Export Pandas DataFrame to CSV: Complete Guide to to_csv()
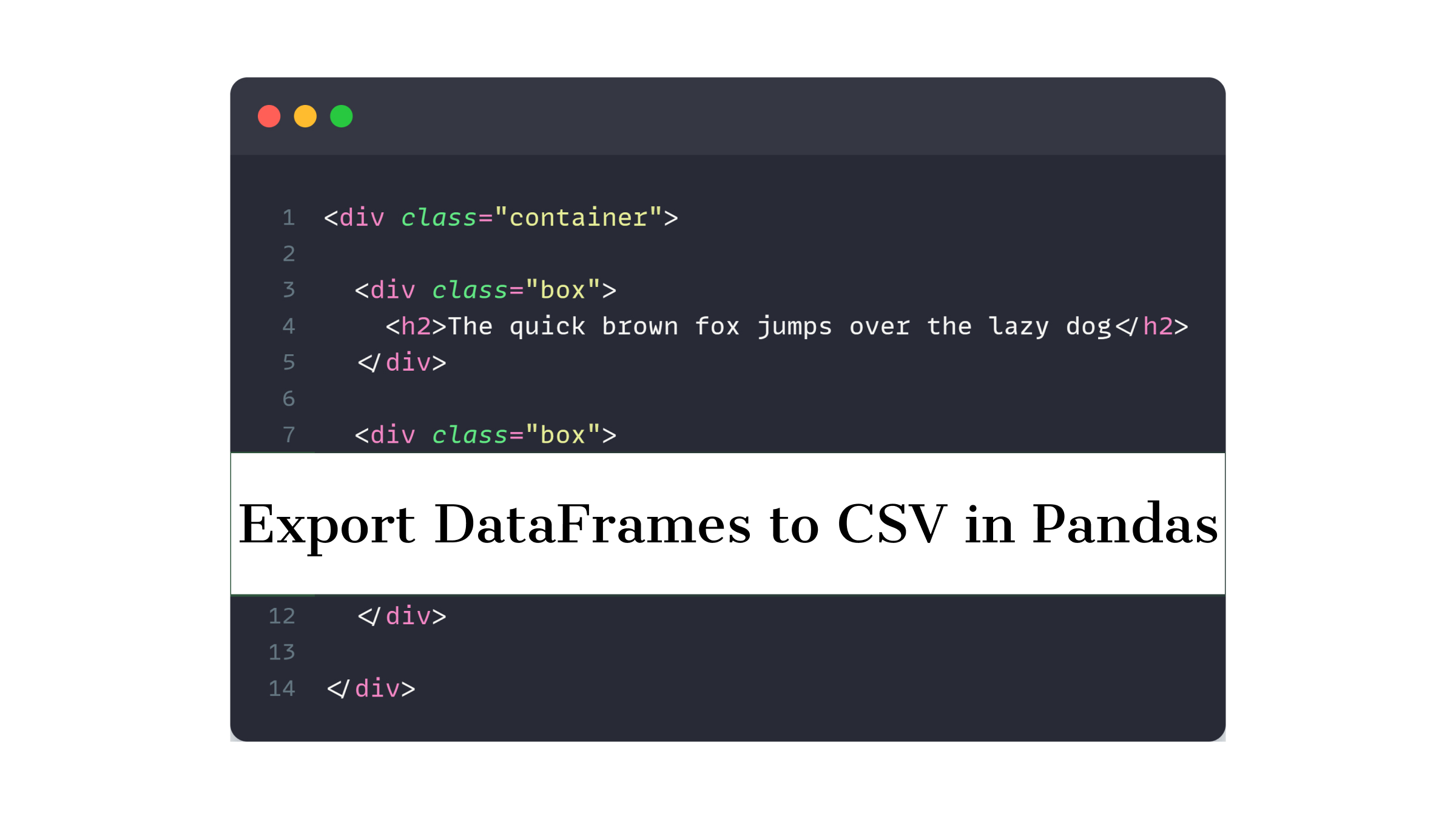
Are you trying to save a Pandas DataFrame as a CSV file? The to_csv()
method in Pandas makes it easy to export your data to a comma-separated values (CSV) file with just a few lines of code.
In this guide, we’ll walk through how to use to_csv()
to save a DataFrame to CSV, covering key parameters to customize the output. You’ll also see examples of common options like excluding the index, specifying the separator, handling missing data, and more.
Key Takeaways
- Use
df.to_csv('file.csv', index=False)
to save a DataFrame to CSV without the index. - Specify the separator with the
sep
parameter. Common options are comma,
and tab\t
. - Represent missing data with the
na_rep
parameter. - Save a subset of columns by passing a list to the
columns
parameter. - Format DateTime columns using
date_format
and strftime codes. - Export directly to compressed CSV files by providing a
compression
type.
How to Export a DataFrame to CSV with to_csv()
The basic syntax to save a Pandas DataFrame df
to a CSV file is:
df.to_csv('data.csv', index=False)
This will export the DataFrame to a file named data.csv
in the current directory. The index=False
parameter excludes the DataFrame’s index from being saved to the CSV.
Some other frequently used parameters of to_csv()
include:
sep
: String of length 1 specifying the field delimiter. Default is,
.na_rep
: String representation of missing data. Default is an empty string.header
: Write column names to CSV. Default isTrue
.encoding
: File encoding to use. Default is'utf-8'
.
We’ll explore these options further in the examples below.
Examples of Exporting DataFrames to CSV
Basic DataFrame to CSV Export
import pandas as pd
data = {'name': ['John', 'Anna', 'Peter', 'Linda'],
'age': [25, 31, 42, 28],
'city': ['New York', 'Paris', 'Berlin', 'London']}
df = pd.DataFrame(data)
df.to_csv('people.csv', index=False)
This saves the DataFrame to people.csv
with default options - comma separator and no index column.
Specify Separator and Missing Data Representation
df.to_csv('people.csv', sep='\t', na_rep='MISSING', index=False)
Uses tab (\t
) instead of comma as separator and represents missing data as 'MISSING'
string.
Save Subset of Columns to CSV
columns_to_save = ['name', 'age']
df.to_csv('people.csv', columns=columns_to_save, index=False)
Saves only the 'name'
and 'age'
columns to CSV.
Handling DateTime Columns
df['birth_date'] = pd.to_datetime(['1997-05-23', '1991-04-12',
'1980-09-01', '1994-11-18'])
df.to_csv('people.csv', date_format='%B %d, %Y', index=False)
Specifies a custom date format string for DateTime columns using date_format
parameter.
Compressed CSV Exports
Pandas allows saving directly to compressed CSV files by specifying a compression type:
# Save to gzip compressed CSV
df.to_csv('people.csv.gz', compression='gzip', index=False)
# Save to zip compressed CSV
df.to_csv('people.zip', compression='zip', index=False)
Supported compression types include 'gzip'
, 'bz2'
, 'zip'
, 'xz'
, and 'zstd'
.
FAQs
Specify the encoding with the `encoding` parameter:\n\n```python\ndf.to_csv('data.csv', encoding='utf-16')\n```
Yes, use `mode='a'` to append:\n\n```python\ndf.to_csv('data.csv', mode='a', header=False)\n```\n\nExclude the header to avoid repeating column names.
Use `sep=';'` for semicolon separator and `decimal=','` for comma decimal:\n\n```python\ndf.to_csv('data.csv', sep=';', decimal=',', index=False)\n```
Conclusion
With these options, Pandas’ to_csv()
provides a flexible way to export DataFrames to CSV files. By customizing parameters like separator, encoding, and compression, you can generate CSV files compatible with a variety of applications and locales.