Pure components in React: how they work and when to use them
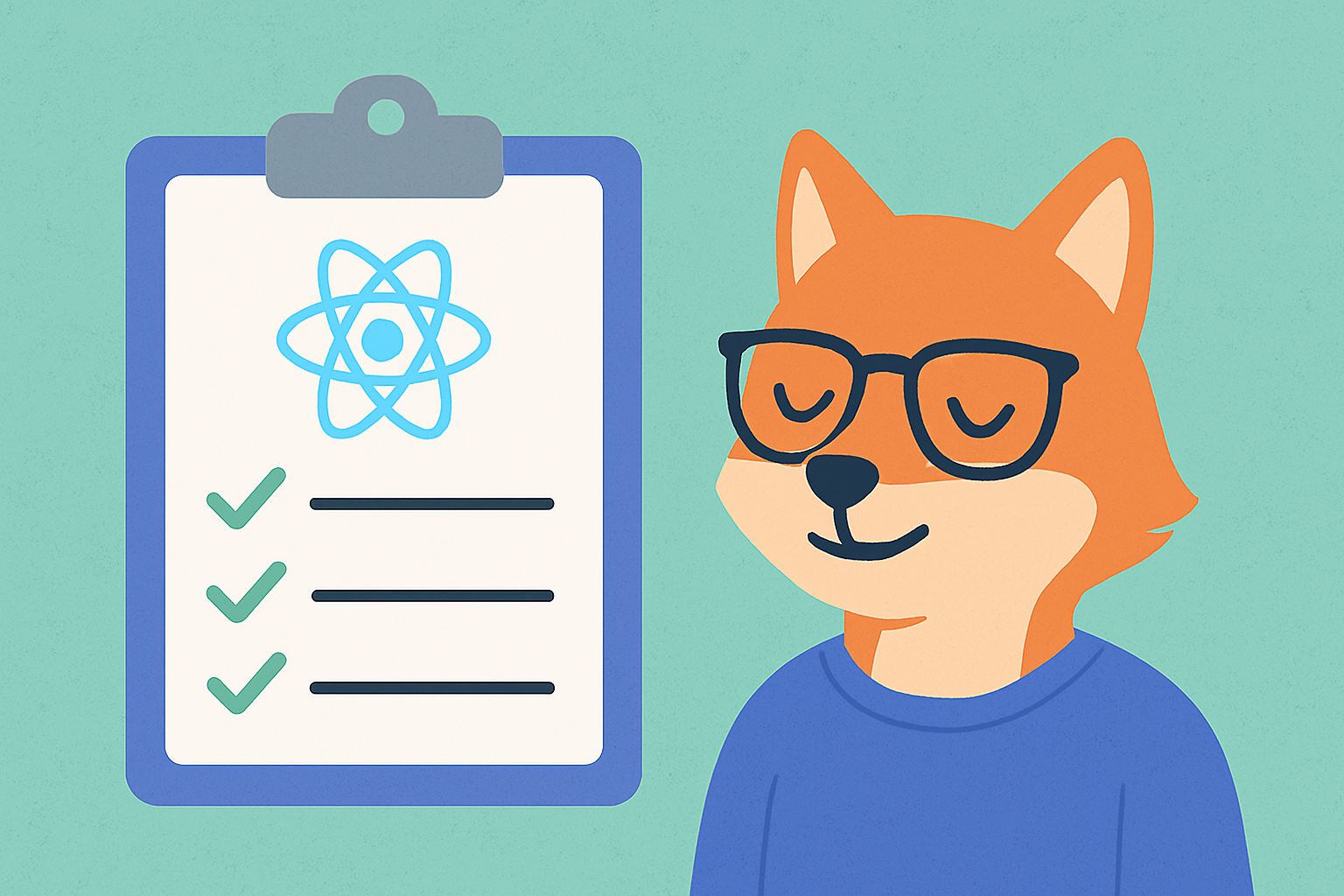
Pure components in React are all about performance. They help your application avoid unnecessary re-renders by checking whether the component’s inputs (props or state) have actually changed. In this article, we’ll look at how pure components work, how to use them, and when they’re useful — with clear, modern examples.
Key Takeaways
- Learn what makes a component “pure” in React
- See when to use
React.PureComponent
orReact.memo
- Understand shallow comparison and its impact on re-renders
What is a pure component?
A pure component is a component that only re-renders when its props or state have changed. React determines whether it should re-render by doing a shallow comparison of the current props/state with the previous ones.
Using React.PureComponent
React.PureComponent
is the class component equivalent of a pure function. It implements a built-in shouldComponentUpdate()
method that performs a shallow comparison.
class MyComponent extends React.PureComponent {
render() {
return <div>{this.props.text}</div>;
}
}
If this.props.text
hasn’t changed, the component won’t re-render.
Functional alternative: React.memo
For function components, use React.memo()
.
const MyComponent = React.memo(function MyComponent({ text }) {
return <div>{text}</div>;
});
React will skip rendering if text
is the same as in the previous render.
How shallow comparison works
A shallow comparison means:
- Primitive values (like numbers or strings) are compared by value
- Objects and arrays are compared by reference
{ a: 1 } === { a: 1 } // false
const obj = { a: 1 }
obj === obj // true
So, if you’re passing an object that gets mutated instead of recreated, a pure component might not notice the change.
Real-world example
Without optimization
const Item = ({ data }) => <div>{data.label}</div>;
This will re-render whenever the parent re-renders, even if data
hasn’t changed.
With React.memo
const Item = React.memo(({ data }) => <div>{data.label}</div>);
Now the component only re-renders when data
is a new object.
When to use PureComponent or memo
- You’re rendering lists with many items
- The component’s props stay stable between renders
- You want to reduce render cycles in performance-sensitive parts of your app
- You’re using
useCallback
oruseMemo
to preserve references
Common gotchas
- Shallow comparison doesn’t detect deep changes (nested objects/arrays)
- Don’t use it unless you’re confident props don’t mutate silently
- Can introduce bugs if you pass unstable references
React.memo
vs React.PureComponent
Feature React.PureComponent
React.memo
Component type Class component Function component Comparison logic Props + state (shallow) Props only (shallow) Custom compare fn ❌ ✅ React.memo(fn)
Modern usage Less common Widely used
Conclusion
Pure components can help React skip re-renders and keep your UI performant — but they’re not a silver bullet. Prefer React.memo
for functional components, and understand that shallow comparison means references matter. Use it when performance matters and props are stable.
FAQs
It prevents unnecessary re-renders when props/state haven’t changed (using shallow comparison).
Use it when your function component receives stable props and re-rendering would be wasteful.
No. It uses shallow comparison. You should avoid mutating objects or arrays in place.