How to use React Native Vector Icons
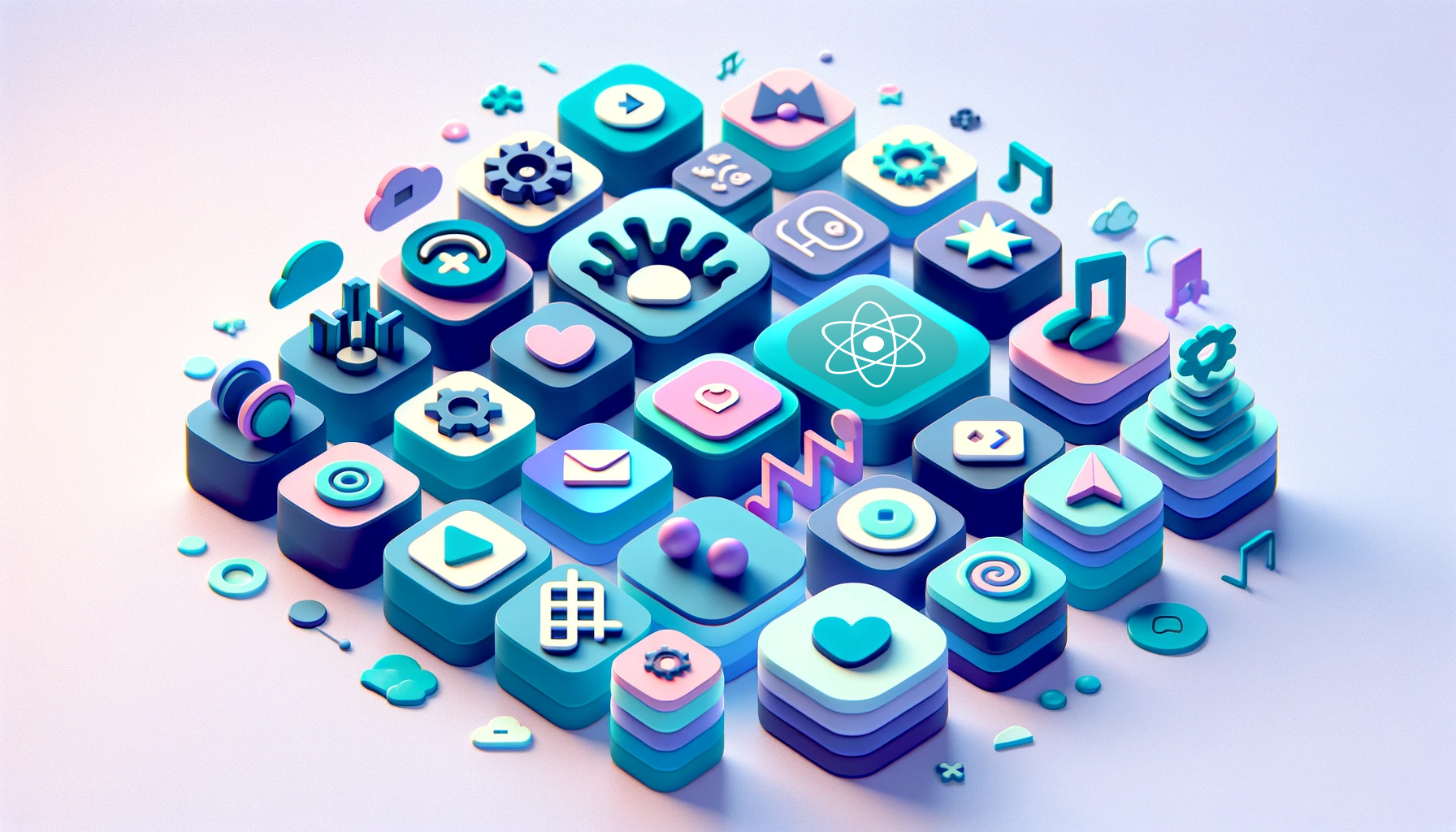
React Native Vector Icons is a powerful library for developers building mobile apps with elegant and scalable icons on Android and iOS using React Native. This article will explore the problems it addresses and the significance and practical applications of React Native Vector Icons.
Discover how at OpenReplay.com.
Before React Native Vector Icons, developers faced challenges dealing with inconsistent icon libraries and pixelated icons that appeared blurry on various devices, and they encountered difficulties in maintaining a consistent UI across different platforms.
By leveraging React Native Vector Icons, you can eliminate the need for platform-specific icon libraries. This ensures the provision of sharp and scalable icons, promoting consistency across diverse devices and screen sizes and contributing to a unified design language.
Let’s take a quick detour to understand the building blocks: vector icons. Unlike their pixelated cousins, vector icons are built using mathematical formulas and points. This magic trick makes them scalable to any size without losing sharpness, ensuring crisp and consistent visuals on any device, big or small.
Vector icons play a vital role in mobile app development due to their scalability, guaranteeing a consistent and sharp appearance across various screen sizes. Their adaptability minimizes the necessity for multiple versions of the same image, fostering efficiency and elevating the visual appeal of mobile applications.
In mobile applications, achieving a seamless integration of icons is vital for enhancing the user experience. Well-integrated icons play a role in creating a smooth and intuitive interface, guiding users effortlessly through the app’s functionalities. Consistency and thoughtful design in icons enhance navigation, making it easier for users to grasp the app’s features and actions. When icons are seamlessly integrated into the overall design, it creates visual harmony, reinforcing the app’s branding and aesthetics. To sum up, a well-integrated set of icons significantly influences user experience by promoting clarity, ease of use, and a visually pleasing interface.
Getting Started with React Native Vector Icons
In this section, we’ll guide you through the installations of React Native Vector Icons in your application, covering the necessary configurations. We assume that you already have a React Native application running on your local machine. If not, refer to the React Native official documentation for guidance on installing a React Native application.
Installing Necessary Dependencies
To initiate the installation of the React Native Vector icon, copy and paste the provided code into your project’s terminal:
npm install --save react-native-vector-icons
Once the installation is completed, move on to the next section to configure the React Native Vector icon for both Android and iOS platforms appropriately.
Configuring Android and iOS Platforms
In this section, we’ll guide you through the configuration of React Native Vector Icons for both Android and iOS. The configuration steps are sourced from the official documentation on GitHub, and you can access it through this link. If you encounter any challenges during the configuration process, you can also refer to the official documentation for assistance.
Android: To set up vector icons for Android, navigate to the “app” folder within the “Android” directory and open the build.gradle
file. Paste the provided code snippet at the top section of the build.gradle
file.
apply from: "../../node_modules/react-native-vector-icons/fonts.gradle"
Here is a visual representation illustrating the specific location where the code should be pasted, as previously described:
Following that, sync the gradle file by navigating to the Android folder in your terminal and executing the provided code below:
./gradlew clean
With the minimal setup outlined in this section, you can begin integrating vector icons into your application. For additional information regarding the configuration, feel free to navigate to the provided link for further details.
IOS: To incorporate vector icons into your iOS application, all that’s required is to import the icon files into your Xcode. The following is a detailed guide outlining the steps you must follow for this process to function correctly.
Step 1: Launch Xcode by navigating to your iOS folder and double-clicking on your-app-name.xcworkspace
, as illustrated below:
Following that, your Xcode window will appear, as depicted below:
Subsequently, you must generate a file named “Fonts” within MainApp. To accomplish this, right-click on MainApp (your app name) and select “New Group,” as indicated below:
Here is the newly created “Fonts” folder:
Step 2: Importing vector icons into Xcode To access the icon files, navigate to node_modules > react-native-vector-icons > Fonts. Open the Fonts folder, and you will find a collection of .ttf icon files, as illustrated below:
To import these font icons into Xcode, open the project and right-click on the “Fonts” folder we established. Select “Add files to MainApp,” where “MainApp” is the name of my application in this context. A modal window will appear, allowing you to choose the icons in the “Fonts” folder we mentioned earlier.
Ensure that you check both “Copy items if needed” and “Create folder reference,” select all the font files, and then click the “Add” button.
Step 3: Adding fonts to info.plist
The next step is navigating to the info.plist
file and copying and pasting the provided code from the GitHub page by vector icons developers. Please click here to access the code.
Verify that the files selected through Xcode match the icon names within the <string>
tags. Below is the code copied into my info.plist
file:
After inserting the code into your info.plist
, go to Xcode and click on the “Info” tab in the left sidebar below the “Fonts” folder we initially created. This will allow us to complete the iOS configuration.
The final configuration involves three steps. First, click the “+” icon, as indicated by step 1 in the image below. An input field will appear; type “font,” and an auto-complete option for “Fonts provided by application” will appear (step 2). Click on it, as shown in the image below, to include the icon names, as indicated in the image below where Step 3 is written.
Ensure you include the added fonts in the info.plist
file for each item, as depicted in the image where step 3 is illustrated. Be sure to fill in all the empty spaces in the second column of the image corresponding to step 3.
Step 4: Updating Pod Finally, after completing the step-by-step guides above, in your project terminal, navigate to the “ios” directory and execute the following code below:
pod update
#or
pod install
This action will update the iOS pod and apply the icons to your project. Subsequently, go to Xcode and click the run button to initiate the application.
This concludes the iOS configuration. If you encounter any difficulties with this section, please visit the official GitHub page of React Native Vector Icons for reference.
Popular Vector Icon Libraries
In this segment, we’ll be incorporating various vector icons into our project, including FontAwesome, AntDesign, MaterialCommunityIcons, and Feather. These are popular icons commonly used in React Native, and we’ll explore them in this section. To search for an icon by name, you can easily navigate here to find the icon of your choice.
To utilize vector icons in your React Native project, all you need to do is import the icon library, as demonstrated below:
import FontAwesome5Icon from 'react-native-vector-icons/FontAwesome5Icon';
The icons component accepts props such as color, size, and the icon’s name, as depicted below:
<FontAwesome5Icon name="music" size={80} color={'white'} />
In the subsequent subsections, we’ll employ this format to work with the mentioned vector icons, as discussed earlier.
FontAwesome
FontAwesome stands out as a widely embraced collection of icons, providing an extensive array of graphical elements suitable for various purposes. Whether you require icons for typical actions, social media representations, or specific conceptualizations, FontAwesome offers a versatile solution.
Below is a complete code snippet along with its output, showcasing the effective utilization of FontAwesome in your projects:
import { StyleSheet, View } from "react-native";
import React from "react";
import FontAwesome from "react-native-vector-icons/FontAwesome";
const PlayGround = () => {
return (
<View style={styles.body}>
<FontAwesome name="music" size={80} color={"white"} />
<FontAwesome name="music" size={50} color={"white"} />
<FontAwesome name="music" size={30} color={"white"} />
<FontAwesome name="music" size={20} color={"white"} />
<FontAwesome name="glass" size={50} color={"white"} />
</View>
);
};
export default PlayGround;
const styles = StyleSheet.create({
body: {
flex: 1,
backgroundColor: "black",
padding: 20,
justifyContent: "center",
alignItems: "center",
},
});
Output:
AntDesign
AntDesign icons are known for being straightforward and consistent. They are part of the Ant Design language and are great for making modern and stylish user interfaces. You can easily use them in your project with the following code snippet:
import { StyleSheet, View } from "react-native";
import React from "react";
import AntDesign from "react-native-vector-icons/AntDesign";
const PlayGround = () => {
return (
<View style={styles.body}>
<AntDesign name="backward" size={80} color={"white"} />
<AntDesign name="pluscircle" size={80} color={"white"} />
<AntDesign name="forward" size={80} color={"white"} />
</View>
);
};
export default PlayGround;
const styles = StyleSheet.create({
body: {
flex: 1,
backgroundColor: "black",
padding: 20,
justifyContent: "center",
flexDirection: "row",
alignItems: "center",
},
});
This code shows an easy way to use AntDesign icons in your app, and below is the output in my IOS simulator device:
MaterialCommunityIcons
MaterialCommunityIcons are icons based on the material design guidelines. They offer a wide range of icons that align with the material design language. These icons contribute to a unified and easily recognizable visual style, making them an excellent option for applications striving for a consistent material design experience.
Below is the entire code for integrating MaterialCommunityIcons into your React Native project:
import { StyleSheet, View } from "react-native";
import React from "react";
import MaterialCommunityIcons from "react-native-vector-icons/MaterialCommunityIcons";
const PlayGround = () => {
return (
<View style={styles.body}>
<MaterialCommunityIcons
name="account-cowboy-hat"
size={80}
color={"white"}
/>
<MaterialCommunityIcons name="account-circle" size={80} color={"white"} />
<MaterialCommunityIcons
name="airballoon-outline"
size={80}
color={"white"}
/>
<MaterialCommunityIcons name="airplane" size={80} color={"white"} />
</View>
);
};
export default PlayGround;
const styles = StyleSheet.create({
body: {
flex: 1,
backgroundColor: "black",
padding: 20,
justifyContent: "center",
flexDirection: "row",
alignItems: "center",
},
});
Output:
Feather
Feather is a set of outlined icons recognized for its visually appealing and lightweight design. These icons are ideal for projects seeking a sleek and modern appearance. Feather icons strike a balance between simplicity and clarity, making them a popular choice for various mobile applications.
Here is a code example for incorporating Feather icons into your project:
import { StyleSheet, View } from "react-native";
import React from "react";
import Feather from "react-native-vector-icons/Feather";
const PlayGround = () => {
return (
<View style={styles.body}>
<Feather name="arrow-up" size={80} color={"white"} />
<Feather name="activity" size={80} color={"white"} />
<Feather name="at-sign" size={80} color={"white"} />
<Feather name="align-justify" size={80} color={"white"} />
</View>
);
};
export default PlayGround;
const styles = StyleSheet.create({
body: {
flex: 1,
backgroundColor: "black",
padding: 20,
justifyContent: "center",
flexDirection: "row",
alignItems: "center",
},
});
Output:
Best Practices and Tips
In this section, we’ll explore the recommended practices and tips for using React Native Vector Icons efficiently in your project.
Optimizing Icon Usage:
- Include only essential icons to maintain a lightweight app.
- Choose the appropriate icon set that aligns with your app’s design language.
- Group similar actions under one icon when possible to prevent icon overload.
Choosing Appropriate Icon Sizes and Colors:
- Select icon sizes that remain legible on various screen resolutions.
- Ensure a clear contrast between the icon color and the background.
- Maintain consistent color schemes for icons associated with similar actions or categories.
Maintaining Consistent Icon Styles:
- Adhere to a single icon style across your app for a unified appearance.
- If employing multiple icon sets, do so intentionally while preserving a consistent theme.
- Regularly review and update icons to ensure they stay in line with any design changes.
Conclusion
I hope you enjoyed reading the article. We explored the vital aspects of using React Native Vector Icons, delving into their significance and practical applications. We also discussed the installation steps for both Android and IOS platforms. The insights gained from this article now equip you to effortlessly integrate icons into your React Native project, enhancing your proficiency as a React Native developer.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.