All about the JSON format
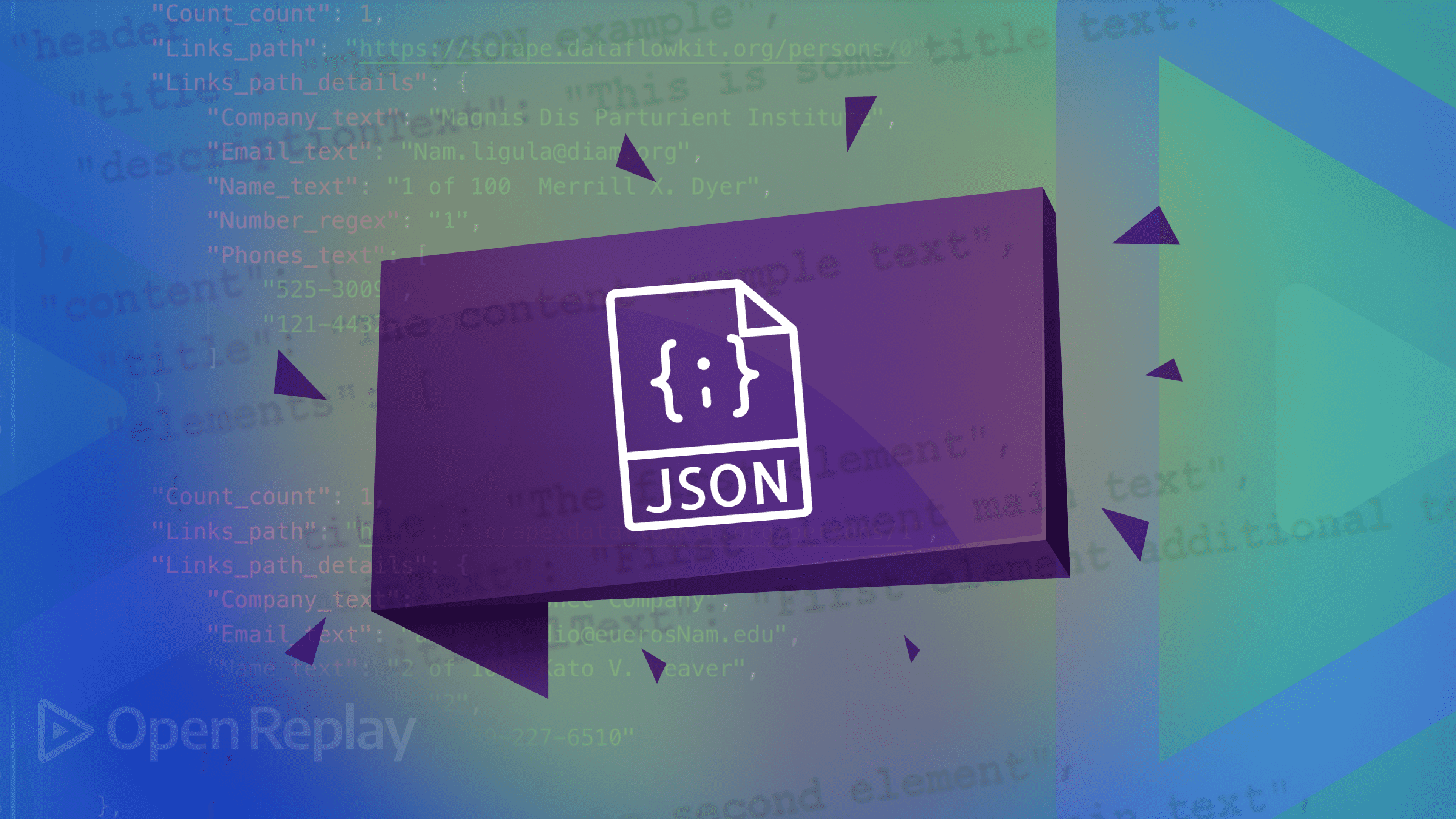
JSON (JavaScript Object Notation) is a text format representing data in key-value pairs. JSON is lightweight and widely used in the tech industry today for various purposes, one of which is presenting data in a human-readable format. Although it looks like an ordinary JavaScript object, it is very different. JSON files are saved using the extension .json
.
JSON is a widely accepted format for many operations like configuring files and interchanging data (e.g., API data). It is fast, efficient, and simple to use as it consists of keys and values where the values can be accessed using their corresponding key.
By the end of this article, you will have learned what JSON is, its benefits, and how it compares to YAML and JavaScript objects. You will also learn some use cases of JSON and JSON methods such as JSON.parse()
and JSON.stringify()
.
What is JSON?
JSON is a text-based format for representing objects. This format basically consists of a key (in quotes) and then a value (which can also be a key to other values in cases where you have nested data. This is what a typical JSON format looks like:
{
"name":"Narudesigns",
"age":25,
"skills":["JavaScript", "git", "React", "Vue"],
"isAfrican":true,
"experience":{
"Google":"Software Engineer",
"Amazon":"Senior Software Engineer"
}
}
In reality, unneeded spaces would be left out, and the format would be:
{"name":"Narudesigns","age":25,"skills":["JavaScript","git","React","Vue"],"isAfrican":true,"experience":{"Google":"Software Engineer","Amazon":"Senior Sofware Engineer"}}
JSON data types are very similar to typical JavaScript object types, and they include the following:
- Strings, as in
{"name":"Narudesigns"}
- Numbers, as in
{"age":25}
- Booleans, as in
{"isAfrican":true}
- nulls, as in
{ "activeUser":null }
- Arrays, as in
{"skills":["JavaScript", "git", "React", "Vue"]}
- Objects, as in:
{"experience":{"Google":"Software Engineer","Amazon":"Senior Software Engineer"}}
Some of the advantages of using JSON are:
- JSON uses plain text format, which makes it lightweight and easy to store data.
- It is super easy to learn, read, and understand.
- Using JSON, data is easily serialized, transferred, and accessed.
- JSON is easily accessible by many applications, given its ability to parse data.
- JSON can be used in several cases, like you learned above, such as APIs, configuring files, storing data, etc.
We may compare JSON to other objects and formats.
JSON vs. JavaScript Object
Like JSON, JavaScript objects use key-value pairs to store and access data, but here are the differences between JSON and JavaScript objects:
- JSON keys must be of string type (i.e., they must be wrapped with double quotes), but they can accept values with various data types, which are covered in a later section of this article. On the other hand, JavaScript objects don’t require keys to be of string type. They can be a string, variable, number, or evaluative expression, e.g.,
{ [state]: "Florida" }
, wherestate
is a variable or computed value. - JSON value can not be a function (i.e., JSON can not have methods as a value). On the other hand, JavaScript objects can have any data type as its value, including methods (functions).
- JSON has just 2 methods which are
JSON.parse()
andJSON.stringify()
. Unlike JSON, JavaScript objects have several methods which can be used to manipulate, access, and store data.
JSON vs. YAML
YAML (YAML Ain’t Markup Language) is also a text-based format used to represent data in a readable format. YAML also uses key-value pairs but in a different syntax from JSON. A YAML document usually begins with three dashes ---
and ends with three dots ...
just like you can find it written on the official YAML website.
YAML uses key-value pairs, which are separated by colons. It uses an indentation style similar to python to represent the data structure.
---
## user details
name: Narudesigns
age: 25
skills:
- JavaScript
- git
- React
- Vue
isAfrican: true
experience:
google: Software Engineer
amazon: Senior Sofware Engineer
...
Some of the differences between JSON and YAML include the following:
- Although both can handle similar situations, JSON is more favorable regarding data serialization due to its available parsing methods. This makes it suitable for APIs.
- YAML allows you to add comments, while JSON does not support using comments.
- JSON can be used for storing data, giving its nearness to JavaScript objects. Unlike JSON, YAML is used chiefly for configurations for tools like Kubernetes, Docker, and code infrastructures.
- JSON uses double quotes for its property names; YAML supports both single and double quotes.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Use Cases of JSON
JSON is popularly used across various fields in the tech industry today. Some of the use cases of JSON are:
APIs
API schemas are defined using JSON format. This schema is a way to describe the structure of the API to users who intend to work with it. Here’s what a typical API schema looks like:
{
"title":"User",
"description":"An entity",
"type":"object",
"properties":{
"name":{
"description":"User's name",
"type":"string"
},
"age":{
"description":"User's age",
"type":"number",
"minimum":18,
"maximum":64
}
}
API requests (payloads) and responses are usually represented in JSON format.
Configuring Files
JSON is also very much used in configuring files. Libraries and tools such as React, Vue, Vite, npm, etc., use JSON format for project configurations. Here’s a sample from cloud.google.com of what such configuration looks like:
{
"deployment": {
"files": {
"example-resource-file1": {
"sourceUrl": "https://storage.googleapis.com/[MY_BUCKET_ID]/example-application/example-resource-file1"
},
"images/example-resource-file2": {
"sourceUrl": "https://storage.googleapis.com/[MY_BUCKET_ID]/example-application/images/example-resource-file2"
},
}
},
"id": "v1",**
"handlers": [
{
"urlRegex": "/.*",
"script": {
"scriptPath": "example-python-app.py"
}
},
],
"runtime": "python27",
"threadsafe": true,
}
Storing Data
JSON data can be serialized and stored on your computer using browsers’ localStorage
and sessionStorage
. This is super helpful when you must cache non-secret or insensitive data and store it in your users’ devices for future usage. A good use case of this is for saving insensitive user details for quick access instead of storing and retrieving them from the database.
JSON Methods
JSON has two methods that come in handy when working with data: JSON.stringify()
and JSON.parse()
. User data is first serialized using the JSON.stringify()
method and then may be stored in the localStorage
or sessionStorage
or used in another way. In case of retrieval, the data needs to be deserialized to a proper JavaScript object before it can be used, and that’s possible with the help of the JSON.parse()
method.
Here are more scenarios where these methods are handy:
Date Serialization
When building APIs, dates or timestamps are one of the most common properties in the API response. However, returning the actual date format in an API response can be problematic due to differences in time zones.
The solution is to serialize the date using JSON.stringify(new Date())
method, and then it is returned as a string like so:
{
"timestamp": "2022-11-10T02:05:20.478Z"
}
Therefore, the same date is used regardless of where this API is consumed. You can use JSON.parse(timestamp)
to get the actual date object.
2022-11-10T02:05:20.478Z
Object Deep Copy
If you’re unsure what deep copy means, please read this. Essentially, it is a type of copy where the original object and copied object points to different memory locations. Therefore, an update in one does not affect the other.
This can be achieved using the spread operator ...
, but when it comes to nested objects, this approach fails as it only deep-copies top-level properties.
The solution is to serialize the object using the JSON.stringify()
method and then assign it to a new variable using the JSON.parse()
method like so:
const obj = {
name: "Narudesigns",
family: {
brothers: ['Pitaz', 'Dalu46']
}
}
const serializedObj = JSON.stringify(obj);
const deepCopy = JSON.parse(serializedObj);
// change in deepCopy doesn't affect obj
deepCopy.family.brothers = ['Peter', 'Dalu']; // ['Peter', 'Dalu']
obj.family.brothers; // ['Pitaz', 'Dalu46']
Conclusion
You’ve come to the end of this article. At this point, you learned about JSON format, what it is, why you should care, how it compares to typical JavaScript objects and YAML syntax. You’ve also learned about cases where JSON can be used, its advantages, and its data types.
JSON is super easy to learn and very much trendy in the tech industry today. Chances are you already use them, but if you haven’t, this is enough information to get you started.
Thanks for reading!
A TIP FROM THE EDITOR: JSON is used in several articles; don’t miss Authentication With JWT For Your Website and Data Fetching Techniques with React, for example!