Node-gyp Troubleshooting Guide: Fix Common Installation and Build Errors
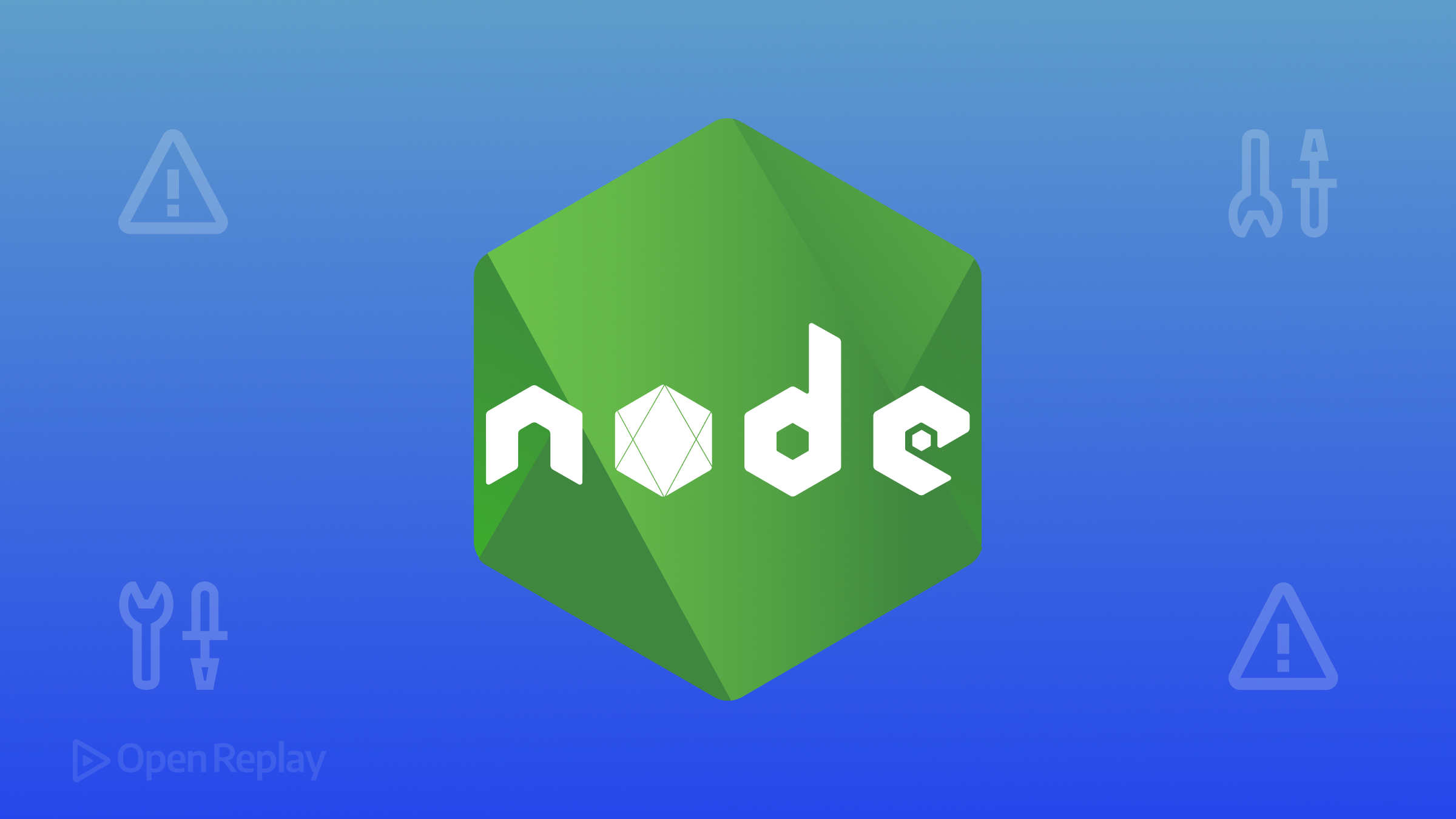
Node-gyp is one of the most frustrating tools in the Node.js ecosystem. When it works, you barely notice it. When it fails, it can bring your entire development workflow to a grinding halt with cryptic error messages and complex dependency requirements.
This guide will help you understand what node-gyp is, why it causes so many problems, and most importantly, how to fix the most common errors you’ll encounter.
Key Takeaways
- Node-gyp is a build tool for compiling C/C++ code into native Node.js addons
- Most node-gyp errors stem from missing system dependencies or incompatible versions
- Platform-specific setup is crucial (Windows, macOS, and Linux each have different requirements)
- Proper configuration of Python and C++ compilers solves most common issues
- For complex projects, consider using pre-built binaries or Docker containers
What is node-gyp and Why Do We Need It?
Node-gyp is a cross-platform command-line tool that compiles native addon modules for Node.js. It’s not a compiler itself, but rather a build automation tool that generates platform-specific project files and calls the appropriate compiler for your system.
Native addons are modules written in C/C++ that can be loaded into Node.js using the require()
function. They’re used when:
- You need better performance than JavaScript can provide
- You need to interface with system-level APIs
- You want to use existing C/C++ libraries in your Node.js application
When you install a package that contains C/C++ code (like bcrypt
, canvas
, or sqlite3
), npm runs node-gyp behind the scenes to compile that code for your specific platform and Node.js version.
Why node-gyp Causes So Many Problems
Node-gyp is often the source of installation errors because:
- Complex dependency chain: It requires Python, C++ compilers, and other system tools that many JavaScript developers don’t have installed
- Cross-platform challenges: Each operating system needs different compilers and build tools
- Version compatibility: Different Node.js versions require different compilation settings
- System permissions: It often needs administrator/root access to install dependencies
Prerequisites for node-gyp
Before troubleshooting specific errors, make sure you have the correct prerequisites installed for your platform:
On Windows
# Option 1: Using Chocolatey (recommended)
choco install python visualstudio2022-workload-vctools -y
# Option 2: Using npm
npm install --global --production windows-build-tools
On macOS
# Install Xcode Command Line Tools
xcode-select --install
# If using Homebrew
brew install python
On Linux (Ubuntu/Debian)
sudo apt-get update
sudo apt-get install python3 make g++ python3-pip
Common node-gyp Errors and Solutions
1. Python Not Found or Wrong Version
Error message:
gyp ERR! find Python
gyp ERR! configure error
gyp ERR! stack Error: Could not find any Python installation to use
Solution:
-
Install Python (3.x is now supported in newer node-gyp versions):
# Windows choco install python # macOS brew install python # Linux sudo apt-get install python3
-
Tell node-gyp which Python to use:
# Set Python path for npm npm config set python /path/to/python # Or use environment variable export npm_config_python=/path/to/python
-
For Windows, you can also specify Python version:
npm config set python python3
2. Missing C++ Compiler
Error message:
gyp ERR! stack Error: not found: make
or
gyp ERR! stack Error: `msbuild` failed with exit code: 1
Solution:
Windows:
# Install Visual Studio Build Tools
npm install --global --production windows-build-tools
Or install Visual Studio 2022 with ""Desktop development with C++"" workload.
macOS:
xcode-select --install
Linux:
sudo apt-get install build-essential
3. Node.js Version Compatibility Issues
Error message:
gyp ERR! stack Error: This Node.js version is not supported by this node-gyp version
Solution:
-
Update node-gyp:
npm install -g node-gyp@latest
-
Specify Node.js target version:
node-gyp rebuild --target=v14.17.0
-
Use a Node.js version manager like nvm to switch to a compatible version.
4. Permission Errors
Error message:
gyp ERR! stack Error: EACCES: permission denied
Solution:
-
On Unix systems, avoid using
sudo
with npm. Instead, fix npm permissions:mkdir -p ~/.npm-global npm config set prefix '~/.npm-global' export PATH=~/.npm-global/bin:$PATH
-
On Windows, run your terminal as Administrator.
5. Visual Studio Version Issues (Windows)
Error message:
gyp ERR! find VS
gyp ERR! stack Error: Could not find any Visual Studio installation to use
Solution:
-
Specify Visual Studio version:
npm config set msvs_version 2022
-
For older packages that don’t support newer Visual Studio versions:
npm config set msvs_version 2017
-
Install the VSSetup PowerShell module:
Install-Module VSSetup -Scope CurrentUser
6. Missing binding.gyp File
Error message:
gyp ERR! configure error
gyp ERR! stack Error: Could not find a binding.gyp file in /path/to/module
Solution:
This usually indicates a problem with the package itself, not your setup. Try:
-
Clearing npm cache:
npm cache clean --force
-
Reinstalling the package:
npm uninstall package-name npm install package-name
-
Check if the package has been updated to fix this issue.
7. Proxy or Network Issues
Error message:
gyp ERR! stack Error: connect ETIMEDOUT
Solution:
-
Configure npm to use your proxy:
npm config set proxy http://your-proxy-address:port npm config set https-proxy http://your-proxy-address:port
-
For node-gyp specifically:
npm config set node_gyp_proxy http://your-proxy-address:port
Advanced Troubleshooting Techniques
Verbose Logging
Enable detailed logging to get more information about errors:
npm install --verbose
# or
node-gyp rebuild --verbose
Force Rebuild
Sometimes a clean rebuild can fix issues:
node-gyp clean
node-gyp configure
node-gyp rebuild
Using Pre-built Binaries
Some packages offer pre-built binaries as an alternative to compiling with node-gyp:
- node-pre-gyp: Downloads pre-built binaries when available
- prebuild: Creates pre-built binaries for multiple platforms
Example with sqlite3:
npm install sqlite3 --build-from-source
# vs
npm install sqlite3 --fallback-to-build
Docker for Consistent Build Environments
For team development or CI/CD pipelines, consider using Docker to create a consistent build environment:
FROM node:16
# Install build dependencies
RUN apt-get update && apt-get install -y
python3
make
g++
build-essential
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD [""npm"", ""start""]
Best Practices for Working with node-gyp
- Document dependencies: Include all system requirements in your project’s README
- Use package.json scripts: Add scripts to help team members install dependencies
- Consider alternatives: Before using a native addon, check if there’s a pure JavaScript alternative
- Keep build tools updated: Regularly update your compilers and build tools
- Use LTS Node.js versions: They tend to have better compatibility with native addons
Conclusion
By understanding what node-gyp is and how it works, you can more effectively troubleshoot and resolve the common errors that occur during installation and building of native Node.js addons. While it may seem complex at first, with the right setup and knowledge, you can minimize the frustration and get back to building your Node.js applications.
FAQs
Sometimes. Look for packages that offer pre-built binaries or pure JavaScript alternatives. For example, use `bcryptjs` instead of `bcrypt` if you don't need the performance benefits of the native implementation.
Node-gyp uses Python to run Google's GYP (Generate Your Projects) tool, which creates platform-specific build files.
Yes, newer versions of node-gyp (≥ v5.0.0) support Python 3. For node-gyp v10 and above, Python 3.12 is also supported.
Often, yes. Native modules are compiled for specific Node.js ABI (Application Binary Interface) versions. When you upgrade Node.js, you typically need to rebuild native modules.
Check if the package has a `binding.gyp` file in its repository, or if it lists `node-gyp` in its dependencies or scripts in `package.json`.