Integrating Custom Fonts in React Native for iOS and Android Platforms
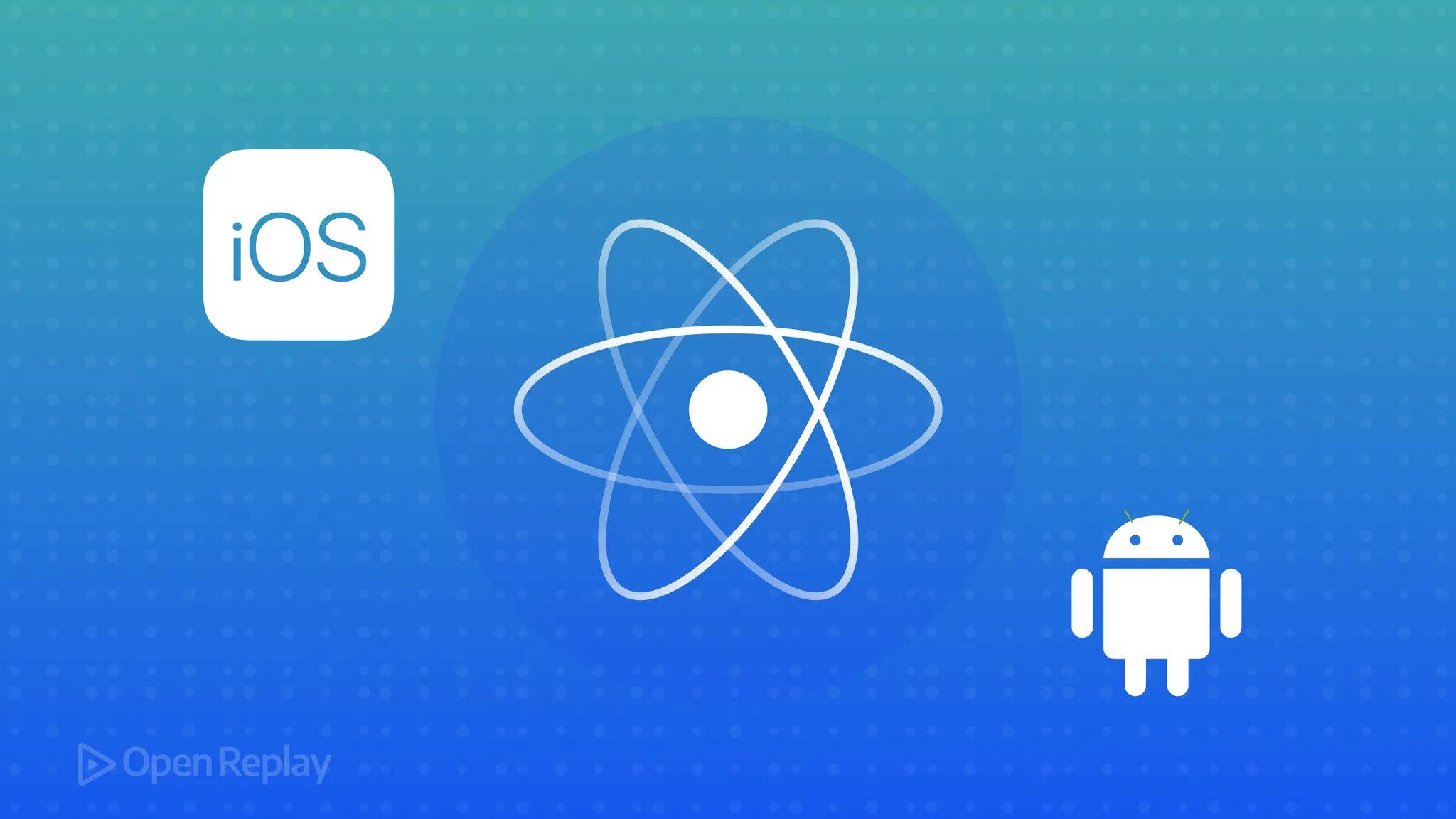
Are you looking to add a unique touch to your React Native app by using custom fonts? In this article, we’ll guide you through the process of integrating custom fonts in your React Native projects for both iOS and Android platforms. By the end, you’ll have a clear understanding of how to use custom fonts effectively and avoid common pitfalls.
Key Takeaways
- React Native supports custom fonts on both iOS and Android platforms.
- For React Native CLI projects, add font files to the
assets/fonts
directory, configurereact-native.config.js
, and link the fonts. - For Expo projects, use the
expo-font
package and theuseFonts
hook to load custom fonts. - Be mindful of common pitfalls like font loading time, mismatched names, incorrect paths, and unsupported formats.
Why Use Custom Fonts in React Native?
Using custom fonts in your React Native app can:
- Enhance the visual appeal and user experience
- Create a unique brand identity
- Improve readability and accessibility
React Native provides built-in support for custom fonts, making it easy to integrate them into your projects.
Adding Custom Fonts to a React Native CLI Project
Step 1: Download and Add Font Files
- Download the desired font files (TTF or OTF) from sources like Google Fonts or Font Squirrel.
- Create an
assets/fonts
directory in your project’s root folder. - Place the font files in the
assets/fonts
directory.
Step 2: Configure react-native.config.js
- Create a
react-native.config.js
file in your project’s root directory (if it doesn’t exist). - Add the following code to the file:
module.exports = {
project: {
ios: {},
android: {},
},
assets: ['./assets/fonts'],
}
Step 3: Link the Fonts
Run the following command in your terminal to link the fonts:
npx react-native link
Step 4: Use the Custom Fonts
In your React Native components, use the fontFamily
style property to apply the custom fonts:
<Text style={{ fontFamily: 'CustomFontName', fontSize: 18 }}>
Hello, custom font!
</Text>
Using Custom Fonts with Expo
Step 1: Install expo-font
Run the following command to install the expo-font
package:
expo install expo-font
Step 2: Load the Fonts
Use the useFonts
hook from expo-font
to load the custom fonts:
import { useFonts } from 'expo-font'
export default function App() {
const [fontsLoaded] = useFonts({
'CustomFont': require('./assets/fonts/CustomFont.ttf'),
})
if (!fontsLoaded) {
return null
}
return (
<View style={styles.container}>
<Text style={{ fontFamily: 'CustomFont', fontSize: 18 }}>
Hello, custom font!
</Text>
</View>
)
}
Common Pitfalls and Solutions
Font Loading Time
Problem: The UI renders before the fonts are loaded, causing fallback fonts to appear.
Solution: Use conditional rendering to ensure the app only renders after the fonts are loaded.
Mismatched Font Family Names
Problem: Inconsistent font family names between the font file and the code.
Solution: Ensure the font family names match exactly between the font file and the code.
Incorrect Font Path
Problem: Using the wrong path for the font file.
Solution: Double-check the file structure and ensure the paths match the exact location of the font files.
Using Unsupported Font Formats
Problem: Using font formats not supported by the target platform.
Solution: Verify that the font format (TTF, OTF) is supported by the target platform (iOS, Android).
Conclusion
Integrating custom fonts in your React Native app is a great way to enhance its visual appeal and create a unique brand identity. By following the steps outlined in this article and being aware of common pitfalls, you can successfully use custom fonts in your React Native projects for both iOS and Android platforms.
FAQs
Yes, you can use multiple custom fonts in a single project. Just add each font file to the `assets/fonts` directory and reference them correctly in your code.
To optimize font loading times, consider using font loading strategies like subsetting or asynchronous loading. Also, minimize the number of custom fonts used in your project.
Yes, libraries like `react-native-google-fonts` and `expo-font` can simplify the process of integrating custom fonts in your React Native projects.