How to fix the 'Unexpected End of JSON Input' error in JavaScript
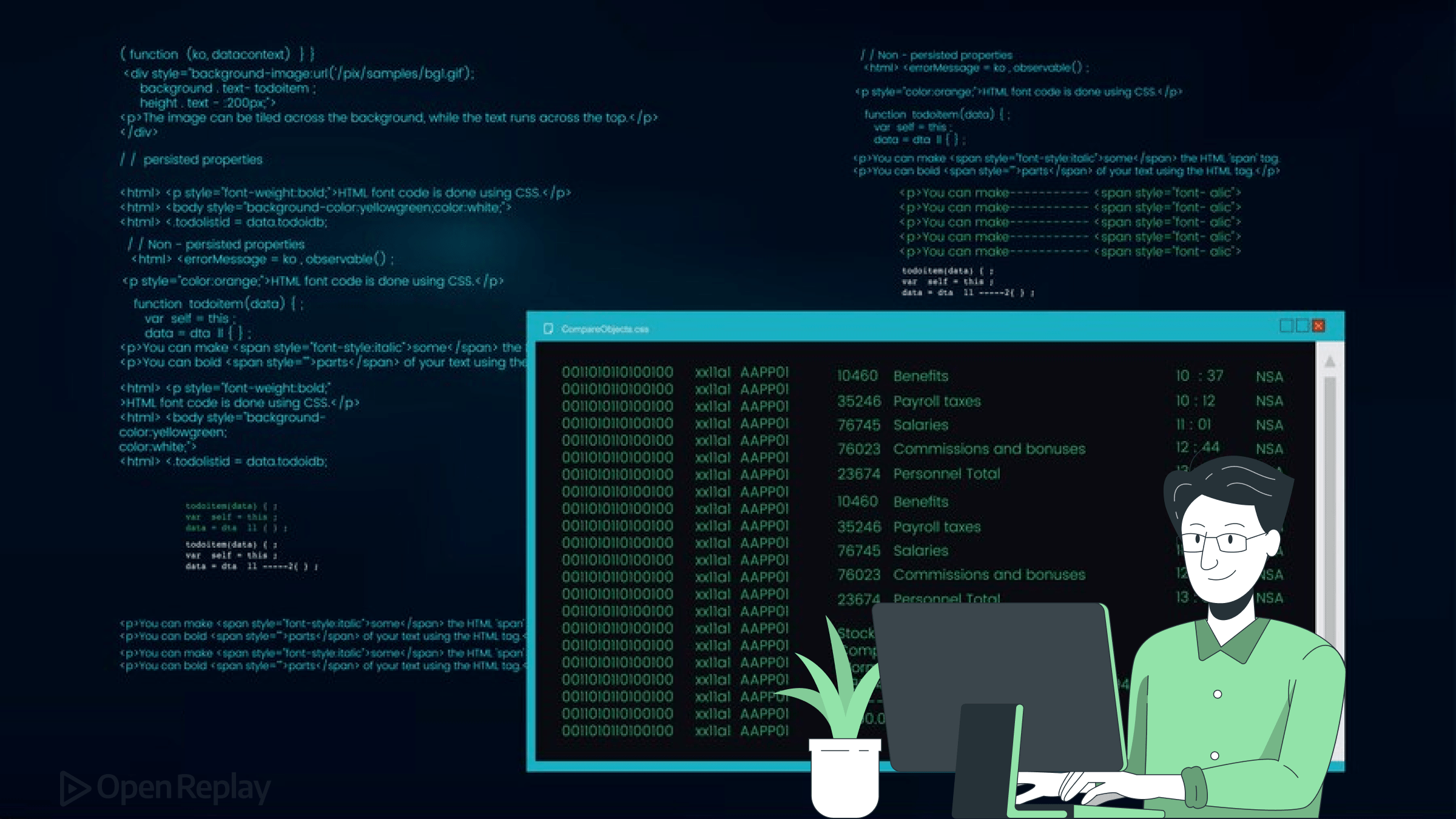
Most JavaScript applications use the JSON format to communicate with each other. Thus, parsing JSON is more common than ever. However, that task does not always work as expected, resulting in “Unexpected end of JSON input” errors. This article shows how to fix that error.
Discover how at OpenReplay.com.
“Unexpected end of JSON input” is a popular JavaScript error raised by JSON.parse()
. The error occurs when your code attempts to parse:
- An empty string
- An empty array
- A malformed JSON string
The error indicates that the application cannot consume some JSON because it is not parsable or malformed. Learn more about how to read and write JSON in JavaScript.
Fix the JSON Input Syntax Error in JavaScript
In most cases, a JavaScript web application deals with JSON data by either reading it from a local file—such as a configuration file—or retrieving it from a web server, typically via an API call. See how to approach the “Unexpected end of JSON input” error in both scenarios!
Local JSON Data
Suppose you are trying to read a local config.json
file in Node.js and parse its content with JSON.parse()
:
const configFile = fs.readFileSync("config.json", "utf-8");
const config = JSON.parse(configFile);
Or, equivalently, you are importing the file directly:
const config = require("./config.json");
// for Node.js ESM users:
// import config from "./config.json" with { type: "json" };
Do not forget that Node.js allows you to import a JSON file directly using require()
—or with import
in ESM applications. Behind the scenes, the application automatically parses the JSON content into a JavaScript object using JSON.parse()
.
The same logic applies to frontend applications using a bundler like Webpack. In this case, the syntax for importing JSON directly from a file is:
import config from "./config.json";
To avoid the “Unexpected end of JSON input” error, verify that your local JSON file contains valid JSON. A recommended way to do so is to open the file, copy its content, and paste it into an online JSON linting service, such as JSONLint:
As you can see, this tool indicates whether the JSON is valid and, if not, where it contains invalid data.
Note that this approach also works with empty strings, as an empty string is not valid JSON:
Remote JSON Data
If a remote endpoint is responsible for returning invalid JSON, you will encounter the “Unexpected end of JSON input” error:
- Manually, when parsing the response data with
JSON.parse()
- Directly, if your HTTP client automatically parses the response for you
If you are not in control of the server’s response, you cannot do much in this scenario. The recommendation is to wrap your API call with a try...catch
block to handle the error gracefully:
try {
// make an API request
const response = await fetch("https://example.com/api/v1/users");
// parse the response as JSON (uses JSON.parse() behind the scenes)
const data = await response.json();
// handle the response data...
} catch (error) {
console.error("Error while fetching data:", error);
}
This way, the JSON parsing issue will not crash your entire application. Also, you should log the error to check that the response data contains invalid JSON. If that is the case, report the issue to the backend team.
FAQs
Can the ‘Unexpected end of JSON input’ occur both in Node.js and the browser?
Yes, parsing JSON in JavaScript is possible both in the back and front ends, whether from local files or remote responses.
Is it possible to automatically fix broken JSON in JavaScript?
Yes, by feeding the broken JSON data to automatic JSON repair libraries like jsonrepair
, json-fixer
, or jsonfix
.
Conclusion
Following the approaches described in this article, you can fix the “Unexpected end of JSON input” error when dealing with local and remote JSON data.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.