Learn how Mapping Works In VueX
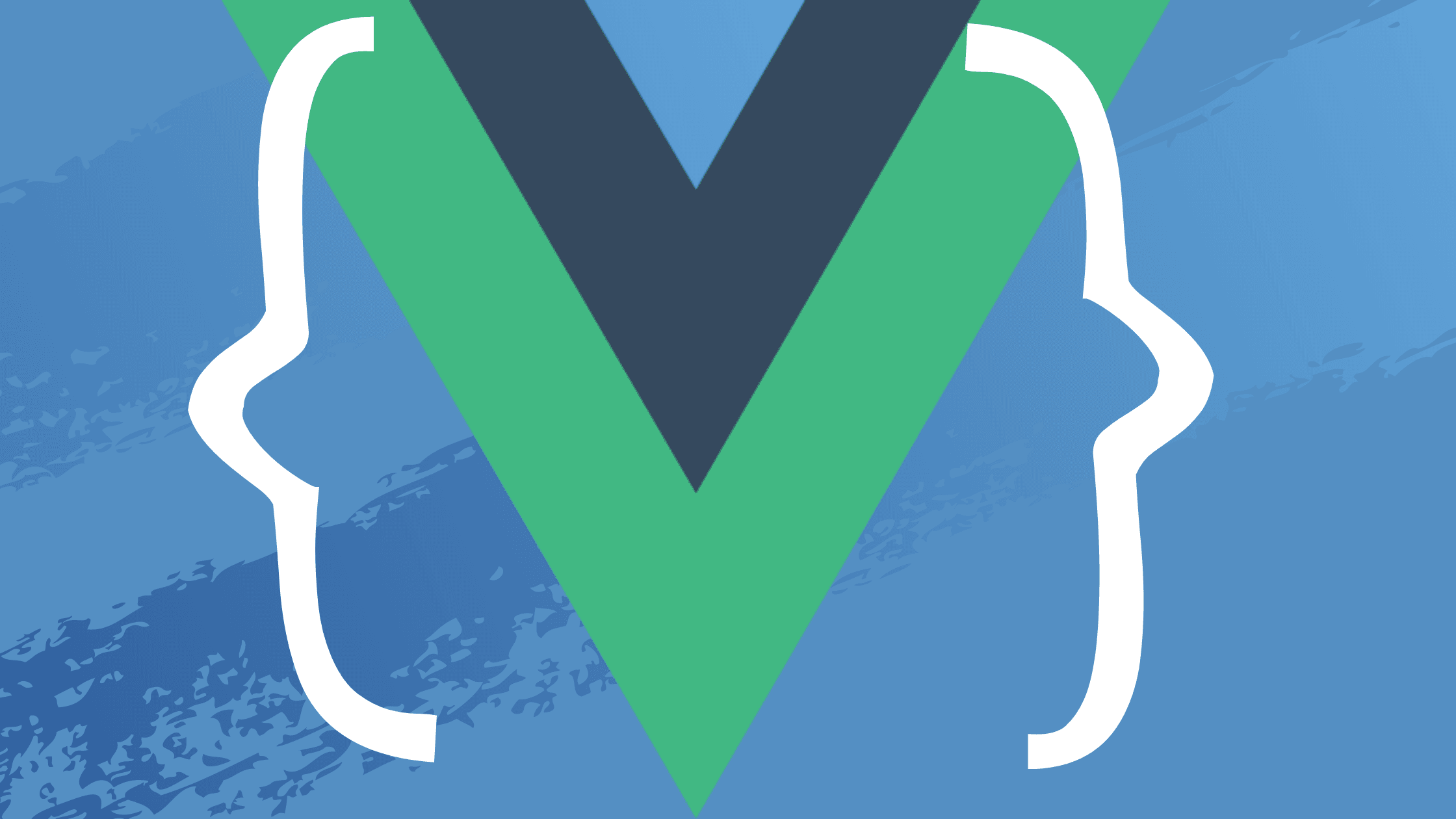
Managing state in Vue applications can be difficult especially when there are so many moving parts in your application. Vuex a state management library for Vue applications helps simplify that process but can also get cumbersome and bloat your codebase which brings us to mapping.
Mapping in Vuex helps you make use of multiple store properties (state, getters, actions, and mutations) in your Vue components. In this article we will be looking at how to use Mapping to map data from a Vuex store to Vue components.
This article assumes you have prior knowledge of Vue and Vuex. If not, I suggest you read this article I wrote to become more familiar with the primary concepts of Vuex.
Why Mapping?
As I already explained Vuex allows us to manage state in Vue applications and provides a central store to keep properties such as state, mutations, getters, and actions. Below is an example of a typical Vuex store
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export const store = new Vuex.Store({
state: {
products: [],
},
mutations: {
setProduct() {},
},
getters: {
getProduct() {},
},
actions: {
FETCH_PRODUCTS() {}
},
});
To access the items in state in our Vue components we make use of the computed
property by doing this:
computed: {
getProducts(){
return this.$store.state.products
}
}
While this method for handling data seems efficient for most applications It has a drawback: when the application becomes larger (and because we have a centralized store) the code may become bloated when we introduce more data to our application. Check out the following example:
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export const store = new Vuex.Store({
state: {
products: [],
cart: [],
notifications: []
product: null
},
mutations: {
setProduct() {},
setCart() {},
setNotificationStatus(id) {}
},
getters: {
getProduct() {}
getCartTotalPrice() {},
getCartItemTotal() {}
getNotifications() {}
},
actions: {
SET_PRODUCT() {},
SET_CART() {},
FETCH_PRODUCTS(){},
SET_NOTIFICATION_STATUS() {}
},
});
If we need all this data in a component our computed
property becomes bloated as well:
computed: {
getProducts(){
. return this.$store.state.products
}
getCart(){
return this.$store.state.cart
}
getProduct(){
return this.$store.state.product
}
getNotifications(){
return this.$store.state.notifications
}
getCartTotalPrice(){
return this.$store.getters.getCartTotalPrice
}
getCartItemTotal(){
return this.$store.getters.getCartItemTotal
}
},
methods: {
setProduct: function() {
this.$store.commit('setProduct');
},
setCart: function() {
this.$store.commit('setCart');
},
setNotificationStatus: function(notificaionId) {
this.$store.commit('setProduct', notificationId);
}
fetchProducts: function() {
this.$store.dispatch('FETCH_PRODUCTS');
}
}
Mapping allows us to structure the code better by binding the store properties (state, getters, mutations, and actions) to computed properties so we can use these properties directly in the state and save time.
In the next sections, we will look at how to use mapping to write less verbose code.
Mapping State
The state is a representation of our application at any point in time and contains data like objects, arrays, strings, etc. Vuex uses a single state tree to keep all our application-level states.
The question then becomes: What happens when we have “too much state” in our Vue components. Vuex provides a helper function called mapState
to solve this problem. It is used for mapping state
properties in the store to computed
properties in our components.
import { mapState } from 'vuex'
export default{
computed: {
...mapState([
'products','product','cart','notifications'
])
}
}
The mapState
helper function returns an object which contains the state in the store. This state can then be accessed in components by doing this {{products}}
.
We can also provide aliases for the state returned by the mapState
function by returning an object instead of an array like the example below
import {mapState} from 'vuex'
export default{
computed: {
...mapState({
// using an arrow function
productList: (state) => state.products,
// passing the string value is the same as state => state.cart
cartList: 'cart'
})
}
}
The snippet’s code is cleaner and more readable than creating individual methods like the example below to return state properties:
computed: {
getProducts(){
return this.$store.state.products
}
getCart(){
return this.$store.state.cart
}
getProduct(){
return this.$store.state.product
}
getNotifications(){
return this.$store.state.notifications
}
},
Notice that the mapState
helper should only be used when you have lots of data in the state. This is to avoid memory and performance issues in the long run.
Mapping Getters
Mapping getters is similar to mapping state. Getters provide a way of getting a derived computed state from the store e.g a function that returns products based on popularity. Vuex also provides a mapGetters
helper function to map getters to local computed properties. The example below shows how to use the mapGetters
function:
import {mapGetters} from 'vuex'
export default{
computed: {
...mapGetters([
'getProduct' ,
'getCartTotalPrice',
'getCartItemTotal',
'getNotifications'
])
}
}
The mapGetters
function returns an object of getters from the store. You can also alias the getters by using an object instead of an array
import {mapGetters} from 'vuex'
export default{
computed: {
...mapGetters({
singleProduct: 'getProduct' ,
cartTotalPrice: 'getCartTotalPrice',
cartTotalItems: 'getCartItemTotal',
notifications: 'getNotifications'
})
}
}
Mapping Mutations
Mutations are what differentiate Vuex from other popular state management libraries as they help in changing state in the store by committing a mutation as opposed to using reducer functions. The example below shows how to commit a mutation
methods: {
setProduct: function(todo) {
this.$store.commit('setProduct');
},
setCart: function(todo) {
this.$store.commit('setCart');
},
setNotificationStatus: function(notificationId) {
this.$store.commit('setProduct', notificationId);
}
}
Mutations can also be mapped using the mapMutations
helper function but unlike states and getters mutations are mapped to methods
and not computed
properties. The example below shows how to map a mutation
import { mapMutations } from 'vuex';
export default {
methods: {
...mapMutations([
'setProduct', // maps this.setProduct to this.$store.commit('setProduct')
'setCart', // maps this.setCart to this.$store.commit('setCart')
// this accepts a payload
'setNotificationStatus', // maps this.setCart to this.$store.commit('setNotificationStatus', notificationId),
]),
},
};
You can also alias the mutations by passing an object instead of an array
import { mapMutations } from 'vuex';
export default {
methods: {
...mapMutations({
addProduct: 'setProduct',
addProductToCart: 'setCart',
markNotification: 'setNotificationStatus', // this accepts a payload,
}),
},
};
Mapping Actions
Mapping actions are very similar to mutations as actions are also mapped to the methods
property and not the computed
property. The major difference between actions and mutations is actions commit mutations and are asynchronous. The example below shows how it is used
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions([
'SET_PRODUCT', // maps this.SET_PRODUCT() to this.$store.dispatch('SET_PRODUCT')
'SET_CART', // maps this.SET_CART() to this.$store.dispatch('SET_CART')
'FETCH_PRODUCTS', // maps this.FETCH_PRODUCTS() to this.$store.dispatch('FETCH_PRODUCTS'),
]),
},
};
You can also alias the actions by passing an object instead of an array to the mapActions
function
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions({
setProduct: 'SET_PRODUCT',
setCart: 'SET_CART',
fetchProducts: 'FETCH_PRODUCTS',
}),
},
};
Mapped mutations and actions can be used in templates the same way other mutations and actions are used. The example below shows how to use an action in a template
<button v-on:click="setCart">Add to Cart</button>
Conclusion
In this article, we looked at the concept of mapping in Vuex and how it can help you structure your code better. Something to note is that Mapping is beneficial when a component needs to use multiple store properties and should only be used for that.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.