localStorage VS sessionStorage: A Comprehensive Comparison
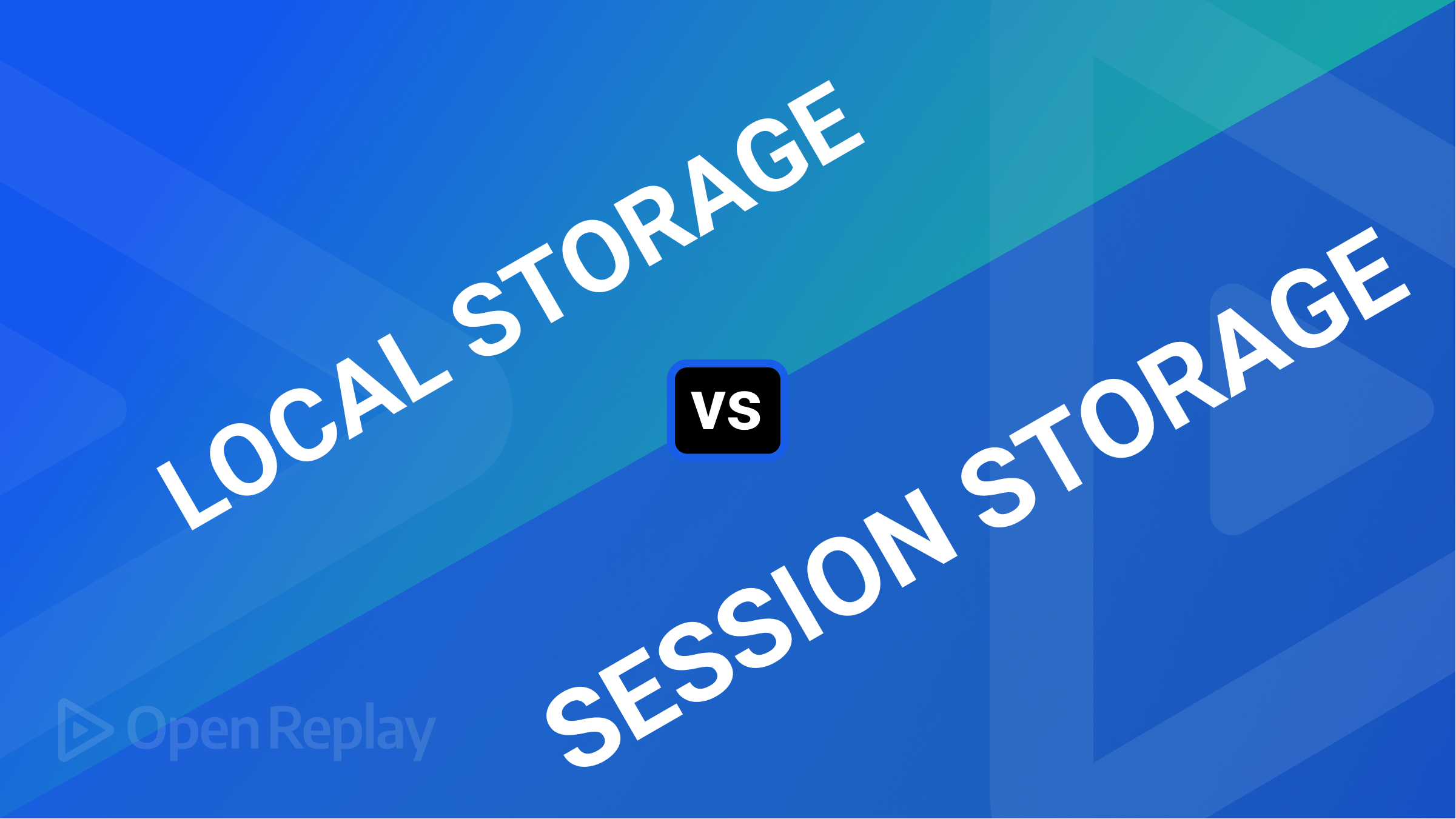
Web applications have become integral to our daily lives. As the amount of data and functionality these applications provide increases, so does the need for efficient and reliable storage solutions. localStorage and sessionStorage are two such solutions, provided by the web storage API, that allow developers to store key/value pairs locally within web browsers.
While localStorage
and sessionStorage
share similarities, they also have some differences that make them suitable for different use cases. In this article, we’ll compare their features and explore when and why you should use one over the other.
Checking Web Storage Compatibility
Before we can utilize localStorage
or sessionStorage
, we must ensure that the browser supports it or that the user has enabled it. We can do this with the code below.
if (typeof Storage !== undefined) {
alert("Web storage supported");
} else {
alert("Your browser does not support the web storage API");
}
The code above checks to see if the Storage type is defined in the browser, after which we can now use localStorage
and sessionStorage
within the first if-block.
Utilizing the localStorage and sessionStorage API
When using both the local
and session storage
APIs, they both use the same methods. Below are some effective methods for local
and session storage
.
Set and retrieve items
Both localStorage
and sessionStorage
offer two syntax options for setting items, as demonstrated in the code below.
localStorage.setItem(identifier, value); // Set localStorage data
sessionStorage.setItem(identifier, value); // Set sessionStorage data
// OR
localStorage.identifier = value; // Set localStorage data
sessionStorage.identifier = value; // Set sessionStorage data
Both options have two parameters: an identifier
(a preferred name) and a value
(string
, integer
, or boolean
). For example, we could store a user’s name with localStorage
or sessionStorage
like below:
localStorage.name = "Oluwole Opeyemi";
// OR
sessionStorage.userCart = ["Shoe", "Bag", "Belt"];
We can then access these values anywhere within the same script with the following code
localStorage.getItem("name");
sessionStorage.getItem("userCart");
// OR
sessionStorage.name;
// Output "Oluwole Opeyemi"
Remove item(s)
To remove an item stored in localStorage
or sessionStorage
, we can use the removeItem()
method as shown below.
localStorage.removeItem(name);
// OR
sessionStorage.removeItem(userCart);
Executing the code above, the previously stored name
and userCart
are now erased from the browser’s storage. In plain terms, the key/value
pair is removed from the browser’s storage.
Furthermore, to erase all the data stored in the web storage at once, we could utilize the clear()
method:
localStorage.clear();
// OR
sessionStorage.clear();
This method provides a way to remove all data stored in localStorage
or sessionStorage
at once, effectively resetting them to their original state.
Comparing localStorage and sessionStorage
localStorage
can be useful in many situations, such as saving user preferences or storing small amounts of data that must persist between website visits. However, it may not be the best choice for more extensive data storage needs or for storing sensitive information, as it is accessible to any script of the same origin and can be vulnerable to malicious attacks.
In practical terms, here are some situations where using localStorage
could be helpful:
- Remembering user preferences such as the color scheme of a website
- Storing small amounts of data that need to persist between visits, such as a shopping cart for an e-commerce site
- Saving user progress in a game or application.
sessionStorage
helps store temporary data that does not need to persist between website visits. For instance, it can store information such as the current state of a form or the page of a paginated list.
In practical terms, here are some situations where using sessionStorage
could be helpful:
- Storing temporary data for a single website session, such as the items in a user’s shopping cart
- Maintaining the state of a form or the current page of a paginated list, even if the user closes the page
- Storing temporary data that is not sensitive, such as user-specific preferences for a single session.
What is sessionStorage?
sessionStorage
is a web storage option for storing key-value pairs
data within a browser for a single session/tab. The data is stored per origin and accessible to all scripts from that origin, and it is deleted when the tab/window closes.
Set and retrieve items
As demonstrated in the code below, sessionStorage
offers two syntax options for setting items.
sessionStorage.setItem(identifier, value);
// OR
sessionStorage.identifier = value;
Both options have two parameters: an identifier
(a preferred name) and a value
(string
, integer
, or a boolean
). For example, we could store a user’s name with sessionStorage like the below:
sessionStorage.name = "Oluwole Opeyemi";
We can then access the value anywhere within the same script with the following code
sessionStorage.getItem("name");
// OR
sessionStorage.name;
// Outputs "Oluwole Opeyemi"
Remove item(s)
To remove an item stored in sessionStorage
, we can use the removeItem()
method as shown below.
sessionStorage.removeItem(name);
Executing the code above, the previously stored name with the’Oluwole Opeyemiis now erased from the browser's storage. In plain terms, the key/value pair is removed from the browser's storage. Furthermore, to erase all the data stored in a session at once, we could utilize the
clear()` method:
sessionStorage.clear();
This method provides a way to remove all data stored in sessionStorage at once, effectively resetting it to its original state.
When is session storage useful?
sessionStorage
is most useful when data needs to be stored temporarily for a single browsing session. Here are a few instances when sessionStorage
may be helpful:
- Storing form data temporarily
- Saving shopping cart contents during an active user session
- Caching API responses for faster page load times
- Storing user preferences or settings for the current session
- Holding temporary data while navigating between pages on a website.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
What is localStorage?
localStorage
is a key-value store that enables local data storage for web apps within the user’s browser. The data stored in localStorage
persists even after the browser is closed, allowing web applications to store and retrieve data across multiple user sessions.
Set and retrieve items
Like sessionStorage
, localStorage
offers two syntax
options for setting items, as shown in the code below.
localStorage.setItem(identifier, value);
// OR
localStorage.identifier = value;
For example:
localStorage.name = "Oluwole Opeyemi";
We can now access this data anywhere in our application.
localStorage.getItem("name");
// OR
localStorage.name;
// Outputs "Oluwole Opeyemi"
Remove item(s)
To remove an item from localStorage
. We can use the removeItem()
method, as shown below.
localStorage.removeItem(name);
Upon executing the code above, the key will be removed from local storage and no longer accessible via the localStorage
object.
Furthermore, to erase all the data stored in localStorage
at once, we could utilize the clear()
method:
localStorage.clear();
Doing this will delete all key-value pairs in the localStorage
.
When is localStorage useful?
Here are some of the most common situations where localStorage
can be helpful:
- When the data must be available even after the browser is closed or the user leaves the website.
- When data must be shared across multiple pages within the same website.
- When the data must be saved quickly without needing a server-side call.
- When the data is small and can be stored within the browser’s
localStorage
capacity limits. - When the data must be stored securely and not accessible by other websites.
Practical use-case for localStorage and sessionStorage
To demonstrate the differences between localStorage
and sessionStorage
, let’s quickly develop a basic application that stores an input name using both methods. Create a new HTML file (index.html
) and paste the following code.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="list.css" />
<title>Login Form</title>
</head>
<body>
<div class="form">
<input type="text" id="fullname" placeholder="Enter Fullname" />
<p>
My name is <b id="userId">Anonymous</b>, A software developer<br /><br />
and a technical writer
</p>
<br />
<button type="button" onclick="update()">Update Name</button>
</div>
</body>
<script>
if (localStorage.getItem("fullname") === null) {
document.getElementById("userId").innerHTML = "Anonymous";
} else {
document.getElementById("userId").innerHTML =
localStorage.getItem("fullname");
}
function update() {
let fullname = document.getElementById("fullname").value;
localStorage.setItem("fullname", fullname);
document.getElementById("userId").innerHTML =
localStorage.getItem("fullname");
}
</script>
</html>
From the code above, we created a simple HTML input form so that when a user enters their name, we store it in the local storage. Finally, we retrieve the stored name and display it in the user’s bio information, as shown below:
When we closed the tab, the user’s name stored in the localStorage
remained unchanged.
On the other hand, sessionStorage
only stores data for the current session and will be lost when the user closes the browser. Let’s update the script with the following sessionStorage
code.
<script>
if (sessionStorage.getItem("fullname") === null) {
document.getElementById("upd").innerHTML = "Anonymous";
} else {
document.getElementById("upd").innerHTML =
sessionStorage.getItem("fullname");
}
function update() {
let fullname = document.getElementById("fullname").value;
sessionStorage.setItem("fullname", fullname);
document.getElementById("upd").innerHTML =
sessionStorage.getItem("fullname");
}
</script>
In the code above, we use sessionStorage
to store the user’s name and then display it in the user bio info, as shown below.
As we can see in the two examples above, the user’s name is deleted from the sessionStorage
when the tab is closed.
Local/Session Storage vs. Cookies
When to use localStorage
and sessionStorage
over Cookies?
localStorage
and sessionStorage
can be preferable in the following situation.
- Security: if security is an issue,
localStorage
andsessionStorage
are less susceptible to cross-site scripting (XSS) attacks as the data is stored on the client-side and is not included with everyHTTP
request like cookies which is mainly vulnerable to various attacks - Privacy: In terms of privacy, many developers may choose to use local and session storage over cookies as it gives them more control over the data stored on their device.
- API: Cookies have a more complex API, whereas local and session storage is more straightforward.
- Storage capacity: Local and session storage give room to store more important data than cookies, which have a storage capacity of 4kb.
Conclusion
localStorage
is preferable for data that must be kept across multiple browser sessions, while sessionStorage
is appropriate for temporary data needed only for the current browser session. Selecting the best storage mechanism for your application based on the specific use case is important.