Implementing pull-to-refresh with JavaScript
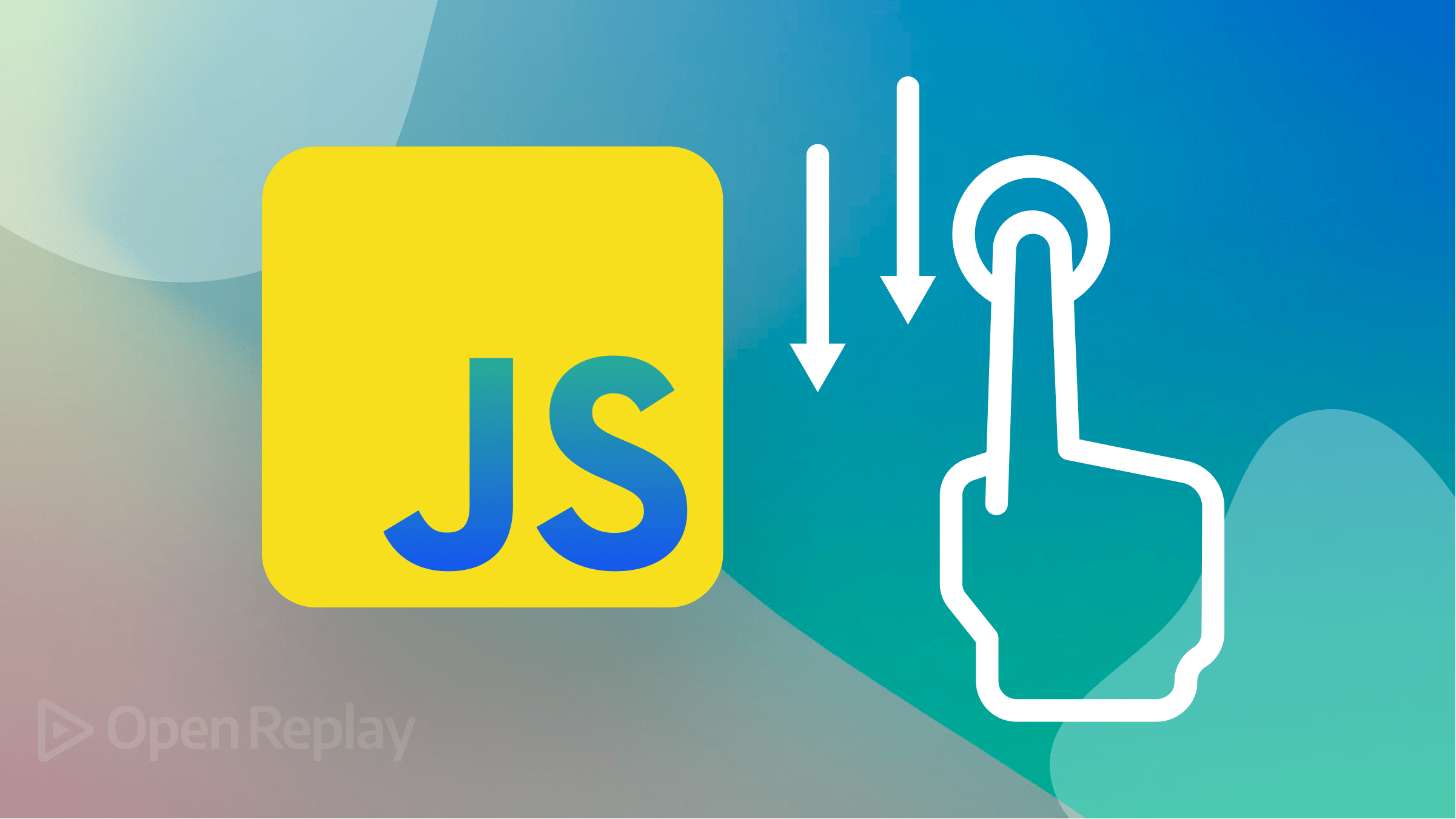
Pull-to-refresh is a widespread interaction pattern that allows users to refresh content in an application by pulling it down on the screen. This interaction pattern is widely used in mobile applications but can also be applied to web applications using JavaScript, and this article will show you how.
This article will explore how to implement pull-to-refresh using vanilla JavaScript and CSS overscroll behavior.
Pull-to-refresh Gesture
Imagine you’re a bartender with a magical tap that pours an endless stream of refreshing drinks. You’re serving customers left and right, and everyone is happy. But suddenly, the tap starts to slow down, and the drinks aren’t as cold and refreshing as they used to be.
So what do you do? You don’t want your customers to be disappointed, right? You roll up your sleeves and give the tap a quick pull, just like you would with a lever or handle. And voila! The tap is now flowing smoothly again, and the drinks are as cold and refreshing as ever.
That’s essentially what pull-to-refresh does in mobile and web applications. For example, when you’re scrolling through your Twitter feed and notice that the content isn’t as interesting or up-to-date as you’d like, you can give the screen a quick pull with your finger or cursor, just like pulling a lever. This triggers a refresh of the content, and voila! Your feed is now updated with the latest tweets, just like the bartender’s tap was refreshed with cold, refreshing drinks.
So, pull-to-refresh is a handy interaction pattern that helps users stay up-to-date with the latest content and ensures they don’t miss out on anything interesting. Like the bartender’s tap, it’s a simple but effective way to keep things flowing smoothly and everyone happy.
The benefits of pull-to-refresh are primarily related to its ease of use and intuitive nature. Instead of having to navigate to a separate refresh button or menu, users can simply swipe down to trigger a refresh, making the process quicker and more convenient. It also provides a visual cue that the content is being updated, which can help increase user engagement and satisfaction.
In a typical pull-to-refresh interaction, the user swipes downward on the screen or page with their finger or cursor. As they pull, a visual cue, such as an arrow or spinning wheel, indicates that the content is being refreshed. The content is updated once the user releases their finger or cursor, and the visual cue disappears.
The implementation of pull-to-refresh can vary between mobile and web applications. In mobile applications, the interaction typically involves pulling down a scrollable list or feed of content. In contrast, in web applications, it can be used to refresh an entire page or a specific section of a page. Additionally, some mobile applications may use variations on the pull-to-refresh interaction, such as swipe-to-refresh or drag-to-refresh.
OverScroll Behaviour
Overscroll behavior is a property that allows you to control how a webpage or app behaves when the user scrolls beyond the end of its content. The overscroll behavior can be set to auto
, contain
, none
, or inherit
.
When set to auto
, the overscroll behavior will be determined by the user’s device and the type of content being displayed. For example, on a touch-enabled device, the overscroll behavior might trigger a pull-to-refresh action when the user pulls down on the page beyond its top boundary, and this is what will be used in the course of this article.
Implementing Pull-to-refresh Gesture
This is an easy task to accomplish. Your first task should be setting up HTML, which should look like the code below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="pull-to-refresh">
<span>Loading more tweets</span>
</div>
<div class="tweets">
<p>PULL DOWN FOR MORE TWEETS</p>
</div>
</body>
<script src="app.js"></script>
</html>
In the code above, the <body>
element contains the page’s visible content. It has two child div
elements, one with the class pull-to-refresh
and one with the class tweets
.
The first div
has a child span
element that displays the text “Loading more tweets”. This UI component is used to refresh the content on the page by pulling it down with a gesture. The document ends with a reference to an external JavaScript file with the script
element.
The Javascript file of cause holds the implementation of this gesture. The code below should be able to get this going:
const pullToRefresh = document.querySelector('.pull-to-refresh');
let touchstartY = 0;
document.addEventListener('touchstart', e => {
touchstartY = e.touches[0].clientY;
});
document.addEventListener('touchmove', e => {
const touchY = e.touches[0].clientY;
const touchDiff = touchY - touchstartY;
if (touchDiff > 0 && window.scrollY === 0) {
pullToRefresh.classList.add('visible');
e.preventDefault();
}
});
document.addEventListener('touchend', e => {
if (pullToRefresh.classList.contains('visible')) {
pullToRefresh.classList.remove('visible');
location.reload();
}
});
The code above listens for touch events on the webpage, and when a user starts to drag their finger downwards on the screen (i.e., during the touchmove
event), the code checks if the user has pulled down enough (by comparing the current touch position with the initial touch position).
If the user has pulled down enough and they have reached the top of the page (i.e., window.scrollY === 0
), the code displays a pull-to-refresh
element on the page by adding the “visible” CSS
class to it. When the user lifts their finger off the screen (i.e., during thetouchend
event), the code checks if the pull-to-refresh element is currently visible. If it is, the code removes the “visible” CSS
class from the element and triggers a page reload (i.e., location.reload()
) to refresh the content.
Before we run this code in our browser, let’s add styles to it. For our styles, we should have the following:
body {
overscroll-behavior-y: auto;
}
.pull-to-refresh {
position: fixed;
top: -50px;
width: 100%;
height: 60px;
display: flex;
justify-content: center;
align-items: center;
transition: top 0.7s ease-in-out;
}
.pull-to-refresh.visible {
top: 0;
}
.tweets {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Spinner
A spinner serves a crucial role in enhancing the user interface experience by providing visual feedback for certain actions. Typically, users associate spinners with loading or reloading, so incorporating a spinner is important for a complete pull-to-refresh gesture. Fortunately, integrating a spinner using Bootstrap is simple and straightforward. We can easily activate the spinner and improve the user experience by linking our app with Bootstrap.
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css"
/>
</head>
<body>
<div class="pull-to-refresh">
<div class="spinner-border"></div>
</div>
When we run these in the browser, we should have what resembles the GIF below:
Looking at the GIF illustration above, it appears that the GIF illustration does not display any refreshing behavior. To address this, a function will be added to change the background color with each refresh. To implement this, an id
attribute will be added to the body element, specifically as id="color"
. The JavaScript file will then include the necessary logic to achieve this background color changing functionality.:
const color = document.getElementById("color")
let touchstartY = 0;
let colors=['red', 'blue', 'green', 'orange', 'black' ]
window.addEventListener("load", function() {
setTimeout(function() {
color.style.backgroundColor = colors[Math.floor(Math.random() * colors.length)];;
}, 100);
});
Now we have this:
Just like this, we have been able to implement a pull-to-refresh gesture using Javascript and HTML.
Conclusion
In conclusion, pull-to-refresh is a user interface feature that allows users to update web content by pulling down and releasing the screen. It has become a popular and widely adopted feature in mobile applications and has gained traction in web applications. Some key benefits of pull-to-refresh in web applications include its simplicity, ease of use, and ability to provide real-time updates without page refreshes.
It can also improve the overall user experience by reducing the time it takes for users to access updated content. There are many potential use cases for pull-to-refresh in web applications, including social media feeds, news sites, and e-commerce websites.
In addition to these use cases, there are opportunities for pull-to-refresh to be used in new and innovative ways. As web technology continues to evolve, many possibilities exist for enhancing pull-to-refresh in web applications.
Some of these possibilities include incorporating additional features, such as pull-to-load or pull-to-search, or improving the overall performance and speed of the feature.
Pull-to-refresh has proven to be a valuable and versatile feature in web applications, and its continued use and development will undoubtedly bring new benefits and opportunities for developers and users alike.