How to Debug API Issues with JWT Decoders
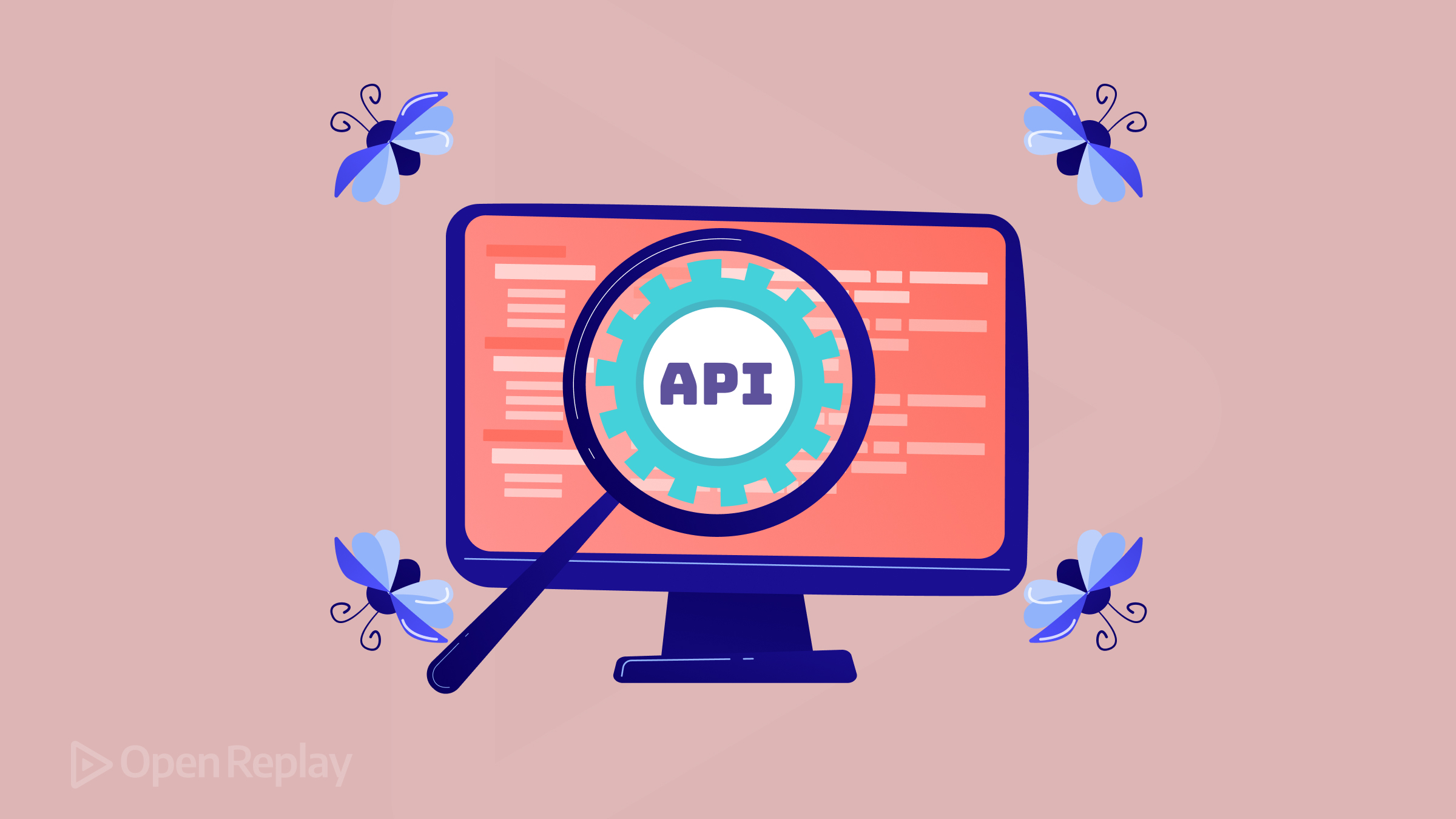
APIs are the bacone of modern applications, facilitating seamless communication between systems. However, debugging API issues can often feel like searching for a needle in a haystack—especially when dealing with JSON Web Tokens (JWTs).
A JWT Decoder is an essential tool for inspecting and validating tokens used in API authentication. This guide explains how to leverage a JWT decoder to debug common API issues effectively.
Key Takeaways
- JWT decoders help identify and resolve common issues like expired tokens, incorrect claims, and invalid signatures.
- Use a JWT decoder to inspect tokens for debugging authentication and API issues.
- The JWT Decoder is a free, secure tool for decoding and validating tokens.
Understanding the Role of JWTs in APIs
JWTs are commonly used for:
- Authentication: Verifying a user’s identity.
- Authorization: Controlling access to resources.
- Data Integrity: Ensuring the payload remains tamper-proof.
When an API call fails, decoding the JWT can provide valuable insights into why the token was rejected.
Identifying Common JWT Issues in APIs
1. Expired or Invalid Tokens
- APIs reject expired tokens or those with incorrect issuance (
iat
) or expiration (exp
) times. - Use a JWT decoder to check the
exp
claim and verify token validity. - Confirm the
iat
claim matches the server’s time.
2. Incorrect Claims
- JWTs include claims like
sub
(subject),aud
(audience), andiss
(issuer). - A JWT decoder lets you inspect these claims to ensure they align with the API’s expectations.
3. Signature Validation
- API signatures validate JWTs to ensure they haven’t been tampered with.
- Decode the JWT header to identify the signing algorithm.
- Verify the signature using the API’s secret or public key.
Steps to Debug API Issues with a JWT Decoder
- Retrieve the JWT from the API Request
- Extract the token from the
Authorization
header or request payload.
- Extract the token from the
- Decode the JWT Using the Tool
- Use the JWT Decoder:
- Paste the token into the tool.
- View the header, payload, and signature.
- Use the JWT Decoder:
- Inspect Claims for Discrepancies
- Identify invalid or unexpected values in the payload claims.
- Validate the Signature
- Cross-check the token signature using the API’s key.
- Identify and Resolve Issues
- Compare decoded values against the API’s expected configuration and resolve mismatches.
Best Practices for Using JWT Decoders
- Keep Tokens Secure: Never share sensitive JWTs with third parties.
- Use HTTPS: Decode tokens only over secure connections.
- Validate Regularly: Test and inspect tokens during development to avoid runtime issues.
FAQs
A JWT decoder extracts and displays the header, payload, and signature of a JWT, helping inspect its contents.
Yes, the [JWT Decoder](https://openreplay.com/tools/jwt-decoder/) processes tokens locally in your browser, ensuring data security.
Yes, the tool supports signature validation, making it easy to confirm token integrity.
Conclusion**
A JWT Decoder. is an invaluable tool for debugging API issues, allowing you to inspect and validate tokens with ease. By decoding the header, payload, and signature, you can quickly identify mismatches and resolve common issues like invalid claims or expired tokens.