How to Integrate the YouTube Player in your React App
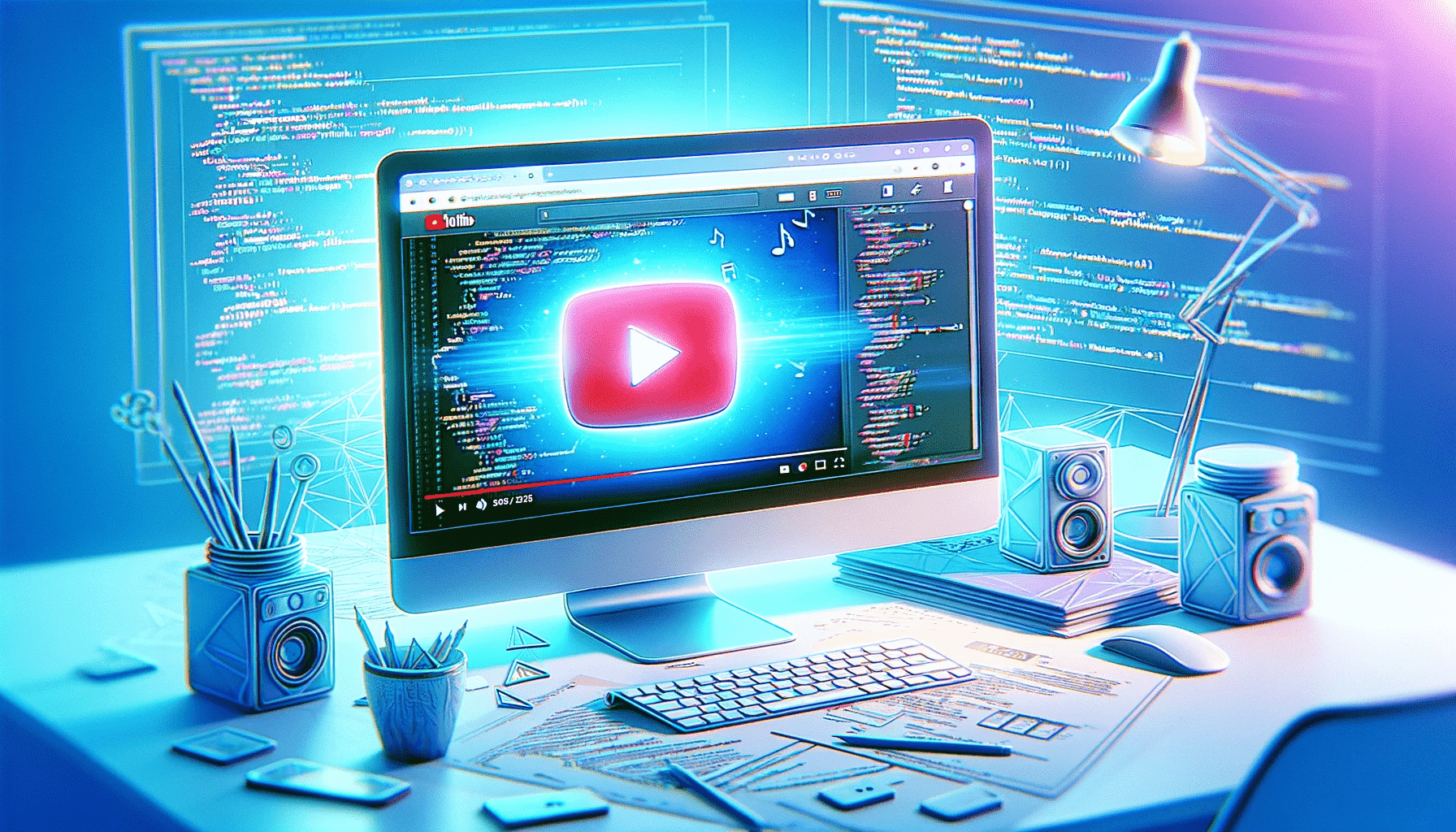
YouTube, the world’s most popular video-sharing platform, hosts a diverse range of content, including vlogs, tutorials, music, and more. Many sites have this social media as a way to enhance their content, enrich user engagement and experience, and promote interaction without having to leave the website. This article will look at how to easily integrate a YouTube player into a React application.
Discover how at OpenReplay.com.
The react-youtube
library is a React component that wraps thinly around the YouTube IFrame Player API, enabling integration of YouTube functionalities into React applications. YouTube IFrame Player API allows embedding a YouTube player on your website. This player is controlled by JavaScript.
This YouTube API within the react-youtube library controlsf the video playe,r, like pausing, playing, stopping, queueing videos for playback, volume adjustment, etc. It is the core of the library.
Importantly, the library serves as the bridge between the React application and the YouTube IFrame Player API, simplifying the integration process with the API functionalities for developers.
Use Cases
Several common use cases exist for integrating the react-youtube
library into your React applications. Some of the common scenarios include:
YouTube Integration
When your application is integrated with the YouTube API, you can provide information about a specific channel and easily display videos using react-youtube
. You can easily hop from one video to another with this API.
To use the YouTube API, you must register your application in the Google Developers Console to obtain an API key. This key is a uniquely generated code used as a means of access and authentication for using an API. Once obtained, it can be used to make authorized requests to the YouTube Data API.
The API key is important for making queries to retrieve channel information, such as video uploads, channel metadata, and playlists. Through this integration, the application can fetch and display data dynamically from a specified YouTube channel provided.
With the YouTube API connected to your React app, you can access videos, channel IDs, video comments, categories, etc. Users can navigate the channel’s video content displayed directly within your application using the react-youtube
player component.
Tutorials
Online courses and other e-learning resources can enhance user engagement and experience by visually representing the content with react-youtube
.
In an educational website, using react-youtube
allows for integrating tutorial videos related to various subjects. With this feature, learners can easily access relevant video content to watch alongside their learning, improving the learning experience on the website.
Cooking sites with step-by-step video guides for recipes embedded in them are popularly used these days to make users easily follow along with the recipe instructions, improving user interaction and retention.
For online courses, the react-youtube
player allows for integrating course introduction videos, module summaries, or additional resource materials, adding a multimedia angle to the learning process.
Movie Site
A movie website for reviews, trailers, or promotional blogs can benefit greatly from using the react-youtube
library.
Movie review websites can offer snippets of movie trailers to view or specific scenes using the react-youtube
player. This allows users to gain a preview of the movie being reviewed, enhancing their understanding and potentially influencing their decision to watch the film.
For movie downloading sites, embedding trailers using react-youtube
can entice users by providing a brief yet captivating view of the movies available for download.
Promotional blogs for movies can add exclusive behind-the-scenes footage or interviews with the cast and crew, enhancing the audience’s engagement and interest in the movie.
Content-driven Websites
Articles on blogs or news outlets can be enhanced with related videos added using the react-youtube
library to significantly enhance user engagement and comprehension.
For news outlets, embedding relevant video content alongside articles provides a better understanding of the reported events or topics.
Blogs can be integrated with react-youtube
to supplement the written content with video explanations, interviews, or demonstrations, enriching the user’s reading experience.
Setting Up The App
- Begin by creating a React application:
npx create-react-app youtube-player-app
- Navigate to your application directory:
cd youtube-player-app
- Start your application:
npm start
With this successful setup, your application is ready for integration.
- To use the
react-youtube
library, you’ll need to install it via npm:
npm install react-youtube
YouTube Component
After installing the library, create a components
folder within the src
directory and add a component named YoutubePlayer.jsx
.
Next, clear out the default content in the App.js
component and import the newly created YoutubePlayer
component into it:
import './App.css';
import YoutubePlayer from './components/YoutubePlayer';
function App() {
return (
<div className="App">
<YoutubePlayer />
</div>
);
}
export default App;
Here is the YoutubePlayer.jsx
component:
import React from "react";
import YouTube from "react-youtube";
const YoutubePlayer = () => {
// YOUTUBE VIDEO FUNCTION
const opts = {
width: "100%",
borderRadius: "2rem",
playerVars: { autoplay: 1 },
};
const videoReady = (event) => {
event.target.pauseVideo();
};
return (
<>
<h1>YouTube Player</h1>
<div>
<div
style={{
maxWidth: "800px",
margin: "auto",
marginTop: "12px",
minHeight: "30vh",
borderRadius: "12px",
overflow: "hidden",
}}
>
<YouTube
videoId="2g811Eo7K8U"
opts={opts}
onReady={videoReady}
/>
</div>
</div>
</>
);
};
export default YoutubePlayer;
The application should look like this:
The YouTube component from the react-youtube
library takes in several variables. The most important of them all is the videoId
.
The videoId
variable, as the name implies, is the video’s id. Every YouTube video has an ID that’s part of its URL. To access any video on YouTube, this is the format:
https://www.youtube.com/watch?v={videoId}
So, using the react-youtube
library, an ID is simply needed to integrate the video into the application. As shown in the image above, the videoId
provided displays the video of the given ID.
The opts
variable takes in an object that allows customization of the player’s behavior. This includes styling the video player like height, width, etc., and also playerVars
, which take in player parameters like autoplay
(play video after page load), controls
, loop
, etc., used to control the behavior and appearance of the player.
react-youtube
also takes in other important props. These include onReady
, a callback function triggered when the video player is ready for interaction, onError
, onPause
, title
, etc., for use depending on how you want your player to be.
Conclusion
In conclusion, integrating the react-youtube
library into a React application offers a very easy way to enhance user engagement and content presentation. This library opens the door to various use cases, making it a valuable asset for developers aiming to incorporate dynamic video content into their projects. We’ve demonstrated the advantages of using the’ react-youtube’ library by exploring use cases such as YouTube API integration, tutorials, movie sites, and content-driven websites. Whether you aim to provide a curated selection of YouTube videos, offer tutorial content, or movie reviews with trailers, this library is a reliable solution.
Using react-youtube
goes beyond technical integration. It transforms the user experience by providing visual accessibility and dynamic content. This not only captivates the audience but also makes the website more engaging and informative. Whether you’re developing educational platforms, movie review sites, or content-driven blogs, react-youtube
is a valuable addition to your toolkit, enhancing your web presence and captivating your audience with compelling video content.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.