Exploring the new Array Methods from ECMAScript 2023
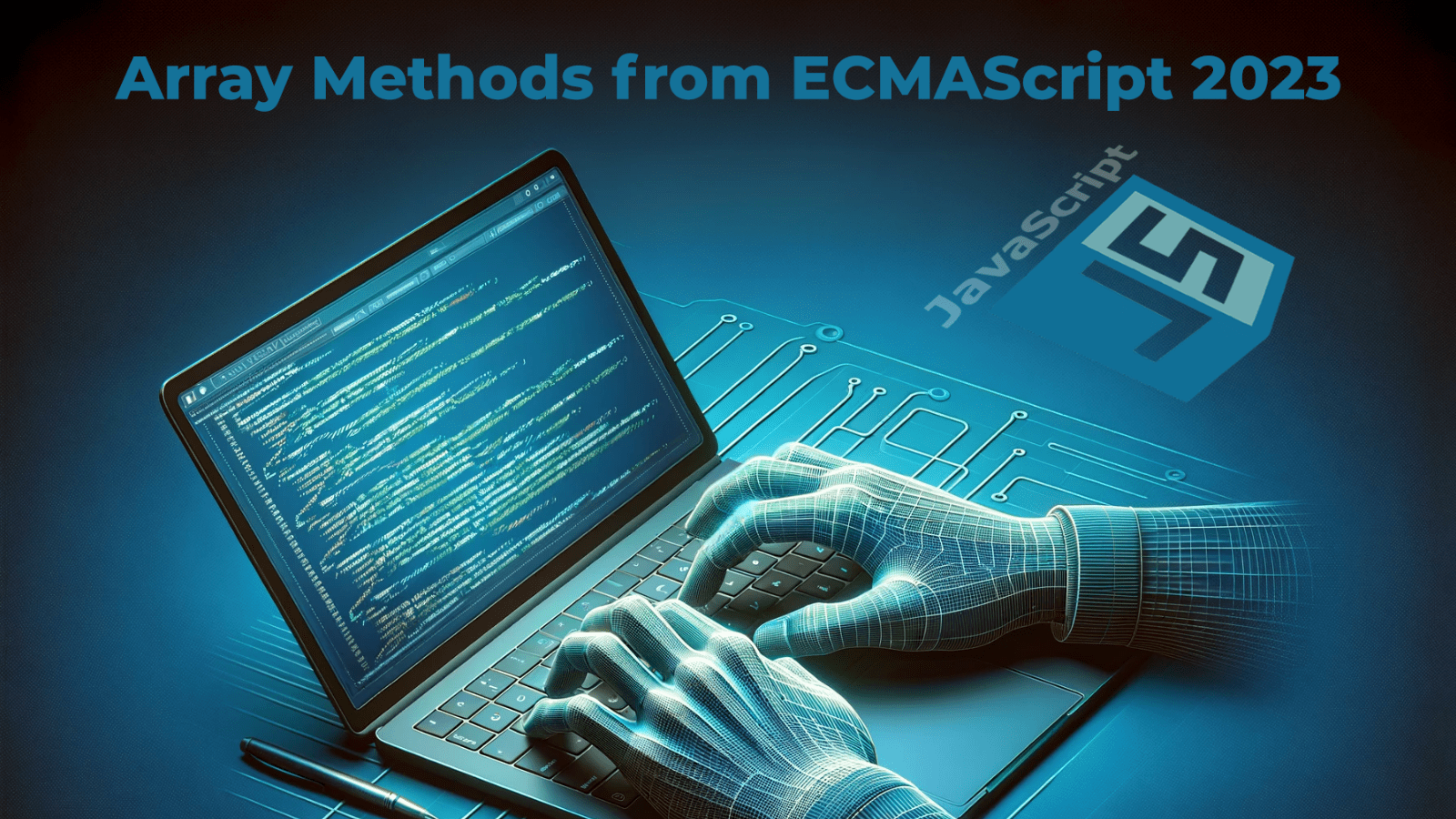
ECMAScript 2023 came in with new features to improve the language and make it more powerful and seamless. This new version comes with exciting features and new JavaScript array methods that make programming with JavaScript more enjoyable and easy. This article will thoroughly take readers through the functionalities of the new JavaScript methods on the array prototypes.
Discover how at OpenReplay.com.
JavaScript is constantly evolving, much like the web development industry altogether. ECMAScript 2023 is the latest version of JavaScript programming language. ECMAScript is in the fourteenth edition of the language specification and was released in June 2023. Over time, ECMAScript 2023 has developed into a general-purpose programming language. The web is run by it. It is therefore utilized for web apps and other programming activities that are linked.
ECMAScript is a standardized scripting language, and it is a specification for JavaScript. ECMAScript 2023 is an update of the JavaScript programming language to bring in improvements and make JavaScript programs predictable and maintainable. With this improvement, the Array
prototype has new methods. The JavaScripts array methods toSorted
, toReversed
, with
, findLast
, and findLastIndex
were introduced in ECMAScript 2023.
ECMAScript 2023 methods offer developers powerful tools to manipulate arrays without changing the original array. These methods operate on replicates of the array, thus allowing for safer and more predictable array manipulation. With these array methods, developers can embrace the immutability of the array data as they are sure that the original array remains unchanged. They will enhance the work of developers while working with arrays. It is advantageous to leave data unchanged, as demonstrated by these array methods from ECMAScript 2023. These benefits apply to any JavaScript objects, not just arrays.
Without further ado, let’s explore the new array methods in JavaScript.
The toReversed() method
The toReversed()
and the JavaScript reverse()
methods are comparable but not the same. The toReversed()
method returns the elements of an array in reverse order without changing the original array.
Note that the new JavaScript methods from ECMAScript 2023 code snippets run on the modern web browser. Check the browser capability from MDN. It works on the browser since the methods are still very new. Also, these methods are not supported by Node.js. They are supported by the Node.js version 20+. This does not favor developers as developers use the Node.js LTS version (Version 18).
Let’s look at the scenario where we have a list of the days of the week arranged in chronological order. The results will be displayed in reverse order.
//This is the original array
const days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday'];
//Using reverse()
const reverseDays=days.reverse();
console.log(reverseDays);
//Output: ['Friday', 'Thursday', 'Wednesday', 'Tuesday', 'Monday']
console.log(days);
//Output of original array is changed: ['Friday', 'Thursday', 'Wednesday', 'Tuesday', 'Monday'
The original array is modified with the reverse()
method.
The toReversed()
method reverses the array without changing the original array. Check out this example:
//Using toReversed() method
const reversedDays=days.toReversed();
console.log(reversedDays);
//Output: ['Friday', 'Thursday', 'Wednesday', 'Tuesday', 'Monday']
console.log(days);
//Output of original array is not changed: ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday']
The toReversed
function is a remarkable feature that developers appreciate. This is because the original array was mutated with the reverse
method. Thanks to ECMAScript 2023 for introducing the toReversed
method. With this method, you can change the copy of the array instead of the original array.
The toSorted() method
The toSorted()
is similar to the JavaScript sort()
method. The two methods differ from each other and, yes! You guessed it right. Unlike the sort()
, the toSorted()
method will not change the original array. This array method operates like the toReversed()
method. These methods return a new array, leaving the original arrays unchanged.
Consider this scenario where we need to sort numbers in the ascending order. This example will illustrate the difference between sort()
and toSorted()
.
//This is the original array
const numbers=[9, 4, 8, 1, 6, 3];
//Using sort
const sortNumbers=numbers.sort();
console.log(sortNumbers);
// Output:[1, 3, 4, 6, 8, 9]
console.log(numbers)//original array
//Output:[1, 3, 4, 6, 8, 9]
//Using toSorted
const sortNumbers=numbers.toSorted();
console.log(sortNumbers);
// Output:[1, 3, 4, 6, 8, 9]
console.log(numbers)//original array
//Output:[9, 4, 8, 1, 6, 3]
//Original array is not mutated
The toSorted()
array method modifies the copy version in the above instance. It returns a new array with the elements sorted in ascending order. However, the original array is not changed. On the other hand, the sort()
method mutates the original array in place, as seen in the above example.
The toSpliced() method
The toSpliced()
array method is a new feature in ECMAScript 2023. The toSpliced()
is similar to the JavaScript splice()
array method, but there is a slight difference. The difference between the two array methods is in toSpliced()
, the original array is not modified.
toSpliced()
creates a new array with changed elements, but the original array remains unchanged. toSpliced()
does multiple things in an array. You can add, remove, and replace elements in an array.
Let’s consider a scenario where we have a list of elements and want to remove some elements without altering the original array. This example will illustrate the difference between splice()
and toSpliced
.
//Original array
const fruits=['Grapes', 'Oranges', 'Bananas', 'Mangoes', 'Pineapples'];
//Using Splice
const spliceFruits= fruits.splice(2,1);//removing one fruit(Bananas)
console.log(spliceFruits);
//Output: ['Grapes', 'Oranges', 'Mangoes', 'Pineapples']
console.log(fruits);//original array is altered
//Output: ['Grapes', 'Oranges', 'Mangoes', 'Pineapples']
//Using toSpliced
const splicedFruits= fruits.toSpliced(4,1);//removing one fruit(Pineapples)
console.log(splicedFruits);
//Output: ['Grapes', 'Oranges', 'Bananas', 'Mangoes']
console.log(fruits);//original array remain unmodified
//Output: ['Grapes', 'Oranges', 'Bananas', 'Mangoes', 'Pineapples']
// Adding an element at index 1
const fruits2 = fruits.toSpliced(1, 0, "Passion");
console.log(fruits2);
//Output: ['Grapes', 'Passion', 'Oranges', 'Bananas', 'Mangoes', 'Pineapples']
// Replacing one element at index 1 with two new elements
const fruits3 = fruits2.toSpliced(1, 1, "Guava", "Melon");
console.log(fruits3);
//Output: ['Grapes', 'Guava', 'Melon', 'Oranges', 'Bananas', 'Mangoes', 'Pineapples']
//Original array remain unchanged
console.log(fruits)
//Output: ['Grapes', 'Oranges', 'Bananas', 'Mangoes', 'Pineapples']
The toSpliced
array method is an important additional feature of the JavaScript language. It allows developers to manipulate the arrays without altering the original arrays. This enables developers to manage and maintain the code easily. As seen in the examples above, this method offers a more convenient way to add, remove, or replace elements at any array index.
The with() method
The with()
array method was added to the JavaScript programming language when ECMAScript 2021 (ES12) was introduced. Updating elements inside an array is common in JavaScript. However, changing the array elements modifies the initial array.
The with()
array method introduced a new feature in ECMAScript 2023. The with()
method offers a safe way of updating elements in an array without changing the original array. This is achieved by returning a new array with the updated elements.
const flowers=['Lily', 'Daisy', 'Iris', 'Lotus', 'Allium'];
//old way of updating an array;
flowers [4]='Rose';
console.log(flowers);
//Output: ['Lily', 'Daisy', 'Iris', 'Lotus', 'Rose']
//New way of updating an array using with()
const updatedFlowers=flowers.with(4, 'Aster');
console.log(updatedFlowers);
//Output: ['Lily', 'Daisy', 'Iris', 'Lotus', 'Aster']
console.log(flowers);//original array
Output: ['Lily', 'Daisy', 'Iris', 'Lotus', 'Allium']
The old way of updating an array used the bracket notation to change an element within an array. Using the bracket notation to update an array, the original array is modified. However, the with()
method achieves the same result after updating an element in a specific array index but doesn’t mutate the original array. You can create a copy of the array, which returns a new array with the updated index.
Wrapping Up
ECMASctript keeps advancing as it gets a new version every year. This is a trend that has been consistent since 2015. This transformation has been taking place every year to improve ECMAScript and JavaScript. ECMAScript 2023 brings several exciting features to JavaScript language. These features improve the language capabilities and the developer experience.
To summarize, you learned the functionalities of the new JavaScript array methods from ECMAScript 2023. Go ahead and use the ECMAScript 2023 array methods:
toReversed()
toSorted()
toSpliced()
with()
ECMAScript ensures web developers build applications that are more illustrative using efficient code. Those are the new JavaScript array methods from ECMAScript 2023. Happy learning!
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.