Python Check if File Exists: Guide with Examples
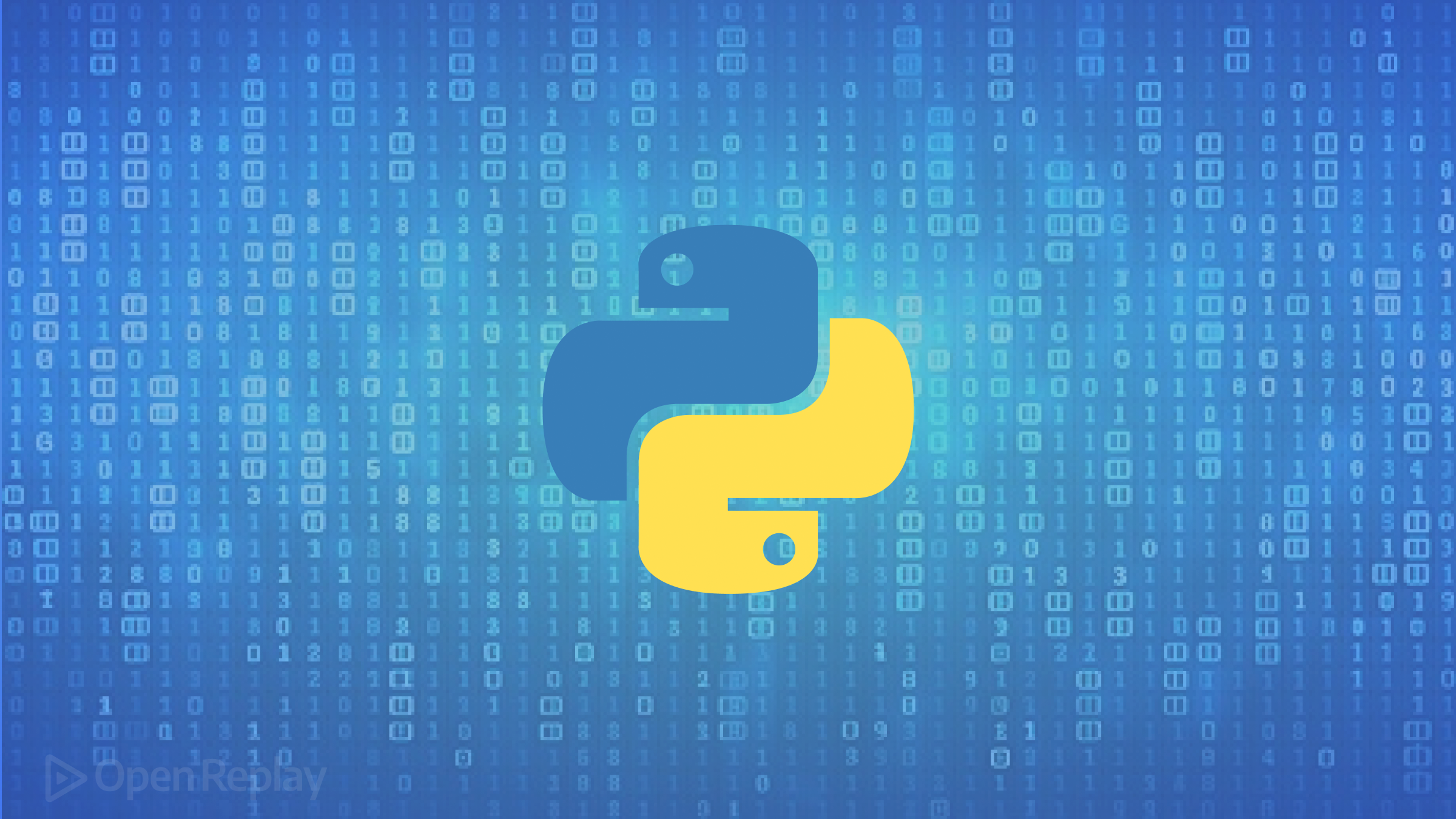
Checking file existence is a crucial step before performing file operations in Python. It helps prevent errors and ensures your code runs smoothly. Python provides built-in modules like os.path
and pathlib
to check if a file exists. This article will guide you through various methods and best practices for checking file existence in Python.
Key Takeaways
- Use
os.path.isfile()
orpathlib.Path.is_file()
to check if a file exists in Python. - Handle exceptions using
try-except
blocks when working with files. - Consider race conditions and use appropriate techniques like file locking or atomic operations.
- Use
os.path.join()
to create platform-independent file paths.
Understanding File Paths and the Current Directory
To check if a file exists, you need to understand file paths. File paths can be relative (based on the current directory) or absolute (starting from the root directory). You can use os.getcwd()
to get the current working directory and os.listdir()
to list files and folders in a directory. Understanding file paths is essential for accurately checking file existence.
Using os.path to Check if a File Exists
The os.path
module provides functions for working with file paths. To check if a file exists, you can use os.path.isfile(path)
. It takes the file path as a parameter and returns True
if the file exists, otherwise False
. Here’s an example:
import os
file_path = "example.txt"
if os.path.isfile(file_path):
print(f"{file_path} exists.")
else:
print(f"{file_path} does not exist.")
You can also use os.path.exists(path)
to check if a file or directory exists. It returns True
if the path exists, regardless of whether it’s a file or directory.
Checking File Existence with pathlib
Starting from Python 3.4, the pathlib
module provides an object-oriented approach to working with file paths. To check if a file exists using pathlib
, you can create a Path
object and use the is_file()
method. Here’s an example:
from pathlib import Path
file_path = Path("example.txt")
if file_path.is_file():
print(f"{file_path} exists.")
else:
print(f"{file_path} does not exist.")
The pathlib
module offers a more intuitive and expressive way to handle file paths compared to os.path
.
Exception Handling and Best Practices
When working with files, it’s important to handle exceptions gracefully. Use try-except
blocks to catch and handle FileNotFoundError
exceptions. The EAFP (Easier to Ask for Forgiveness than Permission) approach is often preferred over LBYL (Look Before You Leap) when checking file existence. Here’s an example:
try:
with open("example.txt", "r") as file:
# Perform file operations
except FileNotFoundError:
print("File not found.")
It’s also a good practice to use absolute paths or relative paths based on a known reference point to ensure reliability and portability of your code.
Handling Race Conditions and Concurrency
Race conditions can occur when multiple processes or threads access the same file simultaneously. Checking file existence before performing operations does not guarantee exclusive access to the file. To handle race conditions, you can use file locking mechanisms or atomic operations provided by libraries like fcntl
or portalocker
. Here’s an example using portalocker
:
import portalocker
with portalocker.Lock("example.txt", timeout=1) as file:
# Perform file operations
Cross-Platform Considerations
When working with file paths, keep in mind the differences between operating systems. Windows uses backslashes (\
) as path separators, while Unix-based systems use forward slashes (/
). To create platform-independent file paths, you can use os.path.join()
. Here’s an example:
import os
file_path = os.path.join("data", "example.txt")
Additionally, be aware of symbolic links and handle them appropriately across different platforms.
FAQs
`os.path.isfile()` checks if the path is an existing regular file, while `os.path.exists()` checks if the path exists, regardless of whether it's a file or directory.
You can use `os.path.isdir()` or `pathlib.Path.is_dir()` to check if a directory exists.
Conclusion
Checking file existence is an essential skill for any Python developer working with files. By understanding file paths, using the appropriate methods from os.path
or pathlib
, and following best practices for exception handling and cross-platform compatibility, you can write more reliable and secure code. Remember to handle race conditions when necessary and use appropriate techniques for concurrent file access. With the knowledge gained from this article, you’re well-equipped to handle file existence checks in your Python projects.