Undoing Git Commits After Push: Safely Revert Changes on Remote Repositories
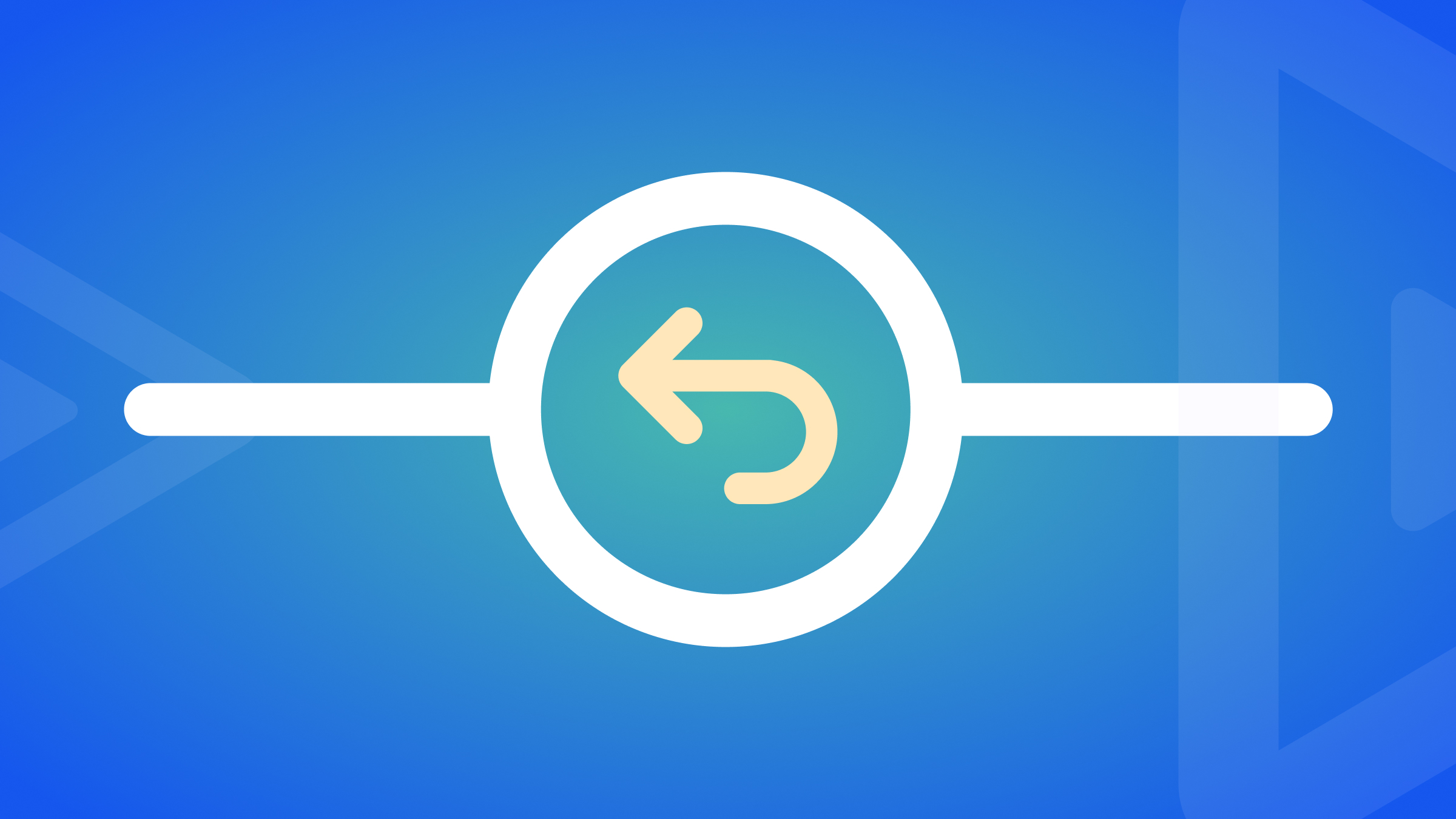
As developers, we’ve all been there - you make a commit, push it to a remote repository, and then realize you need to undo those changes. Don’t worry, Git provides powerful tools to help you safely revert commits, even after they’ve been pushed. In this article, we’ll explore various scenarios and techniques to undo Git commits and recover from mistakes, ensuring the integrity of your project’s history.
Key takeaways
- Use
git reset
to undo local commits before pushing. - Use
git revert
to safely undo pushed commits by creating a new commit that inverts the changes. - Avoid force pushing to remote repositories, as it can cause issues for collaborators.
- Use
git reflog
to recover lost commits after a hard reset.
Understanding Git commits
Before we dive into undoing commits, let’s quickly review how commits work in Git:
- A commit represents a snapshot of your repository at a specific point in time.
- Each commit has a unique identifier (SHA-1 hash) and a reference to its parent commit(s).
- The
HEAD
pointer refers to the current commit you’re on in your local repository.
Undoing the last local commit
If you haven’t pushed your last commit yet and want to undo it, you have a few options:
Using git reset
git reset --soft HEAD~1
: This command moves theHEAD
pointer back one commit, keeping the changes from the last commit staged.git reset --mixed HEAD~1
(orgit reset HEAD~1
): This is the default behavior ofgit reset
. It moves theHEAD
pointer back one commit and unstages the changes but keeps them in your working directory.git reset --hard HEAD~1
: This command discards all changes from the last commit, effectively removing it from your repository. Use this with caution, as it permanently deletes the changes.
Using git commit --amend
If you want to modify the last commit without changing the commit history, you can use git commit --amend
. This allows you to update the commit message or add/remove changes to the last commit.
# Make necessary changes
git add .
git commit --amend
Undoing commits that have been pushed
When you need to undo commits that have already been pushed to a remote repository, you should use git revert
to create a new commit that undoes the changes.
Using git revert
- Identify the commit you want to revert using
git log
. - Use
git revert <commit-hash>
to create a new commit that undoes the specified commit.
git revert 1a2b3c4d
- Push the new commit to the remote repository.
git push origin main
Warnings about force pushing
Avoid using git push --force
to overwrite the remote repository’s history, as it can cause issues for other collaborators. Instead, use git revert
to maintain a clean and transparent commit history.
Undoing Multiple Commits
To undo multiple commits, you can use git revert
with a range of commits or perform an interactive rebase.
Reverting a range of commits
git revert <oldest-commit-hash>..<latest-commit-hash>
Interactive Rebase
- Use
git rebase -i <commit-before-the-one-you-want-to-change>
to start an interactive rebase. - In the editor, change
pick
todrop
for the commits you want to remove. - Save and exit the editor to complete the rebase.
Recovering lost work
If you accidentally performed a hard reset and lost commits, you can use git reflog
to recover them.
- Run
git reflog
to view the log of all actions performed in the repository. - Identify the commit you want to restore and note its SHA-1 hash.
- Use
git checkout <commit-hash>
to switch to that commit. - Create a new branch to save the recovered changes:
git checkout -b recovered-branch
.
Best practices
- Use
git revert
instead ofgit reset
when undoing pushed commits to maintain a clean history. - Be cautious when using
git reset --hard
, as it permanently deletes changes. - Regularly communicate with your team to avoid conflicts when undoing commits on shared branches.
FAQs
Use `git revert -m 1 <merge-commit-hash>` to revert a merge commit, specifying the parent number (`-m 1`) to keep.
Resolve the conflicts manually, stage the changes, and then run `git revert --continue` to complete the revert operation.
Conclusion
Undoing Git commits, whether local or pushed, is a common requirement in any developer’s workflow. By understanding the different approaches, such as git reset
, git revert
, and git reflog
, you can confidently handle various scenarios and maintain a clean and accurate project history. Remember to follow best practices, communicate with your team, and always prioritize the integrity of your repository.