React 19 Server Components: What’s Changed and Why It Matters
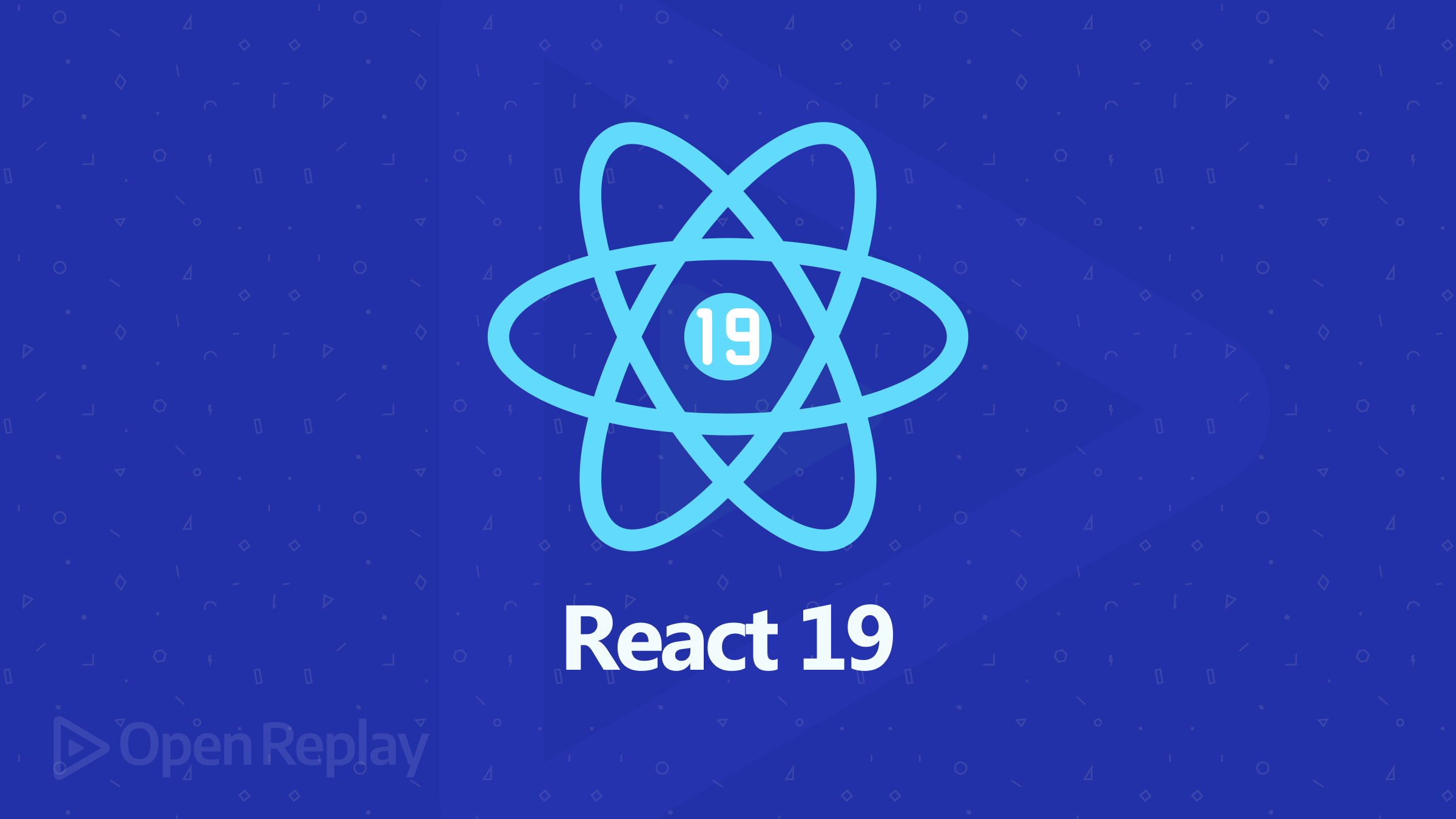
Struggling with inconsistent hydration or clunky data fetching in React Server Components? React 19 introduces significant updates to streamline server-side logic while maintaining seamless client interactivity. This article breaks down exactly what’s changed, how it impacts your workflow, and what you need to know to adopt these improvements effectively.
Key Takeaways
- Simplified APIs for defining and integrating Server Components
- Improved streaming and hydration performance
- Better alignment with frameworks like Next.js
- Reduced boilerplate for data fetching and state management
React 19 Server Components: Key Updates
1. Streamlined Server Component APIs
React 19 removes the need for separate ""use server""
directives in files containing Server Components. Now, components are automatically treated as server-rendered if they’re imported into a server entry point. This reduces boilerplate and clarifies component boundaries:
// Before React 19
'use server';
export function SearchBar() { /* ... */ }
// React 19
export function SearchBar() { /* ... */ } // Automatically server-rendered
2. Unified Hydration for Hybrid Components
The new hydrateRoot API merges server-rendered markup with client-side interactivity more reliably. It handles edge cases like mismatched props or missing client modules gracefully, reducing layout shift and hydration errors.
3. Built-In Streaming with Suspense
React 19’s native streaming support works without framework-specific configurations:
<Suspense fallback={<Loader />}>
<AsyncServerComponent />
</Suspense>
This allows progressive rendering of server-rendered content while JavaScript loads.
4. Simplified Data Fetching Patterns
The react-server module introduces fetchData, a replacement for getServerSideProps-style patterns:
async function ProductPage({ id }) {
const product = await fetchData(`/api/products/${id}`);
return <ProductDetails data={product} />;
}
Results are automatically cached and revalidated using React’s built-in mechanisms.
FAQs
No – React 19 provides native Server Component support, but frameworks like Next.js offer enhanced tooling for routing and caching.
Server Components pass serialized data to Client Components via props, but cannot access useState or useEffect directly.
No – React 19 enforces file-level separation: files containing Client Components must have a 'use client' directive.
React 19 throws clear build errors when server code references client-only APIs like localStorage.
Conclusion
React 19’s Server Component updates resolve critical pain points around hydration mismatches and data management while maintaining backward compatibility. By adopting these changes incrementally and leveraging the simplified APIs, teams can reduce client-side bundle sizes while improving time-to-interactive metrics.