Flutter Fortified: Mastering Updates and Security
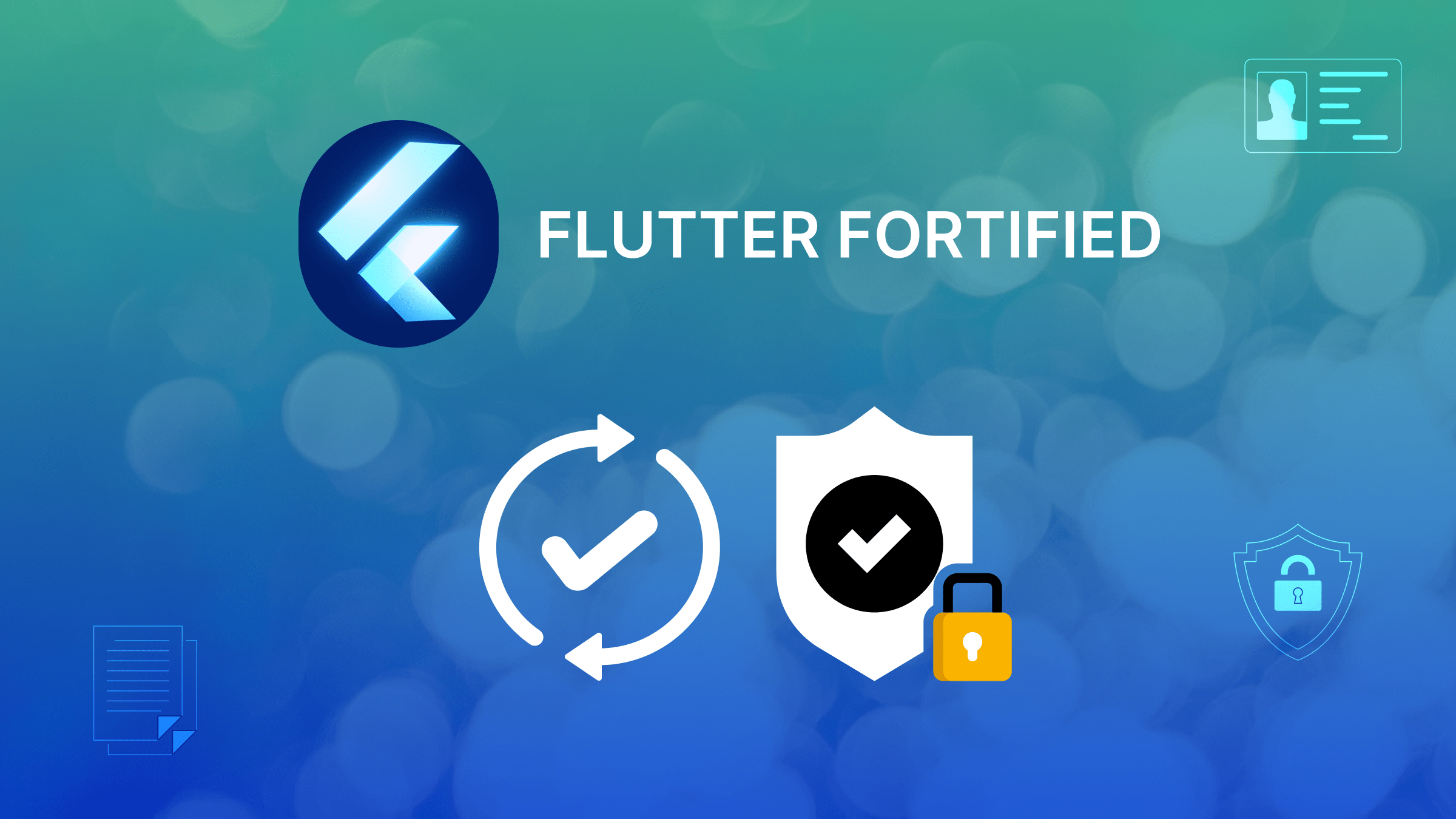
In this modern digital era, keeping users’ data safe and ensuring that apps are working as expected is essential. This article will show you how to handle security and updates in Flutter-written apps.
Spot abnormal user behaviors and iron out the bugs early with OpenReplay. Dive into session replays and reinforce your front-end against vulnerabilities that hackers search for.
Discover how at OpenReplay.com.
While the need for mobile applications is increasing, it is upon developers today to create applications that are not only rich in features but also strong and updated. That is where Flutter, which is Google’s open-source UI toolkit, comes into play as it gives full answers for you when developing cross-platform apps using the same codebase.
This article is geared towards securing and keeping Flutter applications current. Adherence to these procedures will ensure that one’s personal information is secure. Furthermore, it underscores the need for frequent software modifications to enhance effectiveness and eliminate any errors that might arise, as well as testing on various versions to avoid losing relevance with time. Developers can be sure of the reliability of their products if they follow these rules while also protecting them from being hacked because developers could hack into someone else’s creation, thereby causing their career harm if their application falls into unscrupulous hands.
Hashing vs. Encryption
Hashing is an important way to protect information and has different aspects compared to encryption, which is used to conceal plaintext. A Flutter application needs safeguarding—this section contains detailed information on how these compare.
Hashing
We use hashing to know that data has not been tampered with and to verify data. Simply put, hashing turns the data into a unique size fixed normally represented by a digest string of characters. Hash functions have no reverse functions. Once the data is hashed, one cannot convert it back to the initial form. They return a uniform length result irrespective of how long the input size may be to ensure that the hash output is consistent. It’s deterministic such that any given input will always generate similar hashes.
Hashing algorithms are meant to be speedy and effective, thus facilitating rapid calculations. A good hash function also reduces the chances of two different inputs producing similar hash outputs.
To securely preserve email passwords, save them to the database as hashes first. Then, make sure data has not been altered based on how different instances of this data compare with themselves in terms of their hashes on those instances. This is done by using digital signatures to confirm whether a given set of information has been interfered with or not.
Popular Algorithms include:
Encryption
Encryption protects data confidentiality by transforming data into an alternative format only authorized persons can decipher back to the original form. Encryption algorithms are functions that can work in both directions; therefore, encrypted data can be decrypted if the right key is available. Usually, the size of encrypted data differs from the source one. A decoder uses characters to decrypt this formatted information.
Encryption is used to keep things a secret; hence, when the data is intercepted, anyone cannot understand it without a certain key. Make sure you encrypt your data anytime you send it through a network so that other people can’t pick it up. As a preventive measure, protecting sensitive information on disks or any other storage devices from unauthorized access is essential. There must be secure messaging services and tamper-proof messaging mechanisms for secure communication.
Popular Algorithms include:
When do I use Hashing?
Passwords should be kept securely using employing hashing (salt included). Hash functions such as bcrypt or Argon2 were crafted particularly to hash passwords, hence providing extra security mechanisms such as key stretching. Hashing was also applied to confirm the integrity of the locally stored or fetched data from the server.
When do I use Encryption?
Encrypting sensitive data being sent over a network is fundamental. As an illustration, HTTPS (which is reliant on TLS encryption) guarantees data encryption while it’s being transmitted. Moreover, sensitive data saved in the gadget should be encrypted to keep it away from unauthorized individuals. Encrypting sensitive data with flutter_secure_storage helps users control it.
Verdict
Flutter applications’ security heavily relies on how different roles perform hashing and encryption. Hashing is used to guarantee the integrity of data and securely store passwords. Data must be encrypted to ensure that it is confidential when in transit or stored.
Adopting a comprehensive measure toward security would aid in safeguarding an application from a vast array of threats. For example, one can encrypt transmitted information and store secure passwords through hashing. It’s usually important to combine both methods.
Implementing Password Hashing in Flutter
To perform password hashing in the context of a Flutter app, a software developer can utilize a crypto package. The following code snippet shows us how we can encrypt our regular plain text passwords which is a critical aspect when working with user authentication systems in modern web applications.
The Process
The first thing you need to do is to add the crypto
package to your pubspec.yaml
file:
name: your_project_name
description: A new Flutter project.
publish_to: 'none' # Remove this line if you wish to publish to pub.dev
version: 1.0.0+1
environment:
sdk: ">=2.17.0 <3.0.0"
dependencies:
flutter:
sdk: flutter
crypto: ^3.0.1
dev_dependencies:
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
You must replace your_project_name
with what you have named your project. This file contains the required crypto
packager version ^3.0.1
and normal Flutter dependencies.
The following is what includes the hash and verification functions that use dart:convert and crypto packages in Dart:
import 'dart:convert';
import 'package:crypto/crypto.dart';
// Function to hash the password
String hashPassword(String password) {
var bytes = utf8.encode(password); // Convert password to bytes
var digest = sha256.convert(bytes); // Hash using SHA-256
return digest.toString(); // Convert the digest to a string
}
// Function to verify the password
bool verifyPassword(String enteredPassword, String storedHash) {
var enteredHash = hashPassword(enteredPassword); // Hash the entered password
return enteredHash == storedHash; // Compare the hashes
}
void main() {
// User registration: hashing and storing the password
String password = "password";
String hashedPassword = hashPassword(password);
print("Password successfully hashed and stored: $hashedPassword");
// User authentication: verifying the password
String enteredPassword = "password";
bool isPasswordCorrect = verifyPassword(enteredPassword, hashedPassword);
print("Password is correct: $isPasswordCorrect");
}
Explanation:
-
Import Packages:
dart:convert
is employed for encoding passwords into bytes.crypto
is used to hash passwords using SHA-256. -
Hash_Password
Function (hashPassword): Encodes password into bytes using UTF-8 encoding. Hash the bytes using SHA-256. Turn hash digest into a string and give it back. -
Verify_Password
Function (verifyPassword): The same method as that of the stored password is employed while hashing the entered password. Match the hash of the entered password with the hash of the stored password.
Output:
GIF: A user continues to enter a significant secret code in the specified box, clicks “Hash Password” to hash the code, and finally confirms to change the status from false to true.
Enhancing Performance and Features
By regularly updating it, you can improve the performance of your application and introduce upgrades that are meant to match what users look forward to. In this section, we will discuss varying ways of improving the performance and features of our Flutter applications.
Utilizing new Flutter features
Incorporating these features might enrich the user experience and keep the app competitive when Flutter adds new widgets or capabilities, like the introduction of SliverAppBar for flexible app bars.
An example of this is SliverAppBar,
which helps to create application bars that grow and shrink smoothly as the user scrolls, giving them a more fascinating experience. Employing this widget helps to make applications have a more modern and stylish design, offer an improved navigation system, and maximize screen space utilization.
What does it mean by “integrating fresh capabilities such as SliverAppBar
makes customers understand that the app is recent and maintained? Additionally, the feature shows the developer’s dedication to making the user experience better while at the same time being able to compete with others in the field.
Optimizing app performance
For Flutter to remain a carefully planned user expertise one should be in the operation of the app frequently checking and making it better accordingly whereby he can use PerformanceOverlay or Dart DevTools among other tools that provide information about frame rate and GPU render time. Developers can use the live feedback feature in PerformanceOverlay
where the metrics are displayed on top of the application, enabling them to spot any screen lagging within seconds.
Dart DevTools include many useful performance analysis tools that help developers analyze and optimize the performance of their applications in detail. An example of this is to avoid unnecessary rebuilds by using the const constructor for widgets that do not change hence making the app faster. It is important for developers to continually observe their applications for performance bottlenecks especially when they become more sophisticated, so they can still be fast and reliable.
Bug fixes and stability
Bug fixes are important for ensuring that your app made using Flutter remains stable and reliable since they prevent glitches, crashes, and user dissatisfaction. The process of constantly improving often enables you to fix any errors, improving how the end users feel while also increasing both the performance and reducing the chances of app rejection that might result in poor reviews sometimes. Several app aspects can be affected by bugs like app functionality and user interface, thus allowing developers to show dedication to quality and customer satisfaction by ensuring timely repairs.
Identifying bugs efficiently by monitoring and testing them continuously and then prioritizing the fixes based on severity and user impact is crucial. When team members collaborate and take advantage of user feedback, it is easier to resolve bugs. A proactive approach to fixing bugs guarantees an app with no disruptions, which earns trust from clients and leads to loyalty.
Identifying and fixing common bugs
It is very important to constantly monitor crash reports and user feedback so that any common issues can be identified and solved in your Flutter app. Developers who monitor crashes and user complaints can find out how often certain problems, like memory leakages, which cause apps to crash after running for long periods repeatedly, occur. These problems can then be corrected.
Showing developers’ resolutions of these issues helps reassure users that the app will function properly all the time. Dealing with them will also keep bad reviews at bay while minimizing user dissatisfaction. Additionally, developers need to implement solutions based on crash reports and feedback so that the app works well on different devices and conditions. This way, the app will succeed in the long run since it never disappoints its customers.
Stability Improvements
When updating your app, it would be best to put more emphasis on making it stable than anything else. This can be done by taking care of any user interface-related hickeys and other signs one may encounter while using this product. Take, for instance, making changes in how pictures load so that they do not eat into computer space; this could make all the difference regarding its overall robustness. Their purpose is to give individuals who use them a seamless experience without causing any harm to them through accidents because they are always running smoothly. This proves his reputation as a trustworthy application developer who strives hard not to let down their potential customers by giving false hopes when their dreams are about to come true.
To maintain a polished look, address such common issues as UI glitches; to avert user frustration and abandonment, prevent app freezes. Updating the software regularly is an active way of showing that you are maintaining and improving it, thus increasing its longevity and user satisfaction. Flutter applications require life extensions for sustained app stickiness and competitiveness by keeping them up-to-date such that they operate normally on all devices regardless of their conventional patterns for such performance.
Regression Testing
It is important to do regression testing before each update to ensure the forward or backward compatibility is okay. This means ensuring that the new changes or updates have not negatively affected any functionality already in place. With the flutter_test package by Flutter, there is flutter_driver also to enable automatic testing as well as UI testing which helps make things easier. The process of testing can be streamlined by using these tools. The automatic testing in flutter_test
is whereby testers can write different test cases checking if every individual function or widget would perform correctly by emphasizing the individual. With these tools, developers can detect early on potential problems, hence diminishing any chances of introducing errors on each update, thus maintaining the app’s consistency and trustworthiness for a good user experience.
Ensuring Compatibility with Android and iOS Updates
It’s so important to ensure that all versions of new operating system releases work correctly once you release them to maintain their performance levels while keeping the user happy. One must adapt to the changes in OS-specific aspects such as Android 13’s enhanced permission scheme and make necessary changes to your app to maintain its functionalities. For Flutter, ensuring constant updates on time about platforms ensures everything remains efficient and functions well. To maintain compatibility and optimal performance, one must keep up with platform-specific updates in Flutter. This means one may have to update dependencies, change the code to fit new APIs, and test the app thoroughly on the updated platform. Guidelines as well as tools to aid in maintaining the app’s compatibility are available through resources such as the Android Developer Console or Apple Developer Portal.
Updating Dependencies
Updating dependencies on Flutter regularly allows you to be compatible with the most recent SDK from Flutter as well as other external libraries (3rd party libraries). This strategy lets you exploit the latest packages’ features, performance boosts, and security improvements. For example, keeping the Firebase packages up to date introduces new ways of doing things to programmers and protects user data better, enhancing applications’ overall performance and dependability.
When you keep up with dependencies, you avoid compatibility troubles due to changes in the Flutter framework or other packages. The Flutter framework is always changing, so one needs continuous updating to avoid having problems with older codes that have become redundant and have discontinuing modifications being done. This way, an author can show that they care about their product and meet the standards set by their peers without making decisions based on personal preference alone. The app appears well-supported when such measures are taken in its development process, portraying the creators’ desire to maintain a high-quality product with features conform to market requirements.
Tools such as flutter_pub_outdated can be used to identify outdated packages and, later on, upgrade them with the help of ‘flutter pub upgrade’. There should be automated testing to guard against issues that come as a result of the updates while at the same time ensuring that no regression is introduced and that there are any unexpected behaviors. By keeping up with all these processes, the company maintains its competitiveness against others within its realm of operation while still keeping its app up-to-date and stable.
Handling deprecated APIs
It is important to replace deprecated APIs with recommended ones within Flutter so as not only to keep compatibility but also to have a future-proof app. For example, using the http package for networking tasks instead of the outdated HttpClient is necessary. With the evolution of Flutter, there is a likelihood that some problematic APIs will be dropped, thus leading to instability in an application.
Using modern replacements like the’ HTTP’ package benefits developers with better performance, error handling, and a simplified API. Switching smoothly with the help of Flutter’s official documentation and migration guides would mean developers have stable applications that benefit from the newest functions and enhancements. Such a move increases both stability and efficiency, leading to progressive reliability.
Stay informed about SDK releases
To keep app stability and compatibility up-to-date, keeping track of Flutter SDK releases and their implications is important. From time to time Flutter introduces new features, performance enhancements, and sometimes even breaking changes. This will inform developers about what new things they may be able to use as well as how well performance can be optimized quickly with some bug fixes that appear, among other things. When developers read release notes and documentation, they can understand changes, APIs that are not supported anymore, and recommended migrations. This will help them to strategize for their upgrade and sync development roadmaps. As a result, developers are expected to follow up on Flutter’s official channels and participate in its community activities to remain relevant and gain experience from others in the field.
Monitoring Flutter Release Notes
It is very important for app security if often people check Flutter release notes for security patches and updates. Security tokens help in addressing vulnerabilities like XSS vulnerabilities in plugins, for example webview_flutter, among many other things. When developers are more informed, they can be able to set up resources for testing new features better than before, which is good because then they can know what they should do first, especially when it comes time to test these fresh capabilities. That also helps us show our commitment to data safety concerning the customers’ information, and the clients themselves do trust us. To get detailed information about security patches and recommended actions, subscribe to official Flutter channels like the blog or GitHub repository. This active strategy will help to ensure safety and protect apps from potential threats.
Understanding security patches
Regarding the safety loopholes that Flutter and its plugins are facing to protect user data, maintaining the safety of any app requires that one is frequently updated on it. Thus, if we were to consider an instance whereby the shared_preferences plugin had undergone a flaw that made it possible for confidential information to be leaked, then there was no alternative but for programmers using this service to be forced to quickly switch to fixed versions. In addition, any potential risk may be managed through regular monitoring of insecurities notifications as well as being a member of such things as Flutter’s forums, among others, which will make it easier so long those who participate avoid any kind or form of threats. By incorporating some security protocols like encrypting data and secure authentication, we boost security levels in our app development, debugging, or deployment of Flutters, which should ensure that security flaws are exposed early and tackled in time for seamless operations and secure data privacy.
Automated Testing
It’s very important to do automated testing using the flutter_test mechanisms within Flutter to validate the app’s behavior and secure it. For instance, if we take unit tests, they test whether user data is cleaned up well before any SQL code execution that could lead to an injection attack on servers when proper security measures are in place.
Depending on constraints set, including these factors in the controls available can be input validation, authentication methods, or data encryption. The vast areas of input validation, authentication mechanisms, and data encryption form the basis of the automated tests. Integration tests, on the other hand, are simulated user experiences that check the authentication process and protect the data. Furthermore, the functionality of the security-related UI element is also tested by widget and UI tests. As these tests are included in the automated suite, developers can adequately uphold app security and integrity.
Manual testing for edge cases
It is important to not overlook the conducting of hand-testing in Flutter when it comes to pinpointing security flaws that automated tests can ignore. This is where we go through apps one by one just to make sure they are functioning right while also addressing their security aspects, like preventing any kind of injection attack by trying to put a number within a username field.
Another part about the manual test is that it has some special purposes too; discovering other interesting usage cases that were missed in automatic testing so far and showing some weaknesses plus strange ways of processing data in the software. It also evaluates how simple it is to do different tasks with it, its accessibility, and overall user experience by running over usability problems and layout errors.
Additionally, it assesses the user interface, accessibility, and overall user experience of the application by identifying usability issues and layout errors. It also evaluates how easy it is to achieve various functionalities using the system, along with how accessible it is and the general user experience by examining usability challenges as well as design mistakes. In security-centered manual testing, it is important to verify that the data encryption and secure network transmission have been done correctly.
During security-focused manual tests, verifying the correct execution of data encryption as well as secure networking becomes very crucial. It helps to investigate compatibility and achieve uniformity by trying various gadgets and platforms. To establish compatibility and consistency, we can check if it works well on different devices through the usage of assorted gadgets and platforms.
Security-focused Testing
Conducting security tests that are focused on critical findings of vulnerabilities in Flutter applications. Rooting out of insecure data storage patterns by security audits while penetration tests interfere with actual life assaulters, revealing weaknesses such as SQL injection or XSS. Regarding coding language alteration, security flaws can be detected within the changes made to the software, for example, through input validation and encryption.
Making use of security testing tools and libraries, such as owasp and dart-secure, improves the effectiveness of security testing. To maintain app security, it is very important to keep these in consideration when the Flutter team and security experts give them disseminate the information. If there is regular security-focused testing, it helps to ensure that varieties of matters that might pose security threats to Flutter apps are well handled and this handles.
Secure data handling
It is important that data records in apps made by Flutter are correctly managed and tightly secured to prevent multiple data breaches and unauthorized entries. The process involves setting up protective data storage measures that guard against confidential data exposure, such as user account details, to encryption technologies. Make sure that when data is transmitted across networks, it is secure by today’s standards: use HTTPS protocols together with SSL/TLS encryption techniques for this matter. Moreover, strong authentication mechanisms such as OAuth 2.0 and Firebase Authentication have to be implemented for any harmful feature and information protection purposes. Such access limitations can be achieved by combining these two advanced authentication forms alongside Role-Based Access Control, among others. Mitigating unnecessary data handling decreases the chances of experiencing data breaches by limiting the amount of what all users provide through reduction techniques, including, among others, minimalism principles and pseudonym creation, which is achieved through this means.
Automated Testing
The quality of your Flutter app depends on automated tests, which are important in detecting problems early in the development process. This section will discuss various techniques for carrying out automatic tests on our Flutter apps.
Unit Tests, Integration Tests, and UI Tests
Flutter_test and flutter_driver are aides that automate examinations which enable rapid test implementation and seamless integration into CI/CD workflows to achieve uninterrupted tests as well as rapid application deployment. A synergy of these verification techniques is an assurance that those mobile applications made using Flutter output are of excellent quality and that consumer satisfaction is achieved.
By adding the above-mentioned tests, developers can get all-inclusive test coverage across different strata of the application. These tests help in detecting defects and regressions earlier within the development process, hence enhancing best coding practices and the application’s dependability.
Test Coverage Analysis
It is important to monitor test coverage in Flutter to ensure that all critical elements of the application are adequately tested. Generating test coverage reports and finding areas for further testing can be done using tools such as pcov. If you look at these reports, you will understand those sections with less coverage than others, which will help you concentrate on them during your testing process. Consequently, it guarantees that the most significant features and crucial parts of the code are well-examined, avoiding hidden errors or bugs.
Routine Monitoring
Identifying performance problems and other issues, like security vulnerabilities, is critical for Flutter applications to function effectively. Without monitoring, we would not know what is going wrong with the app until it has gone so far that we cannot fix it anymore. This explanatory section explains why we need to keep our eyes open through regular checks.
Monitoring App Performance
Developers can track app performance metrics like response time and CPU usage using performance monitoring tools such as Firebase Performance Monitoring by Google. This allows them to keep an eye on the performance of their applications at all times when they have integrated these tools and, therefore, discern what is holding them back in terms of performance to improve upon this.
Crash Reporting
Integrate tools similar to Firebase Crashlytics for improved diagnosis of application failures by collecting logs from such crashes. The moment applications crash, these tools send immediate notifications about this. This enables the app makers to handle these app failures urgently. Consequently, they can boost their app performance by eliminating these crashes, hence enhancing their clients’ user experience altogether. Automating some of these processes, such as gathering data concerning crashes, will automatically make it much easier for you to know what occurred.
Logging and Analytics
Use logging tools and analytics tools such as Firebase analytics for monitoring user activities and app usage trends. Firebase analytics provides data on how users interact with applications and where they are used most. This allows developers to know the behavior and preferences of users so they can make changes in their apps with the help of data. Logging refers to events and activities that users undertake while using an application. The analytics tools analyze the collected data to produce reports and visualize app usage trends, making it possible to make decisions based on facts. Monitoring analytics data regularly enables software engineers to detect parts of the app that must be optimized and prioritize developing features. By following the end user actions, software engineers can improve customer satisfaction through increased app engagement.
Rollback Plan for Failures
It is crucial to have a plan to roll back if the application fails so you can return to an earlier working version immediately. This plan indicates how one can reverse any alterations made to the system and bring back the app to its proper operation mode. Moreover, it contains ways of recognizing such situations that demand going back and those who should be in charge since it will serve as a guideline during execution. Automated rollback protocols simplify this operation, reducing the time wasted trying to solve the problem. Keeping in touch with the people concerned will help guarantee that everybody understands what needs to happen in the system failure recovery process.
Rollback Procedures
It is vital to have rollback procedures that can allow going back to an unstable version of an application swiftly when such instances occur. Having defined what entails a rollback, software engineers get direction on how to recover from such instances and reduce time when the system is not fully operational. Automating this process using scripts ensures timely response to major system errors, reducing cases where manual intervention may lead to increased numbers of errors creeping in unintendedly. These procedures guide as regards at what point is rolling back necessary or who should do it.
Communication strategies for users
To keep Flutter apps transparent and reliable, effective communication with users on rollbacks and potential impacts is important. Notifications on the app and social media platforms draw users’ attention to problems and their resolution steps, thus making sure that they are cognizant of what is going on concerning each case. Instead of waiting until they happen again, notifying them beforehand helps minimize their annoyance or irritation whenever rollbacks occur.
Regular data backups
Backing up data regularly is critical for preventing data loss and ensuring business continuity in Flutter applications. They are like a safety net that allows saving time when emergencies happen by getting back data instantly instead of waiting for long hours or days during which work could be interrupted temporarily, thus causing delays. The significance of backing up is reiterated in the following ways: through holding useful information, like user-generated content as well as database data, which are not supposed to disappear completely; however, the updates have to be quick. For it to happen time after time, one must decide that he/she will not give up on data secrecy, thus managing to show his/her clients that they can rely on him/her at any time without worrying about losing their information since all these measures help safeguard against loss of information along with maintenance of secure networks.
Cloud-based Backup Solutions
Protecting data is critical to ensure that the data is protected and available. With cloud storage options like Firebase Cloud Firestore and Google Cloud Storage, app data backups are automated. This course of action ensures the safety of all information, which can be retrieved whenever required. For example, Firebase Storage allows automatic backup of user-generated content, including images or documents, at a go. This way, reliable, scalable app data storage solutions are provided. For complete archiving, data must be saved in the cloud at all times. Therefore, there is less likelihood of the overall loss of valuable information because records will always be available over the internet cloud. A backup routine should be run regularly so that it does not become overwhelming.
Local Data Backup
Create local backup systems to store app data on the phone or another source for better safety and access. It’s possible to go ahead and make your local SQLite database backup, too, by just taking advantage of Flutter’s path_provider package. It has been designed in such a way that one can use it to create device storage locations as well. The purpose of this package is to help developers back up SQLite databases since it provides this option in these places. Local backups guarantee that app information will always be reachable even when there is no online connection or external assistance.
Scheduled backup jobs
Accomplish automatic backup work by continuously planning them with the use of Flutter’s flutter_local_notification package, which makes it possible for one to have daily copies saved for different categories of details displayed on an application needed also informing users concerning the same hence transparency is a must. Because these copies are scheduled for specific times, they will not require any human interruption, which means that their dependability is ensured throughout. There is no need then again. Schedule copies are done automatically with no manual input needed; hence, stability and reliability are always assured. Backup tends to also make sure it mitigates against all types of unexpected losses. Moreover, one will receive messages that assist in giving comfort regarding the security level of his lance; the same clarifies things that are happening in progress.
Disaster Recovery Planning
For Flutter apps to be able to recover from devastating incidents, they must have a disaster recovery plan. This plan considers ways to lessen risk, handle emergencies, and resume service provision. It also has options for saving data, creating copies, and other special devices for automatic transfer if the current fails. The effectiveness of the plan is regularly checked through testing. This involves putting in place communication strategies that would help in getting in touch with concerned parties when there is an emergency. The key idea in the plan is assessing how bad it is and then deciding what needs to come first when recovering after such incidents.
Backup Restoration
Data protection in a Flutter can be achieved by implementing methods of data recovery in instances of data loss or corruption. An example is scripting for the restoration of Firebase Firestore backups when accidentally deleted. It enables rapid recovery from incidents where information was lost and minimizes interruptions in service provision by the program. With such measures in place, Flutter applications remain dependable by securing essential data.
Failover Systems
Ensuring uninterrupted service when servers are down is important in Flutter. What this means is that, to automatically change to another server in case one fails, such failover systems as load balancers or redundant server configurations must therefore be set up. This will make sure that should anything happen to servers, then all users can still access the app because failover systems have been put in place by the developers. They enhance the reliability of this software application while reducing its unavailability periods.
Data Replication
Of course, since we do not want to lose any data we replicate it in several data centers or geographic regions like this: The same information can be copied to various locations around the globe using a multi-region replication service provided by Firebase Realtime Database.
Version Control
It is important to use version control systems such as git to facilitate the management of amendments in the code foundation of the app. We utilize this method by monitoring for modifications, collaborating well, and maintaining various editions of our application. Tagging stable releases through git enhances identifiable versions that can be rolled back whenever faults come up. Henceforward, there shall be a uniform track in which the changes can be identified for those developers who have felt like moving backward in an earlier HTML era.
Rollback Procedures
There is a need in Flutter for having a backup plan as far as rolling back things is concerned as they may sometimes just not work out due to one thing or another or even because nobody knows how well they might turn out eventually when left alone entirely so they must involve the development of programs that deploy earlier versions of programs should something go wrong at any point during their execution which should then make it possible go back whenever things get too messy thus preventing further problems from occurring just like what usually happens when we encounter an error message saying that some kind bugs cannot be found at all or something similar but not really. In other words employing rollback plans, developers can have a clear idea about how they can always get back to safe grounds, which encompass writing certain algorithms that will make sure our software does not stop on its tracks every time you are near crashing or certain conditions are achieved Therefore in doing this you come up with code so that the systems installed respond quickly when there occurs a catastrophe lowering loss of income.
Communication strategies for users
It is extremely important to inform users in Flutter about rollbacks and potential repercussions on their experience in advance. This requires pushing out notifications through different channels, including an application itself and social networks, showing subscribers what has happened and how it has been resolved. For that reason, the developers can continuously update customers about any interruptions or modifications to their operations. This helps developers retain openness whilst nurturing reliance among consumers, leading them to appreciate the conditions in which people live and act.
Conclusion
In this guide, we have looked at the necessary aspects of upgrading and shielding Flutter applications which are critical for their function, performance as well and integrity. Complying with good practices while at the same time incorporating strong security measures helps developers to produce Flutter apps that are dependable and safe from unauthorized access where client information is concerned and ensures trustworthiness between end users.
Secure Your Front-End: Detect, Fix, and Fortify
Spot abnormal user behaviors and iron out the bugs early with OpenReplay. Dive into session replays and reinforce your front-end against vulnerabilities that hackers search for.