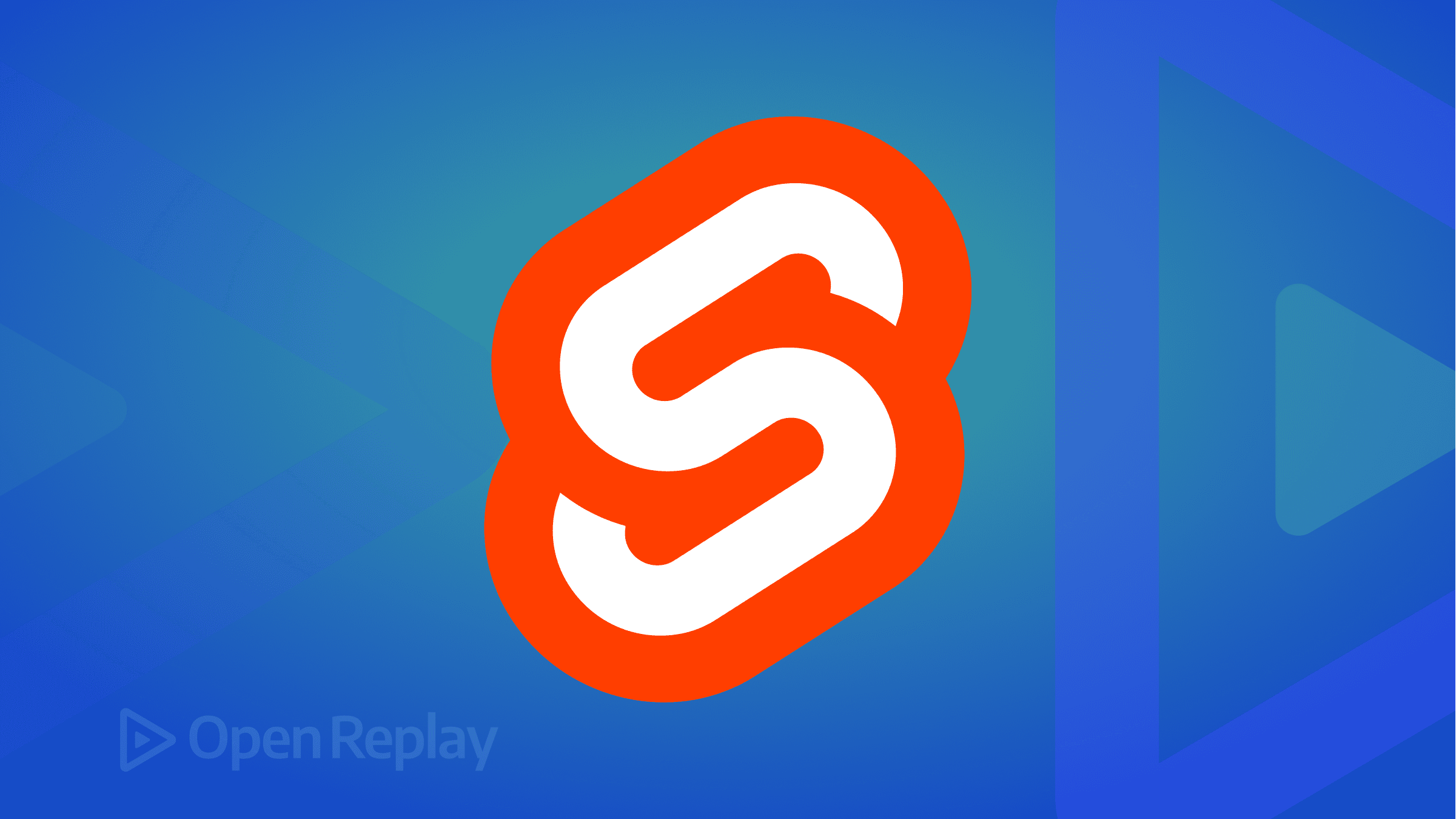
Svelte is a popular JavaScript framework for building web applications, known for its simplicity and performance. Svelte doesn’t have an opinionated of stying; it can be done in a variety of ways depending on the specific needs and preferences of the developer.
In this article, we will explore some of the different approaches that can be taken to style a Svelte application, including using inline styles, CSS files, and CSS-in-JS libraries. We will also look at the pros and cons of these approaches and compare them.
Inline Styles
One way to style a Svelte component is to use inline styles. Inline styles are styles that are defined directly in the HTML tag. Here is an example of how to use inline styles in a Svelte component:
// App.svelte
<p style='color: black'>An example of inline style in Svelte</p>
<p style='color: black; font-size: 3rem; font-family: sans-serif;'>Another example of inline styling</p>
Pros of using inline style:
- Simplicity and ease of use
- No additional setup or configuration is required
- Can be used in conjunction with other approaches
Cons of using inline styling:
- Limited in terms of design and flexibility
- Can become unwieldy with large and complex projects
Component-level Styles
Component-level styles are Svelte’s default approach to styling. As the name implies, your styles reside in your component (.svelte
file). It is as straightforward as adding your styles within the <style></style>
tags. Here is an example of how to add component-level styling in Svelte.
//xyz.svelte
<h1 class='heading'>This is a Svelte article</>
<p>An example of inline style in Svelte</p>
<style>
p{
color: black;
font-size: 1rem;
font-family: sans-serif;
}
.heading{
font-size: 1rem;
text-align: center;
}
</style>
Pros of using component-level styles
- Allows for more modular and reusable code
- Makes it easy to style specific components without affecting the rest of the application
- Can be used in conjunction with other approaches
Cons of using component-level styles
- It can be time-consuming to create and maintain styles for each component
- May result in larger file sizes for the application
Global styles
Another way to style a Svelte application is to use Global styles. With this approach, you create separate CSS files for your styles and import them into your components as needed. Here is an example of how to use Global in a Svelte application:
// app.svelte
<script>
import './styles.css';
</script>
<button> click me</button>
//app.css
button{
color: blue;
font-family: 'Comic Sans MS', cursive;
font-size: 2em;
}
The button in app.svelte will be styled according to what is in the style.css file.
Pros of using Global styles
- Allows you to reuse styles across multiple components
- No need to specify styles for each component
- Can help keep your component templates clean and easy to read
Cons of using Global style
- It can be difficult to override and customize styles for individual components
- Can lead to naming conflicts and specificity issues.
CSS-in-JS Libraries
Another option for styling a Svelte application is to use a CSS-in-JS library. CSS-in-JS libraries allow you to write your styles in JavaScript and handle the translation to CSS for you. CSS-in-JS libraries are ideal for larger-scale projects when you create single-page applications with multiple components.
They can be useful if you want to take advantage of the flexibility and modularity of JavaScript in your styles or if you want to avoid the potential maintenance issues of using CSS files. Several CSS-in-JS libraries are available for use with Svelte, including emotion
and linaria
. Here is an example of how to use emotion in a Svelte component:
- First, install
emotion
in the root directory of your application
$ npm i emotion
Create a style.js file and add the following styles and add the corresponding class to the Svelte file.
import { css } from '@emotion/css'
const color = 'purple'
export const wrapper = css`
display: flex;
flex-direction: column;
align-items: center;
`
export const title = css`
color: ${color};
`
export const paragraph = css`
font-size: 20px;
`
<script>
import { wrapper, title, paragraph } from './styles.js';
</script>
<section class={wrapper}>
<div class={title}>
<p class={paragraph}>
I am a boy
</p>
</div>
</section>
Pros of using a CSS-in-JS library
- Allows you to write your styles in a way that is more closely integrated with your component logic
- It also allows you to use features such as theming and dynamic styles more
- Makes it easy to use advanced features such as variables and functions
Cons of using CSS-in-JS library
- Requires additional setup and configuration
- Can be difficult to use with third-party libraries and components
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Comparison of styling approaches
Styling Approach | Pros | Cons |
---|---|---|
Inline | Simple and easy to use | Can become unwieldy with large and complex projects. Limited in terms of design and flexibility. |
Component-level | Allows for more modular and reusable code. It makes it easy to style specific components without affecting the rest of the application. | It can be time-consuming to create and maintain styles for each individual component. May result in larger file sizes for the application |
Global | Can be used to style the entire application consistently. No need to specify styles for each individual component. | It can be difficult to override and customize styles for individual components. This can lead to naming conflicts and specificity issues. |
CSS-in-JS | Allows for complete control over styles. It makes it easy to use advanced features such as variables and functions. | Requires additional setup and configuration. It can be difficult to use with third-party libraries and components. |
As you can see, each approach has its own set of pros and cons, and the best approach depends on the specific needs of your project. For example, if you need complete control over your styles and want to use advanced features such as variables and functions, then CSS-in-JS might be the best approach. On the other hand, if you just want a simple and easy-to-use approach, then component-level or inline styles might be the best choice.
Conclusion
We have looked at styling approaches in Svelte, code examples of how to use them, their pros and cons, and a table comparing the approaches. When you want to start a new project, just take a few minutes to consider factors like your application size, the specific needs of your project, and duration; then, you should be able to pick the right styling approach. Also, if you want to explore Svelte more, you should check out Sveltekit.