Fingerprint Authentication for your Flutter Applications
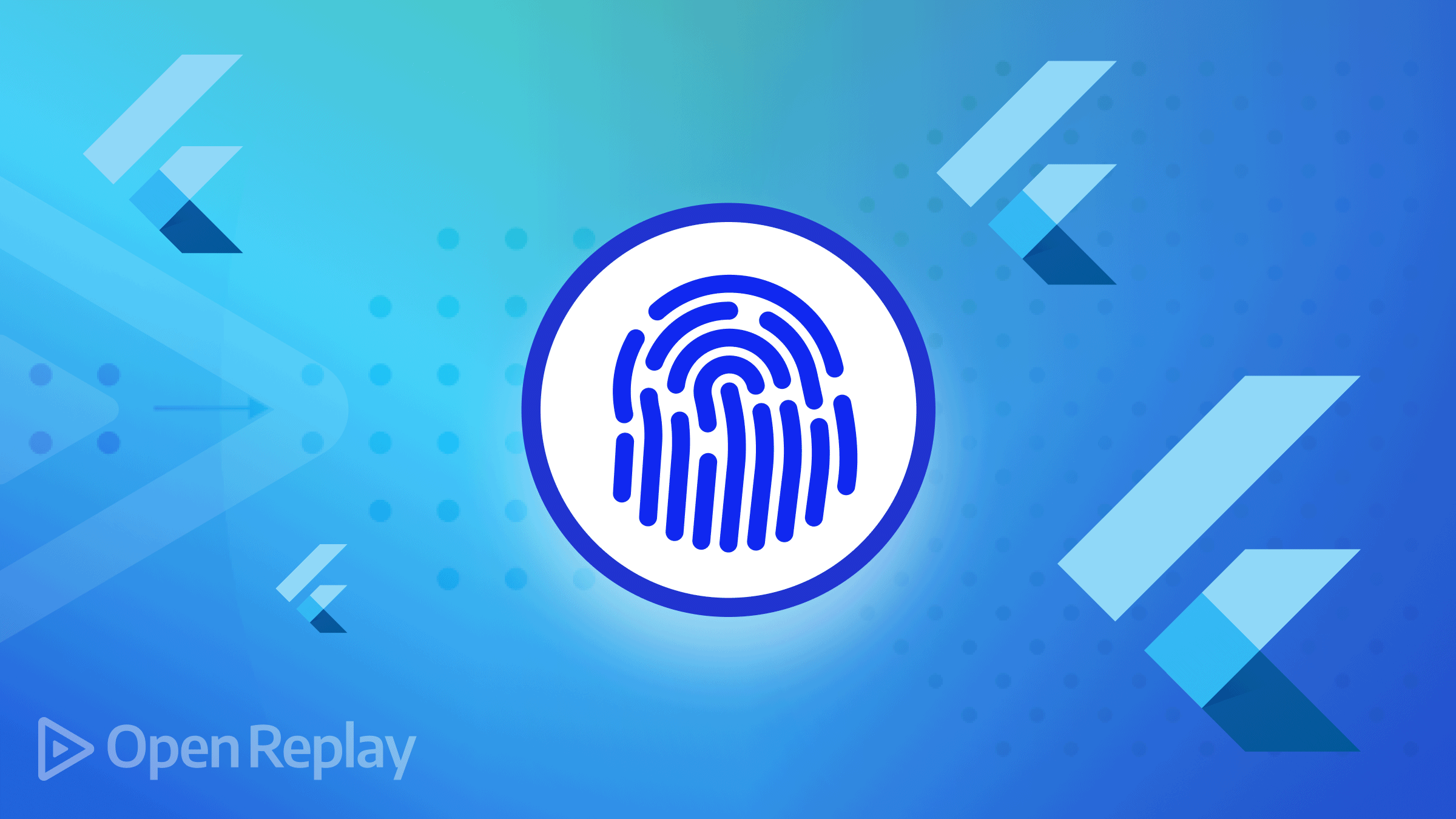
Fingerprint authentication has become an essential requirement in our mobile applications. It is the latest and the most reliable innovation in identity and access management. Fingerprint authentication is a form of biometric technology that enables users to access information or services using fingerprint images. It can be defined as verifying a user’s identity based on a stored fingerprint template. This form of authentication provides the user with a lot of advantages few of which are:
- It is much harder to fake than identity cards
- it cannot be guessed
- It is considered one of the most secure authentication methods.
Flutter provides us a package known as local_auth. This Flutter plugin provides means to perform local, on-device authentication of the user. In this article, users will learn how to implement this functionality in a flutter application.
Setting Up The Flutter Project
To follow up with this tutorial, click here to clone the starter files created for this project and run flutter create .
and then flutter pub_add local_auth
to add needed dependencies.
Open your pubspec.yaml
file, and you should see the local_auth
package added on line 16 below:
name: fingerprint_auth
description: A new Flutter project.
publish_to: 'none'
version: 1.0.0+1
environment:
sdk: ">=2.17.6 <3.0.0"
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.2
local_auth: ^2.1.2 #This line was added
dev_dependencies:
flutter_test:
sdk: flutter
flutter_lints: ^2.0.0
flutter:
uses-material-design: true
Next, go to your android > app > src > main
and open the AndroidManifest.xml
file add the code below:
<uses-permission android:name="android.permission.USE_BIOMETRIC"/>
The code above specifies a system permission the user must grant for the app to operate successfully.
Lastly, go to your android > app > src > main > kotlin
and open the MainActivity.kt
file and change it to the code below:
package com.example.fingerprint_auth
import io.flutter.embedding.android.FlutterFragmentActivity
class MainActivity: FlutterFragmentActivity() {
}
We changed it because local_auth
requires using a FragmentActivity
instead of an Activity
.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Adding Functionalities
With all the configuration complete, let us begin by creating our functionalities. First, let us import the local_auth
packages.
import 'package:local_auth/local_auth.dart';
Next, we will create an instance of our LocalAuthentication
class:
LocalAuthentication auth = LocalAuthentication();
With that done, we will create an asynchronous function to perform authentication when we click the button:
Future authenticate() async {
final bool isBiometricsAvailable = await auth.isDeviceSupported();
if (!isBiometricsAvailable) return false;
try {
return await auth.authenticate(
localizedReason: 'Scan Fingerprint To Enter Vault',
options: const AuthenticationOptions(
useErrorDialogs: true,
stickyAuth: true,
),
);
} on PlatformException {
return;
}
}
Let us break down the code:
- First, we check to see if the device can check biometrics or default to device credentials.
- Next, we authenticate the user with the biometrics available on the device by calling the
authenticate()
method. This method also allows the user to use device authentication such as pin, pattern, and passcode. - The
useErrorDialogs
parameter checks to see if the system will attempt to handle user-fixable issues encountered while authenticating. - The
stickyAuth
parameter is used when the application goes into the background for any reason while the authentication is in progress. While set to true, authentication resumes when the app is resumed.
Time to implement the onPressed
functionality:
onPressed: () async {
bool isAuthenticated = await authenticate();
if (isAuthenticated) {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) {
return const SecretPage();
},
),
);
} else {
Container();
}
},
We checked to see if the authentication returns to true
, then it should take us to the SecretPage
screen.
Testing the Application
Now let us test the application. On your terminal, run the code below:
flutter run
The code above will build and install the app on your device or emulator.
https://www.dropbox.com/s/q7xzpcvgdfyvi1h/VID_20220810_184757.3gp?dl=0
Conclusion & Resources
In this tutorial, we learned about how we can easily integrate fingerprint authentication in a Flutter application.
Here is a link to the Github Source Code.
A TIP FROM THE EDITOR: For other way of implementing authentication, please consider our Implementing Facebook Authentication for Flutter article.