How to Decode JWTs (JSON Web Tokens) Instantly
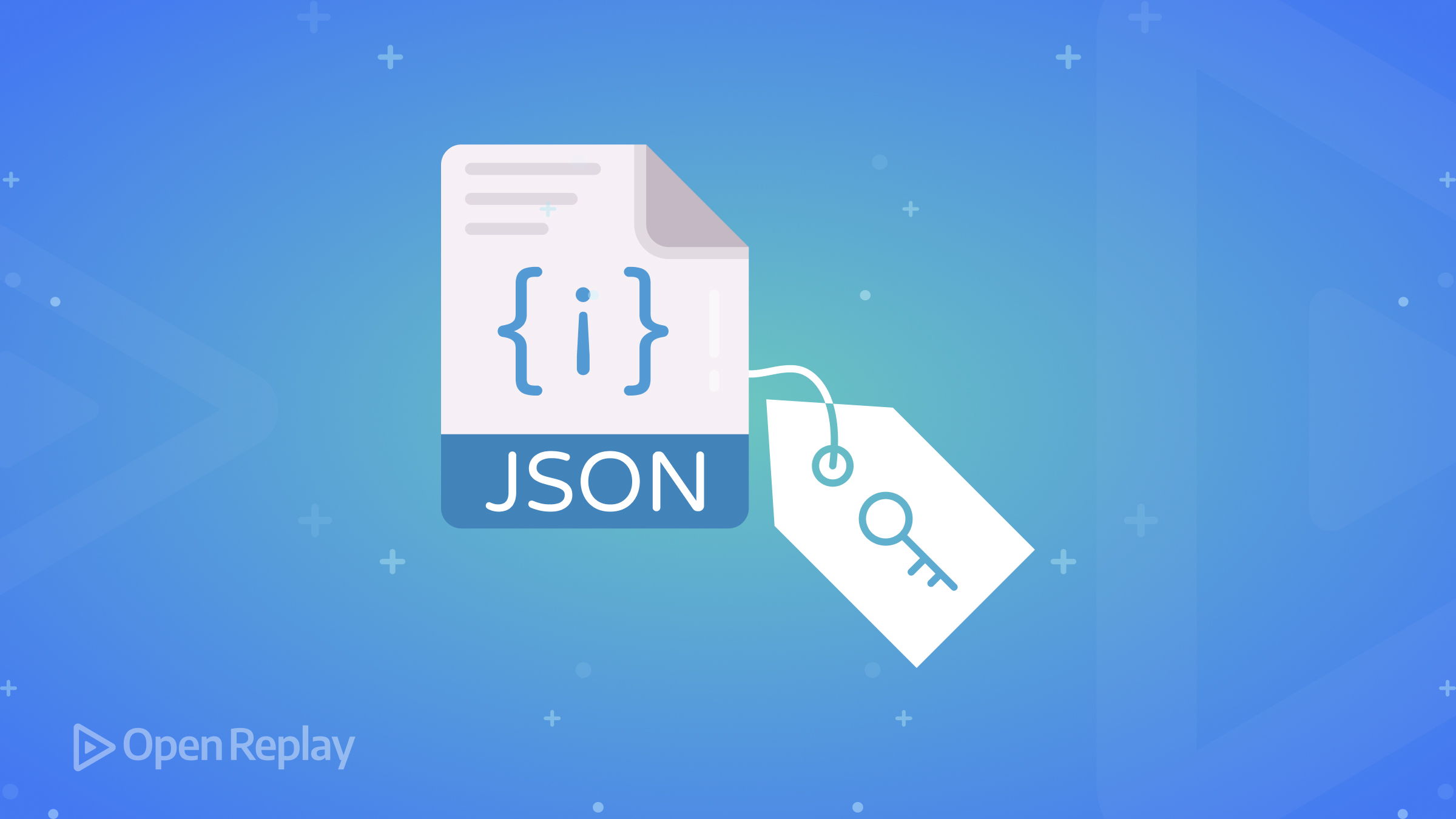
JSON Web Tokens (JWTs) are a popular method for securely transmitting information between parties. However, understanding and decoding JWTs can sometimes feel overwhelming, especially when dealing with intricate payloads or signatures. How do you decode a JWT to verify its content quickly?
This guide explains the structure of a JWT, why decoding it is essential, and how to decode JWTs effortlessly using tools like the JWT Decoder.
Key Takeaways
- JWTs are widely used for secure information exchange and authentication.
- Decoding JWTs helps verify claims, debug issues, and ensure security.
- Use tools like the JWT Decoder for quick and accurate decoding.
What is a JWT?
A JSON Web Token (JWT) is a compact, URL-safe token used for authentication and information exchange. It’s widely used in APIs, web applications, and single sign-on (SSO) systems.
Structure of a JWT
A JWT consists of three parts separated by dots (.
):
- Header: Contains metadata about the token, such as the algorithm used for signing.
- Payload: Includes the claims or data being transmitted.
- Signature: Ensures the token’s integrity and authenticity.
Example JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
Why Decode a JWT?
Decoding a JWT allows you to:
- Verify Claims: Understand the information (claims) it contains, such as user roles or session details.
- Debug API Calls: Identify issues in authentication or data transmission.
- Ensure Security: Check if the token has been tampered with by verifying its signature.
How to Decode a JWT
Method 1: Using an Online Tool
The easiest way to decode a JWT is by using an online tool like the JWT Decoder:
- Paste Your JWT: Copy and paste the token into the input field.
- View Decoded Output: Instantly see the header, payload, and signature in a readable format.
- Analyze Claims: Review the payload’s content to ensure it meets your expectations.
Method 2: Using Programming Languages
For developers, decoding a JWT programmatically provides more control. Here’s an example in Python:
import base64
import json
def decode_jwt(token):
header, payload, signature = token.split('.')
decoded_header = base64.urlsafe_b64decode(header + '==').decode('utf-8')
decoded_payload = base64.urlsafe_b64decode(payload + '==').decode('utf-8')
return json.loads(decoded_header), json.loads(decoded_payload)
jwt_token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c"
header, payload = decode_jwt(jwt_token)
print(header)
print(payload)
Tips for Secure Decoding
- Never Trust the Payload: The payload can be read without verification, so never use it to make critical decisions without validating the signature.
- Validate the Signature: Ensure the token’s integrity by verifying its signature using the correct secret or public key.
- Check Expiry: Always review the
exp
claim to confirm the token has not expired.
Key Benefits of Using the JWT Decoder Tool
- Time-Saving: Decode tokens instantly without needing any setup.
- User-Friendly: Provides a clear breakdown of the token’s parts.
- Secure: Ensures no sensitive data is stored during decoding.
FAQs
Yes, you can decode a JWT to view its header and payload. However, the signature must be validated to ensure the token’s integrity.
Reputable tools like the JWT Decoder prioritize data security, but avoid using online tools for highly sensitive tokens.
If the signature doesn’t match, it means the token has been tampered with and should not be trusted.
Conclusion
Decoding JWTs is an essential step in understanding and verifying the information they contain. Whether you’re debugging APIs, verifying claims, or enhancing security, tools like the JWT Decoder can simplify the process. Start decoding JWTs today to streamline your development and security workflows.