How To Cancel Requests in Axios
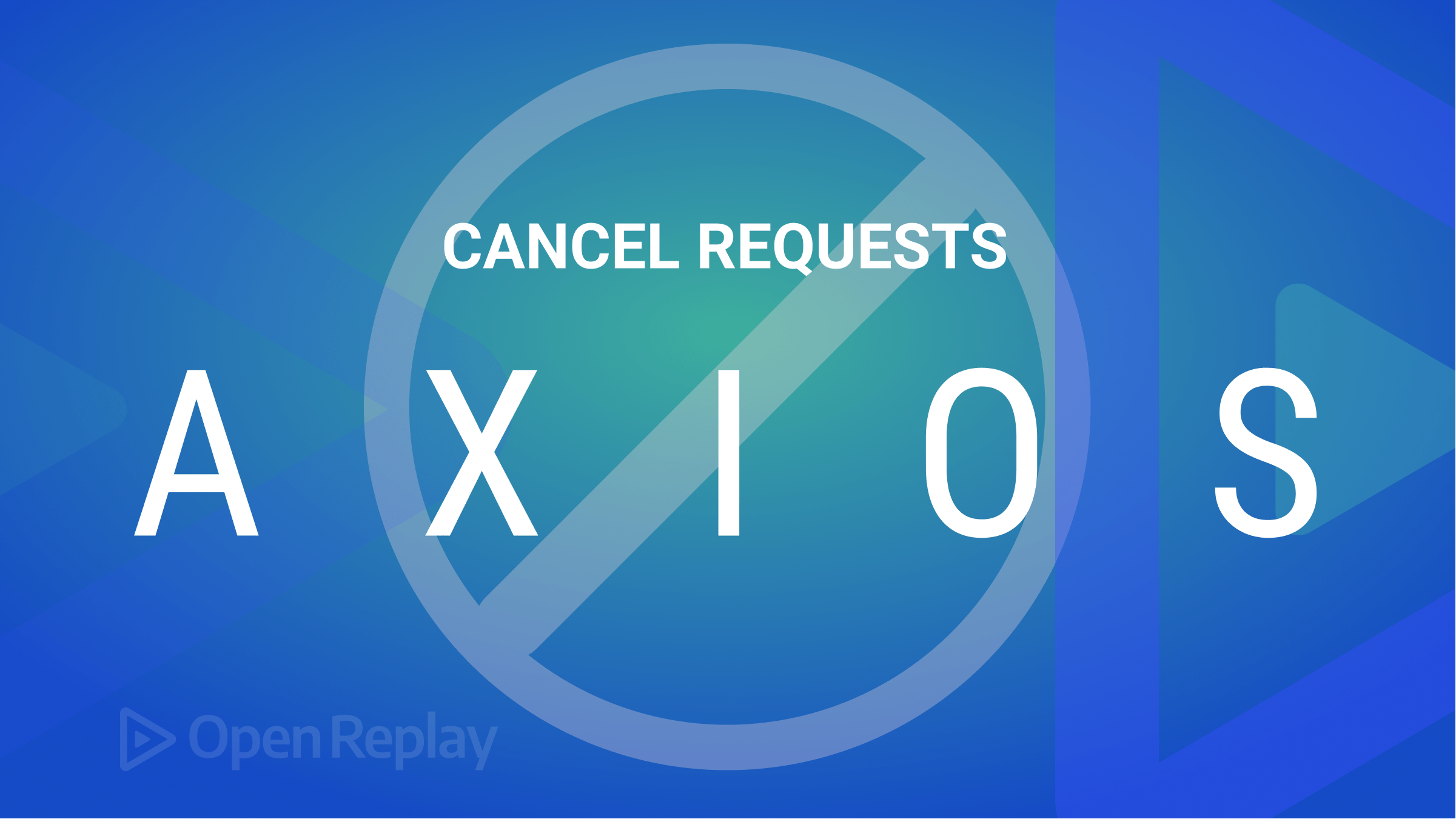
Axios is one of the most popular libraries for making HTTP requests in JavaScript. One of the many great features Axios supports is request cancellation. This allows you to abort a pending HTTP request to save resources when you are no longer interested in its response.
In this article, you will learn everything you need to know to become an expert when it comes to canceling requests in JavaScript. Specifically, you will see what HTTP request cancellation is, what it involves, and how to use it in Axios.
Let’s dig into how to cancel HTTP requests in Axios!
What Is HTTP Request Cancellation?
HTTP request cancellation is aborting a pending HTTP request before it has been completed or responded to by the server. When an HTTP request is canceled promptly, the memory and network resources allocated for it are immediately released.
Canceling HTTP requests is useful whenever the user or application no longer needs the result of the request. Specifically, let’s take a look at three common examples of HTTP request cancellation:
-
Search operations: When there is search
<input>
, the page might perform an AJAX every time the user types a new character. Since the user will likely change their query before the request completes, you should use cancellation to prevent unnecessary network traffic. -
Video streaming: When a user is streaming a video, the webpage keeps making new HTTP requests to download the next video segments. If the user pauses or skips to a different part of the video, you can adopt cancellation to abort the pending requests and save network bandwidth.
-
Upload/download: When uploading/downloading a file, users may change their minds during the process. This is why you should give them the ability to abort the request. One of the best ways to implement that feature is through HTTP request cancellation.
A similar approach to request cancellation is debouncing. This involves delaying the new request until a certain amount of time has elapsed since the last one. Just like request cancellation, debouncing allows you to reduce unnecessary network traffic. At the same time, HTTP request cancellation is more immediate and can quickly free up resources. Consider the example below.
A user is scrolling through a list of products on a web page. The web application makes a request to fetch more products as the user reaches the bottom of the list. Now, suppose the user starts scrolling down quickly. With debouncing, there may be a delay before new products are fetched. Instead, request cancellation can immediately abort unnecessary requests, allowing the page to retrieve new content immediately.
What Happens When You Cancel an HTTP Request?
When an HTTP request is aborted, what happens depends on when the cancellation occurs. Let’s consider the three possible scenarios:
-
Before the request is sent: If the cancellation occurs before the request is sent, the HTTP request is immediately discarded, and no further action is taken.
-
During the request transmission: If the cancellation occurs during the transmission of the request, the HTTP request is aborted, and no further data is sent to the server. The server may still receive and process the data it received before the cancellation, but the client will not wait for any response from the server.
-
After the request has been sent: If the cancellation occurs after the request is sent but before a response is received, the client will stop waiting for a response to that request. The server will process the HTTP request and send a response, but the client will not expect a response from it. So, the client is allowed to move on to new requests.
As you can see, canceling an HTTP request as early as possible is essential to save resources, both on the client and the server. In particular, since the server may still process a request if canceled too late, you should use HTTP request cancellation only on idempotent APIs.
If you are not familiar with this concept, an operation is idempotent when it can be repeated any number of times. The result of the operation will always be the same as if it were applied only once. Thus, you should consider request cancellation only on GET
requests, which are idempotent by nature.
It is time to learn how to cancel HTTP requests in Axios!
Cancelling HTTP Requests in Axios
Axios is a popular HTTP client for JavaScript and Node.js. At the time of writing, Axios supports two ways to cancel HTTP requests:
- With
CancelToken
: Deprecated in Axios sincev0.22.0
. - With
AbortController
: Introduced in Axiosv0.22.0
.
Let’s now dig into these two approaches.
Cancellation With CancelToken
This approach was deprecated with Axios v0.22.0
in October 2021, and you should no longer use it. Yet, considering Axios’s popularity, some legacy apps may still rely on an older version. So, it is still worth mentioning it.
You can cancel an HTTP request in Axios with CancelToken
as in the example below:
import axios from "axios"
// get the CancelToken object from axios
const CancelToken = axios.CancelToken
// create a cancel token instance
const cancelTokenSource = CancelToken.source()
// ...
// perform the HTTP GET request you want to cancel
axios.get('https://your-server.com/products', {
// specify the cancelToken to use
// for cancelling the request
cancelToken: cancelTokenSource.token
}).catch(function (e) {
// if the reason behind the failure
// is a cancellation
if (axios.isCancel(e)) {
console.error(e.message);
} else {
// handle HTTP error...
}
})
// ...
// cancel all the HTTP requests with the same
// cancelToken generated with cancelTokenSource.source()
cancelTokenSource.cancel('Operation canceled')
The snippets above create a cancel token instance called cancelTokenSource
using the CancelToken.source()
factory. Note that the value of the Axios cancel token associated with that instance is stored in cancelTokenSource.token
. Pass this value to the cancelToken
Axios config in all the requests you want to cancel together. When you call cancelTokenSource.cancel()
, all pending HTTP requests involving cancelToken
will be canceled.
Congrats! You know now how to cancel HTTP requests with CancelToken
in Axios.
Cancellation With AbortController
With version v0.22.0
, Axios embraced a more standard approach to HTTP cancellation. Specifically, it deprecated CancelToken
and added support for AbortController
. Note that canceling HTTP requests through AbortController
is supported by all browsers and also by other HTTP clients, such as Fetch or node-fetch
.
AbortController
is a native JavaScript controller object that enables you to abort web requests when desired. Specifically, AbortController
contains an AbortSignal
object instance in the read-only signal
property. This allows you to communicate with a DOM or HTTP request and abort it when the abort()
method is called. When a request gets canceled, a JavaScript AbortError
is raised. This will be wrapped by Axios in a CanceledError
.
Use AbortController
to cancel a request in Axios as in the example below:
import axios from "axios"
// initialize an AbortController instance
const abortController = new AbortController()
// ...
// perform the HTTP GET request you want to cancel
axios.get('https://your-server.com/products', {
// specify the AbortController signal to use
// for cancelling the request
signal: abortController.signal
}).catch(function (e) {
// if the reason behind the failure
// is a cancellation
if (axios.isCancel(e)) {
console.error('Operation canceled');
} else {
// handle HTTP error...
}
})
// ...
// cancel all the HTTP requests with the same
// signal read from abortController.signal
abortController.abort()
Here, an AbortController
instance gets initialized. Then, its signal
property is passed to the Axios signal
config in a cancellable HTTP GET request. When calling the abort()
method on the controller, all pending HTTP requests involving that signal
will be canceled.
Et voilà! You can now cancel HTTP requests using AbortController
in Axios.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
HTTP Request Cancellation in Action
Let’s see what happens in your browser’s developer tools when an HTTP request gets canceled.
In the “Network” section of your browser’s dev tools, you will see something like this:
Note that “Status” is “(canceled),” which specifies that the HTTP request has been canceled.
Then, in the console, you will see some “Operation canceled” errors logged by the console.error()
instruction:
Great! This is how you can monitor canceled requests in your browser’s developer tools.
Conclusion
This tutorial is over! You now know what HTTP request cancellation is, how it works, and how to implement it in Axios. Also, you saw how the browser behaves when your JavaScript application cancels an HTTP request.
As learned here, canceling HTTP requests is easy. In this article, you saw everything you need to know to get started with request cancellation with Axios in both the back-end and front-end.