When to use MCP vs REST vs GraphQL in your project
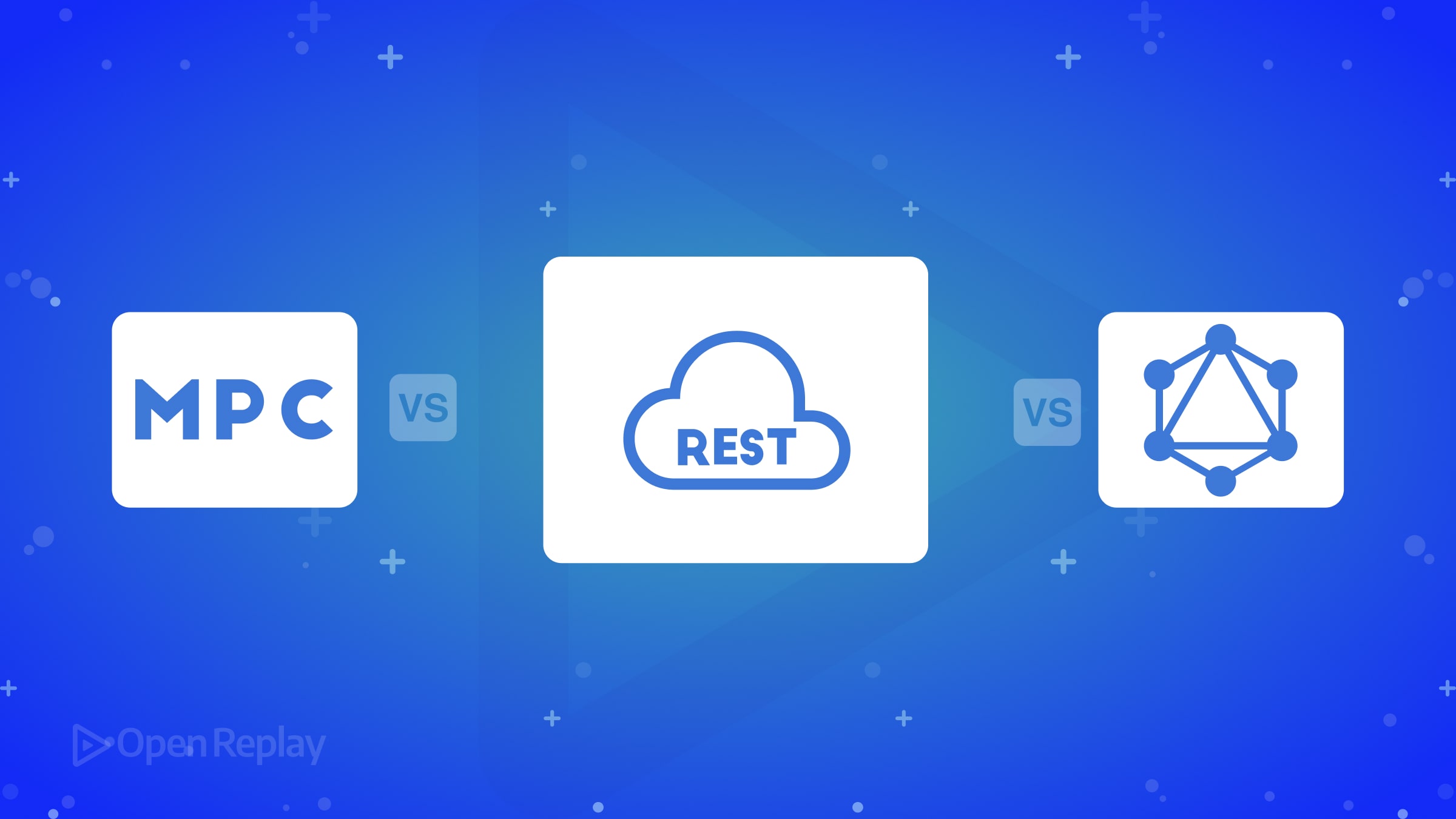
Choosing between REST, GraphQL, and MCP isn’t just about what’s “new” or “popular.” It’s about what matches your project’s needs. As a junior developer, knowing the right tool for the right situation can save you time and frustration. This guide explains when to use each option — practically, simply, and with real examples.
Key Takeaways
- REST, GraphQL, and MCP serve different technical needs: simple APIs, flexible data queries, and AI-powered tool integrations.
- Understanding the type of app you’re building is the first step to choosing the right one.
1. When to use REST
Use REST if:
- Your app deals with simple resources that map cleanly to URLs.
- You want strong caching, simple debugging, and mature community support.
- You don’t need highly dynamic queries from the client side.
Example: A simple blog backend
In a blog platform:
GET /posts # Get all blog posts
GET /posts/1 # Get the blog post with ID 1
POST /posts # Create a new blog post
PUT /posts/1 # Update post 1
DELETE /posts/1 # Delete post 1
You can easily model Posts, Users, Comments, etc., using basic HTTP verbs (GET, POST, PUT, DELETE).
Why REST fits here:
- The structure is predictable.
- HTTP methods map directly to operations.
- It’s easy to work with browser tools and libraries like
fetch
,axios
, etc.
Best for CRUD apps, backends for mobile apps, microservices.
2. When to use GraphQL
Use GraphQL if:
- You often need to fetch related or nested data in a flexible way.
- You want clients (the browser or app) to ask only for the fields they need.
- You aim to minimize the number of network requests.
Example: A product catalog for an e-commerce app
Instead of doing several REST calls for product info and reviews, you can query like this:
query {
product(id: "123") {
name
price
images {
url
altText
}
reviews {
author
rating
comment
}
}
}
One request — and you get everything you need.
Why GraphQL fits here:
- You avoid over-fetching (getting too much data) and under-fetching (missing data).
- You optimize for mobile devices with slower networks.
- It fits applications where front-end developers move fast and want control over data.
Best for SPAs (Single Page Apps), mobile apps, dashboards.
3. When to use MCP
Use MCP if:
- You are building AI-first products that need an LLM (like Claude, GPT) to interact with live systems.
- You want dynamic two-way communication: models discovering and using tools at runtime.
- You need your app to be AI-native, not just AI-assisted.
Example: AI agent writing to a database
Rather than coding each interaction manually, the LLM can discover and use available operations:
# Example of calling an MCP-discovered tool
# Connect to the MCP client
mcp_client = MCPClient()
# Discover available tools
available_tools = mcp_client.list_tools()
# Find the tool for database insert
db_insert_tool = available_tools["insert_user"]
# Execute it
response = db_insert_tool.execute({
"username": "new_user",
"email": "user@example.com"
})
print(response)
The model didn’t need to “know” about the database beforehand. The MCP server described the tool, and the LLM used it.
Why MCP fits here:
- You can add, remove, and upgrade tools without re-training or re-prompting the model.
- LLMs work with APIs, databases, and storage in a universal way.
- It reduces the custom glue code you usually need when building agent-based apps.
Best for AI applications, AI agents, tool-using LLMs.
Quick comparison table
Criteria | REST | GraphQL | MCP |
---|---|---|---|
API design style | Fixed endpoints | Flexible queries | Dynamic tool and resource discovery |
Who controls data shape? | Server | Client | LLM, dynamically |
Real-time support | No | Limited (subscriptions) | Yes, event-driven interaction |
State management | Stateless | Stateless | Stateful sessions possible |
Best use case | Simple web or mobile APIs | Complex front-end driven apps | AI-native applications needing external context |
Developer maturity | Very mature | Growing rapidly | Emerging, still evolving |
Bonus: 5 Quick Questions to Pick Between REST, GraphQL, and MCP
Question | If Yes → |
---|---|
Is your app about CRUD (Create, Read, Update, Delete) operations? | Use REST |
Does the front-end need to control exactly what data it gets? | Use GraphQL |
Are you building something where an AI (LLM) acts, decides, or uses tools? | Use MCP |
Do you need real-time two-way interaction between tools and the app? | Use MCP |
Is stability and broad community support critical? | Use REST |
Conclusion
Choosing between REST, GraphQL, and MCP isn’t about hype — it’s about understanding your app’s needs:
- Pick REST if you need simplicity and broad tool support.
- Pick GraphQL if you want flexible queries and control over payload size.
- Pick MCP if you’re building apps where AI is the main actor, not just a helper.
Each approach fits a different future: REST for traditional apps, GraphQL for dynamic front-ends, and MCP for AI-powered systems.
FAQs
Yes. Some MCP servers can expose GraphQL APIs as tools, letting LLMs query them at runtime.
MCP is relatively new (released in late 2024), but it's growing fast. For AI-first projects, it's worth experimenting now.
Yes, they are different models. MCP isn't just a new API — it's a whole new way for AIs to interact dynamically. It often requires rethinking how you expose functionality.