React 18 - What's New and How it Will Benefit Developers
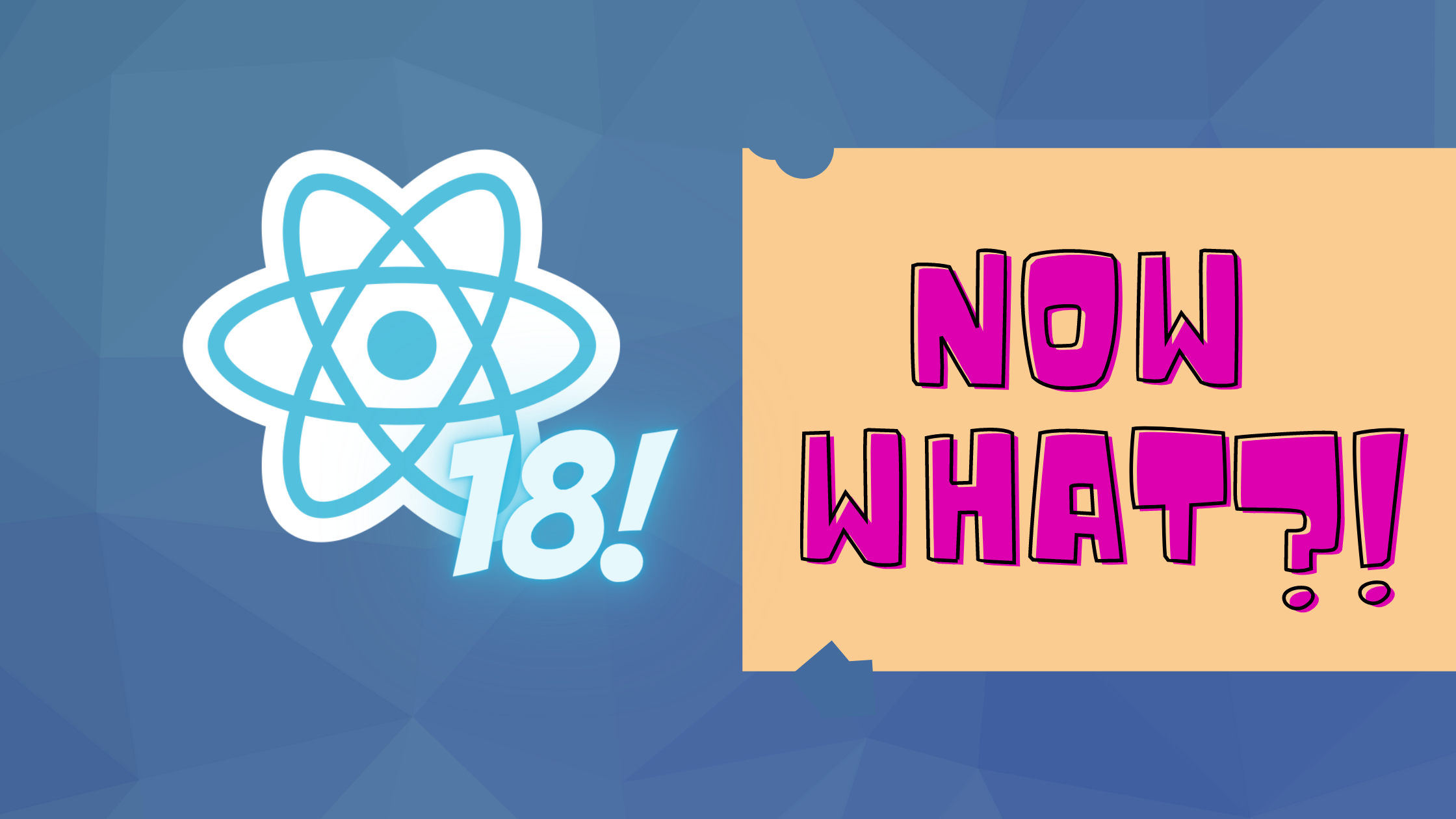
React is a JavaScript library for creating user interfaces. It provides a simple way to build components and manage data flow through your application. It focuses on performance and efficiency with fast component rendering and lower memory usage. The versions prior to React18 focused on the imperative approach, where you would mutate data directly. That led to common issues, such as slow browser updates.
React18 is the latest iteration of the framework, and it is geared at reducing the amount of code you need to write. In this release, it is moving to a functional paradigm where data is immutable. This will require some changes for developers but it should improve the performance and functionality of your applications. It is meant to be incremental and backward compatible, so it should be easy to adopt.
What’s new in React18?
Image optimization and picture element support
Since images make up for most downloaded content on the internet, they play a significant role in how fast web pages load. React17.5 introduced an API that enables you to create dynamic image sizes on the fly. React18 takes it a step further by allowing you to create multiple sizes depending on where the image will be displayed to achieve an optimal load time.
It is now possible for developers to use the picture or source elements when defining srcset. This allows you to specify images that are more appropriate for different screen resolutions.
Cache busting with file revision IDs
Developers can easily reference any cached files by providing the file revision ID, which helps avoid unnecessary downloads of old assets even if they have been changed remotely. The changes are also detected faster, so you won’t have to wait until your browser starts downloading them before seeing recent updates made in content delivery networks (CDNs).
Server-side rendering for Google Chrome
Server-side rendering allows it to render the initial state on the server and then pass that state to the client. This means that the initial state is available instantly, and you don’t have to wait for all of the components to render before displaying data.
Fixed headers
React18 lets us easily set header position as fixed, enabling maximum performance when using scrolling areas such as sticky footers or infinite scroll. Fixed headers use the document’s viewport width and can even be used on touch devices which was previously quite difficult to achieve properly with React.
Component picker
A new component picker was added in React17 that has been made more flexible and functional in React18 through several new features. These features include custom tag names, ordering via drag and drop, highlighted newly added components, custom styles for the selector, etc.
Fiber
A new virtual DOM implementation named fiber was introduced, which can lessen the amount of work needed to produce predictable changes to the application’s state. Fiber also enables server-side rendering with minimal effort even in very dynamic UIs because it works by tracking mutation operations that cause elements to re-render so they can be moved from a previous location when necessary. The result is an ability to render large amounts of data quickly without any loss of responsiveness. This mechanism will be convenient when you need multiple templates or fragments on a single page containing hundreds or thousands of items.
New Hooks API
There are newly introduced hooks, namely useLayoutEffect, useCallback, useMemo, and useImperativeHandle. The first one enables developers to manage the logic necessary for laying out each component. In contrast, the latter four are combined into one hook named effect, designed to replace imperative lifecycle methods.
UseLayoutEffect
The new API allows us to abstract away all the rendering logic related to re-rendering whenever a change occurs in your UI. Such changes might happen when responding to scroll events or animations, window resizing, or any other type of state change. When using this API, you can pass along an object whose current state contains everything needed for it to work.
UseCallback
UseCallback differs from useEffect in that it can be implemented without a class. All you need to do is call setState, which will trigger the logic for re-rendering. UseImperativeHandle: The new API returns a component instead of an opaque, hard to test function because it allows us to see what happened inside of your UI so you can debug any issues more efficiently and optimize performance when necessary.
Memoization
You no longer have to implement memorization. You need to add the memoized keyword where required and then wrap your functions using AutoSuspense (more on that in a later section). To help with the implementation, Hooks also gives us two methods called useMemo and memoized.
Using createRef()
createRef is yet another component you can use for tracking elements in the DOM. By using createRef, you can easily pass data between components and prevent any issues that may arise due to component re-rendering.
React.createClass()
The createClass function was eliminated in favor of the streamlined hooks API. This was because it is unnecessary for any imperative class methods and therefore ends up creating unnecessary barriers to entry.
React.AutoSuspense
React now supports async rendering by default, which is excellent for performance. However, the problem is that the effects are not visible to anyone working on the application because they are hard to debug. Fortunately, it is now possible to use the AutoSuspense component, which will display the effects while debugging, making it easier to optimize performance.
How will React18 benefit developers?
On top of all the new features mentioned above, here are features (some of which we already discussed above) that will make the development process much more manageable.
UseLayoutEffect
This feature will significantly improve performance when using dynamic, large datasets, and you can use it in components with multiple children to optimize rendering times. It’s also beneficial in cases where you need to implement dynamic behavior that requires external data or a callback to a server.
UseCallback
It’s useful when you need to perform imperative operations in your UI because it eliminates the need for having a class component to add a callback to this. In practice, React uses useCallback in its codebase and recommends that any other callbacks passing through be wrapped with the same method to avoid unnecessary re-renders.
Effect
The effects hook is an alternative for implementing stateful logic using lifecycle methods that have been designed for direct use without a class or higher-order components (HOCs). It supports error boundaries, but these are provided by React itself instead of from the effect.
Memoization
Instead of implementing memoization on your own, it provides this feature for us but with conditions that the function is only safe to use in effects and is not allowed to refer to anything outside its scope (outside the component itself). This feature will serve developers well when using hooks because it can speed up performance without drastic changes.
Using createRef()
createRef is one of the most convenient and flexible approaches because you can pass along any value you like. You can even improve your app’s design by passing data from one component to another, and you don’t have to worry about how the data will be used because props can be anything.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
What’s next?
The development team behind React also announced Hooks as alpha version. There are likely still some changes to come before reaching a stable status. While you can try the new features now, you shouldn’t adopt the above features in production until React18 reaches stable status.
While upgrading from React17.5 to React18 is a straightforward and backward compatible process, React18 is not backward compatible with React16. To upgrade from 16 to 18, you must make some changes, such as updating your build tooling, adding new Babel plugins, and updating how you work with Hooks.
Upgrading to React18 will not be overly complicated because you can either remove Hooks from your production bundles or leave them as they are. In this case, all you have to do is replace the imports from a React.AutoSuspense to a Suspense and change your hooks from autorun to runInBatchMode.
Conclusion
React18 promises greater performance while improving the development workflow. One of the most prominent features that React18 offers is the ability to have server-side rendering with minimal effort, even in highly dynamic UIs. This means that your pages will be able to load and refresh faster, which can significantly reduce the bounce rate. It also comes with the new Hooks API, which will allow us to work more efficiently with asynchronous operations because it will handle all of the necessary DOM manipulation.
While React18 looks promising, I highly advise not to use it in production until it reaches a stable status but feel free to play with it and start preparing your tooling to support it.