Next.js vs NuxtJS: a comparison of two powerhouses
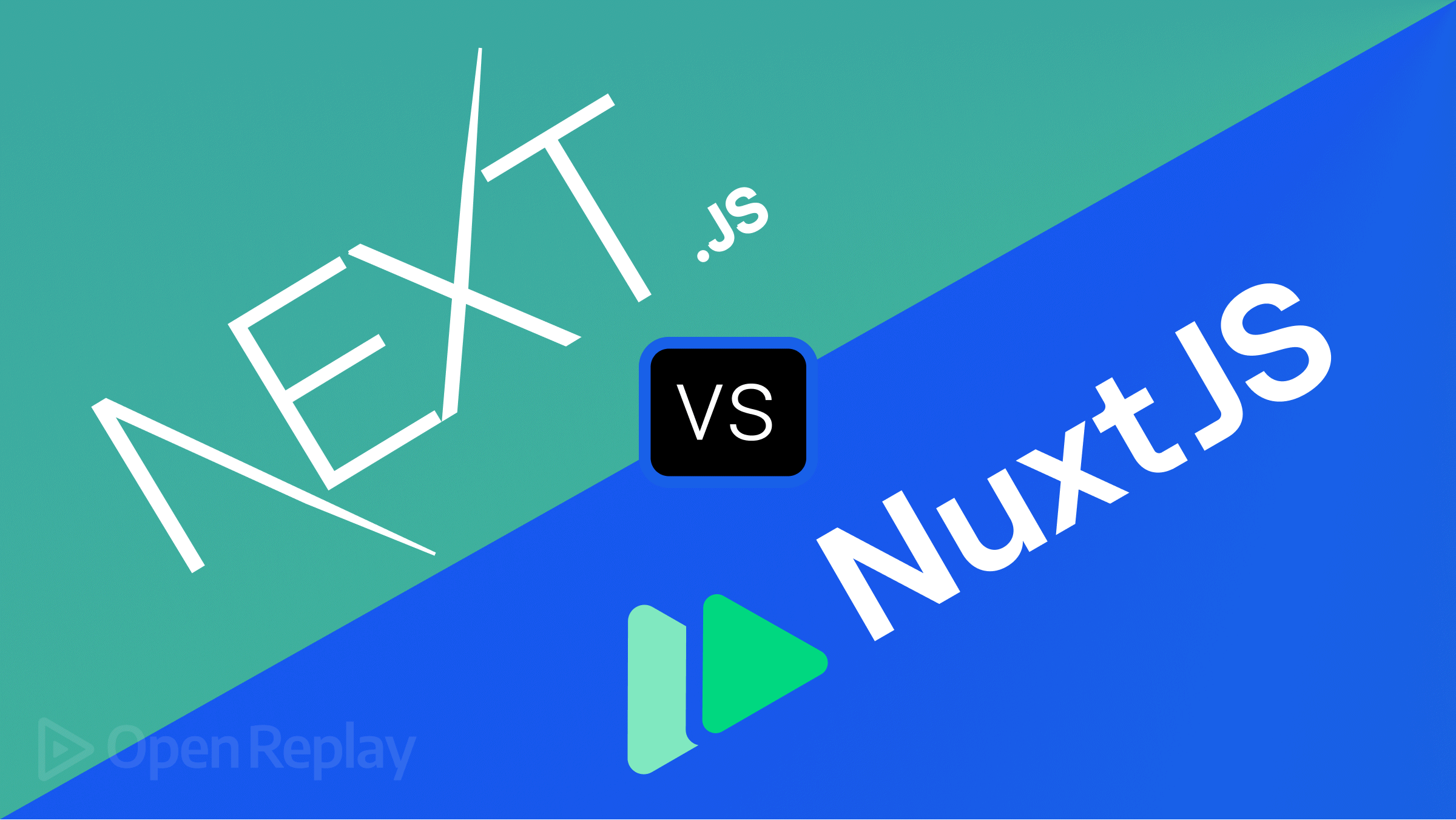
It is challenging to keep up with the rate at which the JavaScript ecosystem is evolving with the release of new frameworks every year. Understanding the different JavaScript frameworks is vital, especially if you are a beginner who would require a more straightforward approach.
Modern front-end web development is not complete without JavaScript frameworks, which give programmers tried-and-true tools for creating scalable, dynamic web applications. Today, familiarity with frameworks is often required for front-end development professions because so many modern businesses use them as a basic tooling component. I hope this article will provide a welcoming place to start while you learn about the different frameworks.
It can be challenging for aspiring front-end developers to decide where to start when learning frameworks because there are so many options, new ones are constantly being created, they largely operate similarly but perform some tasks differently, and there are some things to watch out for when utilizing frameworks.
At the end of this article, you’d be conversant with the components of Next.js and NuxtJS and possess a basic understanding of both frameworks and choose what works for you.
What is NuxtJS?
NuxtJS is a free, open-source JavaScript framework used to build Vue.js apps. Its objective is to make it simple, quick, and organized for Vue developers to benefit from cutting-edge technologies. NuxtJS was influenced by Next.js, a framework built on React.js with a similar goal.
The foundation of your Vue.js project is NuxtJS, which provides structure and flexibility so you can build it with confidence. With a focus on providing a fantastic developer experience, NuxtJS aims to make web development simple and effective. You can concentrate on what matters —creating your application— with it.
The new features in NuxtJS 3’s public beta, released in October, gave developers more time to focus on creating wonderful user experiences. As a result, the NuxtJS community’s growth has exploded.
Modules Ecosystem
To expand the framework’s base and streamline integrations, NuxtJS offers a module system. The higher-order module system offered by NuxtJS enables the core to be expanded. When NuxtJS boots, modules are functions that are called in order. If there is an existing NuxtJS module for it, you don’t need to create it all from scratch or maintain boilerplate. “Nuxt.config” can be used to add NuxtJS modules.
Plugins and configuration settings can be added to NuxtJS, but managing these adjustments across various projects can be laborious, repetitive, and time-consuming. However, if NuxtJS were to meet every project’s requirements out of the box, it would be overly complicated and challenging to use.
This is one of the reasons NuxtJS offers a modular architecture that enables the extension of the core. In addition to many other helpful activities, they can configure webpack loaders, install CSS libraries, and alter template files. The distribution of NuxtJS modules in npm packages is the best part. To know more about NuxtJS modules, click here and here.
Components Auto-Import
To perform data fetching, access the app context and runtime config, manage state, or construct components and plugins, NuxtJS auto-imports functions and composables. Any components in your components/ directory are immediately imported by NuxtJS (along with components registered by any modules you may be using).
| components/
--| TheHeader.vue
--| TheFooter.vue
layouts/default.vue
:
<template>
<div>
<TheHeader />
<slot />
<TheFooter />
</div>
</template>
In NuxtJS 3, we receive a new additional folder called “composables/” that will automatically import the Composition API function that you write. Components are auto-imported in the “components/” folder, and file-based routing is in the “pages/” folder. Click here to learn more about NuxtJS auto-imports.
Rendering Modes
Rendering is the process through which the browser and server translate Vue.js components into HTML elements by reading JavaScript code.
Client-side and global rendering are both supported by NuxtJS. A complex, dynamic user interface (UI) with seamless page transitions can be created using client-side rendering techniques. While maintaining the advantages of client-side rendering, universal rendering enables NuxtJS applications to offer rapid page loads. In addition, since the content is already included in the HTML text, crawlers can index it without further effort.
In addition to determining how the Server should respond to a new request on a specific URL, hybrid rendering permits various caching rules per route.
JavaScript apps frequently struggle with dealing with SEO and Meta tags. This is because the page is initially empty when we rely on JavaScript to obtain our data, such as the content and meta descriptions. Additionally, there is no material to index or process before JavaScript is performed. In addition, a lot of crawlers don’t support JavaScript. As a result, if you share an article from your JavaScript blog on Facebook, it can appear with the title “undefined” rather than the original article’s title. Server Side Rendering (SSR) can save us in this situation. The goal behind SSR is to host a server that will generate the HTML response and provide it to the crawler or client. As a result, in the example mentioned above, the server will handle the call to the API, after which the metadata will be set, and the final page will be delivered. When the crawlers parse the page, all the meta and SEO information will be present.
With NuxtJS 3, hybrid rendering is now possible. This will make it possible for you to use features like Incremental Static Generation, which combines Server Side Rendering (SSR) and Static Site Generation (SSG), as well as other sophisticated modes. Rendering Modes can also be found here and here.
File-System Routing
For every page, NuxtJS offers automatic routing and code-splitting. NuxtJS automatically creates the Vue-router configuration based on your Vue file tree inside the pages directory. You don’t need to do any additional configuration once you create a .vue file in your pages’ directory to get basic routing working.
This file tree:
pages/
--| user/
-----| index.vue
-----| one.vue
--| index.vue
will automatically generate:
router: {
routes: [
{
name: 'index',
path: '/',
component: 'pages/index.vue'
},
{
name: 'user',
path: '/user',
component: 'pages/user/index.vue'
},
{
name: 'user-one',
path: '/user/one',
component: 'pages/user/one.vue'
}
]
}
Using Vue Router as the backend, a file-based routing may be used to construct routes in your online application. If you only use app.vue, vue-router won’t be included because this directory is optional, reducing your application’s bundle size. Throughout your application, NuxtJS offers a customized route middleware framework that is perfect for extracting code you want to execute before going to a specific route.
Run within your NuxtJS app’s Vue component is route middleware. Despite having a similar name, they are not the same as the server middleware that runs on your app’s Nitro server. More about NuxtJS file-routing can be found here.
Zero Configuration
NuxtJS’s default configuration covers the majority of use cases. The nuxt.config.js file allows for the replacement of the default configuration. You can use NuxtJS to specify the global CSS files, modules, and libraries you want to use (included on every page). Add these CSS resources to nuxt.config.js:
export default {
css: [
// Load a Node.js module directly (here it's a Sass file)
'bulma',
// CSS file in the project
'~/assets/css/main.css',
// SCSS file in the project
'~/assets/css/main.scss'
]
}
NuxtJS will automatically determine the type of file based on its extension and utilize the proper pre-processor loader for webpack. You must still install the necessary loader if you want to use them. You can omit the file extension for files specified in the CSS array in your NuxtJS configuration file, such as CSS, SCSS, Postcss, Less, Stylus, etc.
export default {
css: ['~/assets/css/main', '~/assets/css/animations']
}
NuxtJS handles the rest as you get directly to work on your app’s code. Here’s more information on NuxtJS Configuration.
Data Fetching
You may use NuxtJS to get content from any source into your SSR-capable Vue components. NuxtJS offers the Fetch Method and the asyncData Method as its two (2) methods for obtaining data from an API.
After the component instance is established, Fetch is a hook invoked both on the client during navigation and server-side rendering. The fetch hook should (either explicitly or implicitly via async/await) return a promise that will be fulfilled: Before the first page is rendered on the server, and after the component has been mounted on the client.
When using static hosting, the fetch hook is only used when creating pages, and the output is then cached for client use. It could be essential to provide your component with a name or, failing that, to offer a special fetchKey implementation to prevent cache conflicts.
Another hook for global data retrieval is asyncData. As opposed to Fetch, which requires you to execute Vuex actions or change properties on the component instance to preserve your async state, asyncData just combines the return value with the local state of your component. Using the @nuxt/http library, consider the following example:
<template>
<div>
<h1>{{ post.title }}</h1>
<p>{{ post.description }}</p>
</div>
</template>
<script>
export default {
async asyncData({ params, $http }) {
const post = await $http.$get(`https://api.nuxtjs.dev/posts/${params.id}`)
return { post }
}
}
</script>
N/B: asyncData is solely accessible for pages; the hook has no access to it. More information on Data Fetching can be found in this guide.
SEO Optimization
You have three options with NuxtJS for including metadata in your application:
- Utilizing nuxt.config.js globally
- Locally utilizing the head as an object
- Using the head locally as a function to retrieve data and computed properties
NuxtJS offers management of the meta tags and a quicker time to content for excellent indexing. Here’s more guide on Meta Tags and SEO.
Pros of NuxtJS
NuxtJS is extensible and makes it simple to integrate your favorite REST or GraphQL endpoints, CMS, CSS frameworks, and more, thanks to its robust module ecosystem and hooks engine. The following are some of the many benefits of utilizing NuxtJS :
- You can deploy full-stack Vue apps that are fast and SEO-friendly with the help of NuxtJS, which also provides a compelling solution and a great ecosystem.
- With its innovative approach, NuxtJS accelerates the creation and functionality of your upcoming website or application by fusing a superb developer experience with reusable, fully integrated features.
- NuxtJS’s simplicity and gradual learning curve make it a fantastic option.
- Between Server-Side Rendering (SSR) and Static Site Generation, NuxtJS provides versatility (SSG).
- In addition to its opinionated setup and structure, NuxtJS offers automatic code splitting, effective teamwork, and solid directory layout and conventions.
Cons of NuxtJS
Every benefit has a matching drawback. The drawbacks of utilizing NuxtJS are listed below:
- There are fewer resources and perhaps less thorough product documentation available for NuxtJS due to its small community.
- On your server, high traffic might be especially taxing.
- Only specific Hooks allow you to interact with and query the DOM.
- It can be challenging to get customized libraries to work with NuxtJS.
- There are undoubtedly popular, reliable plugins or missing elements, such as Calendar, Google Maps, vector maps, etc. Although there are additional components, they are typically not well maintained.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
What is Next.js?
Next.js is the best tool for any React developer to learn and improve their projects. It is an open-source web development framework built on top of Node.js and facilitates server-side rendering and the deployment of static websites for React-based web applications.
The finest developer experience is provided by Next.js, which has all the capabilities required for production, including TypeScript support, smart bundling, route pre-fetching, and hybrid static & server rendering. It is not necessary to configure.
Several cutting-edge capabilities offered by Next.js will advance your online application. The features of Next.js are as follows:
File System Routing
Using the idea of pages as its foundation, Next.js has a file-system-based router. A route becomes automatically available when a file is added to the pages directory. You can define most patterns using the files in the pages directory.
The segments for the Index route, Nested route, and Dynamic routes are available here. The router will automatically direct files with the name index to the directory’s root when using this Index route.
'pages/index.js' → '/'
'pages/blog/index.js' → '/blog'
The router is compatible with nested files in the Nested route, and a nested folder structure will still automatically transport files similarly.
'pages/blog/first-post.js' → '/blog/first-post'
'pages/dashboard/settings/username.js' → '/dashboard/settings/username'
Additionally, you can utilize the bracket syntax in the Dynamic route segments. You can now match named parameters thanks to this.
'pages/blog/[slug].js' → '/blog/:slug' ('/blog/hello-world')
'pages/[username]/settings.js' → '/:username/settings' ('/foo/settings')
'pages/post/[...all].js' → '/post/*' ('/post/2020/id/title')
Check out the routes documentation to learn more about how they work.
Server Side Rendering
Depending on the use case for your application, data fetching in Next.js enables you to render your information in various ways. These include rendering beforehand using static site generation (SSG) or server-side rendering (SSR), as well as dynamic content creation or updating using incremental static regeneration. Next.js will pre-render a page using the information supplied by a function called ‘getServerSideProps’ (Server-Side Rendering) if you export it from a page.
export async function getServerSideProps(context) {
return {
props: {}, // will be passed to the page component as props
}
}
Every ‘prop’ will be sent to the page component regardless of the rendering method, and the first HTML can be read on the client-side. To properly hydrate the page, this is necessary.
Static Site Generation
A list of paths that should be statically created must be defined if a page utilizes ‘getStaticProps’ and contains Dynamic Routes. Any paths supplied by a function called ‘getStaticPaths’ (Static Site Generation) exported from a page that employs dynamic routes will be statically pre-rendered by Next.js.
export async function getStaticPaths() {
return {
paths: [
{ params: { ... } }
],
fallback: true // false or 'blocking'
};
}
The API reference covers all the options and properties that can be used with ‘getStaticPaths’. Next.js pre-renders pages at build time (SSG) or request time (SSR) in a single project.
Image Optimization
The HTML img
element has been extended for the modern web by the ‘next/image’ component of Next.js. You can get good Core Web Vitals with the help of a range of built-in performance enhancements. These ratings are a crucial indicator of how well users respond to your website and are considered by Google when determining search results.
Next.js will automatically calculate the image’s width and height using the imported file as a basis. While your image is loading, these settings are utilized to stop cumulative layout shifts.
import Image from 'next/image'
import profilePic from '../public/me.png'
function Home() {
return (
<>
<h1>My Homepage</h1>
<Image
src={profilePic}
alt= "Picture of the author"
// width={500} automatically provided
// height={500} automatically provided
// blurDataURL="data:..." automatically provided
// placeholder="blur" // Optional blur-up while loading
/>
<p>Welcome to my homepage!</p>
</>
)
}
Automatic Code-Splitting
Only the JavaScript and libraries that are required to render pages are used. Instead of creating a single JavaScript file containing all of the app’s code, Next.js automatically divides the app into several resources. Only the JavaScript required for that specific page is loaded when a page is loaded. To achieve it, Next.js examines the imported resources.
One noteworthy exception exists. If an import is utilized in at least 50% of the site pages, it is moved into the main JavaScript bundle.
TypeScript Support
Next.js has strong TypeScript support because it is TypeScript-written. With zero configuration setup and built-in types for Pages, APIs, and more, Next.js offers an integrated TypeScript experience. Using the ‘—ts, —typescript flag’, you may use ‘create-next-app’ to create a TypeScript project as follows:
npx create-next-app@latest --ts
# or
yarn create next-app --typescript
# or
pnpm create next-app --ts
Type checking is a standard feature of Next.js’s ‘next build’. To learn more on Next.js typescript, click here.
API Route
One of Next.js’s game-changing features is its all-in-one approach to building full-stack React apps by enabling you to write server code using API routes. Next.js’s API routes offer a way to construct your API.
Any file in the folder ‘pages/api’ is mapped to ‘/api/*’ and is handled more like an API endpoint than a ‘page’. They don’t raise the size of your client-side bundle because they are server-side only bundles.
Pros of Next.js
The largest and most well-known businesses worldwide frequently use Next.js. It’s regarded as one of the React frameworks with one of the highest growth rates and is excellent for use with static sites. The advantages of adopting Next are as follows:
- For SEO, Next.js is fantastic. It enables you to create web applications with all the necessary capabilities and interactivity while retaining the SEO advantages of a static text-based webpage. You can establish a powerful online presence thanks to it.
- The user experience is excellent with Next.js. You are not required to adhere to any plugins, templates, or other limitations imposed by eCommerce or CMS platforms. You have complete freedom to alter the front-end whatever you see fit, and you can also creatively edit things without any restrictions.
- The performance of your website or web application is enhanced using Next.js. While downloading and running a significant amount of JavaScript code at once does not cause the browser to halt, it can greatly enhance metrics like total blocking time (TBT). Always aim for a time that is less than 300ms. The faster your TBT increases, the more quickly people will find value in your online app.
- Your website or application will be secure if you use Next.js. Next.js static websites don’t have any direct connections to the database, dependencies, user data, or any other private data because they don’t have any of those things. Data security is thereby guaranteed.
- Webpack, a component of Next.js, allows for out-of-the-box assistance for asset compilation, hot reloading, and code-splitting, all of which can speed up development. As React and Next.js get more popular, so does the developer community that uses them. It will be simple to locate a business or independent contractor to make alterations. The finest support for asset compilation, code-splitting, and hot reloading is consistently found by developers to be provided by Next.js.
Cons of Next.js
Although Next.js offers a fantastic development experience, there are always limitations. The Next.js team is working furiously to fix the issues. Thus, the constraints are getting smaller and smaller every day. The following are some restrictions on Next.js usage:
- A weak plugin ecosystem exists for Next.js. Simple to adapt plugins are limited in their use.
- There is no internal state manager either. This implies that you must include Redux, MobX, or another state manager in your app if you need one.
- There is a drawback to the versatility Next.js offers, and that is continual maintenance. You will require a committed individual with the necessary knowledge to implement any requested adjustments successfully.
- Only a single router is allowed to be used by Next.js. In other words, the method that uses routes cannot be tweaked. If your project involves dynamic routes, you must use the Node.js server.
Next.js vs NuxtJS Comparison
Next.js is the first choice for developers when building sophisticated web applications because of its capacity to construct complex websites quickly. The developers of Next.js promote it as a single-command, zero-configuration toolchain for React projects. It is a React framework that transparently manages server-side rendering for you and offers a common architecture that makes it simple to construct a front-end React application. When Next.js notices a modification saved to disk, the page is reloaded. It functions well with other JavaScript, Node, and React components. Before transmitting the HTML to the client, you can render React components on the server side using Next.js.
A well-liked Vue.js framework is NuxtJS. It makes it easier to construct single-page or global Vue.js programs for the web. The NuxtJS framework aims to be versatile enough to serve as the main project base for web developers. You may concentrate on your web application’s logic by sharing the same code between the server and the client, thanks to NuxtJS. NuxtJS allows you access to numerous properties on your components, such as “isClient” and “isServer,” so you can quickly determine whether you are rendering something on the server or the client. This is merely the start of NuxtJS’s extensive list of benefits for developing universal web applications.
Is Next.js Better than NuxtJS ?
There is no superior structure. It depends on what you find to be effective and its various qualities.
In essence, Next.js is a React framework that can be utilized when a user requires quick rendering in challenging circumstances.
On the other hand, NuxtJS is a Vue framework that aids in streamlining and speeding up the web development process.
Best Use Cases for NuxtJS
NuxtJS was created to address problems associated with creating Single Page Applications (SPAs), which are the focus of many contemporary JavaScript frameworks. Enforcing convention over configuration strategy is the most distinctive application of NuxtJS. You don’t have to create many configuration files while using NuxtJS. Instead, you set up the folder structure so NuxtJS can comprehend it and then construct the app’s final bundle following that.
Your app’s highly optimized bundle is created using NuxtJS. A sample use case is Storyblok and Pentest Tools sites.
Best Use Cases for Next.js
One of the best features of Next.js is that you can create static pages that nonetheless function like dynamic ones. This is ideal when: (a) the content will be changed regularly or needs to be current at all times. (b) Real-time publishing capabilities are required, for instance, for multi-user sites. (c) Rebuilding the entire website would take a lot of time (and money) using SSG and is not at risk. A significant eCommerce website is a good example.
Next.js aids programmers in avoiding typical problems. Next.js prevents developers from accidentally adding issues, such as over-fetching deep in the component tree, by providing a single abstraction for performing challenging UI tasks. Developers understood exactly where to seek to acquire new data if needed. The complex underlying APIs didn’t need to be learned by anyone.
Some very good case studies are the Hulu and Typeform sites.
Conclusion
The advantages and disadvantages of the Next.js and NuxtJS frameworks may be clear to you once you’ve read the entire text. We cannot emphasize enough how prevalent Javascript is and how nearly all current mobile and online applications are built using it, making the use of frameworks like Next.js and NuxtJS necessary. Therefore, one must select and use the most effective framework for their needs. I’m hoping this post will assist you in deciding between Next.js and NuxtJS for your upcoming project.
This article’s objective is not to teach you everything; rather, it is to progressively introduce you to the full potential of Next.js and NuxtJS.
To learn more about all the capabilities and functionality I didn’t include, some of which are very great, I suggest having a thorough read through the official Next.js and NuxtJSs documentation.
Finally, let me say that you wouldn’t lose anything if you started learning any of them for your vocation or knowledge.
Coding is fun!
A TIP FROM THE EDITOR: To compare both frameworks for a similar task, check out our Creating a Markdown Blog Powered by Next.js in Under an Hour and How to build a CMS-powered blog with Nuxt articles.