Node.jsプロジェクトをTypeScriptとExpressでセットアップする方法
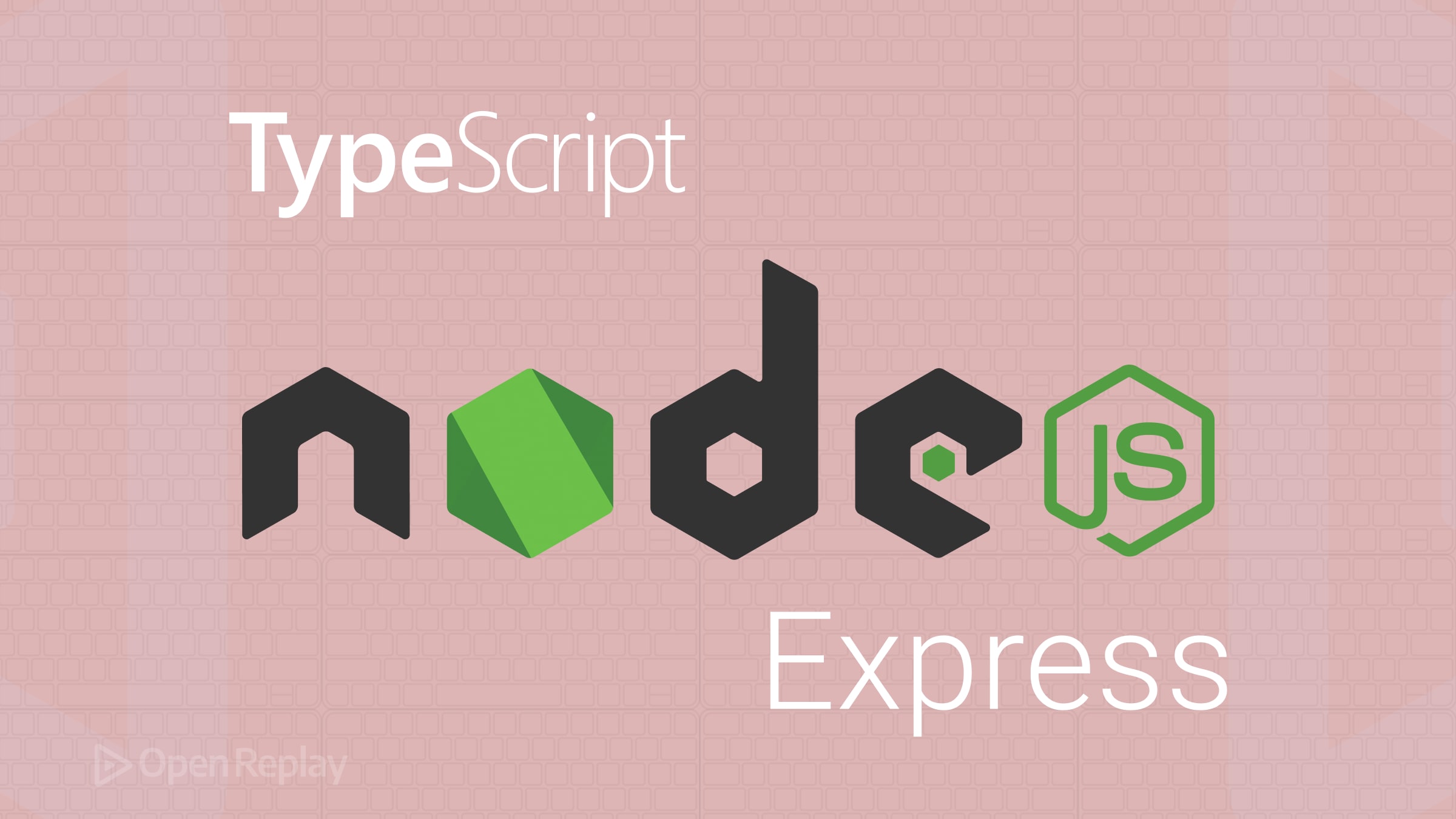
Node.jsプロジェクトをTypeScriptとExpressでセットアップすることで、アプリケーションに構造、スケーラビリティ、明確さを与えることができます。このガイドでは、Node.jsプロジェクトの初期化、TypeScript、Expressの統合、そして必須の開発ツールの追加について、ステップバイステップで説明します。
重要ポイント
- TypeScriptとExpressを使用した堅牢なNode.jsプロジェクトを迅速にセットアップします。
- ESLint、Prettier、Jest、nodemonなどの必須ツールを統合してスムーズな開発を実現します。
ステップ1: Node.jsプロジェクトの初期化
プロジェクトフォルダを作成し、npmを初期化します:
mkdir my-node-project
cd my-node-project
npm init -y
これによりpackage.json
が生成されます。次に、nodeモジュールとビルドファイルを除外する.gitignore
を作成します:
node_modules
dist
ステップ2: TypeScriptの追加
TypeScriptと関連する依存関係をインストールします:
npm install --save-dev typescript ts-node @types/node
以下のコマンドでTypeScript設定を作成します:
npx tsc --init
tsconfig.json
を以下のように調整します:
{
"compilerOptions": {
"target": "ES2016",
"module": "commonjs",
"rootDir": "./src",
"outDir": "./dist",
"esModuleInterop": true,
"strict": true,
"skipLibCheck": true
}
}
コード用のsrc
フォルダを作成します:
mkdir src
ステップ3: Expressのセットアップ
Expressとその型定義をインストールします:
npm install express
npm install --save-dev @types/express
src/index.ts
に基本的なサーバーを作成します:
import express, { Application, Request, Response } from 'express';
const app: Application = express();
const PORT = 3000;
app.get('/', (req: Request, res: Response) => {
res.send('Hello, TypeScript + Express!');
});
app.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}`);
});
以下のコマンドで実行します:
npx ts-node src/index.ts
ステップ4: ESLintとPretterのセットアップ
ESLint、Prettier、およびプラグインをインストールします:
npm install --save-dev eslint prettier @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-plugin-prettier eslint-config-prettier
.eslintrc.json
でESLintを設定します:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint", "prettier"],
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:prettier/recommended"
],
"env": { "node": true, "es6": true, "jest": true }
}
.prettierrc.json
を作成します:
{
"semi": true,
"singleQuote": true,
"trailingComma": "all"
}
package.json
のスクリプトを更新します:
"scripts": {
"lint": "eslint 'src/**/*.{ts,js}'",
"format": "prettier --write ."
}
ステップ5: テスト用のJest
JestとTypeScriptサポートを追加します:
npm install --save-dev jest ts-jest @types/jest
npx ts-jest config:init
簡単なテストsrc/__tests__/sum.test.ts
を作成します:
import { sum } from '../sum';
test('sum works', () => {
expect(sum(1, 2)).toBe(3);
});
対応するモジュールも作成します:
export function sum(a: number, b: number): number {
return a + b;
}
package.json
を更新します:
"scripts": {
"test": "jest --watch"
}
ステップ6: 開発用のNodemon
nodemonをインストールして設定します:
npm install --save-dev nodemon
nodemon.json
を追加します:
{
"watch": ["src"],
"ext": "ts,json",
"ignore": ["src/**/*.test.ts"],
"exec": "ts-node ./src/index.ts"
}
package.json
に開発環境用のスクリプトを追加します:
"scripts": {
"dev": "nodemon"
}
開発中にサーバーを自動リロードするにはnpm run dev
を実行します。
ベストプラクティス
- 別々のフォルダを使用します(コード用の
src
、ビルド出力用のdist
)。 - ソースファイルのみをコミットし、コンパイルされたコード(
dist
)やnodeモジュールはコミットしないでください。 npm run lint
とnpm run format
を頻繁に実行してください。- デプロイ前にコードをコンパイル(
npm run build
)し、npm start
で起動してください。
よくある質問
はい。npmコマンドを同等のyarnやpnpmコマンドに置き換えるだけです。
いいえ。本番環境では常に`tsc`でプロジェクトをコンパイルし、`dist`フォルダからJavaScriptを実行してください。
Jestはオプションですが、信頼性とコード品質を大幅に向上させるため、シンプルなプロジェクトでも価値があります。