Optimize Vue with Web Workers
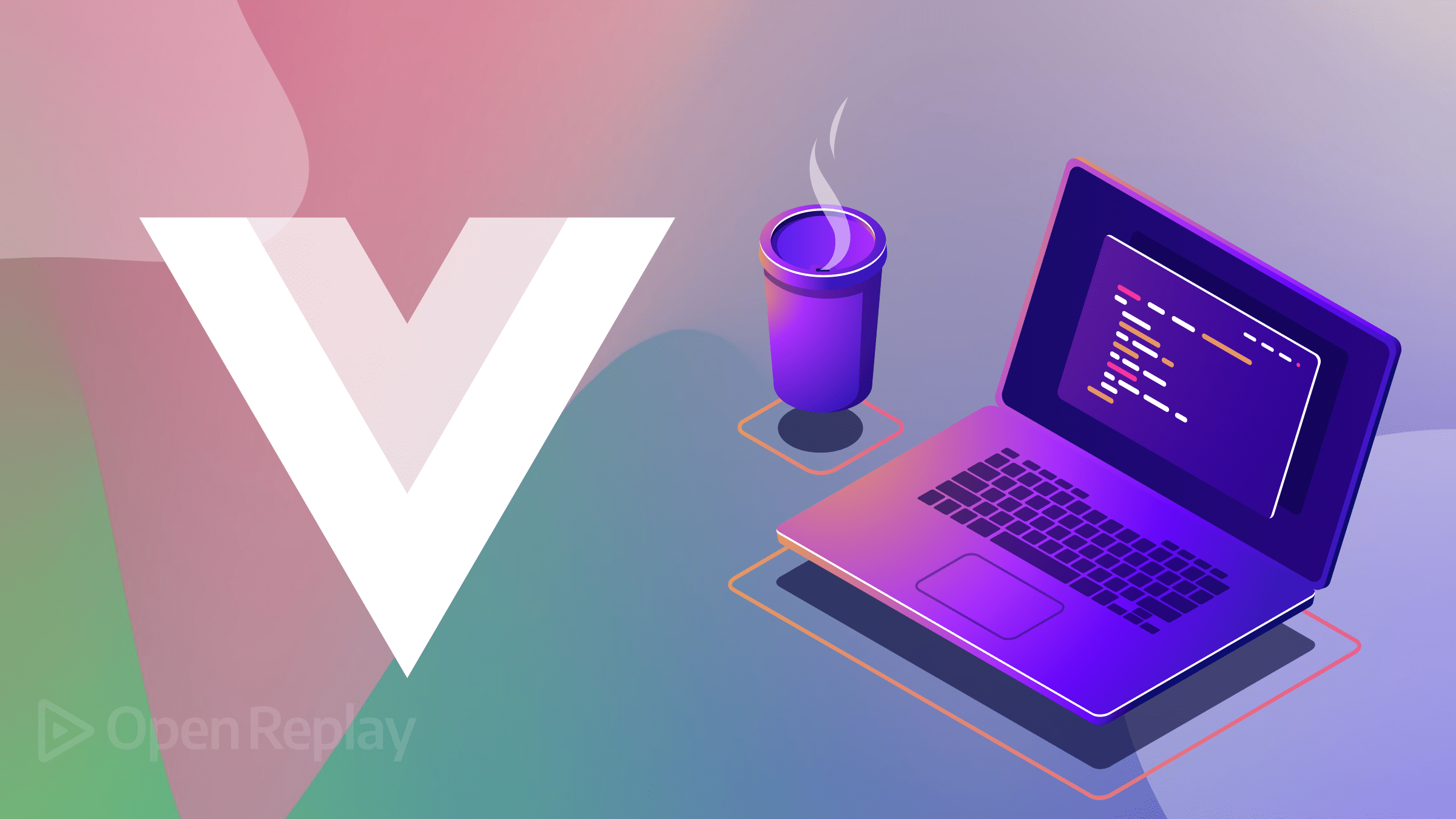
Web workers are a modern web technology that provides a way to run JavaScript in the background, parallel to the main execution thread. This concurrency model is especially beneficial in the browser environment, where, traditionally, JavaScript runs in a single-threaded fashion. They enable web applications to run background scripts without interfering with the main thread, and this article will show you how to use them.
Discover how at OpenReplay.com.
Vue.js is a progressive JavaScript framework used for building user interfaces, especially single-page applications (SPAs). It is renowned for its simplicity and component-based architecture. Since its introduction, it has evolved as one of the leading JavaScript frameworks, primarily because:
- Reactivity: Vue’s reactive data-binding system lets developers synchronize the UI with the underlying data model effortlessly.
- Component-based: This architecture facilitates code reusability and modular development.
- Lightweight: Vue is incrementally adoptable, meaning developers can start small and scale with complexity as needed.
Developers cherish Vue because it is easy to integrate and facilitates the creation of powerful single-page applications (SPAs) and user interfaces.
In today’s web, where applications are becoming increasingly dynamic and data-driven, performance is no longer a luxury—it’s a necessity. Users expect snappy responses, fluid animations, and instantaneous feedback. Here’s where web workers come in:
- Non-blocking Operations: Web workers can perform tasks without interfering with the main thread. This means the user interface remains entirely responsive while a web worker can process data or perform computations. Heavy computations or processing can hinder a web application’s responsiveness. Web workers mitigate this by running tasks in parallel, ensuring the main thread remains unblocked.
- Optimal CPU Utilization: On multi-core processors common in today’s devices, web workers can truly shine by leveraging parallel processing capabilities.
- Scalable Web Applications: As web applications grow and take on more complex tasks, web workers provide a means to manage this complexity without sacrificing performance. They are, in essence, a step forward in building scalable, high-performance web applications.
Understanding Web Workers
To understand the significance of Web Workers, it is crucial first to comprehend the environment in which JavaScript traditionally operates. JavaScript, by design, is a single-threaded language. This means it has one call stack and one memory heap, executing one operation at a time. While this single-threaded nature simplifies state management and ensures consistency in operation, it can also introduce challenges, particularly with performance. For instance, long-running or computationally intensive tasks can block the main thread, leading to an unresponsive user interface, an issue often termed the “JavaScript freeze”.
The traditional solution to this issue involved techniques such as asynchronous callbacks, promises, or, more recently, async/await. However, while these solutions provide a way to work around the blocking issue, they don’t solve it entirely – the code is still technically running on the same single thread.
This is where Web Workers come into play. A web worker is a script the browser runs in the background, separate from the main execution thread. This means that no matter how intensive the operations a worker performs, it won’t block the user interface or other user-triggered scripts.
A web worker is, in essence, a JavaScript file hosted on a server, created using the Web Worker API’s Worker() constructor. Once instantiated, the worker operates in its own execution environment parallel to the main thread.
Each web worker has its own JavaScript context and global scope. It doesn’t share any state with the main thread, which helps ensure data consistency and eliminates the risk of race conditions, a common issue in multi-threaded environments.
Running tasks in the main thread versus a web worker has distinct advantages and drawbacks.
- Main Thread: When tasks run on the main thread, they can access the page’s DOM and directly manipulate it. They can also use window methods and objects. However, long-running or complex tasks can block user interactions, leading to a “frozen” or laggy interface.
- Web Worker: Tasks running inside a web worker do not block the main thread, ensuring a smooth, responsive user interface, even when heavy computation occurs in the background. However, web workers cannot access the DOM, limiting their use to non-UI tasks. Furthermore, they require a message-passing mechanism for communication with the main thread, which can be slightly more complex to manage.
Despite their limitations, web workers represent a significant step forward in JavaScript’s journey towards efficient multithreading and parallel processing capabilities, opening up new possibilities for performance optimization in web applications.
Integrating Web Workers in Vue.js
Before you start, identify the tasks in your application causing the UI to become unresponsive or are computationally heavy. These are prime candidates to be moved into a Web Worker.
One situation where Web Workers excel is in the realm of image processing. For example, when it comes to blurring an image, each pixel must be adjusted based on its neighboring pixels. This task can be computationally demanding for images. This may lead to unresponsive user interfaces if performed on the main thread.
Why Choose a Web Worker for Image Blurring? Three reasons come to mind:
- Enhanced Responsiveness: Blurring an high-resolution image can take time. If done on the thread, it can temporarily freeze the application and hinder user interactions. The main thread remains available by delegating this task to a web worker. Ensures that the application remains responsive.
- Improved Performance: Parallel processing can significantly boost image processing capabilities. On devices with cores, leveraging a web worker allows for processing by utilizing these cores effectively.
- Separation of Concerns: Image processing algorithms can be intricate and involved. We maintain focused application logic that prioritizes UI-related tasks by isolating this functionality within a web worker.
The following sections will illustrate how to create a Vue application that utilizes a Web Worker for blurring images. Users can upload images and instantly view the version within the application without experiencing any noticeable lag or delay. This is a feature that truly highlights the capabilities and efficiency of Web Workers in real-world usage scenarios.
Create the Worker File
Web Workers run in a separate file. Let’s create a new file called imageBlurWorker.js
.
In imageBlurWorker.js
, you can add your computation-intensive code:
self.onmessage = function (e) {
const imageData = e.data;
const blurredData = applyBoxBlur(imageData);
postMessage(blurredData);
};
function applyBoxBlur(imageData) {
// Your image blurring logic here.
// E.g;
const width = imageData.width;
const height = imageData.height;
const data = new Uint8ClampedArray(imageData.data);
const outputData = new Uint8ClampedArray(data.length);
for (let y = 0; y < height; y++) {
for (let x = 0; x < width; x++) {
const pixelIndex = y * width * 4 + x * 4;
const redSum = [0, 0, 0];
const pixelCount = 9;
for (let dy = -1; dy <= 1; dy++) {
for (let dx = -1; dx <= 1; dx++) {
const neighborY = y + dy;
const neighborX = x + dx;
if (
neighborY >= 0 &&
neighborY < height &&
neighborX >= 0 &&
neighborX < width
) {
const neighborIndex = neighborY * width * 4 + neighborX * 4;
redSum[0] += data[neighborIndex];
redSum[1] += data[neighborIndex + 1];
redSum[2] += data[neighborIndex + 2];
}
}
}
const outputIndex = y * width * 4 + x * 4;
outputData[outputIndex] = redSum[0] / pixelCount;
outputData[outputIndex + 1] = redSum[1] / pixelCount;
outputData[outputIndex + 2] = redSum[2] / pixelCount;
outputData[outputIndex + 3] = data[pixelIndex + 3]; // Alpha channel
}
}
return {
width: width,
height: height,
data: outputData,
};
}
In the applyBoxBlur
function, we implemented a basic box blur algorithm, a type of neighborhood averaging. The idea is to replace each pixel’s color value with the average of its neighboring pixels, including itself.
Here’s a step-by-step breakdown:
function applyBoxBlur(imageData) {
// 1 Extract the width, height, and data from the image
const width = imageData.width;
const height = imageData.height;
const data = new Uint8ClampedArray(imageData.data);
const outputData = new Uint8ClampedArray(data.length);
// 2 Iterate over each pixel in the image
for (let y = 0; y < height; y++) {
for (let x = 0; x < width; x++) {
const pixelIndex = y * width * 4 + x * 4;
let redSum = 0,
greenSum = 0,
blueSum = 0;
let pixelCount = 0;
// 3 For each pixel, look at its immediate neighbors (the 3x3 grid around the pixel)
for (let dy = -1; dy <= 1; dy++) {
for (let dx = -1; dx <= 1; dx++) {
const neighborY = y + dy;
const neighborX = x + dx;
// 4 Check boundaries to ensure we don't access pixels outside the image
if (
neighborY >= 0 &&
neighborY < height &&
neighborX >= 0 &&
neighborX < width
) {
const neighborIndex = neighborY * width * 4 + neighborX * 4;
redSum += data[neighborIndex];
greenSum += data[neighborIndex + 1];
blueSum += data[neighborIndex + 2];
pixelCount++;
}
}
}
// 5 Compute the average color value
outputData[pixelIndex] = redSum / pixelCount;
outputData[pixelIndex + 1] = greenSum / pixelCount;
outputData[pixelIndex + 2] = blueSum / pixelCount;
outputData[pixelIndex + 3] = data[pixelIndex + 3]; // Alpha channel (transparency) remains unchanged
}
}
// Return the new image data (blurred version)
return {
width: width,
height: height,
data: outputData,
};
}
- Initialization: We extract the image’s dimensions and data. We extract the width and height of the image. The
Uint8ClampedArray
is a typed array that clamps the values between 0 and 255, which is perfect for our RGBA values - Iterate Over Pixels: For each pixel in the image, we determine the average color value of its neighbors.
- Determine Neighbors: We look at each pixel’s immediate neighbors (the 3x3 grid around the pixel).
- Sum Color Values:
pixelIndex
determines the index in the data array for the current pixel.redSum
is then initialized to store the summed values for red, green, and blue channels, respectively, and we iterate over the neighboring pixels using the nested loops. For each neighbor, we calculate its position in the data array using neighborIndex. We then add the neighbor’s red, green, and blue values to our redSum array. - Calculate Averages:
outputIndex
determines the position of the current pixel in the output data array. We then divide the summed values for each channel bypixelCount
(9, representing the 3x3 grid) to get the average. These averaged values are then stored in the outputData array.
The box blur is a simple and effective method for image blurring, but it’s worth noting that other, more advanced blurring techniques are available. The box blur provides a uniform blur, but other methods can provide more nuanced or directional blurs, depending on the desired effect.
Instantiate and Use the Worker in Your Main Application
In your main JavaScript file or within a specific module where you need to use the worker:
// Initialize the worker
const worker = new Worker("imageBlurWorker.js");
// Set up an event listener to receive messages from the worker
worker.onmessage = function (event) {
const result = event.data;
console.log("Received result from worker:", result);
};
// Send data to the worker
const someData = { /.../ };
worker.postMessage(someData);
// Remember to terminate the worker when you're done
// worker.terminate();
Implement a web worker into Vue to create an image blur app
For example, Let us incorporate the web worker into a Vue component.
In your Vue component, you can instantiate a worker using the Worker
constructor, passing the path to your worker script as an argument:
const worker = new Worker('./imageBlurWorker.js');
Post messages to the worker and listen for its response.
To send data to the worker, use the postMessage
method:
self.postMessage(blurredData);
To listen for messages from the worker, assign a function to the onmessage
event handler of the worker:
self.onmessage = function (e) {
const imageData = e.data;
const blurredData = applyBoxBlur(imageData);
};
Demonstration in a Vue component
Here’s how you might use the Image blur worker within a Vue component:
<template>
<div>
<input type="file" accept="image/*" @change="handleImageUpload" />
<div v-if="blurredImage">
<img :src="blurredImage" alt="Blurred Image" />
</div>
</div>
</template>
<script>
export default {
data() {
return {
imageBlurWorker: null,
blurredImage: null,
};
},
created() {
this.imageBlurWorker = new Worker("imageBlurWorker.js");
this.imageBlurWorker.onmessage = (e) => {
const blurredImageData = e.data;
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
canvas.width = blurredImageData.width;
canvas.height = blurredImageData.height;
ctx.putImageData(
new ImageData(
blurredImageData.data,
blurredImageData.width,
blurredImageData.height,
),
0,
0,
);
const blurredImageURL = canvas.toDataURL();
this.blurredImage = blurredImageURL;
};
this.imageBlurWorker.onerror = (error) => {
console.error(`Worker error: ${error.message}`);
};
},
methods: {
handleImageUpload(event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = (e) => {
const arrayBuffer = e.target.result;
const blob = new Blob([arrayBuffer], { type: file.type });
const img = new Image();
img.onload = () => {
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
const imageData = ctx.getImageData(0, 0, img.width, img.height);
this.imageBlurWorker.postMessage(imageData);
};
img.src = URL.createObjectURL(blob);
};
reader.readAsArrayBuffer(file);
}
},
},
beforeDestroy() {
this.imageBlurWorker.terminate();
},
};
</script>
Image before blurring:
Output of result of blurred image:
Clean up and terminate the worker
When the component is destroyed, or when you no longer need the worker, you should terminate it to free up resources:
worker.terminate();
This should be done in the beforeDestroy
lifecycle hook in Vue to ensure it happens when the component is destroyed.
Following this guide, you can offload intensive computations to a separate thread in your Vue.js applications, resulting in smoother, more responsive user interfaces.
Benefits of Using Web Workers in Vue.js
There are several benefits to using web workers.
Enhanced Performance and Scalability
When you delegate computational tasks to a Web Worker, the main thread remains free to handle other critical tasks. As a result, your application can accommodate more concurrent operations and scale more effectively, leading to a noticeable performance boost.
To illustrate, if your application includes complex data processing tasks or sophisticated algorithms that can create a noticeable delay, you can offload these tasks to a Web Worker. This approach ensures that these time-consuming tasks won’t block the main thread, leading to a more responsive application.
Improved User Experience
With Web Workers, the user interface remains highly responsive, leading to a better user experience. This is because the main thread, responsible for user interactions and UI rendering, remains unblocked and can immediately respond to user actions. Therefore, users won’t experience a frozen or unresponsive interface, even during heavy computations.
Asynchronous Operations
Web Workers thrive in handling tasks that are asynchronous or involve operations like fetching data, working with indexedDB, or crunching large datasets. They can handle such operations independently, sending the results back when ready. This allows Vue.js to continue executing other tasks, including updating the DOM and responding to user events.
Ultimately, using Web Workers in Vue.js provides considerable performance benefits, improves user experience, and allows for more efficient use of modern hardware. It represents a significant evolution in how we can build complex and highly responsive web applications.
Drawbacks and Limitations of Web Workers
Of course, there are some drawbacks as well.
Lack of DOM Access
Web Workers operate on their own thread and cannot access the DOM. This limitation stems from the potential conflicts that could arise from having multiple threads interact with the DOM simultaneously. While this design helps ensure thread safety, any DOM manipulation must happen on the main thread. For instance, you can’t manipulate Vue components directly from a Web Worker or use Vue’s reactivity system within a worker. This means you’ll have to send messages back to the main thread when you want to update the UI based on a computation done by the worker.
Limited Access to Web APIs
Web Workers don’t have access to all Web APIs that the main thread does. Besides the DOM, they can’t access APIs such as localStorage or the methods provided by window
object. This limits the tasks that can be offloaded to a Web Worker.
Complexity of Multithreading
While Web Workers offer the advantage of concurrent execution, they also introduce the complexities of multi-threaded programming into the web environment. Developers need to manage the synchronization between the main and every worker thread, handle potential race conditions, and deal with error handling in a distributed environment. Debugging can also be more challenging with Web Workers due to their isolated execution context.
While Web Workers can greatly improve the performance of a Vue.js application, these advantages come with their own challenges. A careful assessment of the tasks to be offloaded, the data to be processed, and the necessary synchronization can help you navigate these limitations and make the most out of Web Workers.
Conclusion
In this article, we explored Web Workers and their integration within Vue.js. We covered the intricacies of how JavaScript and Web Workers operate, showcasing a practical implementation of a Web Worker in a Vue.js component. In examining the benefits, we highlighted the potential performance enhancements and improved user experience. Despite acknowledging their limitations, including lack of DOM access and communication overhead, we concluded that the effective use of Web Workers can significantly improve the efficiency and interactivity of Vue.js applications.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.